Control Section
- It is a part of program that maintains its identity after assembly; each such control section can be loaded and relocated independently of the others
- Different control sections are most often used for subroutines or other logical subdivisions of a program
- The programmer can assemble, load, and manipulate each of these control sections separately
- The resulting flexibility is a major benefit of using control sections
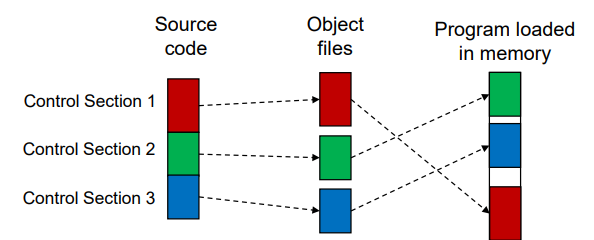
control section으로 나누지 않았다면 프로그램 일부를 수정하게 되면 전체 소스 코드를 어셈블 해줘야 한다. 하지만 control section을 나누면 수정한 control section만 어셈블 해주면 되서 이점이 된다.
Program Linking
- When control sections form logically related parts of a program, it is necessary to provide some means for linking them together.
- Instructions in one control section(CS) might need to refer to instructions or data located in another section.
- Such references between CSs are called “external references”.
- However, the assembler is unable to process these references because CSs are independently loaded and relocated.
