회원 가입 페이지
1) index.html
<h1>회원 가입(JSP 버전)</h1>
<form action="/JSPProject1/signIn" method="post">
<table>
<tr>
<th>아이디</th>
<td><input type="text" name="inputId"></td>
</tr>
<tr>
<th>비밀번호</th>
<td><input type="password" name="inputPw"></td>
</tr>
<tr>
<th>비밀번호 확인</th>
<td><input type="password" name="inputPw"></td>
</tr>
<tr>
<th>이름</th>
<td><input type="text" name="inputName"></td>
</tr>
<tr>
<th>이메일</th>
<td><input type="email" name="inputEmail"></td>
</tr>
<tr>
<th rowspan="2">취미</th>
<td>
공부 <input type="checkbox" name="hobby" value="공부">
게임 <input type="checkbox" name="hobby" value="게임">
헬스 <input type="checkbox" name="hobby" value="헬스">
</td>
</tr>
<tr>
<td>
독서 <input type="checkbox" name="hobby" value="독서">
영화 <input type="checkbox" name="hobby" value="영화">
코딩 <input type="checkbox" name="hobby" value="코딩">
</td>
</tr>
<tr>
<td colspan="2" style="text-align: center;">
<button>회원 가입</button>
</td>
</tr>
</table>
</form>
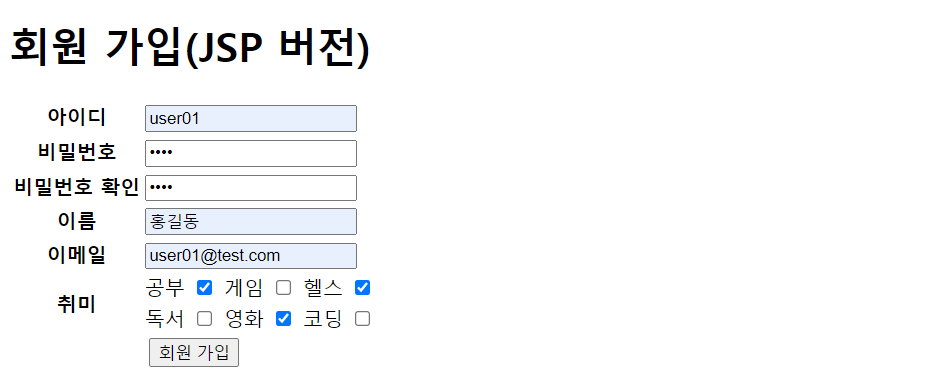
2) Servlet
@WebServlet("/signIn")
public class SignIn extends HttpServlet {
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
req.setCharacterEncoding("UTF-8");
RequestDispatcher dispatcher =
req.getRequestDispatcher("/WEB-INF/views/signIn.jsp");
dispatcher.forward(req, resp);
}
}
3) JSP
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" %>
<%
String id = request.getParameter("inputId");
String pw[] = request.getParameterValues("inputPw");
String name = request.getParameter("inputName");
String email = request.getParameter("inputEmail");
String hobby[] = request.getParameterValues("hobby");
%>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>회원가입 결과</title>
</head>
<body>
<%
String hobbyResult = "";
for(String temp : hobby){
hobbyResult += temp + " ";
}
%>
<% if(!pw[0].equals(pw[1])){ %>
<h3>비밀번호가 일치하지 않습니다</h3>
<% } else{ %>
<ul>
<li>아이디 : <%= id %></li>
<li>비밀전호 : <%= pw[0] %></li>
<li>이름 : <%= name %></li>
<li>이메일 : <%= email %></li>
<li>취미 : <%= hobbyResult %></li>
</ul>
<h3><%= name %>님의 회원 가입이 완료되었습니다.</h3>
<% } %>
</body>
</html>
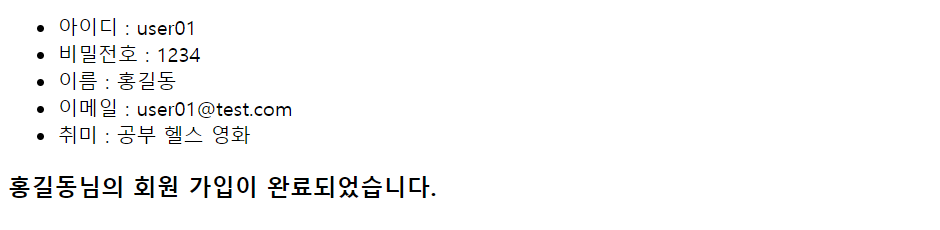