재밌는 이벤트의 세계, JavaScript
자바스크립트에 작성된 변수들은 유효범위를 가지고있어요.
<script>
var vscope = 'global';
function fscope(){
alert(vscope);
}
function fscope2(){
alert(vscope);
}
fscope();
fscope2();
</script>
<script>
var vscope = 'global';
function fscope(){
var lv = 'local value';
alert(lv);
}
fscope();
alert(lv); // 오류발생
</script>
<script>
// 같은 이름의 지역변수와 전역변수가 동시에 정의되어 있다면, 지역변수된다.
var vscope = 'global';
function fscope(){
var vscope = 'local';
alert('함수 안 ' + vscope);
}
fscope();
alert('함수 밖 ' + vscope);
</script>
<script>
// fscope 변수 안에서 vscope가 재할당 되었다.
var vscope = 'global';
function fscope(){
vscope = 'local';
alert('함수 안 ' + vscope);
}
fscope();
alert('함수 밖 ' + vscope);
</script>
다음의 예제로 그 문제점을 살펴볼게요.
<script>
var name = 'html';
console.log(name);
var name = 'javascript';
console.log(name);
alert(name);
</script>
<script>
let name = 'html';
console.log(name);
// Uncaught SyntaxError: Identifier 'name' has already been declared
//let name = 'javascript';
console.log(name);
// 변수 재할당이 가능
name = 'javascript';
console.log(name);
</script>
<script>
const name = 'html';
console.log(name);
// Uncaught SyntaxError: Identifier 'name' has already been declared
// ES6가 잘 녹아들지 않은 툴에서는 문법 오류시 체크가 안될 수 있다.
//const name = 'javascript';
console.log(name);
// Uncaught TypeError: Assignment to constant variable.
// const 변수는 재할당이 안된다.
name = 'javascript';
console.log(name);
</script>
(1) let myarray = new Array(값1, 값2, ..., 값n);
(2) let myarray = [값1, 값2, ..., 값n];
배열이름[인덱스]
<script>
let myarray = ["html", "css", "javascript"];
// 배열값 읽기
document.write("<h1>" + myarray[0] + "</h1>");
document.write("<h1>" + myarray[1] + "</h1>");
document.write("<h1>" + myarray[2] + "</h1>");
// 배열에 저장된 값 재할당(변경)
myarray[0] = "JAVA";
myarray[1] = "JSP";
myarray[2] = "Spring Boot";
document.write("<h1>" + myarray[0] + "</h1>");
document.write("<h1>" + myarray[1] + "</h1>");
document.write("<h1>" + myarray[2] + "</h1>");
document.write("<h1>----------------------------</h1>");
// 반복문을 통하여 배열 출력
for( let i = 0; i < myarray.length; i++ ){
document.write("<p>" + myarray[i] + "</p>");
}
</script>
배열이름[행][열]
<script> let myarray = new Array( new Array(값1, 값2, ... 값n), new Array(값1, 값2, ... 값n) ); let myarray = [ [값1, 값2, ... 값n], [값1, 값2, ... 값n] ]; </script>
- 행 : 배열이름.length;
- 열 : 배열이름[n].length;
<script> let myarray = [ ['html', 'css', 'javascript'], ['Java', 'JSP', 'Spring'] ];
// 반복문, myarray 배열안의 항목 출력
for( let i = 0; i < myarray.length; i++ ){
for( let j = 0; j < myarray[i].length; j++ ){
document.write("<h1>" + myarray[i][j] + "</h1>");
}
}
</script>
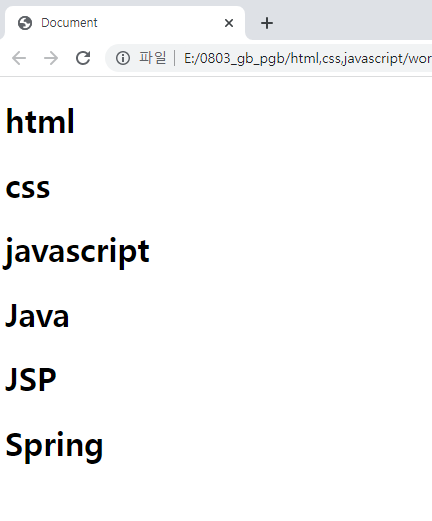
### 📎 배열 함수
#### ⚫️ push()
배열에 값을 추가, **요소 추가**
```html
<script>
let li = ['a', 'b', 'c', 'd'];
li.push('e');
document.write(li);
document.write
</script>
배열에 값을 추가, 배열 추가
<script<>
let li3 = ['a', 'b', 'c'];
li3 = li3.concat(['d', 'e', 'f']);
document.write(li3);
document.write
</script>
맨 앞에 배열의 값을 추가
<script>
let li4 = ['a', 'b', 'c'];
li4.unshift('zzz');
document.write(li4);
</script>
'이재영'을 파라미터로 넘기는 함수를 생성하여 '이재영' 이라는 이름이 몇 번 반복되는지 출력해라.
<script>
let s = [
'이유덕', '이재영', '권종표', '이재영', '박민호', '강상희',
'이재영', '김지완', '최승혁', '이성연', '박영서', '박민호', '전경헌', '송정환', '김재성', '이유덕', '전경헌'
];
function name(param){
let name = param;
let count = 0;
for( let i = 0; i < s.length; i++ ){
if( s[i] === name ){
count++;
}
}
return name + " : " + count;
};
document.write(name('이재영'));
</script>
함수를 생성하여 중복된 이름을 제거한 배열을 출력해라.
결과 값 : 권종표,강상희,김지완,최승혁,이성연,박영서,송정환,김재성
<script>
let s = [
'이유덕', '이재영', '권종표', '이재영', '박민호', '강상희',
'이재영', '김지완', '최승혁', '이성연', '박영서', '박민호', '전경헌', '송정환', '김재성', '이유덕', '전경헌'
];
function name2(){
let uniq = [];
for( let i = 0; i < s.length; i++ ){
let uniq_cnt = 0;
for( let j = 0; j < s.length; j++ ){
if( s[i] == s[j] ){
uniq_cnt++;
}
}
if( uniq_cnt < 2 ){
uniq.push(s[i]);
}
}
return uniq;
}
document.write(name2());
</script>
출처
https://media.giphy.com/media/qqtvGYCjDNwac/giphy.gif
https://media.giphy.com/media/26tPplGWjN0xLybiU/giphy.gif