1. 로그인 요청
2. passport.authenticate 메서드 호출
- back/routes/user.js
- 해당 api 라우터에서 passport.authenticate 실행
passport.authenticate('local', (err, user, info) => { ...
3. 로그인 전략 수행
- back/passport/local.js
- 프론트에서 전달한 userId, password를 usernameField, passwordField필드에 지정하고 전략 수행
passport.use(new LocalStrategy({
usernameField : 'userId',
passwordField : 'password',
}, async (id, password, done) => {
try {
const user = await db.User.findOne({ where : { userId }});
if (!user) {
return done(null, false, { reason : '존재하지 않는 사용자입니다!' });
}
const result = await bcrypt.compare(password, user.password);
if (result) {
return done(null, user);
}
return done(null, false, { reason : '비밀번호가 틀립니다.' })
} catch (e) {
console.error(e);
return done(e);
}
}));
4. 로그인 성공 시 사용자 정보 객체와 함께 req.login 호출
- back/routes/user.js
- 로그인 성공 시 다시 라우터로 들어가서 (err, user, info) => 콜백 부분이 실행되어 프론트로 로그인된 유저 정보 보내줌
passport.authenticate('local', (err, user, info) => {
if (err) {
console.error(err);
next(err);
}
if (info) {
return res.status(401).send(info.reason);
}
return req.logIn(user, (loginErr) => {
if (loginErr) {
return next(loginErr);
}
const filteredUser = Object.assign({}, user);
delete filteredUser.password;
return res.json(filteredUser);
});
})(req, res, next);
4-1.req.logIn 성공 시 serialize 실행
- req.logIn이 성공하면 성공한 유저 id를 세션에 저장, 쿠키 만들어 서버 메모리에 저장
passport.serializeUser((user, done) => {
return done(null, user.id);
});
5. front / user-saga 에서 axios 결과값 result.data로 전달
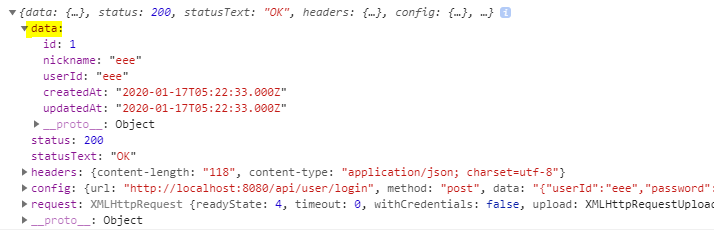
function loginAPI(loginData) {
// 서버 요청
return axios.post('http://localhost:8080/api/user/login', loginData);
}
function* login(action) {
try {
const result = yield call(loginAPI, action.data);
yield put({
type : LOG_IN_SUCCESS,
data : result.data,
});
} catch (e) {
console.error(e);
yield put({
type : LOG_IN_FAILURE
});
}
}
6. front / user-reducer 에서 me 값에 result.data 넣기 (req.user정보)
const reducer = (state = initialState, action) => {
switch (action.type) {
...
case LOG_IN_SUCCESS : {
return {
...state,
isLoggingIn : false,
isLoggedIn : true,
me : action.data,
}
}