- 스프링시큐리티는 다양한 프레임워크 및 API와의 통합을 제공하고 있으며 Servlet3 과Spring MVC와 통합을 통해 여러 편리한 기능들을 사용 할 수 있다
- 인증 관련 기능들을 필터가 아닌 서블릿 영역에서 처리 할 수 있다
1. Servlet API 통합
Servlet 3+ 통합
1) SecurityContextHolderAwareRequestFilter
- HTTP 요청이 처리될 때 HttpServletRequest 에 보안 관련 메소드를 추가적으로 제공하는 래퍼(SecurityContextHolderAwareRequestWrapper) 클래스를 적용한다 • 이를통해개발자는서블릿API의보안메소드를사용하여인증,로그인,로그아웃등의작업을수행할수있다
- request 객체 + 보안 관련 메소드 -> warpper
- HttpServlet3RequestFactory 생성
2) HttpServlet3RequestFactory
- Servlet 3 API 와의 통합을 제공하기 위한 Servlet3SecurityContextHolderAwareRequestWrapper 객체를 생성한다
- Servlet3SecurityContextHolderAwareRequestWrapper 클래스는 SecurityContextHolderAwareRequestFilter를 상속받은 자식 클래스(구현체)이다.
3) Servlet3SecurityContextHolderAwareRequestWrapper
- HttpServletRequest 의 래퍼 클래스로서 Servlet 3.0의 기능을 지원하면서 동시에 SecurityContextHolder 와의 통합을 제공한다(인증 관련 기능 제공)
- 이 래퍼를 사용함으로써 SecurityContext 에 쉽게 접근할 수 있고 Servlet 3.0의 비동기 처리와 같은 기능을 사용하는 동안 보안 컨텍스트를 올바르게 관리할 수 있다
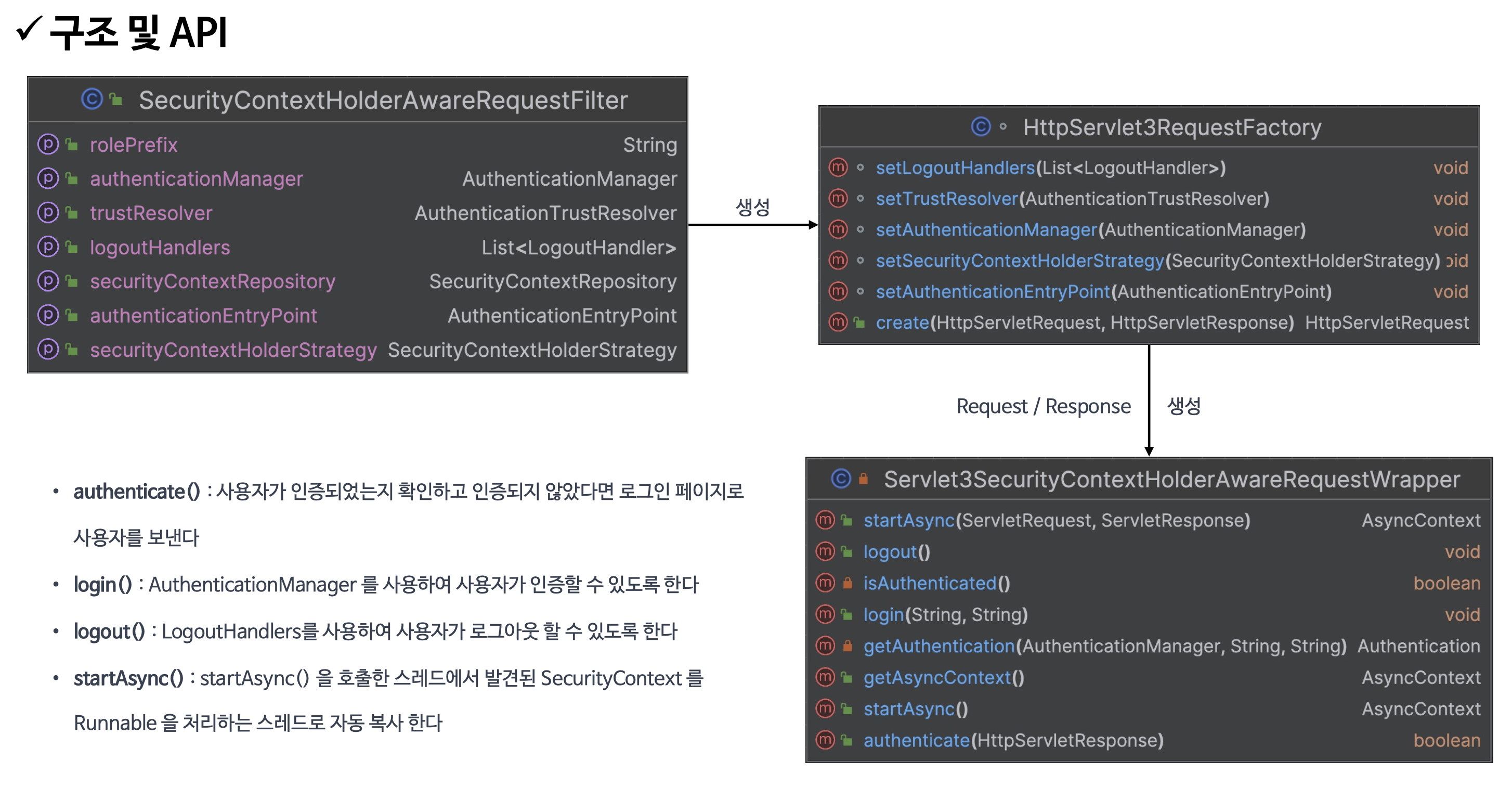
@GetMapping("/login")
public String login(HttpServletRequest request, MemberDto memberDto) throws ServletException, IOException {
