from typing import Union
from fastapi import FastAPI
app = FastAPI()
@app.get("/items/")
async def read_items(q: Union[str, None] = None):
results = {"items": [{"item_id": "Foo"}, {"item_id": "Bar"}]}
if q:
results.update({"q": q})
return results
- q (Query Parameter)는 Union[str, None] 으로 선언되어 있다. 이는 string type 이거나 None일 수 있다는 것을 의미한다.
- default가 None이므로 non required다.
Aditional validation
from typing import Union
from fastapi import FastAPI, Query
app = FastAPI()
@app.get("/items/")
async def read_items(q: Union[str, None] = Query(default=None, max_length=50)):
results = {"items": [{"item_id": "Foo"}, {"item_id": "Bar"}]}
if q:
results.update({"q": q})
return results
- 위 처럼 문자 개수를 50개로 제한할 수 있다.
- non required지만, 추가적인 제한을 줄 수 있는 것이다.
- default로 Query를 사용해서 max_length를 줄 수 있다. 또한 default 값을 줄 수 있어서 이전과 동일한 목적으로 제한만 추가할 수 있다.
- Query Parameter를 명시적으로 선언했기 때문에, 오히려 더 좋은 문법이 될 수 있다.
- 이를 통해 data를 검증할 수 있고, error를 방지 할 수 있다.
@app.get("/items/")
async def read_items(
q: Union[str, None] = Query(default=None, min_length=3, max_length=50)
):
@app.get("/items/")
async def read_items(
q: Union[str, None] = Query(
default=None, min_length=3, max_length=50, regex="^fixedquery$"
)
):
- regular expression도 줄 수 있다 !
- ^ : 해당 문자로 시작하는 것을 의미한다.
- fixedquery : exact value를 갖고 있는 문자열
- $ : 해당 문자로 끝나는 것을 의미한다.
@app.get("/items/")
async def read_items(q: Union[List[str], None] = Query(default=None)):
- 명시적으로 list(multiple values) 값을 받는 것으로 선언할 수도 있다.
http://localhost:8000/items/?q=foo&q=bar
{
"q": [
"foo",
"bar"
]
}
@app.get("/items/")
async def read_items(
q: Union[str, None] = Query(
default=None,
title="Query string",
description="Query string for the items to search in the database that have a good match",
min_length=3,
)
):
- title, description 등으로 파라미터에 대한 더 많은 정보를 제공할 수도 있다.
Alias parameters
@app.get("/items/")
async def read_items(q: Union[str, None] = Query(default=None, alias="item-query")):
- item-query 라는 것으로 alias를 선언할 수 있다.
http://127.0.0.1:8000/items/?item-query=foobaritems
Deprecating paramters
@app.get("/items/")
async def read_items(
q: Union[str, None] = Query(
default=None,
alias="item-query",
title="Query string",
description="Query string for the items to search in the database that have a good match",
min_length=3,
max_length=50,
regex="^fixedquery$",
deprecated=True,
)
):
- deprectaed = True를 하면 docs에서 깔끔하게 나중에 쓰지않는 API라는 것을 명시할 수 있다.
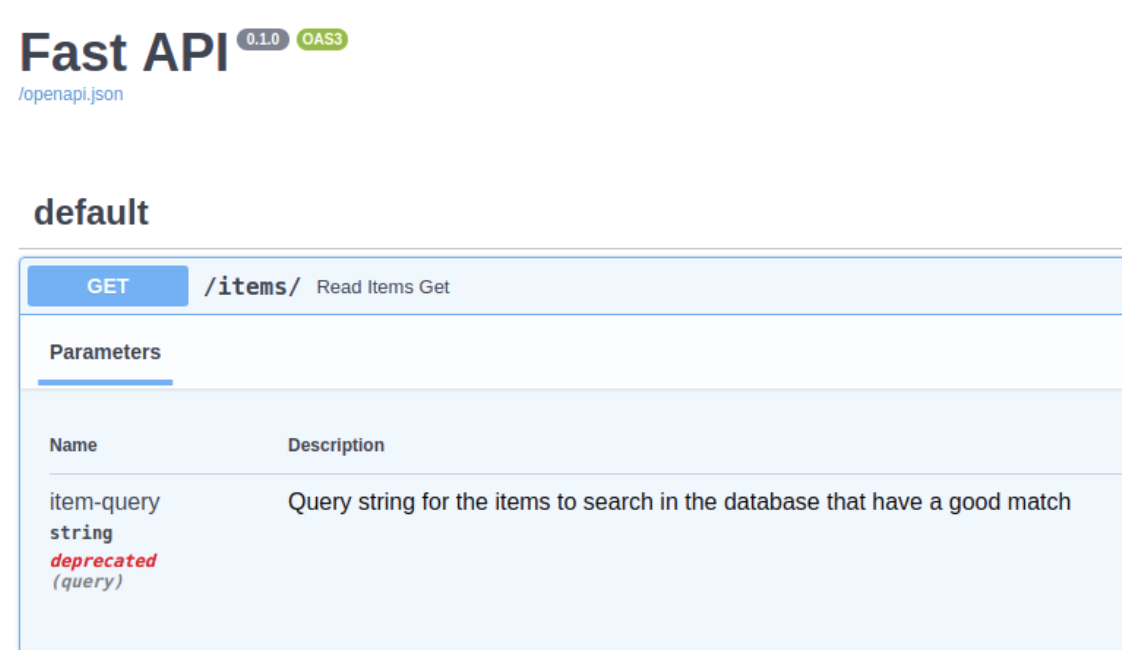
Exclude from OpenAPI
@app.get("/items/")
async def read_items(
hidden_query: Union[str, None] = Query(default=None, include_in_schema=False)
):
- include_in_schema = False로 두면 OpenAPI 스키마에서 Query Parameter가 제외된다.