기초
- jsp : HTML 코드에 JAVA 코드를 넣어 동적웹페이지를 생성하는 웹어플리케이션 도구
- html 코드에 java 코드를 사용하기 위해서는 <% java %> 사용이 필요하며 가운데에 java 구문을 넣으면 된다.
- 중간중간 섞어서 써야해서 코드가 굉장히 복잡해 보인다.
- <%! %> 앞쪽 느낌표가 포함되면 선언문이다.
- html 태그를 제외한 기본 문법은 java 기반이다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link href="https://fonts.googleapis.com/css2?family=East+Sea+Dokdo&family=Moirai+One&family=Nanum+Pen+Script&family=Orbit&display=swap" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.7.0.js"></script>
<title>Insert title here</title>
</head>
<body>
<h2>JSP는 HTML5구조에서 자바코드를 사용할수 있는 서버언어이다.</h2>
<h3>Servlet은 확장자가 java이며 자바클래스 구조에 <br> html.css.js도 사용할 수 있다.</h3>
<h3>jsp 주석은 2가지 가능하다. </h3>
<!-- 주석1 : 소스보기에서는 보인다. -->
<%-- jsp주석: 소스보기에서도 안보인다. --%>
<%-- <%=name %>님의 나이는 <%=age %>세 입니다. <!-- 여기서는 못읽는다. --> --%>
<%!
int age=20;
String name="김영환";
public String getCheck(){
if(age>20){
return "성인입니다.";
} else {
return "미성년자 입니다.";
}
}
%>
<%-- <%=name %>님의 나이는 <%=age %>세 입니다. --%>
<h3 class='alert alert-info'>멤버변수 출력 </h3>
<b>이름: <%=name %></b><br> <!-- 표현식 -->
<b>나이: <%=age %>세(<%=getCheck() %>)</b><br>
</body>
</html>
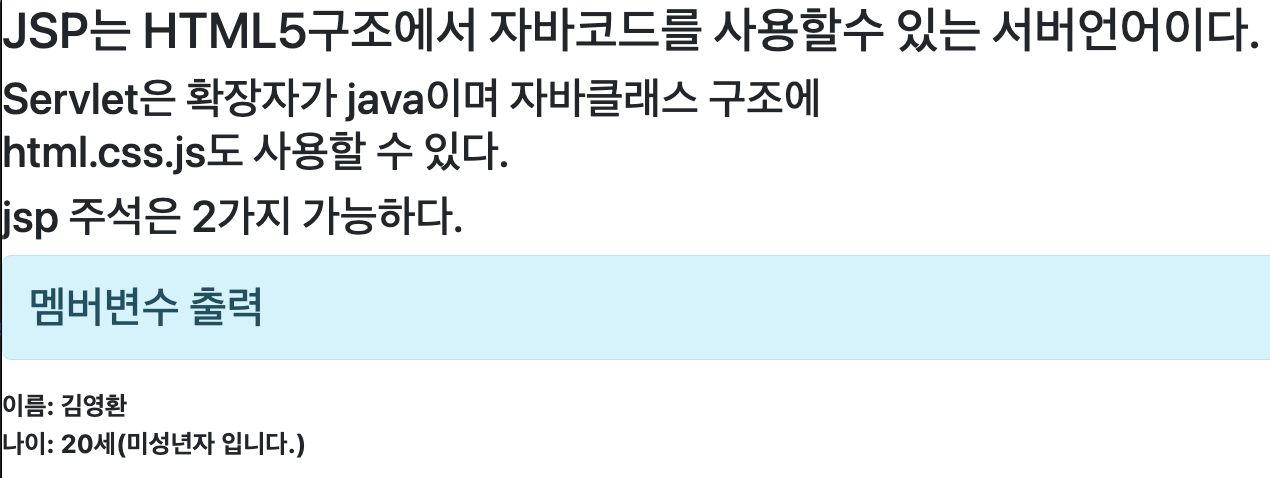
배열
색상배열
- 기본 배열문제이다. java와 html 태그를 섞기 위해 <% %> 를 중간중간 섞어놨다. 주의하자.