request.login(memberDto.getUsername(),memberDto.getPassword());
System.out.println("PreAuthorize");
return "/index";
}
@GetMapping("/users")
public List<User> users(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
boolean authenticate = request.authenticate(response);
if(authenticate){
UserService.users();
}
return Collection.emptyList();
}
2. Spring MVC 통합
@AuthenticationPrincipal
- Spring Security는 Spring MVC 인수에 대한 현재 Authentication.getPrincipal()을 자동으로 해결 할 수 있는 ⭐️AuthenticationPrincipalArgumentResolver 를 제공한다
• Spring MVC 에서 @AuthenticationPrincipal 을 메서드 인수에 선언하게 되면 Spring Security 와 독립적으로 사용할 수 있다
- AuthenticationPrincipalArgumentResolver가 어노테이션의 작업을 구현하여 실행하는 과정을 수행하는것
- 📌기본적으로 인증을 받지 못한 사용자는 Principal이 anonymouseUser 익명 사용자 문자열로 들어온다 따라서 객체명, 필드명이 존재하지 않는다.
@Target({ ElementType.PARAMETER, ElementType.TYPE })
@Retention(RetentionPolicy.RUNTIME)
@Documented
@AuthenticationPrincipal(expression = "#this == 'anonymouseUser' ? null : User")
public @interface CurrentUser {
}
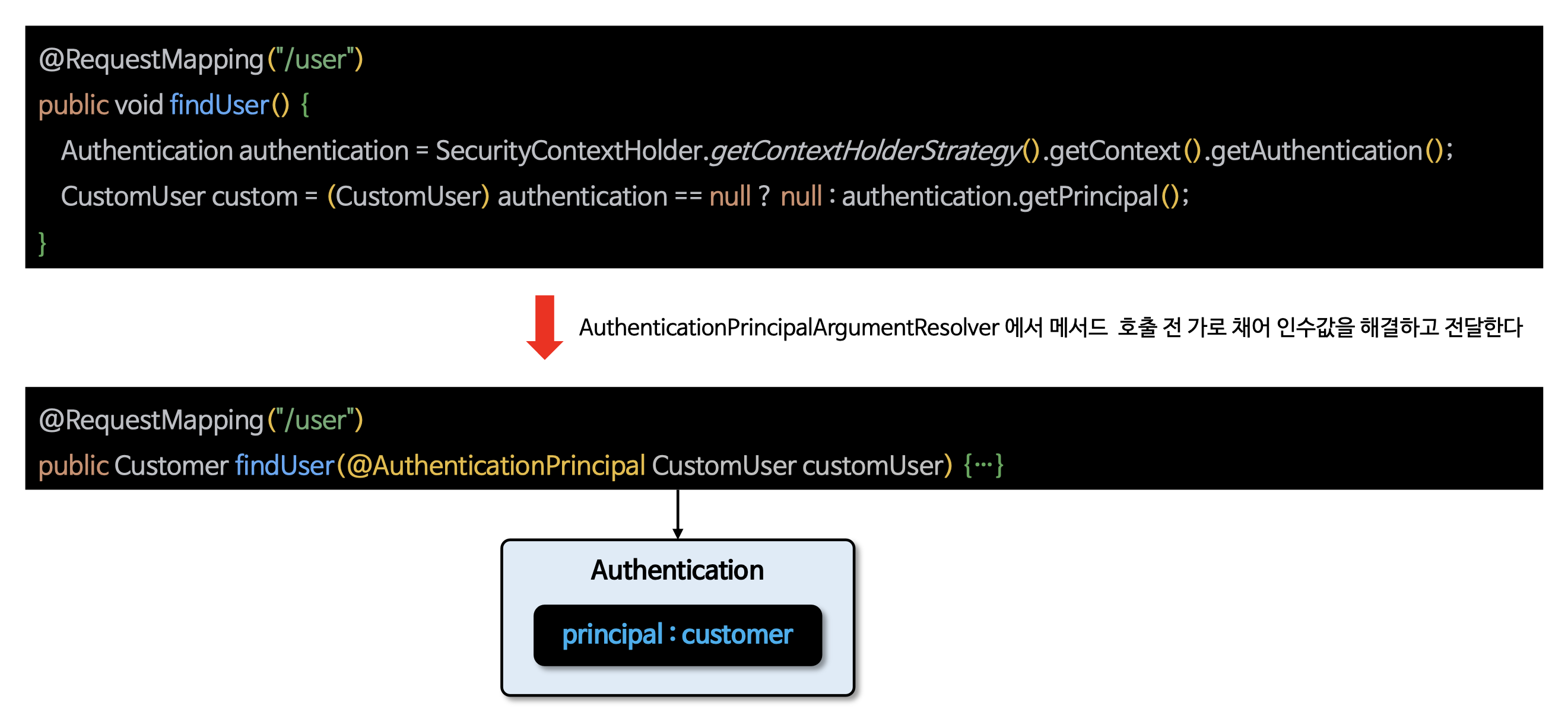
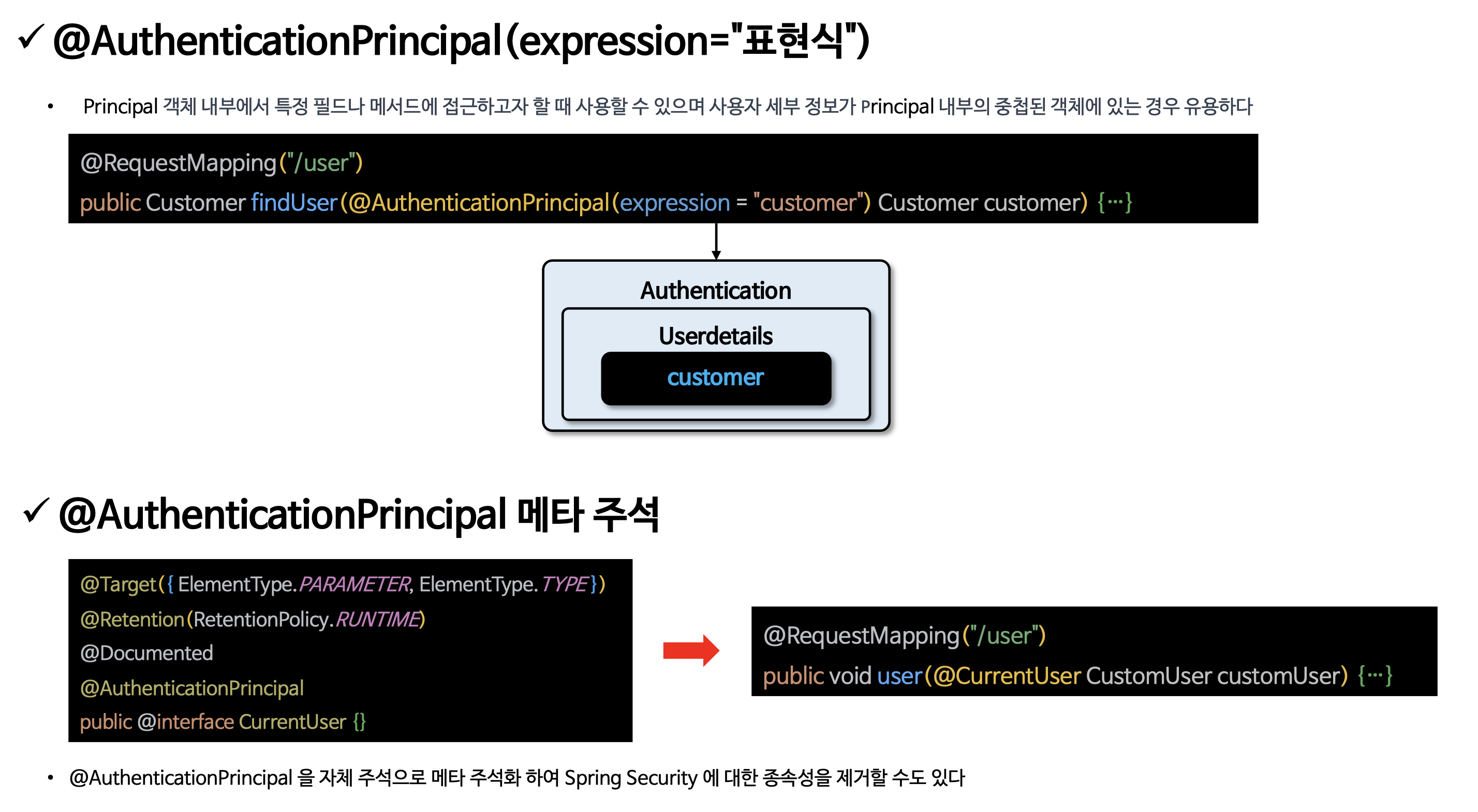
차이
-
Authentication 내의 Principal 객체를 의미한다. 이미 해당 Principal 객체가 존재해야하는 것. Authentication에서 Principal 객체를 바로 가져오는 것
