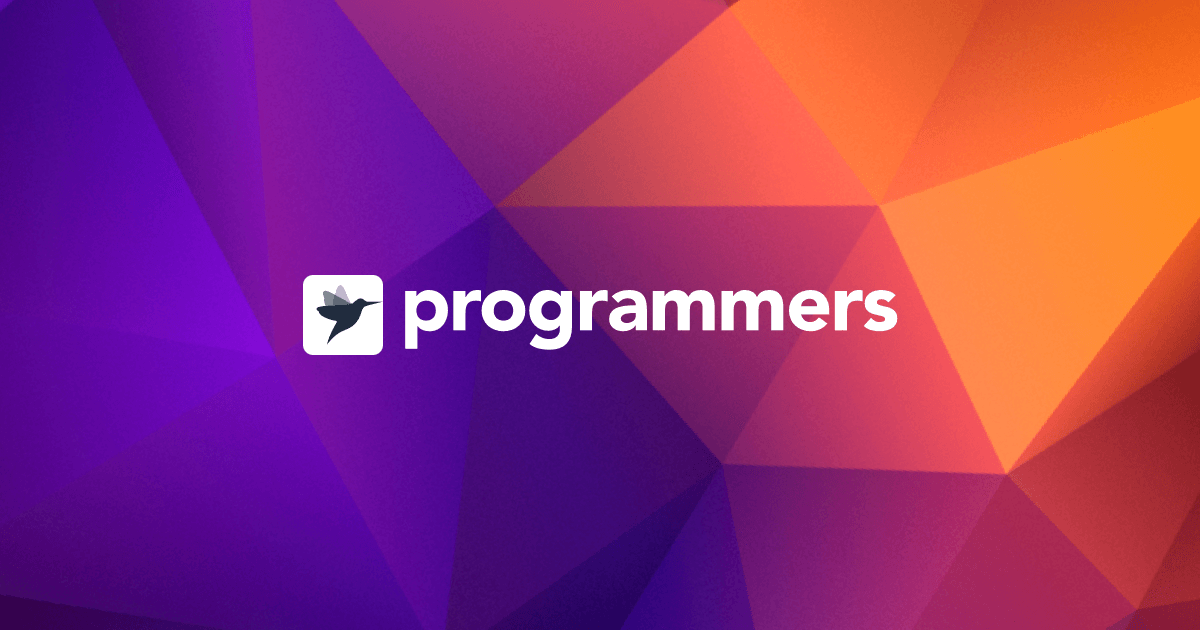
😀요점
준 선물 수
, 받은 선물 수
, 선물 지수
를 담는 객체 생성
- 2중 반복을 통한 다음 달 선물 수 계산
- 선물을 주지도, 받지도 않은 사람이 있을 수 있음 (테스트 케이스
17
, 19
)
서로 선물 이력이 없는 경우
, 선물 빈도까지 모두 같은 경우
두 경우 모두 선물 지수로 비교하여 더해야함
😎풀이
function solution(friends, gifts) {
const giftDict = {}
const nextMonthGift = {}
gifts.forEach(gift => {
const [from, to] = gift.split(" ")
if(giftDict[from]) {
giftDict[from].giveList.push(to)
giftDict[from].giveCount++
giftDict[from].giftScore++
} else {
giftDict[from] = {
giveCount: 1,
giftScore: 1,
takeCount: 0,
giveList: [to]
}
}
if(giftDict[to]) {
giftDict[to].giftScore--
giftDict[to].takeCount++
} else {
giftDict[to] = {
giftScore: -1,
takeCount: 1,
giveCount: 0,
giveList: []
}
}
})
for(let i = 0; i < friends.length; i++) {
const curFrom = friends[i]
nextMonthGift[curFrom] = nextMonthGift[curFrom] ?? 0
for(let j = i+1; j < friends.length; j++) {
const curTo = friends[j]
nextMonthGift[curTo] = nextMonthGift[curTo] ?? 0
if(!giftDict[curFrom]) {
nextMonthGift[curTo]++
continue
}
if(!giftDict[curTo]) {
nextMonthGift[curFrom]++
continue
}
const fromIdx = giftDict[curFrom].giveList.indexOf(curTo)
const toIdx = giftDict[curTo].giveList.indexOf(curFrom)
if(toIdx < 0 && fromIdx < 0) {
const fromGiftScore = giftDict[curFrom].giftScore
const toGiftScore = giftDict[curTo].giftScore
if(fromGiftScore === toGiftScore) continue
if(fromGiftScore > toGiftScore) nextMonthGift[curFrom]++
else nextMonthGift[curTo]++
continue
}
if(toIdx < 0 && fromIdx >= 0) {
nextMonthGift[curFrom]++
continue
}
if(toIdx >= 0 && fromIdx < 0) {
nextMonthGift[curTo]++
continue
}
const fromSum = giftDict[curFrom].giveList.reduce((acc, cur) => cur === curTo ? acc+1 : acc, 0)
const toSum = giftDict[curTo].giveList.reduce((acc, cur) => cur === curFrom ? acc+1 : acc, 0)
if(fromSum !== toSum) {
let addGift = null
if(fromSum > toSum) addGift = curFrom
else addGift = curTo
nextMonthGift[addGift]++
continue
}
const fromGiftScore = giftDict[curFrom].giftScore
const toGiftScore = giftDict[curTo].giftScore
if(fromGiftScore === toGiftScore) continue
if(fromGiftScore > toGiftScore) nextMonthGift[curFrom]++
else nextMonthGift[curTo]++
}
}
return Math.max(...Object.values(nextMonthGift))
}