코드
#include <iostream>
using namespace std;
#include <string>
#include <vector>
#include <iostream>
#include <string>
class People
{
std::string name;
public:
People(std::string s) : name(s) {}
People(const People& p) : name(p.name)
{
std::cout << "copy" << std::endl;
}
People(People&& p) noexcept : name(std::move(p.name))
{
std::cout << "move" << std::endl;
}
People& operator=(const People& p)
{
name = p.name;
std::cout << "copy=" << std::endl;
return *this;
}
People& operator=(People&& p) noexcept
{
name = std::move(p.name);
std::cout << "move=" << std::endl;
return *this;
}
};
int main()
{
vector<People> v;
v.push_back(People("A"));
v.push_back(People("B"));
v.push_back(People("C"));
v.push_back(People("D"));
cout << "v의 size는" << v.size() << endl;
cout << "------------------------" << endl;
//vector<People> v2(begin(v), end(v));
cout << "v2 부분" << endl;
vector<People> v2(make_move_iterator(begin(v)),
make_move_iterator(end(v)));
cout << "v의 size는" << v.size() << endl;
cout << "v2의 size는" << v2.size() << endl;
cout << "v3 부분" << endl;
vector<People> v3(move(v2));
cout << "v의 size는" << v.size() << endl;
cout << "v2의 size는" << v2.size() << endl;
cout << "v3의 size는" << v3.size() << endl;
cout << "hello" << endl;
/*
auto p1 = begin(v);
People peo1 = *p1; // 복사 생성자..
// 방법 1.
move_iterator< vector<People>::iterator > p2(p1);
People peo2 = *p2; // move 생성자..
//People peo3 = move(*p1); // move 생성자..
// 방법 2.
auto p3 = make_move_iterator(begin(v));
*/
}
결과가 다름...
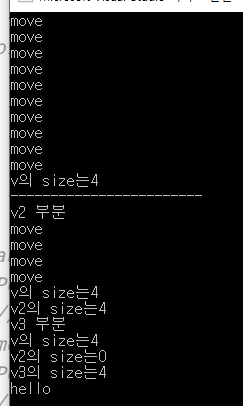