- The assembler has no idea where any other CS will be located at execution time, so it just generates information for each external reference that will allow the loader to perform the required linking
Fig. 2.15 & 16
- Here, we describe how external references are handled by our
assembler.
- Figures 2.15 and 16 show an example program using 3 control sections: one for the main program and one for each subroutine
- CSECT: assembly directive to signal the start of a new CS
- EXTDEF: assembly directive to name symbols defined in a CS, used by other section
- Such symbols are called external symbols
- Control section names are automatically considered to be external symbols
- EXTREF: assembly directive to name symbols used in a CS, defined elsewhere
- The assembler establishes a separate LOC for each CS, just as it does for program blocks.
Fig. 2.16
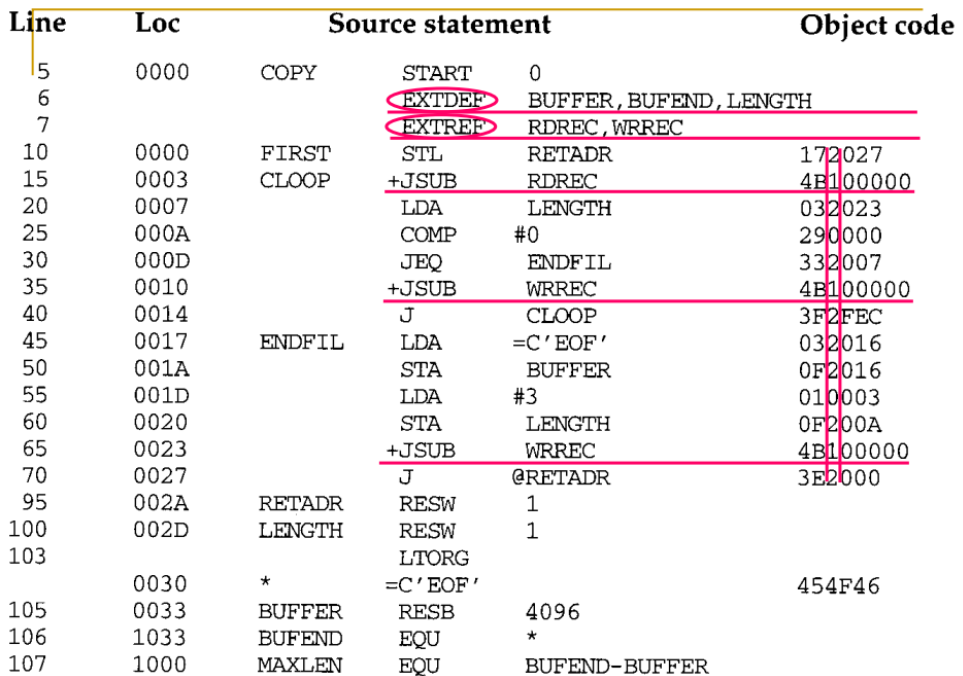
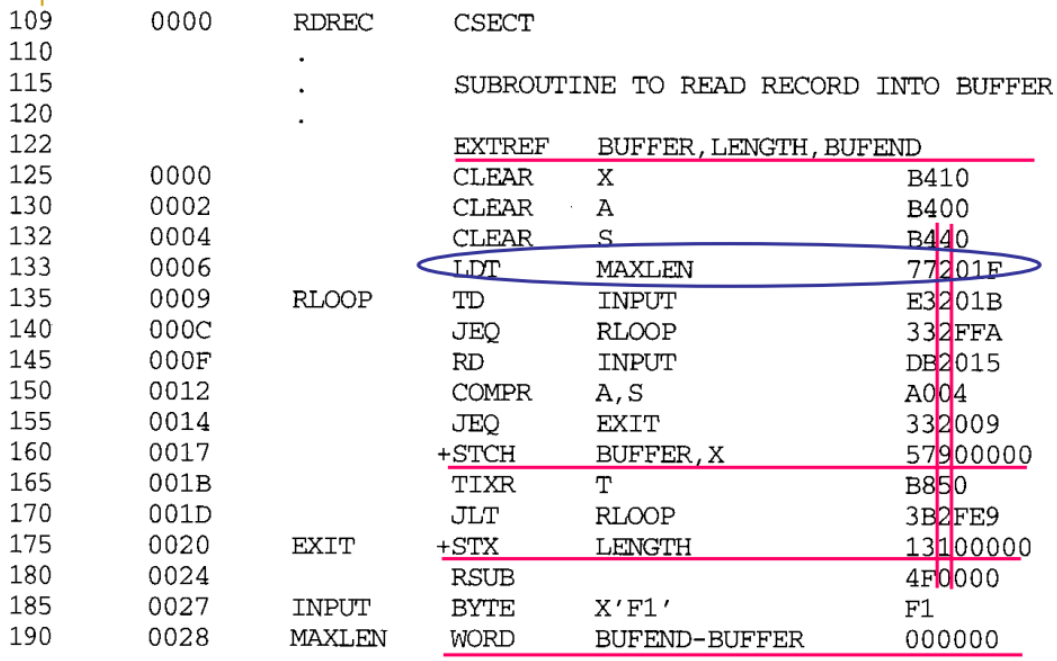
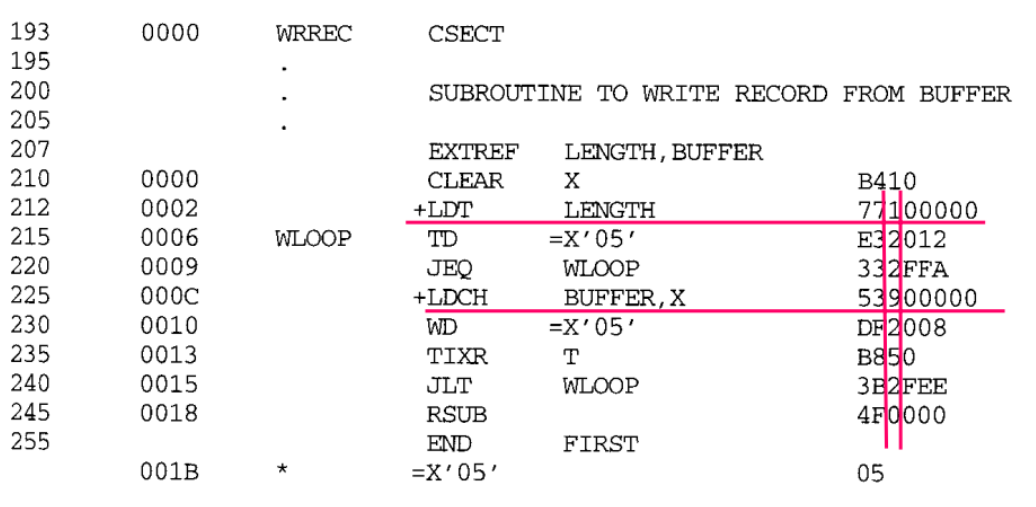
How to handle External References?
- The assembler has no idea where the CS containing an external reference (e.g., RDREC) will be loaded, so it cannot assemble the address for the reference.
