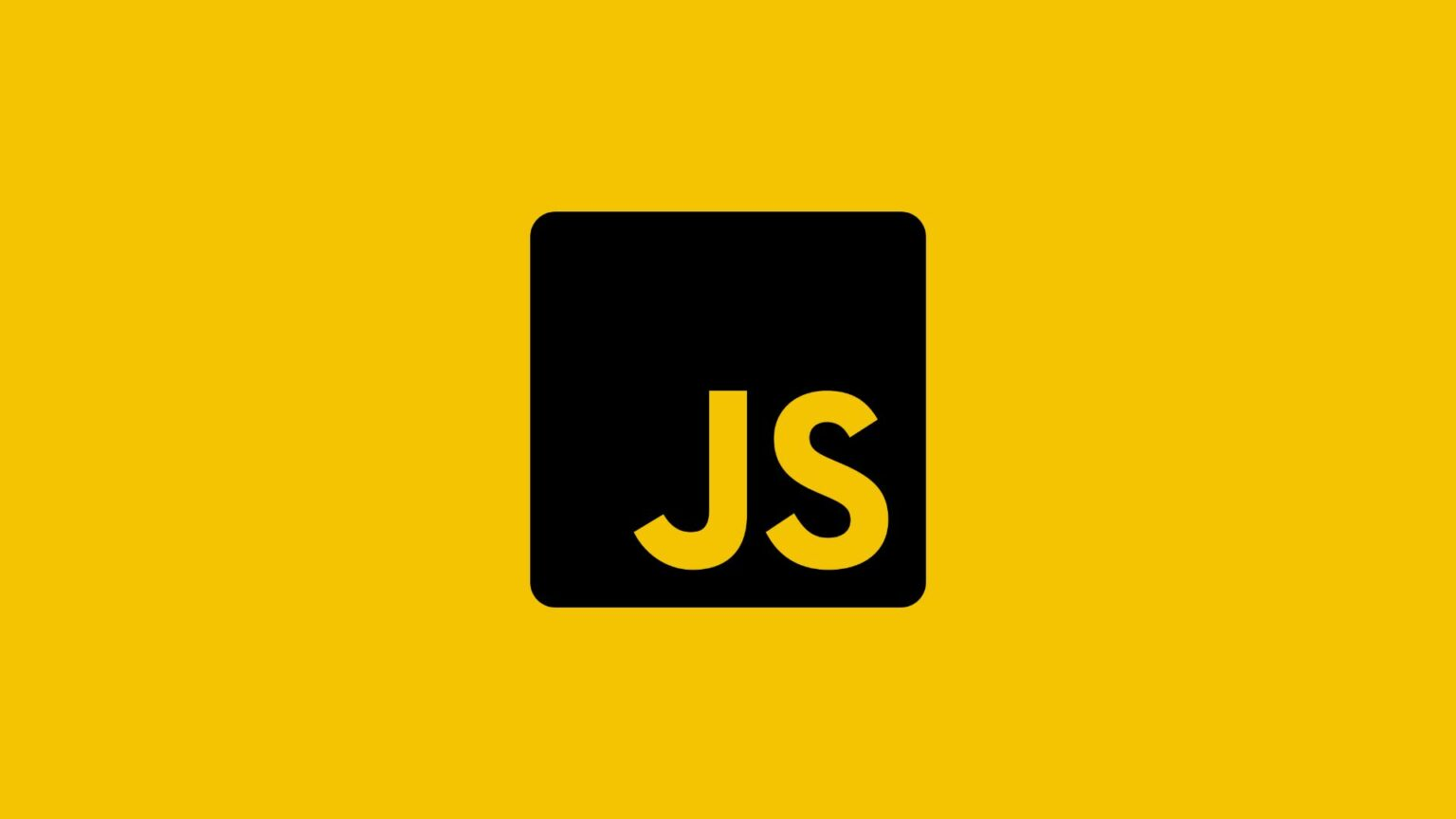
map() 함수
- 배열을 순회하며 지정된 콜백 함수를 적용하여 각 요소를 변환하고
- 그 변환된 값을 모아서 새로운 배열로 반환
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(function(number) {
return number * 2;
});
console.log(doubledNumbers);
const users = [
{id: 1, name: "Alice"},
{id: 2, name: "Bob"},
{id: 3, name: "Charlie"}
];
const names = users.map(function(user) {
return user.name;
});
console.log(names);
forEach()
- 배열을 순회해서 처리하는 데 사용되는 메서드
- 배열의 각 요소에 대해 주어진 함수를 순서대로 한 번씩 실행
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(function(number) {
console.log(number);
});
const numbers = [1, 2, 3, 4, 5];
let sum = 0;
numbers.forEach(function(number) {
sum += number;
});
console.log(sum);
const people = [
{name: 'Alice', age: 30},
{name: 'Bob', age: 25},
{name: 'Charlie', age: 35}
];
people.forEach(function(person) {
person.age += 5;
});
console.log(people);
스프레드(…) 문법
- 여러 값들의 집합을 펼쳐서(spread) , 즉 전개해서 개별적인 항목으로 만드는 기능을 제공
console.log(...[1, 2, 3]);
console.log(..."Hello World");
console.log(...new Map([["apple", "red"], ["banana', "yellow"]]));
console.log(...new Set(["a", "b", "c"]));
function fn(arg1, arg2, arg3) {
console.log(...arguments);
};
fn("arg_1", "arg_2", "arg_3");
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const mergedArray = [...arr1, ...arr2];
console.log(mergedArray);
const extendedArray = [...arr1, 4, 5];
console.log(extendedArray);