Callable
Future
결과 가져오기 get()
public static void main(String[] args) throws ExecutionException, InterruptedException {
ExecutorService executorService = Executors.newSingleThreadExecutor();
Callable<String> hello = () -> {
Thread.sleep(2000L);
return "Hello";
};
Future<String> submit = executorService.submit(hello);
System.out.println("Started!!");
submit.get();
System.out.println("End!!");
}
작업 상태 확인하기 isDone()
작업 취소하기 cancle()
여러 작업 동시에 실행하기 invokeAll()
ExecutorService executorService = Executors.newSingleThreadExecutor();
Callable<String> hello = () -> {
Thread.sleep(2000L);
return "Hello";
};
Callable<String> the = () -> {
Thread.sleep(3000L);
return "the";
};
Callable<String> java = () -> {
Thread.sleep(1000L);
return "java";
};
List<Future<String>> futures = executorService.invokeAll(Arrays.asList(hello, the, java));
for(Future<String> f : futures){
System.out.println(f.get());
}
executorService.shutdown();
여러 작업 중에 하나라도 먼저 응답이 오면 끝내기 invokeAny()
ExecutorService executorService = Executors.newFixedThreadPool(4);
Callable<String> hello = () -> {
Thread.sleep(2000L);
return "Hello";
};
Callable<String> the = () -> {
Thread.sleep(3000L);
return "the";
};
Callable<String> java = () -> {
Thread.sleep(1000L);
return "java";
};
String s = executorService.invokeAny(Arrays.asList(hello, the, java));
System.out.println(s);
executorService.shutdown();
```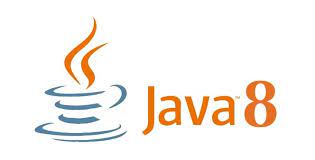