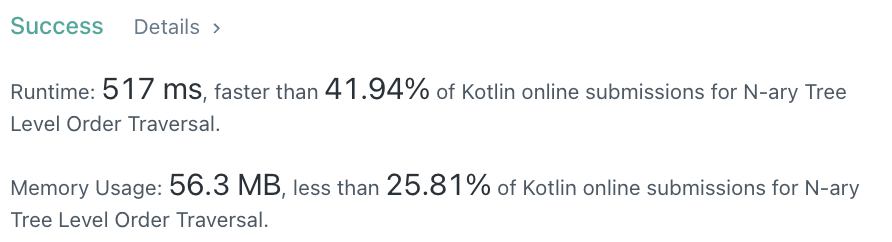
class Solution {
fun levelOrder(root: Node?): List<List<Int>> {
if (root == null) return listOf<List<Int>>()
val orders = mutableListOf<MutableList<Int>>()
val queue: Queue<Node> = LinkedList()
queue.add(root!!)
orders.add(mutableListOf(root.`val`))
while (queue.isNotEmpty()) {
val size = queue.size
orders.add(mutableListOf())
for (i in 0 until size) {
val curr = queue.poll()
for (child in curr.children) {
if (child != null) {
orders.last().add(child.`val`)
queue.add(child)
}
}
}
}
return orders.dropLast(1)
}
}