1. 클래스
- 클래스 (붕어빵 만드는 틀), 인스턴스 (붕어빵)
- 클래스: 표현하고자 하는 대상의 공통 속성을 한 군데에 정의해 놓은 것 (객체의 속성을 정의)
- 멤버 변수: 클래스 내부 정보 (모델, 색깔, 가격)
- 인스턴스: 클래스로 부터 만들어진 객체 (갤럭시, 아이폰)
class Phone {
String model;
String color;
int price;
}
public class Main {
public static void main(String[] args) {
Phone galaxy = new Phone();
galaxy.model = "Galaxy10";
galaxy.color = "Black";
galaxy.price = 100;
Phone iphone =new Phone();
iphone.model = "iPhoneX";
iphone.color = "Black";
iphone.price = 200;
System.out.println("철수는 이번에 " + galaxy.model + galaxy.color + " + 색상을 " + galaxy.price + "만원에 샀다.");
System.out.println("영희는 이번에 " + iphone.model + iphone.color + " + 색상을 " + iphone.price + "만원에 샀다.");
}
}
- 메소드: 작업을 수행하는 코드를 하나로 묶어 놓은 것 (동사로 시작, camel case)
int[] heights = new int[5];
initHeight(heights);
sortHeight(heights);
printHeight(heights);
- 메소드 내 변수는 지역변수이르로 서로 다른 메소드라면 같은 이름의 지역변수 선언 가능
class Calculation {
int add(int x, int y) {
int result = x + y;
return result;
}
int subtract(int x, int y) {
int result = x - y;
return result;
}
}
public class Main {
public static void main(String[] args) {
Calculation calculation = new Calculation();
int addResult = calculation.add(100, 90);
int subResult = calculation.subtract(90, 70);
System.out.println("두 개를 더한 값은 " + addResult);
System.out.println("두 개를 뺀 값은 " + subResult);
}
}
- 생성자: 인스턴스가 생성될 때 사용되는 인스턴스 초기화 메소드
- 생성자의 이름은 클래스명과 같음
- 생성자는 리턴값이 없음
- 기본 생성자: 클래스에 생성자가 1개도 작성이 되어있지 않을 경우, 자바 컴파일러가 기본 생성자를 추가함
(매개변수와 내용이 없는 생성자)
- this는 생성된 객체 자신을 가리킴 (생성자의 매개변수 값을 객체의 해당하는 데이터에 넣어줌)
class Phone {
String model;
String color;
int price;
Phone(String model, String color, int price) {
this.model = model;
this.color = color;
this.price = price;
}
}
public class Main {
public static void main(String[] args) {
Phone galaxy = new Phone("Galaxy10", "Black", 100);
Phone iphone =new Phone("iPhoneX", "Black", 200);
System.out.println("철수는 이번에 " + galaxy.model + galaxy.color + " + 색상을 " + galaxy.price + "만원에 샀다.");
System.out.println("영희는 이번에 " + iphone.model + iphone.color + " + 색상을 " + iphone.price + "만원에 샀다.");
}
}
2. 상속
- 상속: 기존의 클래스를 재사용하는 방식
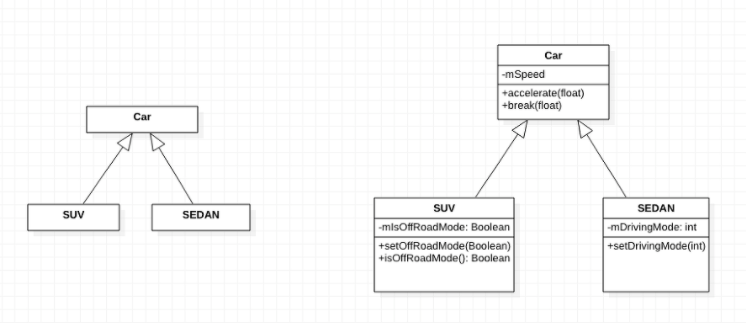
- 클래스간의 계층구조 형성
- 부모 클래스에서 정의된 필드와 메소드 물려 받음 (새로운 필드와 메소드 추가 가능)
- 부모 클래스에서 물려받은 메소드 수정 가능
- extends 이용
- super(): 부모 클래스로부터 상속받은 필드나 메소드를 자식 클래스에서 참조하여 사용하고 싶을 때 사용
- 오직 하나의 클래스만 상속 가능
- 자식 객체는 자식, 부모 타입으로 선언된 변수 할당 가능 / 부모 객체는 자식 타입으로 선언된 변수 할당 불가능
class Animal {
String name;
public void cry() {
System.out.println(name + " is crying.");
}
}
class Dog extends Animal {
Dog(String name) {
this.name = name;
}
public void swim() {
System.out.println(name + " is swimming!");
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog("코코");
dog.cry();
dog.swim();
Animal dog2 = dog;
dog2.cry();
}
}
- 오버로딩: 한 클래스 내에 동일한 이름의 메소드를 여러 개 정의하는 것
- 메소드 이름 동일
- 매개변수의 개수 또는 타입이 달라야함
int add(int x, int y, int z) {
int result = x + y + z;
return result;
}
long add(int a, int b, long c) {
long result = a + b + c;
return result;
}
int add(int a, int b) {
int result = a + b;
return result;
}
- 오버라이딩: 부모 클래스로부터 상속받은 메소드의 내용을 변경하는 것 (필요에 의해 변경할 경우 사용)
- 부모클래스 메소드 이름과 동일
- 부모클래스 메소드 매겨변수 동일
- 부모클래스 메소드 반환타입 동일
class Animal {
String name;
String color;
public void cry() {
System.out.println(name + " is crying.");
}
}
class Dog extends Animal {
Dog(String name) {
this.name = name;
}
public void cry() {
System.out.println(name + " is barking!");
}
}
public class Main {
public static void main(String[] args) {
Animal dog = new Dog("코코");
dog.cry();
}
}
- 오버로딩 : 기존에 없는 새로운 메소드를 정의하는 것
- 오버라이딩 : 상속받은 메소드의 내용을 변경하는 것