- 완전 java는 +로 변수를 연결하지만 여기에서는 <%= %> 를 이용하여 변수를 연결한다.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link href="https://fonts.googleapis.com/css2?family=East+Sea+Dokdo&family=Moirai+One&family=Nanum+Pen+Script&family=Orbit&display=swap" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.7.0.js"></script>
<title>Insert title here</title>
</head>
<body>
<%
String [] colors={"green","yello","pink","blue","magenta"};
String title="배열출력";
%>
<table class="table table-bordered" style="width:200px;">
<caption><b><%=title %></b></caption>
<tr>
<th>번호</th>
<th>색상</th>
</tr>
<%
for(int i=0;i<colors.length;i++){
%>
<tr>
<td align="center"><%=i+1 %></td>
<td>
<b style="color: <%=colors[i]%>"><%=colors[i] %></b>
</td>
</tr>
<%}
%>
</table>
</body>
</html>
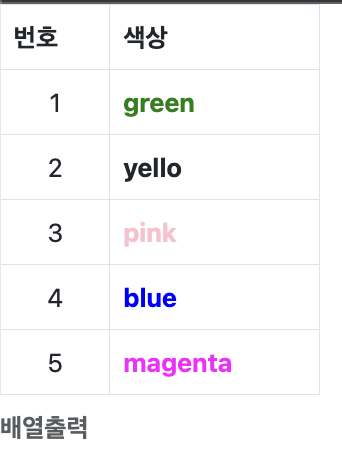
구구단
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link href="https://fonts.googleapis.com/css2?family=East+Sea+Dokdo&family=Moirai+One&family=Nanum+Pen+Script&family=Orbit&display=swap" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.7.0.js"></script>
<title>Insert title here</title>
</head>
<body>
<table class="table table-bordered">
<tr>
<td colspan="8" align="center">
<b style="font-size: 2em;">전체 구구단</b>
</td>
</tr>
<tr>
<%
for (int dan=2;dan<=9;dan++){
%>
<td align="center"><%=dan %> 단 </td>