- 어셈블러는 외부 레퍼런스(예: RDREC)를 포함하는 CS가 어디에 로딩될지 모르기 때문에 레퍼런스를 위한 어드레스를 어셈블러할 수 없습니다
- Instead, the assembler inserts an address of 0 and passes information to the loader, so the proper address can be inserted at load time.
- 대신 어셈블러는 0의 주소를 삽입하고 로더에 정보를 전달하므로 로드 시 적절한 주소를 삽입할 수 있습니다.
- [e.g.] CLOOP +JSUB RDREC 4B100000
- [e.g.] +STCH BUFFER,X 57900000
- An extended format instruction (i.e., format 4) must be used to provide room for the actual address to be inserted.
- Relative addressing (i.e., format 3) is not possible
New Record Types
- The assembler must include information in the object program that will cause the loader to insert the proper values where they are required.
- Define record:
- For external symbols defined in the control section by EXTDEF
- Indicates the relative address of each external symbol within this control section
- Refer record:
- For external symbols used in the control section by EXTREF
- No address information is available
Record Types
Fig. 2.17
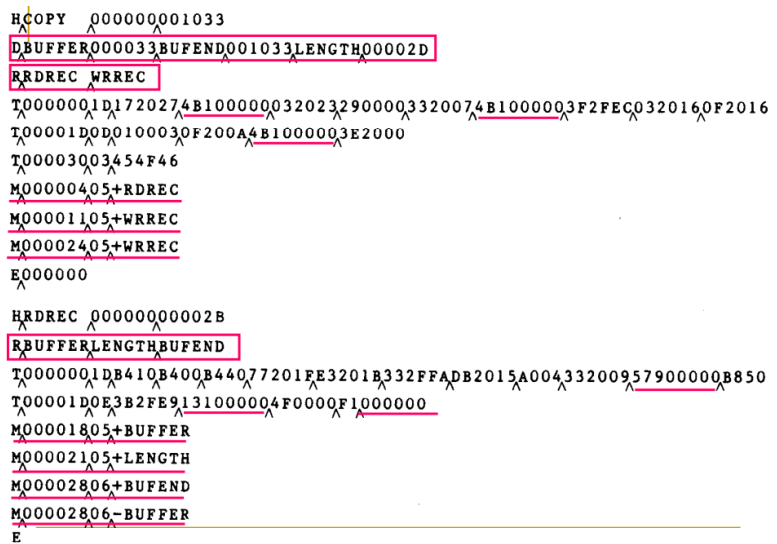
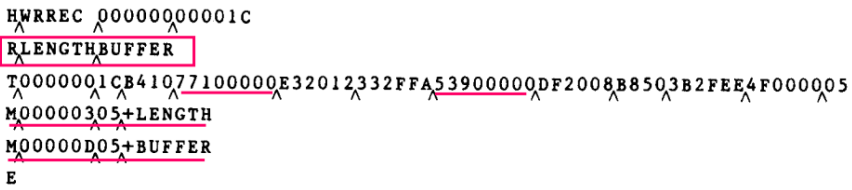
Control Section and Program Linking
- Loader
- For every external symbol
- Find the relative address from the define record
- Add the starting address of the control section where the symbol is defined
- Modify the field
- More details will be covered later!
과정 혹시 모르니 암기
Assembler Design Option
- 2-pass assembler scans source code twice!
- First, to produce a symbol table and secondly to produce object codes.
- Is this the only way to design an assembler?
- Of course, NOT… We can make it with the 1-pass algorithm or even a multipass algorithm!
- In this lecture, we will just study on the “one-pass assembler”, which scans the source code only one time (-> avoids the overhead of an additional pass over the source program) but often must patch the object code produced during this scan
One-Pass Assemblers
- Two different types
- Type I “Load-and-Go Assembler”: produces object code directly in memory for immediate execution, with no object program written out, and no loader needed.
- Type II: produces the usual kind of object program for later execution, so object program is produced, and loader is yet needed.
- The main problem in trying to assemble a program in one pass involves “forward references”
- Instruction operands often are symbols that have not yet been defined in the source program. Thus, the assembler does not know what address to be inserted into the translated instruction
아직 정의 되지 않은 symbol을 사용하는 것을 forward reference라고 한다.
