1. 프로젝트 설계하기
필요한 기능 확인하기
- 키워드로 상품 검색하고 그 결과를 목록으로 보여주기
- 관심 상품 등록하기
- 관심 상품 조회하기
- 관심 상품에 원하는 가격 등록하고, 그 가격보다 낮은 경우 표시하기
API 설계하기
3계층 설계하기
- Controller
- ProductRestController: 관심 상품 관련 컨트롤러
- SearchRequestController: 검색 관련 컨트롤러
- Service
- ProductService: 관심 상품 가격 변경
- Repository
- Product: 관심 상품 테이블
- ProductRepository: 관심 상품 조회, 저장
- ProductRequestDto: 관심 상품 등록하기
- ProductMypriceRequestDto: 관심 가격 변경하기
- ItemDto: 검색 결과 주고받기
2. 관심 상품 조회하기
- models 패키지 생성 (src > main > java > com.sparta.week04 > models)
Timestamped 클래스 생성하기
- 멤버 변수 선언 (생성일자, 수정일자)
public class Timestamped {
private LocalDateTime createdAt;
private LocalDateTime modifiedAt;
}
- 최초 생성 / 마지막 변경 시점 선언
- 변경 시 자동 기록
@EntityListeners(AuditingEntityListener.class)
public class Timestamped {
@CreatedDate
private LocalDateTime createdAt;
@LastModifiedDate
private LocalDateTime modifiedAt;
}
- 추상 클래스 설정 (abstract)
- 상속 받은 클래스의 멤버 변수 되도록 설정
- getter 메소드 자동 생성: 정보 받았을 때 조회하기 위해서 사용
@Getter
@MappedSuperclass
@EntityListeners(AuditingEntityListener.class)
public abstract class Timestamped {
@CreatedDate
private LocalDateTime createdAt;
@LastModifiedDate
private LocalDateTime modifiedAt;
}
Application 수정하기
- 시간 자동 변경 기능 설정: JPA Timpstamped 기능 사용하기 위해 사용
@EnableJpaAuditing
@SpringBootApplication
public class Week04Application {
public static void main(String[] args) {
SpringApplication.run(Week04Application.class, args);
}
}
Product 클래스 생성하기
- 멤버 변수 선언 (ID, 제목, 이미지, 링크, 최저가, 관심가격)
public class Product{
private Long id;
private String title;
private String image;
private String link;
private int lprice;
private int myprice;
}
- DB 테이블 설정
- DB 열 설정
- Timestamped 상속 설정 (extends)