<%
}
%>
</tr>
<%
for(int i=1;i<=9;i++){
%>
<tr>
<%
for(int dan=2;dan<=9;dan++){
%>
<td align="center">
<%=dan %>X<%=i %>=<%=dan+i %>
</td>
<%
}
%>
</tr>
<%
}
%>
</table>
</body>
</html>
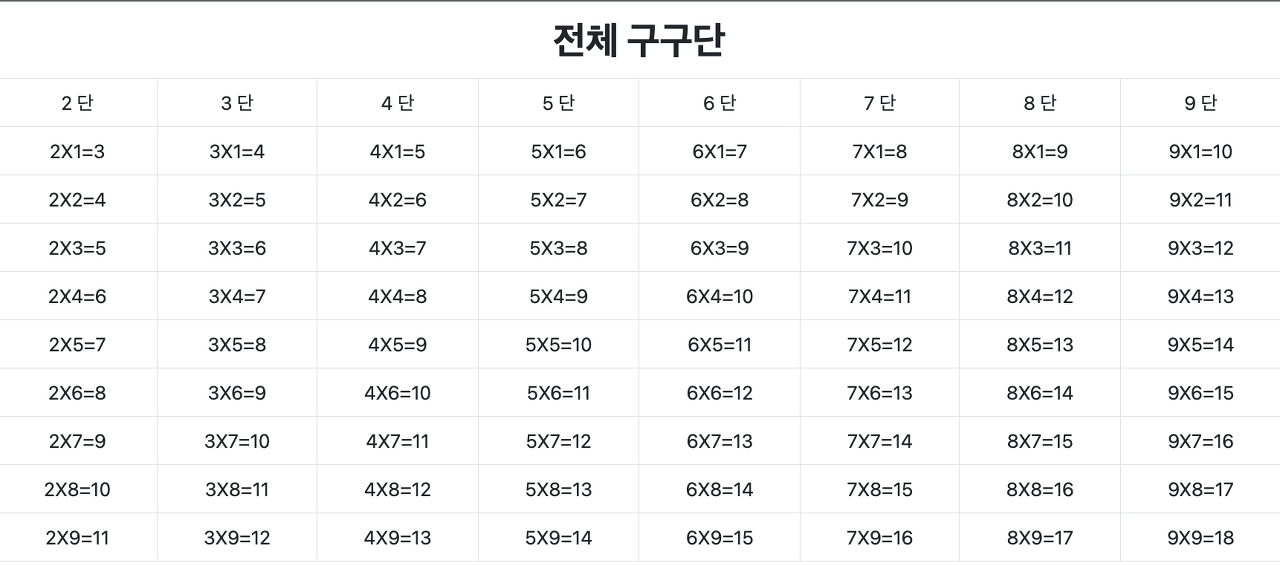
이미지 배열
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link href="https://fonts.googleapis.com/css2?family=East+Sea+Dokdo&family=Moirai+One&family=Nanum+Pen+Script&family=Orbit&display=swap" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.7.0.js"></script>
<title>Insert title here</title>
</head>
<body>
<%
String [] str={"1.jpg","2.jpg","3.jpg","4.jpg","5.jpg","6.jpg","7.jpg","8.jpg","9.jpg"};
int n=0;
%>
<table class="table table-bordered" style="width: 460px">
<%
for(int i=0;i<3;i++){
%>
<tr>
<%
for(int j=0;j<3;j++){
%>
<td>
<img alt="" src="../Img/shoppingmall/<%=str[n] %>" width="150" height="150">
</td>
<%
n++;
}
%>
</tr>
<%
}
%>
</table>
</body>
</html>
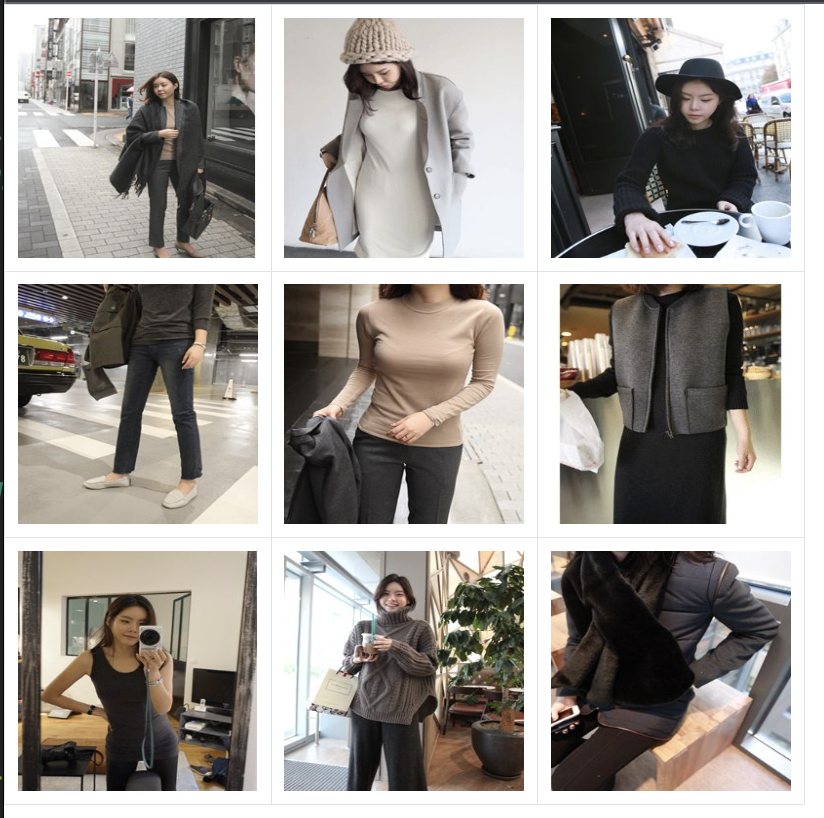
이미지 배열 2
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link href="https://fonts.googleapis.com/css2?family=East+Sea+Dokdo&family=Moirai+One&family=Nanum+Pen+Script&family=Orbit&display=swap" rel="stylesheet">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.7.0.js"></script>
<title>Insert title here</title>
</head>
<body>
<!
