1. Flask를 생성하고 아래 코드들을 요청(request), 다시강조하는(redirect)
2. Flask에 이름을 준다 (생성)
3. 제일 마지막에 읽을수 있게 app.run() 표기 (읽기)
4. topics를 설정하며 ID값을 설정
5. ID값들을 브라우저에 표기한다. ID의 기본값은 None
5-1. 각 ID값을 처리할수 있는 담당자(route)를 배정한다(Routing)
6. body에서 어떻게 보이게할지 설정
7. read라는 라우트를 설정
8. read 라우트를 어떻게 보이게할지 설정
9. get코드를 post로 변환하는 과정: form태그 활용
10. create_process 추가: 위에 있는 코드들을 읽기위해
11. request form추가 : body 값을 알기위해
newtopic은 새로운 topic을 추가하는 과정
global nextId가 있어야지 쓰기가 가능해진다(없다면 읽기만 가능)
12. delete 버튼 추가 : 추가된 ID값을 삭제가능함
from flask import Flask, request, redirect
import sqlite3
app = Flask(__name__)
topics = [
{"id":1, "title":"html", "body":"html is ...."},
{"id":2, "title":"css", "body":"css is ...."},
{"id":3, "title":"js", "body":"js is ...."}
]
def template(content, id=None):
conn = sqlite3.connect('db.sqlite3')
cs = conn.cursor()
cs.execute('SELECT * FROM topics')
topics = cs.fetchall()
conn.close()
print('topics',topics)
liTags = ''
for topic in topics:
liTags = liTags + f'<li><a href="/read/{topic[0]}/">{topic[1]}</a></li>'
return f'''
<html>
<head>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js" integrity="sha384-ka7Sk0Gln4gmtz2MlQnikT1wXgYsOg+OMhuP+IlRH9sENBO0LRn5q+8nbTov4+1p" crossorigin="anonymous"></script>
<style>
h1{{
border-bottom:10px solid yellow;
padding:30px;
}}
h1>a{{
text-decoration:none;
}}
</style>
</head>
<body class="container">
<input type="button" value="night" onclick="
document.querySelector('body').style.backgroundColor = 'black';
document.querySelector('body').style.color = 'white';
">
<h1><a href="/">WEB</a></h1>
<ol>
{liTags}
</ol>
{content}
<form action="/delete/{id}/" method="POST">
<div class="btn-group" role="group" aria-label="Basic example">
<a href="/create/" class="btn btn-info">create</a>
<input type="submit" value="delete" class="btn btn-dark">
</div>
</form>
</body>
</html>
'''
@app.route("/")
def index():
return template('<h2>Welcome</h2>Hello, WEB!')
@app.route("/read/<int:id>/")
def read(id):
conn = sqlite3.connect('db.sqlite3')
cs = conn.cursor()
cs.execute('SELECT * FROM topics WHERE id=?', (id,))
topic = cs.fetchone()
conn.close()
title = topic[1]
body = topic[2]
return template(f'<h2>{title}</h2>{body}', id)
@app.route('/create/')
def create():
content = '''
<form action="/create_process/" method="POST">
<p><input type="text" name="title" placeholder="title"></p>
<p><textarea name="body" placeholder="body"></textarea></p>
<p><input type="submit" value="create"></p>
</form>
'''
return template(content)
@app.route('/create_process/', methods=['POST'])
def create_process():
title = request.form['title']
body = request.form['body']
conn = sqlite3.connect('db.sqlite3')
cs = conn.cursor()
cs.execute('INSERT INTO topics (title, body) VALUES(?,?)',(title,body))
id = cs.lastrowid
conn.commit()
conn.close()
return redirect(f'/read/{id}/')
@app.route('/delete/<int:id>/', methods=['POST'])
def delete(id):
conn = sqlite3.connect('db.sqlite3')
cs = conn.cursor()
cs.execute('DELETE FROM topics WHERE id = ?',(id,))
conn.commit()
conn.close()
return redirect('/')
# # @app.route('/update/')
# # def update():
# # return 'Update'
app.run()
"delete"키를 세부메뉴 진입시에만 나타나게하기 = Contect UI
sqlite파일명을 생성했으나 중복코드로 인하여 생성이 안됨!