@Entity
public class Product extends Timestamped{
@GeneratedValue(strategy = GenerationType.AUTO)
@Id
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String image;
@Column(nullable = false)
private String link;
@Column(nullable = false)
private int lprice;
@Column(nullable = false)
private int myprice;
}
- 기본 생성자 생성
- getter 메소드 자동 생성
@Getter
@NoArgsConstructor
@Entity
public class Product extends Timestamped{
@GeneratedValue(strategy = GenerationType.AUTO)
@Id
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String image;
@Column(nullable = false)
private String link;
@Column(nullable = false)
private int lprice;
@Column(nullable = false)
private int myprice;
}
ProductRepository 인터페이스 생성하기
- JpaRepository 기능 추가
public interface ProductRepository extends JpaRepository<Product, Long> {
}
ProductRestController 클래스 생성하기
- RestController 선언: CRUD 요청을 JSON 형시으로 자동 응답하기 위해 사용
@RestController
public class ProductRestController {
}
- GetMapping 설정 (/api/products)
- getProducts 메소드 생성
- productRepository 이용하여 찾는 기능 설정
@RestController
public class ProductRestController {
ProductRepository productRepository;
@GetMapping("/api/products")
public List<Product> getProducts() {
return productRepository.findAll();
}
}
- RestController에게 Repository가 꼭 필요한 것임을 알려서 자동으로 생성되도록 설정 (RequiredArgsConstructor, final 한쌍을 이룸)
@RequiredArgsConstructor
@RestController
public class ProductRestController {
private final ProductRepository productRepository;
@GetMapping("/api/products")
public List<Product> getProducts() {
return productRepository.findAll();
}
}
ARC 정상 동작 확인하기
- GET -> /api/products -> 상태: 200 확인
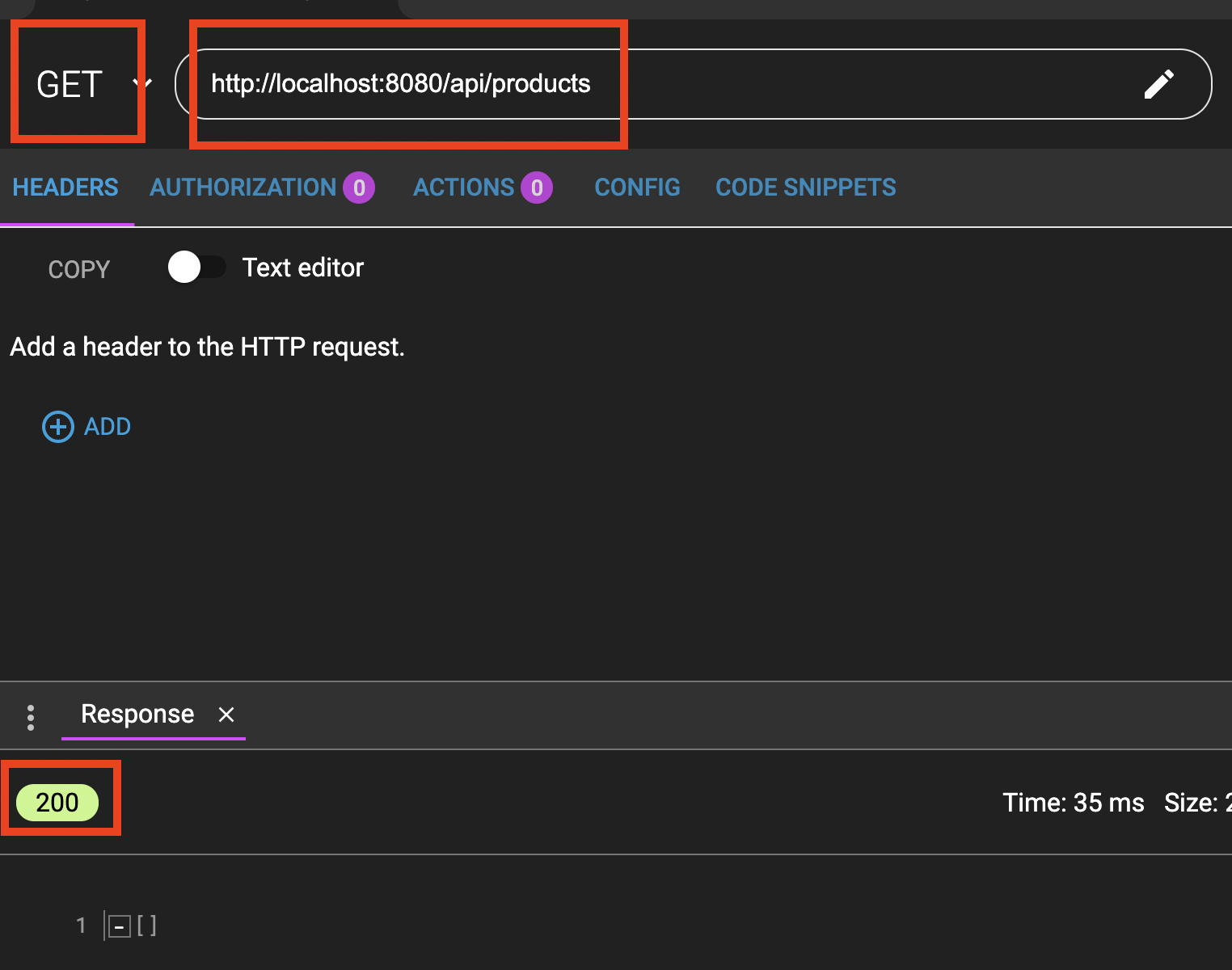