* Web Component
긴 HTML을 함수처럼 재사용 가능하게 커스텀 태그로 만듬
// HTML
<custom-input name="id"></custom-input>
<custom-input name="pw"></custom-input>
class customInputClass extends HTMLElement {
connectedCallback() {
let name = this.getAttribute('name');
this.innerHTML = '<label>${name}-input</label><input>'
}
}
customElements.define("custom-input", customInputClass);
}
attribute 변경될때 특정 코드 실행
class customInputClass extends HTMLElement {
connectedCallback() {
let name = this.getAttribute('name');
this.innerHTML = `<label>${name}-input</label><input>`
}
static get observedAttributes() {
return ['name']
}
attributeChangedCallback() {
console.log(this.getAttribute('name'));
}
}
customElements.define("custom-input", customInputClass);
* Shadow DOM
일반적으로는 볼 수 없는 숨겨진 HTML
class customInputClass extends HTMLElement {
connectedCallback() {
this.attachShadow({mode : 'open'});
this.shadowRoot.innerHTML = `<label>input-label</label><input>
<style> label { color : red } </style>`
}
}
customElements.define("custom-input", customInputClass);
* HTML 모듈화
WebComponent + Shadow DOM
사용하는 이유: 외부코드에 영향을 주지 않고 스타일링 가능
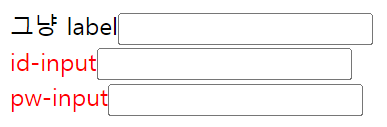
// html
<label>그냥 label</label><input>
<custom-input name="id"></custom-input>
<custom-input name="pw"></custom-input>
class customInputClass extends HTMLElement {
connectedCallback() {
let name = this.getAttribute('name');
this.attachShadow({mode : 'open'});
this.shadowRoot.innerHTML = `<label>${name}-input</label><input>
<style>label { color: red }</style}`
}
static get observedAttributes() {
return ['name']
}
attributeChangedCallback() {
console.log(this.getAttribute('name'));
}
}
customElements.define("custom-input", customInputClass);
}
태그 가독성을 높혀줄 <template>
: HTML 임시 보관함
직접 사용하지 않으면 body에 출력되지 않음
// html
<template id="temp">
<label>~~</label><input>
<style>label { color: red }</style>
</template>
this.shadowRoot.append(temp.content.cloneNode(true))
let label = this.shadowRoot.querySelector('label');
label.addEventListener('click', function () {
console.log('click했습니다')
})
* Optional Chaining
?. : ?.앞에 나오는 값이 null, undefined면 뒤에 코드 실행하지 않고 undefined 리턴
중첩된 Object 자료에서 자료를 추출 시, reference 에러 없이 안전하게 추출 가능
var user = {
name : 'kim',
};
console.log(user.age?.value);
??: "nullish coalescing"
?? 앞에 나오는 값이 null, undefined면 뒤에 코드 실행
var user = {
name : 'kim',
};
console.log(user.age??"이 값은 없습니다")