Fig. 2.18
- Sample program for a one-pass assembler
- It has the same logic as in Fig. 2.2 for SIC
- All data item definitions are placed ahead of instructions that refer to them in order to eliminate forward references to the data items
- However, forward references to labels on instructions cannot be eliminated as easily.
- E.g., line 15, 30, 35, 65, and 155(외부에서 가져오는 값들)
- How can Type 1 & 2 one-pass assemblers address this issue?
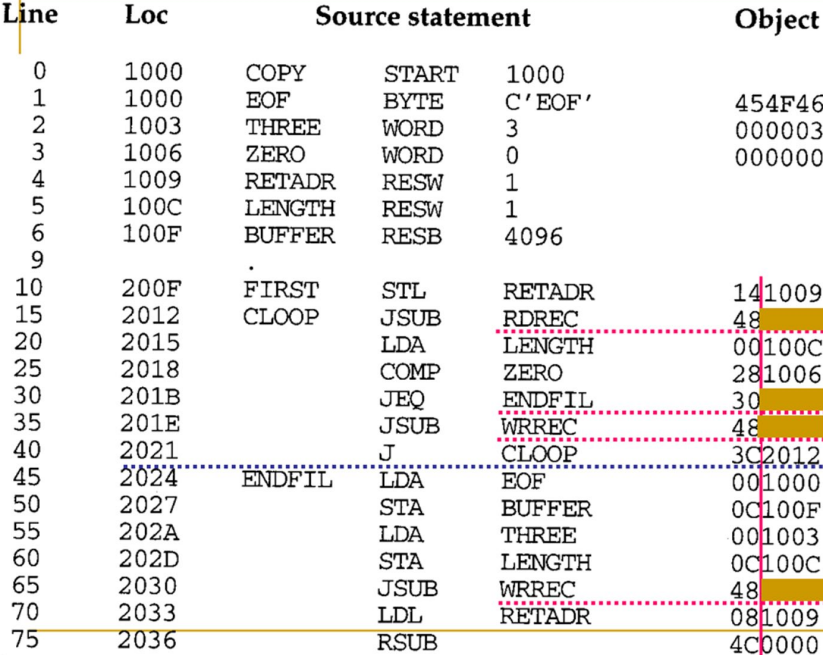
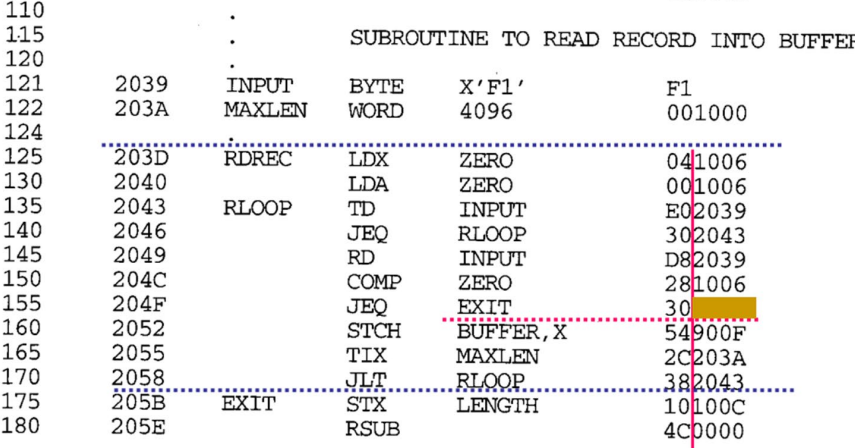
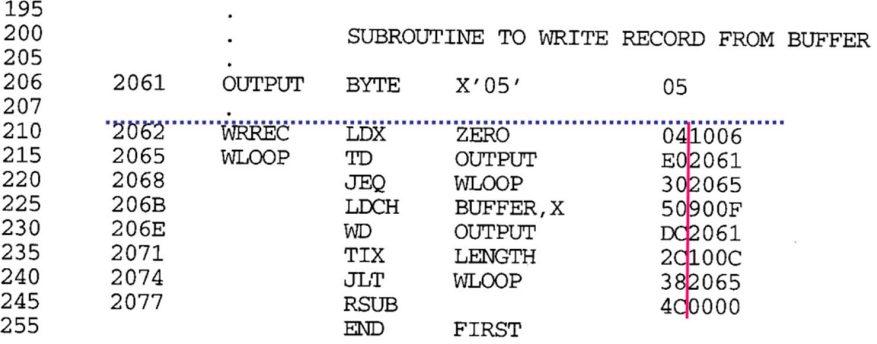
Type I One-Pass Assemblers: “Load-and-Go”
- Useful in a system that is oriented toward program development & testing.
