자료구조 문제풀이
- 리스트 - 약수, 소수
inputNum = int(input('1보다 큰 정수 입력 : '))
listA = []
listB = []
for n in range(1, inputNum+1):
if inputNum % n == 0:
listA.append(n)
for num in range (2, inputNum+1):
flag = True
for n in range (2, num):
if num % n == 0:
flag = False
break
if flag:
listB.append(num)
print(f'{inputNum}의 약수: {listA}')
print(f'{inputNum}까지의 소수: {listB}')
- 리스트 - 짝수, 홀수
import random
randomList = random.sample(range(1,101), 10)
evens = []
odds = []
for n in randomList:
if n % 2 == 0:
evens.append(n)
else:
odds.append(n)
print(randomList)
print('짝수({}개): {}'.format(len(evens), evens))
print('홀수({}개): {}'.format(len(odds), odds))
- 리스트 - 공원입장료
import random
visitors = []
for n in range(100):
visitors.append(random.randint(1, 100))
group1, group2, group3, group4, group5 = 0,0,0,0,0
for age in visitors:
if age <= 7:
group1 += 1
elif age <= 13:
group2 += 1
elif age <= 19:
group3 += 1
elif age <= 64:
group4 += 1
else:
group5 +=1
group1Price = group1 * 0
group2Price = group2 * 200
group3Price = group3 * 300
group4Price = group4 * 500
group5Price = group5 * 0
print('유아:\t{}명\t\t{}원'.format(group1, group1Price))
print('소아:\t{}명\t\t{}원'.format(group2, group2Price))
print('청소년:\t{}명\t\t{}원'.format(group3, group3Price))
print('성인:\t{}명\t\t{}원'.format(group4, group4Price))
print('어르신:\t{}명\t\t{}원'.format(group5, group5Price))
total = group1+group2+group3+group4+group5
totalPrice = group1Price+group2Price+group3Price+group4Price+group5Price
print('-'*30)
print('총:\t\t{}명\t{}원'.format(total, format(totalPrice, ',')))
- 리스트 - 정렬
friends = []
for i in range(5):
friends.append(input('친구 이름: '))
print('친구들 : {}'.format(friends))
friends.sort()
print('오름차순: {}'.format(friends))
friends.sort(reverse=True)
print('내림차순: {}'.format(friends))
- 리스트 - 중복 제거
numbers = [2, 22, 7, 8, 9, 2, 7, 3, 5, 2, 7, 1, 3]
print(numbers)
idx = 0
while True:
if idx >= len(numbers):
break
if numbers.count(numbers[idx]) >= 2:
numbers.remove(numbers[idx])
continue
idx +=1
print(numbers)
- 리스트 - 경우의 수
numbers = [4, 6, 7, 9]
result = []
for n1 in numbers:
for n2 in numbers:
if n1 == n2: continue
for n3 in numbers:
if n1 == n3 or n2 == n3: continue
result.append([n1, n2, n3])
print(len(result))
print(result)
- 튜플 - 학점
scores = ((3.7, 4.2), (2.9, 4.3), (4.1, 4.2))
sum = 0
for n1,n2 in scores:
sum += n1+n2
print('3학년 총학점: {}'.format(sum))
print('3학년 평균: {}'.format(round(sum / len(scores)/2, 1)))
print('-'*30)
targetScore = 4.0 * 8
needScores = round(targetScore - sum, 1)
print('4학년 때 받아야 하는 학점: {}'.format(needScores))
needScore1 = round(needScores/2, 1)
print('4학년 한 학기 최소학점: {}'.format(needScore1))
scores = list(scores)
scores.append((needScore1, needScore1))
scores = tuple(scores)
print(scores)
- 튜플 - 합집합, 교집합
tuple1 = (1,3,2,6,12,5,7,8)
tuple2 = (0,5,2,9,8,6,17,3)
tupleHap = list(tuple1)
tupleGyo = []
for n in tuple2:
if n not in tuple1:
tupleHap.append(n)
else:
tupleGyo.append(n)
tupleHap = tuple(sorted(tupleHap))
tupleGyo = tuple(sorted(tupleGyo))
print(tupleHap)
print(tupleGyo)
- 튜플 - 슬라이싱, 최대값, 최소값
numbers = (8.7, 9.0, 9.1, 9.2, 8.6, 9.3, 7.9, 8.1, 8.3)