from flask import Flask, request, redirect
import sqlite3
app = Flask(__name__)
topics = [
{"id":1, "title":"html", "body":"html is ...."},
{"id":2, "title":"css", "body":"css is ...."},
{"id":3, "title":"js", "body":"js is ...."}
]
nextId = 4
def template(content, id=None):
conn = sqlite3.connect('db.sqlite3')
cs = conn.cursor()
cs.execute('SELECT * FROM topics')
topics = cs.fetchall()
conn.close()
print('topics',topics)
liTags = ''
for topic in topics:
liTags = liTags + f'<li><a href="/read/{topic[0]}/">{topic[1]}</a></li>'
return f'''
<html>
<body>
<h1><a href="/">WEB</a></h1>
<ol>
{liTags}
</ol>
{content}
<ul>
<li><a href="/create/">create</a></li>
<li>
<form action="/delete/{id}/" method="POST">
<input type="submit" value="delete">
</form>
</li>
</ul>
</body>
</html>
'''
@app.route("/")
def index():
return template('<h2>Welcome</h2>Hello, WEB!')
@app.route("/read/<int:id>/")
def read(id):
conn = sqlite3.connect('db.sqlite3')
cs = conn.cursor()
cs.execute('SELECT * FROM topics WHERE id=?', (id,))
topic = cs.fetchone()
> print('topic', topic.title, topic.body) = 삭제하기!!
conn.close()
title = topic[1]
body = topic[2]
return template(f'<h2>{title}</h2>{body}', id)
@app.route('/create/')
def create():
content = '''
<form action="/create_process/" method="POST">
<p><input type="text" name="title" placeholder="title"></p>
<p><textarea name="body" placeholder="body"></textarea></p>
<p><input type="submit" value="create"></p>
</form>
'''
return template(content)
@app.route('/create_process/', methods=['POST'])
def create_process():
global nextId
title = request.form['title']
body = request.form['body']
newTopic = {"id":nextId, "title": title, "body": body}
topics.append(newTopic)
nextId = nextId + 1
return redirect(f'/read/{nextId-1}/')
@app.route('/delete/<int:id>/', methods=['POST'])
def delete(id):
for topic in topics:
if topic['id'] == id:
topics.remove(topic)
break;
return redirect('/')
# # @app.route('/update/')
# # def update():
# # return 'Update'
app.run()
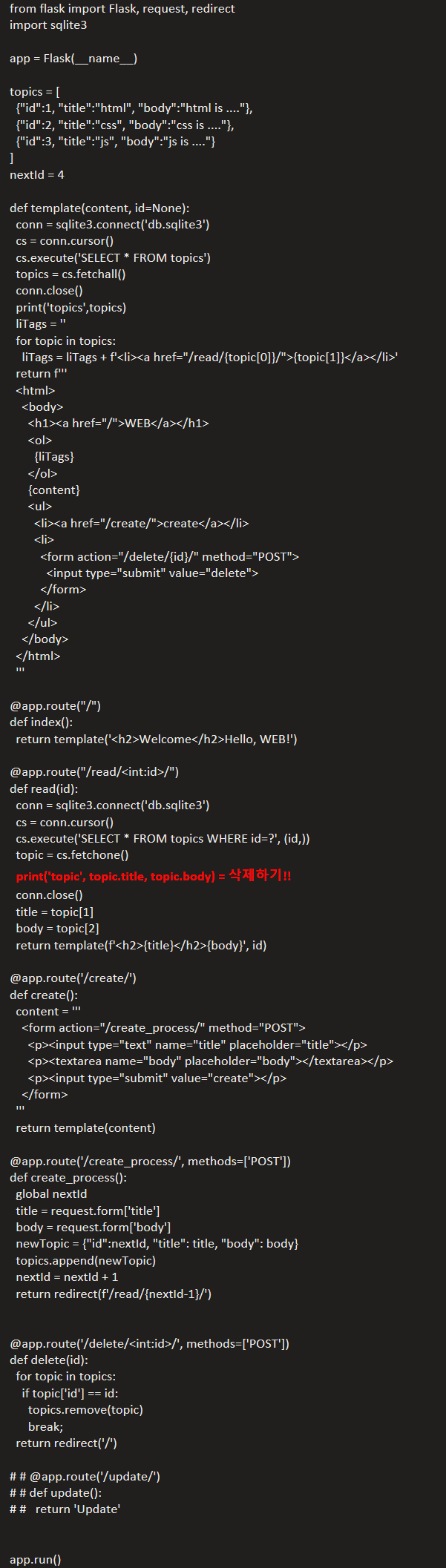
브라우저 내 코딩을 4주간 교육받았다. 난생처음 코딩을 접하면서 처음에는 '무슨 단어들이 저렇게 왜? 나열되있지??'에서 끝났었는데, 직접해보니 답을 얻을수 있었다. 흥미롭고 재미있었다!! 4월도 힘내봅시다!