1주차 실력진단
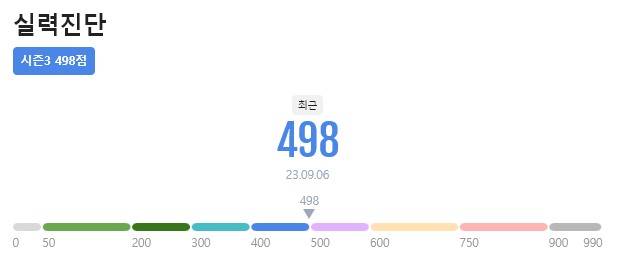
문제
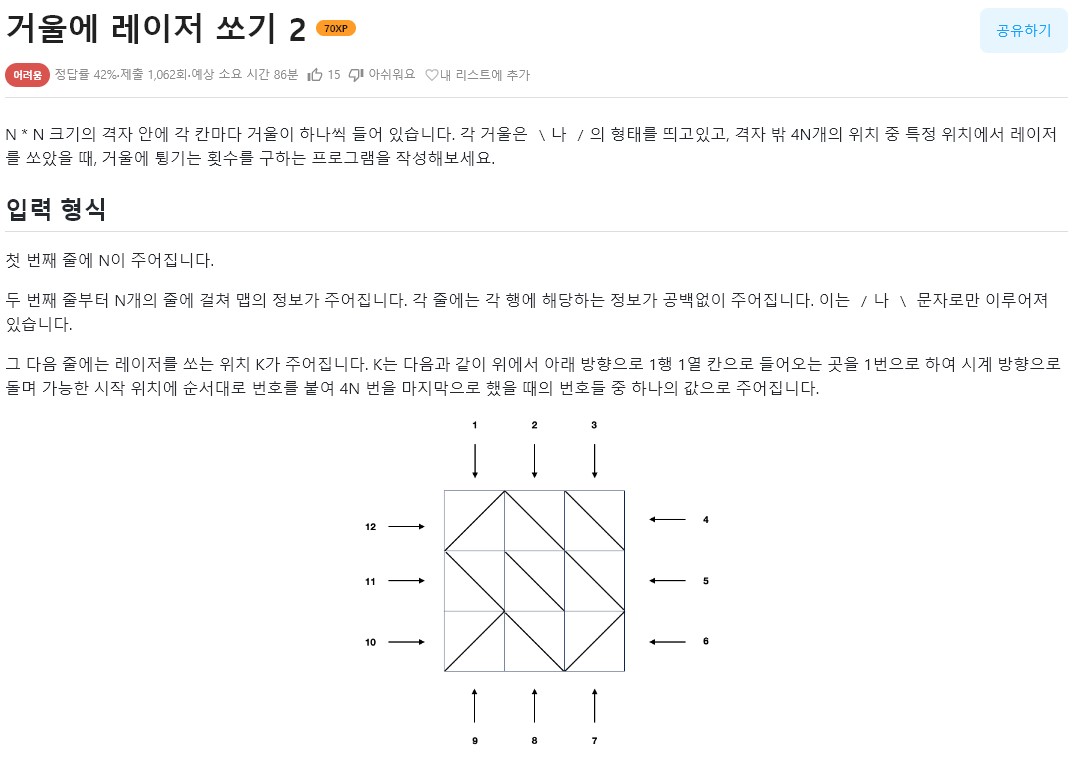
https://www.codetree.ai/missions/13/problems/shoot-a-laser-in-the-mirror-2?&utm_source=clipboard&utm_medium=text
풀이방법
- dx,dy 테크닉을 사용하여 거울에 반사될때 방향이 어떻게 되는지를 먼저 구했다
- 레이저가 들어오는 위치는 격자의 테두리이기 때문에 어떻게 구할까 고민하다 이 또한 dx,dy 테크닉을 사용하여 구해주었다.
코드
import java.util.*;
import java.io.*;
public class Main {
static int n, k;
static char[][] grid;
static int[] dx = {1, 0, -1, 0};
static int[] dy = {0, 1, 0, -1};
static class Pair {
int x, y, dir;
Pair(int x, int y, int dir) {
this.x = x;
this.y = y;
this.dir = dir;
}
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
n = Integer.parseInt(br.readLine());
grid = new char[n][n];
for (int i = 0; i < n; i++) {
String[] str = br.readLine().split("");
for (int j = 0; j < n; j++) {
grid[i][j] = str[j].charAt(0);
}
}
k = Integer.parseInt(br.readLine());
int x = 0, y = 0;
Pair[] arr = new Pair[4 * n];
int dir = 1;
int direction = 0;
arr[0] = new Pair(x, y, direction);
for (int i = 1; i < 4 * n; i++) {
int nx = x + dx[dir];
int ny = y + dy[dir];
if (nx < 0 || ny < 0 || nx >= n || ny >= n) {
dir = (dir + 3) % 4;
direction = (direction + 3) % 4;
arr[i] = new Pair(x, y, direction);
continue;
}
arr[i] = new Pair(nx, ny, direction);
x = nx;
y = ny;
}
System.out.println(bfs(arr[k - 1]));
}
private static int bfs(Pair cur) {
int x = cur.x;
int y = cur.y;
int dir = cur.dir;
int ans = 0;
while (true) {
int nx = 0, ny = 0;
if (grid[x][y] == '/') {
if (dir % 2 == 0) {
dir = (dir + 3) % 4;
nx = x + dx[dir];
ny = y + dy[dir];
ans++;
} else {
dir = (dir + 1) % 4;
nx = x + dx[dir];
ny = y + dy[dir];
ans++;
}
} else {
if (dir % 2 == 0) {
dir = (dir + 1) % 4;
nx = x + dx[dir];
ny = y + dy[dir];
ans++;
} else {
dir = (dir + 3) % 4;
nx = x + dx[dir];
ny = y + dy[dir];
ans++;
}
}
if (nx < 0 || ny < 0 || nx >= n || ny >= n) {
break;
}
x = nx;
y = ny;
}
return ans;
}
}
느낀점
- 솔직히 이 문제를 dx,dy를 사용해서 풀기에는 너무 어렵지 않나 생각했다.
하지만 차근차근 거울의 생김새에 따라 레이저가 어떻게 방향이 설정될까를 고민하고 직접 그려보니 충분히 해결가능한 문제였다.