알고리즘 분류
코드
#include <iostream>
using namespace std;
#include <vector>
#include <string>
#include <algorithm>
#include <map>
#include <set>
bool compare(string &a, string &b)
{
int lA = a.length();
int lB = b.length();
if (lA < lB)
return true;
else if (lA == lB)
{
//길이가 같을 경우 사전 순으로 나오게 하라.
for (int i = 0; i < lA; ++i)
{
if (a[i] == b[i])
continue;
return a[i] < b[i];
}
}
return false;
}
int main()
{
int n;
cin >> n;
vector<string>v(n);
for (int i = 0; i < n; ++i)
{
cin >> v[i];
}
sort(v.begin(), v.end(), compare);
//cout << "output" << endl;
//for (auto iter : v)
// cout << iter << endl;
// 함수 쓰지 말고, 중복 제거 어떻게 할것인가에 대한 생각을 하자.
// 정렬했으니까,,,,, 출력만 하면됨 .
string word = "";
for (auto iter : v)
{
if (word != iter)
{
word = iter;
cout << word << endl;
}
else
continue;
}
// 중복된 원소를 처리해야 함.
//set<string >v;
//
//for (int i = 0; i < n; ++i)
//{
// string s;
// cin >> s;
// v.insert(s);
//}
//
//sort(v.begin(), v.end() , compare);
//
//for (auto iter : v)
// cout << iter << endl;
}
중복 처리를 어떻게 할것인가?
- set으로 진행해야 할까? 생각을 했지만,
이럴경우, compare 함수 만드는데 복잡함, 어려움.
- 어차피 정렬되어 있으니까. temp 변수에 넣고, 인접한 것과 비교하는 방식으로 진행함.
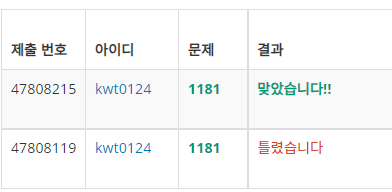
중복된 컨테이저 지우는 함수
#include <iostream>
using namespace std;
#include <vector>
#include <string>
#include <algorithm>
#include <map>
#include <set>
bool compare(string &a, string &b)
{
int lA = a.length();
int lB = b.length();
if (lA < lB)
return true;
else if (lA == lB)
{
//길이가 같을 경우 사전 순으로 나오게 하라.
for (int i = 0; i < lA; ++i)
{
if (a[i] == b[i])
continue;
return a[i] < b[i];
}
}
return false;
}
int main()
{
int n;
cin >> n;
vector<string>v(n);
for (int i = 0; i < n; ++i)
{
cin >> v[i];
}
sort(v.begin(), v.end(), compare);
v.erase(unique(v.begin(), v.end()), v.end());
string word = "";
for (auto iter : v)
{
cout << iter << endl;
}
}
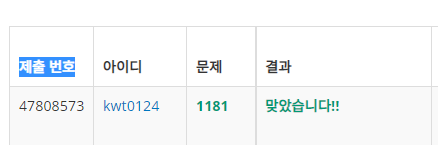