<table class="table table-bordered" style="width: 200px">
<%
int n=0;
for(int row=1;row<=4;row++){
%>
<tr>
<%
for(int col=1;col<=5;col++){
n++;
%>
<td>
<img alt="" src="../Img/flower_ani/f<%=n%>.png" width="150" height="150">
</td>
<%}
%>
</tr>
<%}
%>
</table>
</body>
</html>
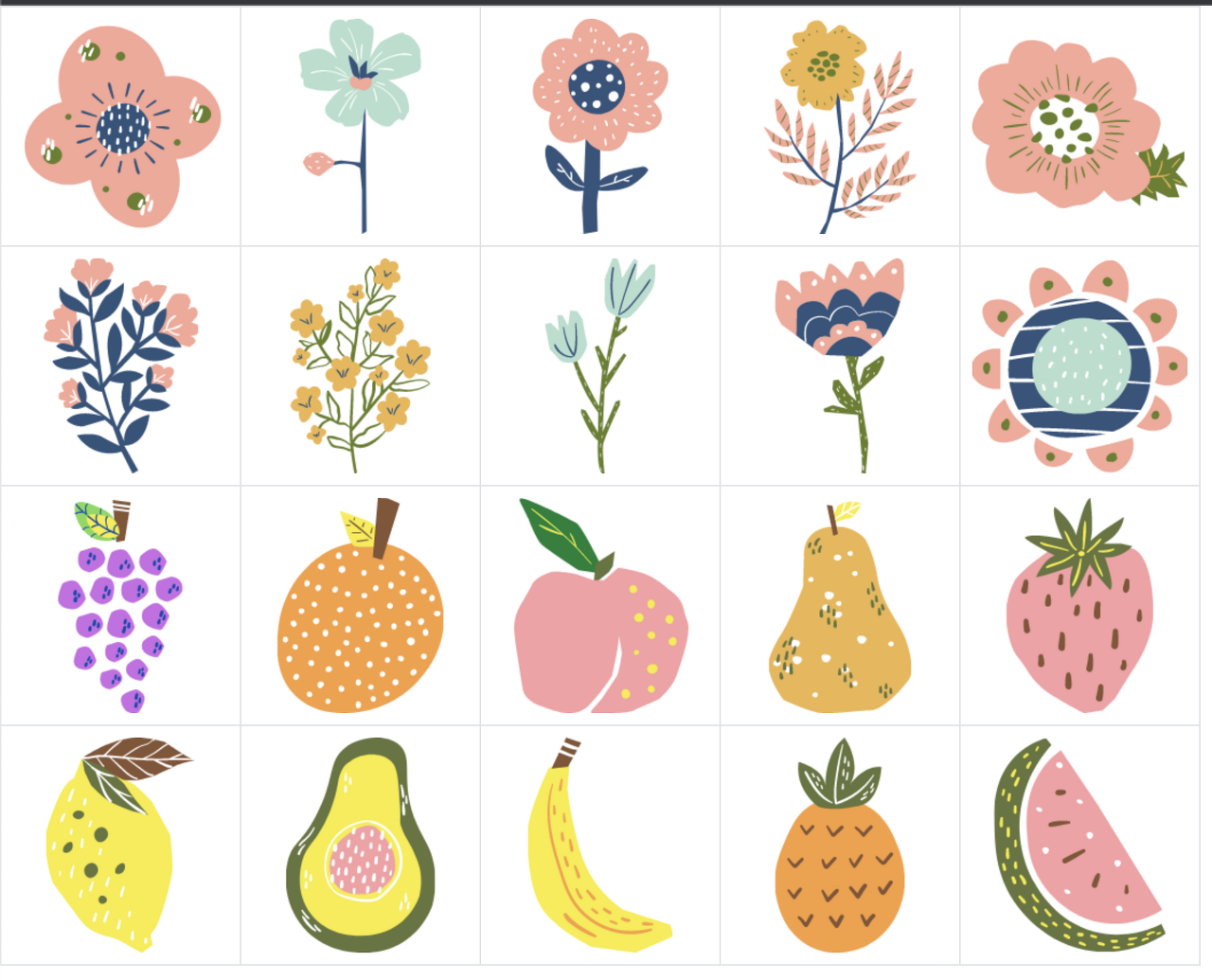