print(numbers)
print(f'index 0~3\t : {numbers[:4]}')
print(f'index 2~4\t : {numbers[2:5]}')
print(f'index 3~끝\t : {numbers[3:]}')
print(f'index 2~뒤에서2 : {numbers[2:-1]}')
print(f'index 0~뒤에에서3 : {numbers[:-2]}')
max = max(numbers)
maxIndex = numbers.index(max)
print(max, end='')
print('\t(index {})'.format(maxIndex))
min = min(numbers)
minIndex = numbers.index(min)
print(min, end='')
print('\t(index {})'.format(minIndex))
- 튜플 - 시험점수, 학점
korScore = int(input('국어 점수: '))
engScore = int(input('영어 점수: '))
matScore = int(input('수학 점수: '))
sciScore = int(input('과학 점수: '))
hisScore = int(input('국사 점수: '))
scores = ({'kor':korScore},
{'eng':engScore},
{'mat':matScore},
{'sci':sciScore},
{'his':hisScore})
print(scores)
for item in scores:
for key in item.keys():
if item[key] >= 90:
item[key] = 'A'
elif item[key] >= 80:
item[key] = 'B'
elif item[key] >= 70:
item[key] = 'C'
elif item[key] >= 60:
item[key] = 'D'
else:
item[key] = 'F'
print(scores)
- 튜플 - 튜플 내 딕셔너리 정렬
fruits = ({'수박':8}, {'포도':13}, {'참외':12}, {'사과':17}, {'자두':19}, {'자몽':15})
fruits = list(fruits)
print(fruits)
cIdx = 0; nIdx = 1
eIdx = len(fruits) -1
flag = True
while flag:
cDic = fruits[cIdx]
nDic = fruits[nIdx]
cDicCnt = list(cDic.values())[0]
nDicCnt = list(nDic.values())[0]
if nDicCnt < cDicCnt:
fruits.insert(cIdx, fruits.pop(nIdx))
nIdx = cIdx + 1
continue
nIdx +=1
if nIdx > eIdx:
cIdx +=1
nIdx = cIdx +1
if cIdx == 5:
flag = False
print(tuple(fruits))
- 튜플 - 학생 수
students = ({'cls01':18},
{'cls02':21},
{'cls03':20},
{'cls04':19},
{'cls05':22},
{'cls06':20},
{'cls07':23},
{'cls08':17})
print(students)
sum = 0
max = 0; min = 0
maxClass= 0; minClass = 0
for item in students:
for key in item.keys():
sum += item[key]
if item[key] > max:
max = item[key]
maxClass = key
elif min==0 or item[key]<min:
min = item[key]
minClass = key
avg = round(sum / len(students),1)
for item in students:
for key in item.keys():
item[key] -= avg
print('전체 학생 수: {}명'.format(sum))
print('평균 학생 수: {}명'.format(avg))
print('학생 수가 가장 많은 학급: {}반 ({}명)'.format(maxClass, max))
print('학생 수가 가장 적은 학급: {}반 ({}명)'.format(minClass, min))
print(students)
- 딕셔너리 - 과목 점수 딕셔너리에 저장
subject = ['국어','영어','수학','과학','국사']
scores = {}
for s in subject:
score = int(input(s + ' 점수: '))
scores[s] = score
print(scores)
- 딕셔너리 - 로그인 프로그램
members = {'a1':'aaa', 'a2':'bbb','a3':'ccc'}
inputId = input('ID 입력: ')
inputPw = input('PW 입력: ')
if inputId not in members.keys():
print('아이디 확인!')
else:
if inputPw != members[inputId]:
print('비밀번호 확인!')
else:
print('로그인 성공!!')
- 딕셔너리 - n각형 내각의 합과 내각
dic = {}
for n in range(3,11):
hap = 180 * (n-2)
ang = int(hap/n)
dic[n] = [hap, ang]
print(dic)
- 딕셔너리 - 약수
dic = {}
for number in range(2,11):
tempList = []
for n in range(1, number+1):
if number % n == 0:
tempList.append(n)
dic[number] = tempList
print(dic)
- 딕셔너리 - 단어 구분
aboutPython = '파이썬은 1991년 프로그래머인 귀도 반 로섬이 발표한 고급 프로그래밍 언어이다.'
wordList = aboutPython.split()
print(wordList)
dic = {}
for idx, word in enumerate(wordList):
dic[idx] = word
print(dic)
- 딕셔너리 - 비속어 대체
txt = '강도는 서로 쪼개다, 짭새를 보고 빠르게 따돌리며 먹튀했다.'