- Programs are re-assembled nearly every time they are run.
- The load-and-go assembler produces the object code in memory rather than writing it out on secondary storage
- Efficiency of the assembly process ↑
- How to solve forward references?
- Store undefined symbols in the SYMTAB with the address of the field that references this symbol
- When the symbol is defined later, look up the SYMTAB and modify the field with correct address
Fig. 2.19(a)
- Object code in memory & symbol table entries for the program in Figure 2.18 after scanning line 40
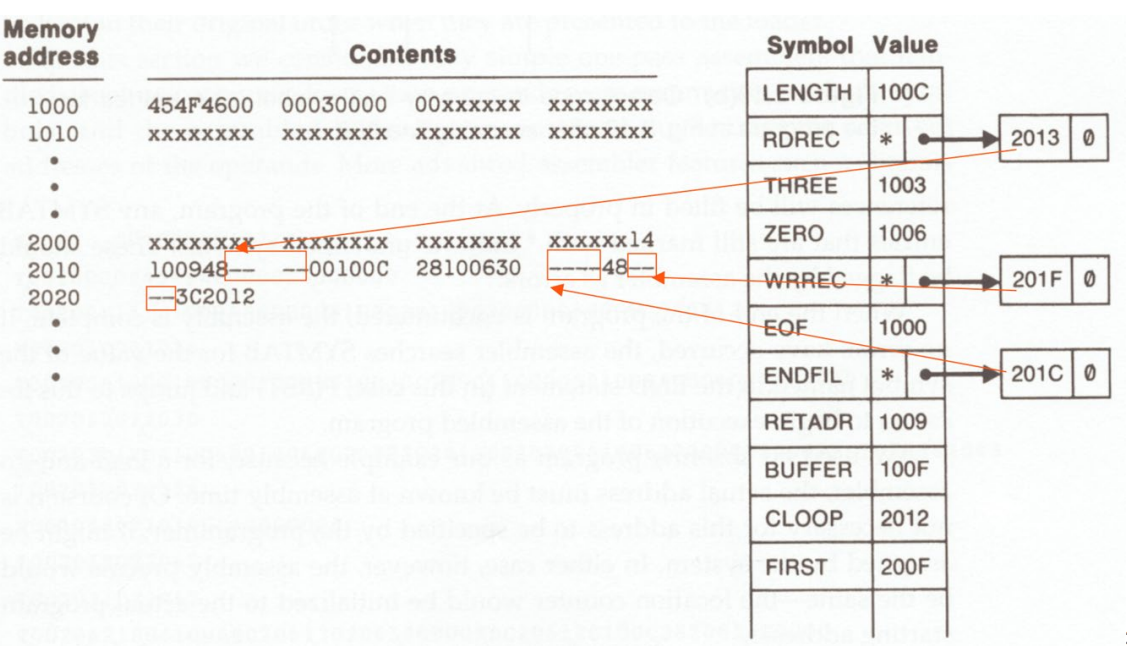
원래 주소값에서 1을 더해줬구나.. 시험때 유의해.
