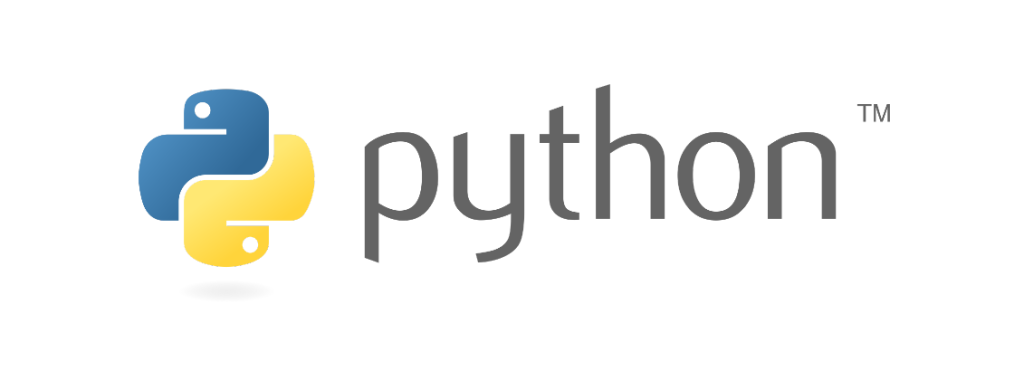
📌Set
- Mutable
- Unordered
- Iterable
📌Set Method
s = {2, 9, 4}
s.add(3)
print(s)
s = {2, 9, 3}
s.update('a', 'bd')
print(s)
.remove(x)
set에서 x
제거, 없으면 KeyError
s = {2, 9, 3}
s.remove(3)
print(s)
.discard(x)
set에서 x
제거, 없어도 에러 X
s = {2, 9, 3}
s.discard(10)
print(s)
s.discard(2)
print(s)
.pop()
임의의 원소 제거, 비어있는 경우 KeyError
s = {2, 9, 3}
s.pop()
print(s)
📌Dictionary
- key, value로 구성되어 있음
- Mutable
- Unordered
- Iterable
📌Dictionary Method
.get(key [, default])
key
를 통해 value
를 가져옴
key
가 딕셔너리에 없어도 KeyError 발생 X, None
반환
dic = {'a': 10, 'b':[1, 2], 'c':{3:'b'}}
dic.get('b')
.pop(key [, default])
key가 딕셔너리에 있으면 제거하고 반환
- 없으면 default 반환, default 없으면 KeyError
dic = {'a': 10, 'b':[1, 2], 'c':{3:'b'}}
dic.pop('b')
print(dic)
.update(key = 'value')
값을 제공하는 key, value로 갱신
dic = {'a': 10, 'b':[1, 2], 'c':{3:'b'}}
dic.update(b=30)
print(dic)
dic = {'a': 10, 'b':[1, 2], 'c':{3:'b'}}
dic.keys()
dic = {'a': 10, 'b':[1, 2], 'c':{3:'b'}}
dic.values()
.items()
(key, value)
로 구성된 결과
dic = {'a': 10, 'b':[1, 2], 'c':{3:'b'}}
dic.items()