words = {'꺼지다':'가다',
'쩔다':'엄청나다',
'짭새':'경찰관',
'꼽사리':'중간에 낀 사람',
'먹튀':'먹고 도망',
'지린다':'겁을 먹다',
'쪼개다':'웃다',
'뒷담 까다':'험담하다'}
keys = words.keys()
for key in keys:
if key in txt:
print(f'{key} : {words[key]}')
txt = txt.replace(key,words[key])
print()
print(txt)
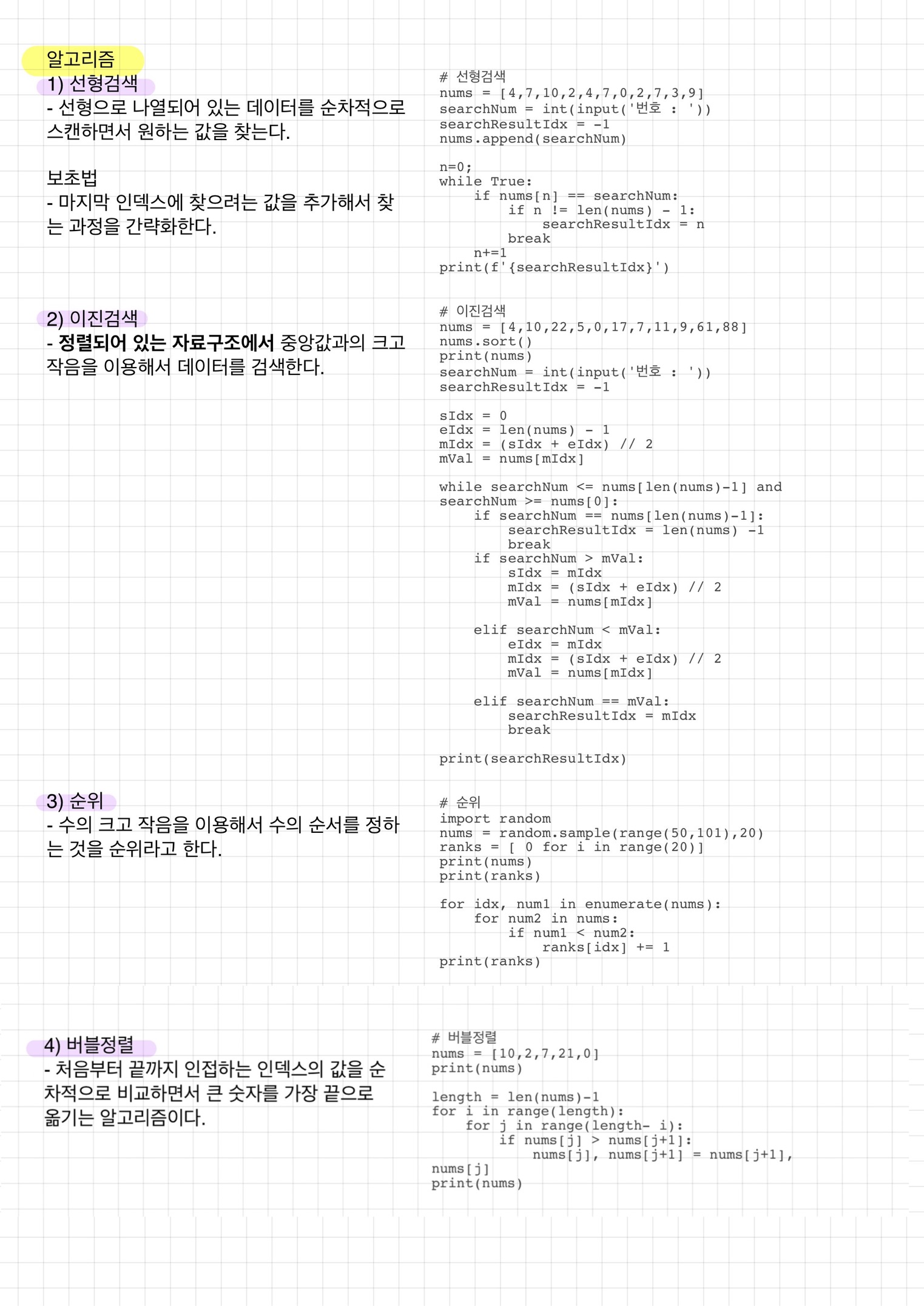