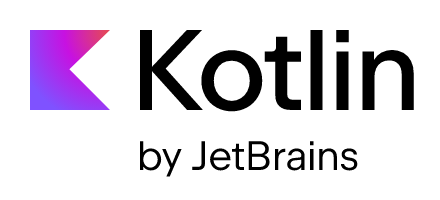
Thread 생성
Thread Class 상속
class SimpleThread : Thread() {
override fun run() {
println("Class Thread ${Thread.currentThread()}")
}
}
func main() {
val thread = SimpleThread()
thread.start()
}
Runnable Interface
class SimpleRunnable : Runnable {
override fun run() {
println("Interface Thread ${Thread.currentThread()}")
}
}
func main() {
val runnable = SimpleRunnable()
val thread2 = Thread(runnable)
thread2.start()
}
익명 객체 생성
object : Thread() {
override fun run() {
println("object thread : ${Thread.currentThread()}")
}
}.start()
람다식 생성
Thread {
println("Lambda Thread : ${Thread.currentThread()}")
}.start()
Thread Pool
newFixedThreadPool()
- 자주 재사용되는 스레드를 이용하기 위해 미리 생성된 스레드풀에서 스레드 이용
- 8개의 스레드로 특정 백그라운드 서비스를 하도록 만든다고 했을 때
val myService: ExecutorService = Executors.newFixedThreadPool(8)
...
myService.submit {
processItem(item)
}
...