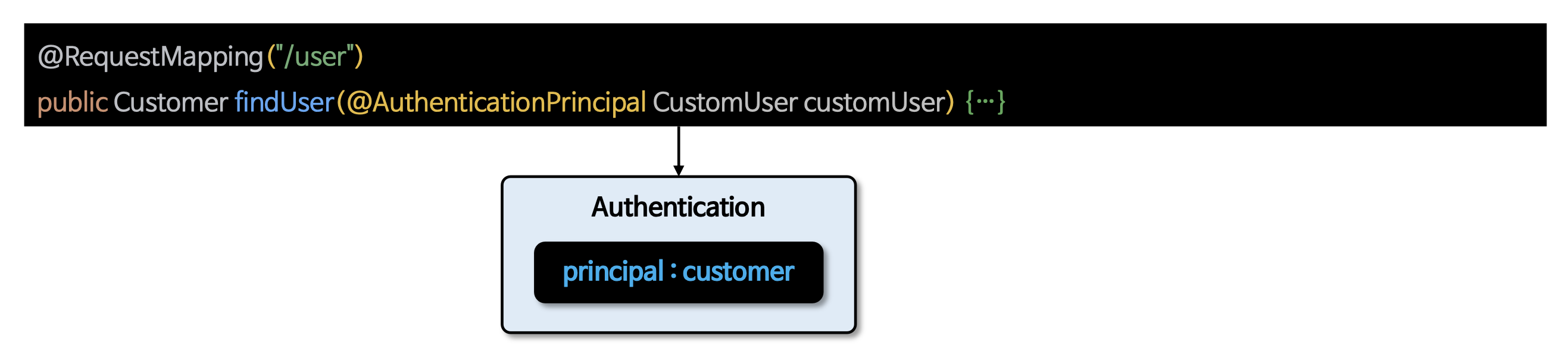
-
Principal에 속하는 Userdetails안에 값을 사용하기 위한것
customer의 객체, 필드 등
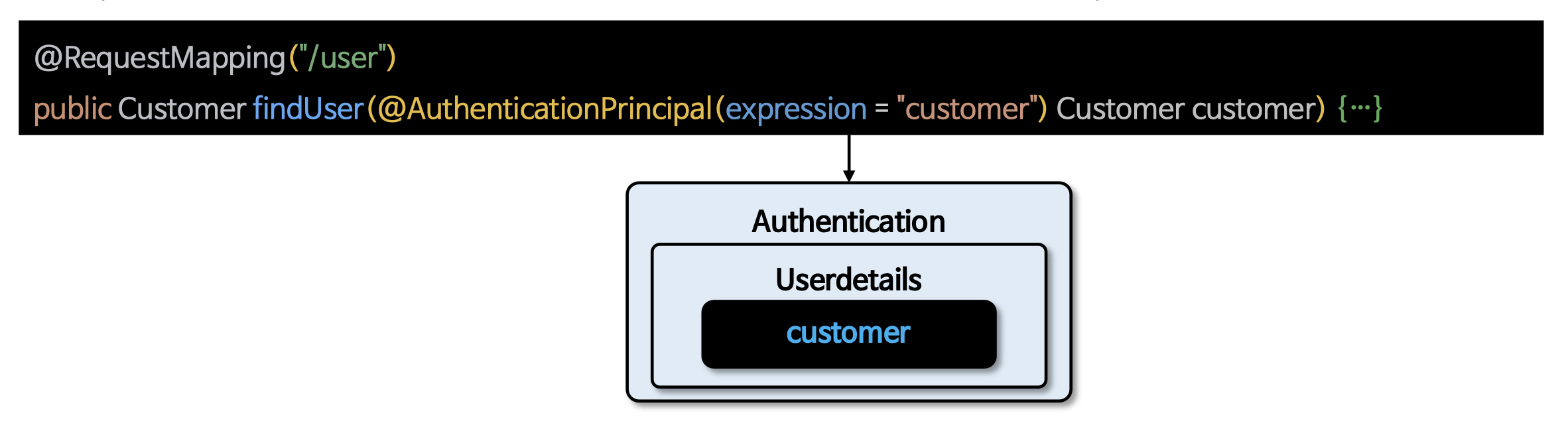
3. Spring MVC 비동기 통합
- Spring Security 는 Spring MVC Controller 에서 Callable 을 실행하는 비동기 스레드에 SecurityContext 를 자동으로 설정하도록 지원한다
- Spring Security 는 WebAsyncManager 와 통합하여 SecurityContextHolder 에서 사용 가능한 SecurityContext 를 Callable 에서 접근 가능하도록 해 준다
- 부모 스레드와 자식 스레드가 있을 때 부모스레드의 SecurityContext를 자식스레드에서도 사용할 수 있도록 자동 설정되는 것
WebAsyncManagerIntegrationFilter
