< 입출력 >
1. 입출력(IO)
1) 입출력
- input과 output의 약자. 컴퓨터 내부 또는 외부 장치와 프로그램 간의 데이터를 주고 받는 것
- 입출력 데이터를 처리할 공통적인 방법으로 스트림 이용
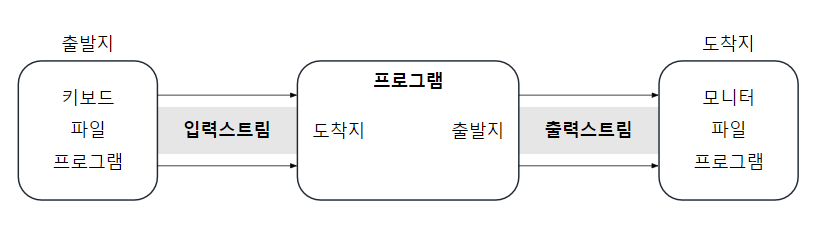
2) 스트림(Stream) 클래스
- 모든 스트림은 단방향이며 각각의 장치마다 연결할 수 있는 스트림 존재
- 단방향이기 때문에 입출력을 동시에 하려면 2개의 스트림이 필요

- 바이트 기반 입력 스트림의 최상위 클래스로 추상클래스이다
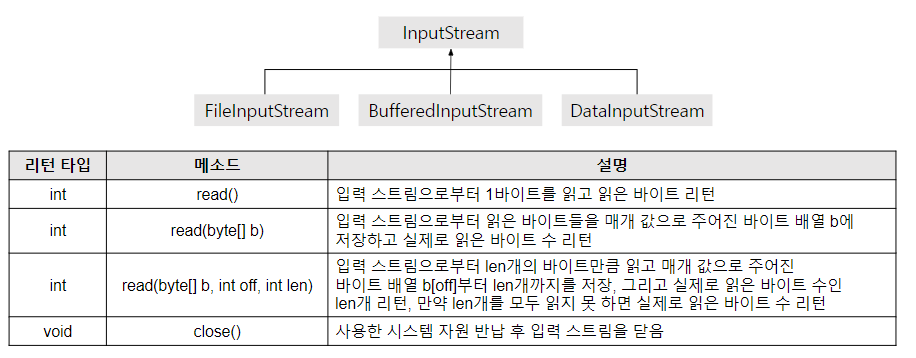
4) OutputStream
- 바이트 기반 출력 스트림의 최상위 클래스로 추상클래스이다
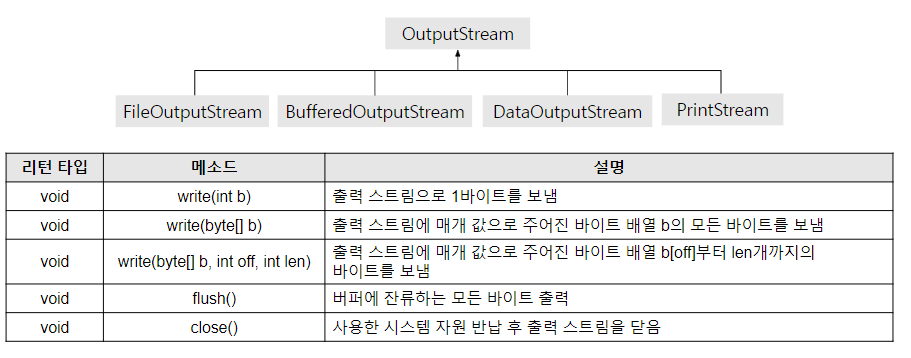
5) Reader
- 문자 기반 입력 스트림의 최상위 클래스
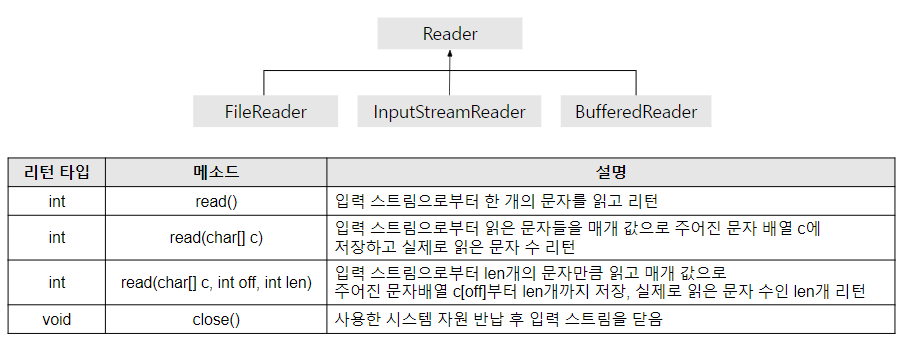
6) Writer
- 문자 기반 출력 스트림의 최상위 클래스
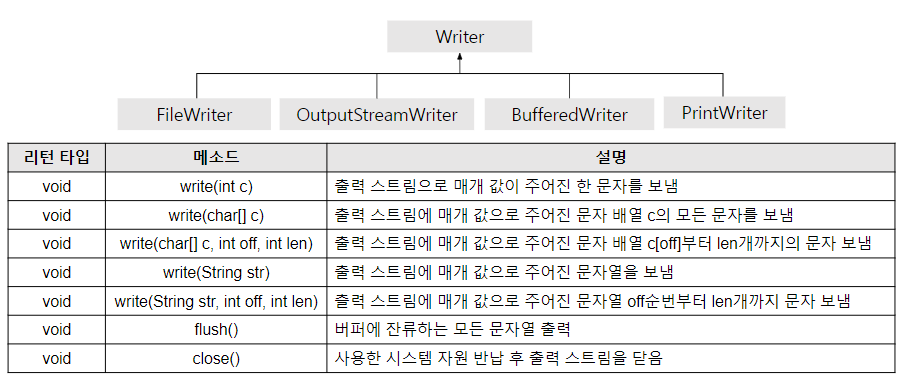
7) 사용예시
- 파일로 사용하려는 프로젝트에서 새로만들기 하여 General 폴더의 file을 만들어준다
< 바이트 기반 OutputStream >
-----------------------------------------------------------------
FileOutputStream fos = null;
try {
fos = new FileOutputStream("test1.txt");
String str = "Hello";
for( int i = 0; i < str.length(); i++){
fos.write(str.charAt(i));
}
} catch (IOException e){
e.printStackTrace();
} finally {
try{
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
< 바이트 기반 InputStream >
-----------------------------------------------------------------
FileInputStream fis = null;
try {
tis = new FileInputStream("test1.txt");
while(true){
int data = fis.read();
if( data == -1 ) break;
System.out.print( (char)data );
}
} catch ( IOException e ) {
e.printStackTrace();
} finally {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
< 문자 기반 OutputStream >
-----------------------------------------------------------------
FileWriter fw = null;
try {
fw = new FileWriter("test1.txt");
String str = "안녕하세요. Hello. 1234. !#";
fw.write(str);
} catch ( IOException e ) {
e.printStackTrace();
} finally {
try {
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
< 문자 기반 InputStream >
-----------------------------------------------------------------
FileReader fr = null;
try {
fr = new FileReader("test1.txt");
while(true){
int data = fr.read();
if( data == -1 ) break;
System.out.print( (char)data );
}
} catch ( IOException e ) {
e.printStackTrace();
} finally {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}