Fig. 2.19(b)
- Object code in memory & symbol table entries for the program in Figure 2.18 after scanning line 160
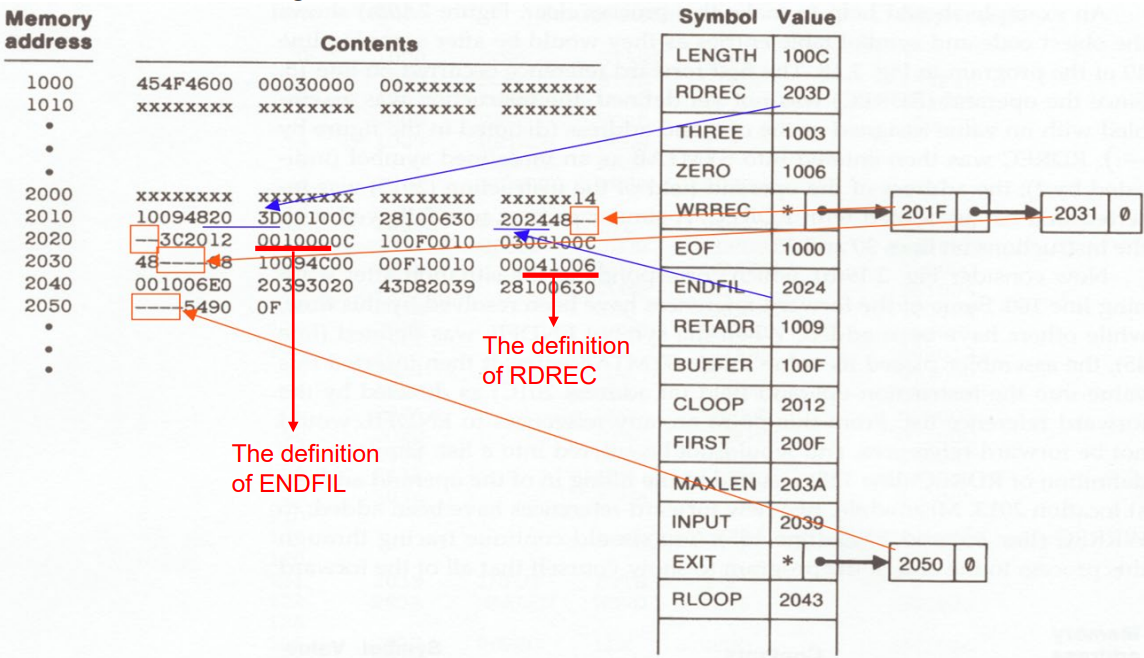
Type II One-Pass Assemblers
- Writes the object program on disk
- How to solve forward references?
- Forward references are entered into lists as before.
- When the definition of a symbol is encountered, generate another Text records with correct operand address
- When loaded, correct address will be inserted by loader
Fig. 2. 20
- Object program from one-pass assembler for program in Figure 2.18
- The 2nd Text record contains the object code generated from line 10 through 40
- When the definition of ENDFIL on line 45 is encountered, the assembler generates the 3rd Text record -> This specifies that the value 2024 (the address of ENDFIL) is to be loaded at location 201C (the operand address field of the JEQ on line 30)
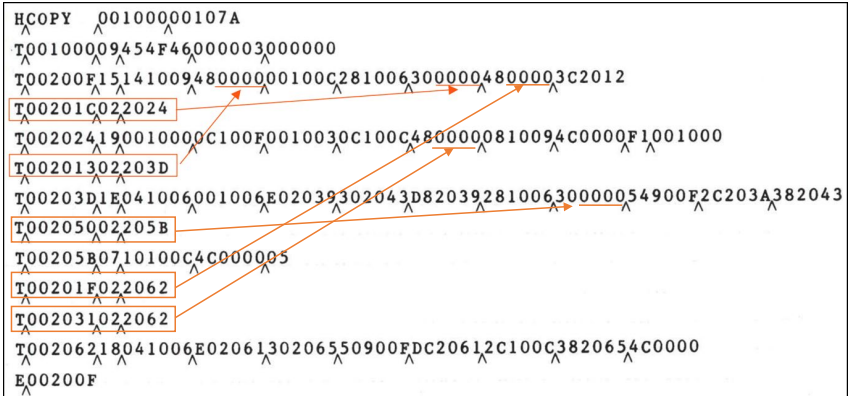
T 00201C 02 2024 => 201C 자리에 2024를 넣어라.는 의미
external reference
linking도 어떤 오브젝트를 수정해야 하는지
컨트롤 섹션 이용하기 위한 3가지
빨간색 2개 extdef extref 의미 알아야한다.
external reference가 나오면 0을 집어 넣는다.
수정할 필드를 어떤 연산을 수행할지
modification 레코드 주어지면 어떤 의미인지
one pass 처리 방법 다르다 이해하자