- SecurityContext 와 WebAsyncManager 사이의 통합을 제공하며 WebAsyncManager 를 생성하고 SecurityContextCallableProcessingInterceptor를 WebAsyncManager 에 등록한다
WebAsyncManager
- 스레드 풀의 비동기 스레드를 생성하고 Callable 를 받아 실행시키는 주체로서 등록된 SecurityContextCallableProcessingInterceptor 를 통해 현재 스레드 가 보유하고 있는 SecurityContext 객체를 비동기 스레드의 ThreadLocal 에 저장시킨다
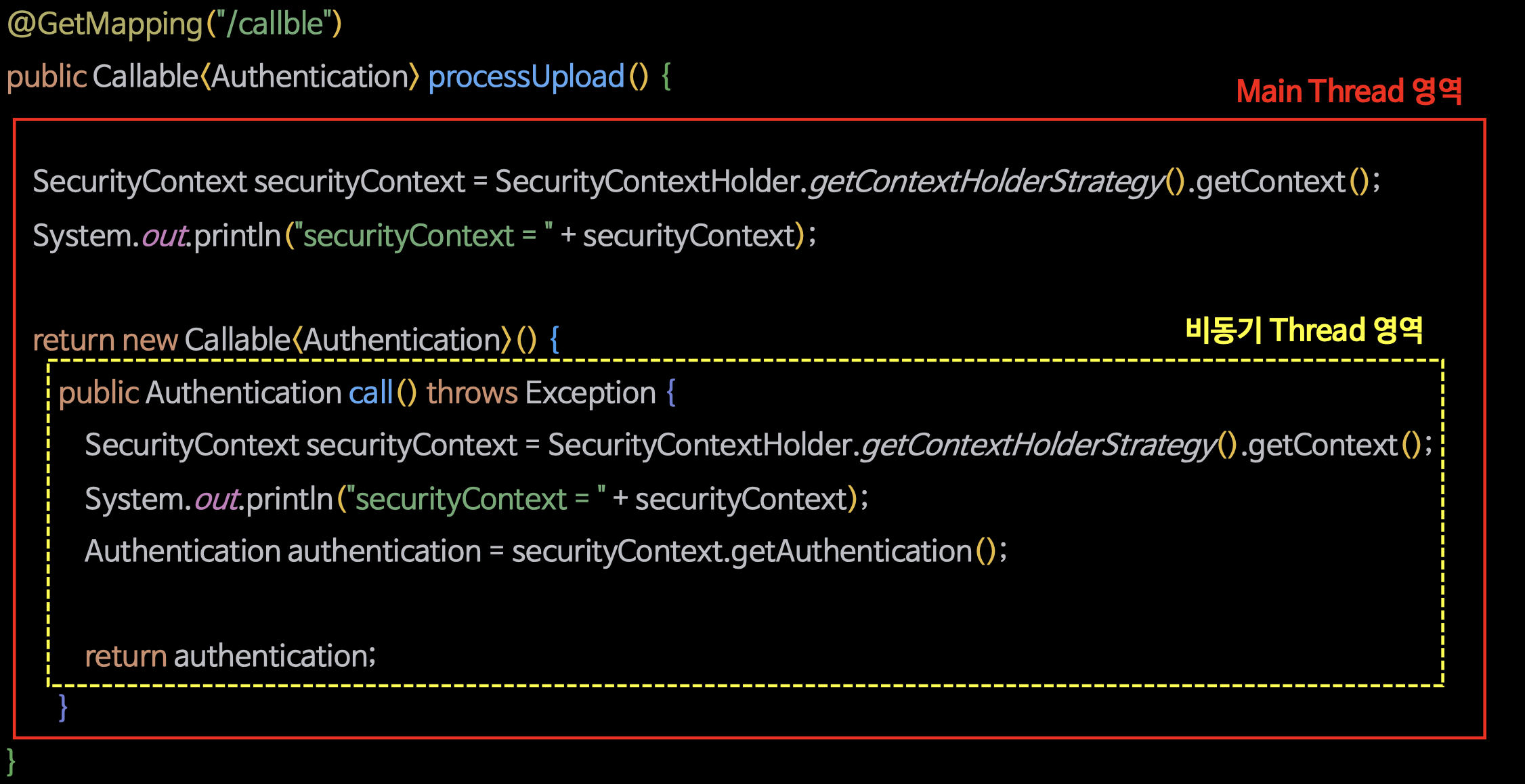
- 비동기 스레드가 수행하는 Callable 영역 내에서 자신의 ThreadLocal 에 저장된 SecurityContext 를 참조할 수 있으며 이는 부모 스레드가 가지고 있는 SecurityContext 와 동일한 객체이다
- @Async 나 다른 비동기 기술은 스프링 시큐리티와 통합되어 있지 않기 때문에 비동기 스레드에 SecurityContext 가 적용되지 않는다 즉 Callable 영역내에서만 가능하다.
