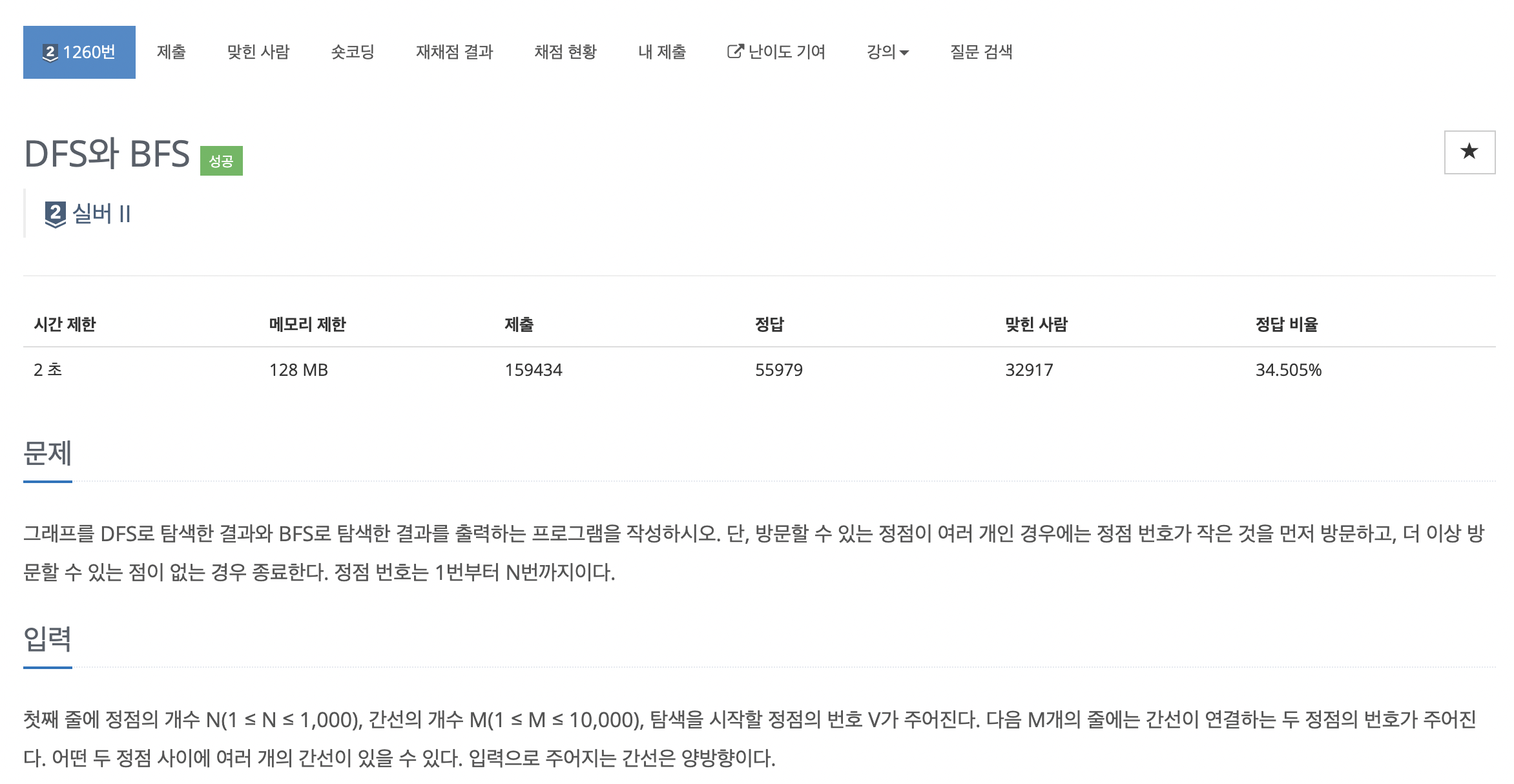
#include <iostream>
#include <queue>
using namespace std;
#define MAX 1001
int N, M, V;
int map[MAX][MAX];
bool visited[MAX];
queue<int> q;
void reset() {
for (int i = 1; i <= N; i++) {
visited[i] = 0;
}
}
void DFS(int v) {
visited[v] = true;
printf("%d ", v);
for (int i = 1; i <= N; i++) {
if (map[v][i] == 1 && visited[i] == 0) {
DFS(i);
}
}
}
void BFS(int v) {
q.push(v);
visited[v] = true;
printf("%d ", v);
while (!q.empty()) {
v = q.front();
q.pop();
for (int w = 1; w <= N; w++) {
if (map[v][w] == 1 && visited[w] == 0) {
q.push(w);
visited[w] = true;
printf("%d ", w);
}
}
}
}
int main() {
scanf("%d %d %d", &N, &M, &V);
for (int i = 0; i < M; i++) {
int a, b;
scanf("%d %d", &a, &b);
map[a][b] = 1;
map[b][a] = 1;
}
reset();
DFS(V);
printf("\n");
reset();
BFS(V);
return 0;
}
오늘의 키포인트
- DFS는 재귀함수, BFS는 큐(for문)로 구현
- 리셋은 visited만 초기화해주면 된다.
- 관련 문제 더 풀어볼 것