- Callable으로 반환이 되어야한다
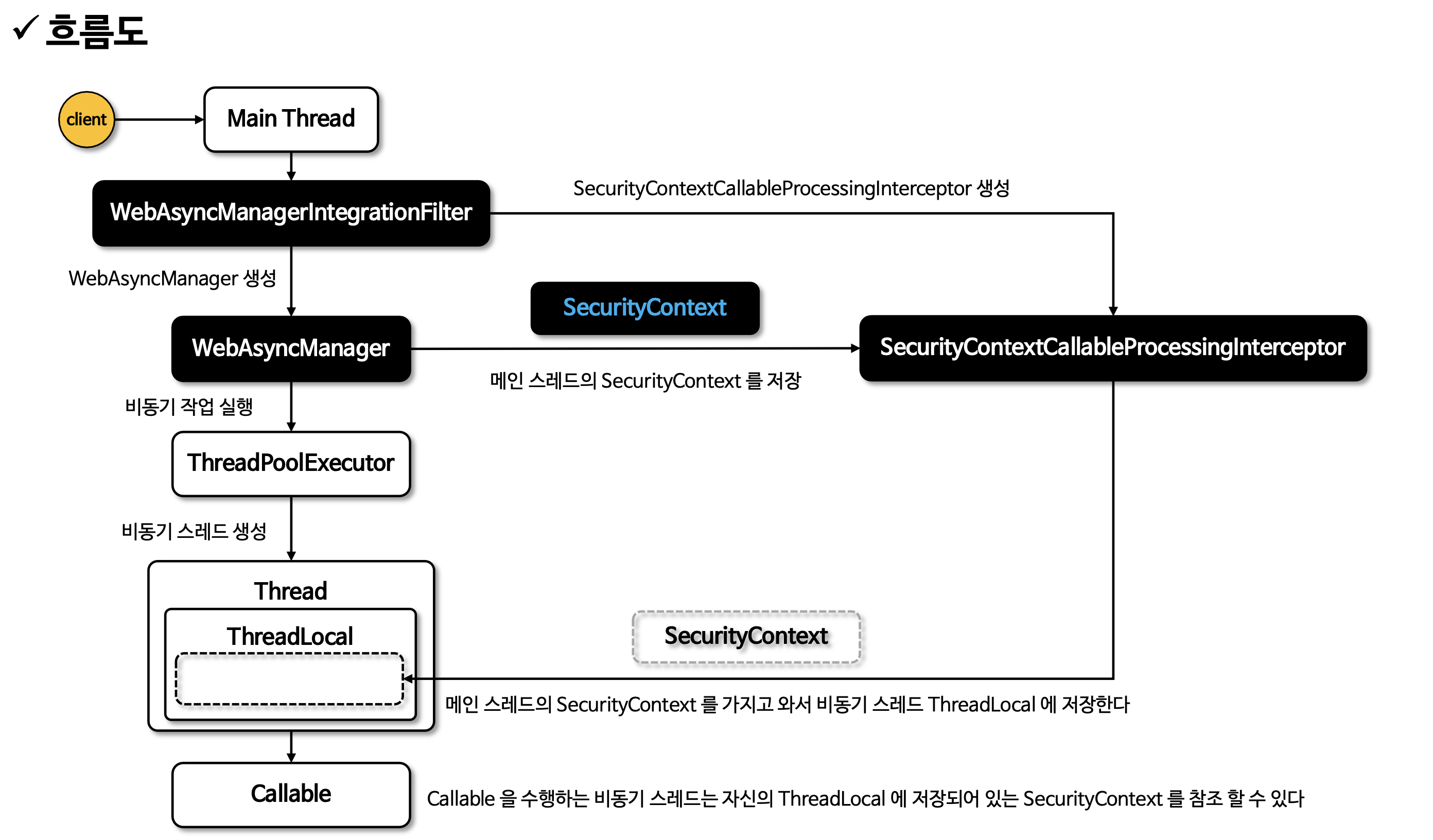
- mode 설정으로 Callable 구현하지 않고 SecurityContext객체를 공유할 수 있도록 사용할 수 있다
SecurityContextHolder.setStrategyName(SecurityContextHolder.MODE_INHERITABLETHREADLOCAL);