Servlet & Jstl
- Model1 방식 : 비지니스 로직과 디자인이 복합적으로 같이 들어가있는 형태 (소규모 프로젝트에 적당)
- Model2 방식 : 프로젝트 규모가 커지면서 나온 방식, 비지니스 로직과 디자인을 분리한 형태
로직처리 : servlet (Model)
디자인과 출력 : jsp,html (View)
- 이때 JSP 에서 출력시 자바코드를 사용하지 않고 주로 JSTL 태그를 이용해서 request 나 session 에 저장된 데이터에 접근을 한다
- JSTL은 라이브러리이기 때문에 사용 전에 core를 header에 추가해주어야 함
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt" %>
EL(Expression Language)
${ name }
Tags | Description |
---|
<c:set> | 변수명에 값을 할당 |
<c:out> | 값을 출력 |
<c:if> | Java의 if 조건문과 동일 |
<c:choose> | Java의 조건문과 동일 |
<c:when> | 조건문의 조건 |
<c:otherwise> | 위의 모든 조건에서 배제 시 조건 |
<c:forEach> | Java의 for 반복문과 동일 |
Operators
- 대체로 java의 연산자와 유사하며 혼용 가능
- Relational Operators (관계연산자)
Java op | JSTL op |
---|
< | lt |
> | gt |
≤ | le |
≥ | ge |
== | eq |
≠ | ne |
- Arithmetic Operators (산술연산자)
Java op | JSTL op |
---|
+ | |
- | |
* | |
/ | div |
% | mod (modulo) |
- Logical Operators (논리연산자)
- Null : operator returning true or false value (Only in Null Table, Java op is unavailable)
- There is No Increment/Decrement Operators in JSTL
<c:set var="su1" value="7"/>
<c:set var="su2" value="4"/>
<table>
<tr><td> ${su1+su2} </td></tr>
<tr><td> ${su1-su2} </td></tr>
<tr><td> ${su1*su2} </td></tr>
<tr><td> ${su1 div su2} </td></tr>
<tr><td> ${su1 mod su2} </td></tr>
</table>
<c:out var="${su1}"/>
<c:set var="su1" value="${su1+1}"/>
<c:set var="today" value="<%=new Date() %>"/>
<fmt:formatDate value="${today }" pattern="yyyy-MM-dd HH:mm"/><br>
<fmt:formatDate value="${today }" pattern="yyyy-MM-dd a hh:mm"/><br>
<fmt:formatDate value="${today }" pattern="yyyy-MM-dd HH:mm EEE"/><br>
<fmt:formatDate value="${today }" pattern="yyyy-MM-dd HH:mm EEEE"/><br>
<fmt:formatDate value="${today }" pattern="yyyy년MM월dd일"/><br>
<c:set var="money" value="1230976342"/>
<c:set var="num1" value="142.19654"/>
<fmt:formatNumber value="${money }" type="number"/>
<fmt:formatNumber value="${money }" type="currency"/>
<fmt:formatNumber value="${num1 }" pattern="0.00"/>
<fmt:formatNumber value="1.5" pattern="0.00"/>
<fmt:formatNumber value="1.5" pattern="0.##"/>
- <c:if>태그의 test 속성의 값으로 조건문의 조건 입력
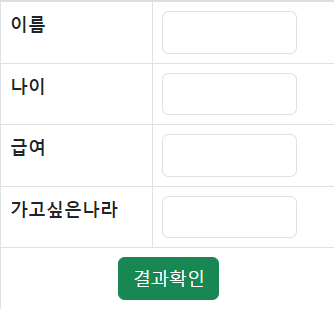
- 위의 <input>태그에 값을 입력하여, 이름이 입력된 경우 아래와 같이 출력되도록 하는 코드
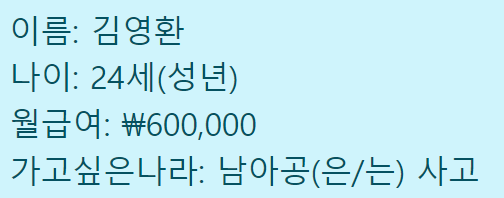
<c:if test="${!empty param.name }">
<div class="alert alert-info" style="width: 600px">
<h3>이름: ${param.name }</h3>
<h3>나이: ${param.age }세(
<c:choose>
<c:when test="${param.age<20 }">미성년자</c:when>
<c:otherwise>성년</c:otherwise>
</c:choose>
)</h3>
<h3>월급여: <fmt:formatNumber value="${param.pay }" type="currency"/></h3>
<h3>가고싶은나라: ${param.nara }(은/는)
<c:choose
<c:when test="${param.nara=='소말리아' }">영환고향</c:when>
<c:when test="${param.nara=='나이지리아' }">영환주소</c:when>
<c:otherwise>사고</c:otherwise></c:choose>
</h3>
</div>
</c:if>
- 위의 코드에서 변수명 앞의 param은 jsp의 request.getParameter()와 동일한 기능이며 (현 페이지로 넘어오는 변수와 값을 호출하는 기능)
- jsp와 같이 <form>태그의 submit 버튼을 이용하여 값을 넘겨주며, 이를 호출 시 <input>의 name 속성 값으로 호출 (위 코드의 param.name, age, nara는 넘겨주는 페이지에서 name 속성 값에 해당)
- <c:forEach>태그의 begin, end 속성으로 시작 값과 끝 값을 지정
- var 속성으로 변수명을 지정하여, 이를 통해 반복 출력
<c:forEach var="a" begin="1" end="10">
${a }
</c:forEach>
<c:forEach var="a" begin="0" end="30" step="3">
${a }
</c:forEach>
- varStatus 속성으로 순서, 인덱스 값 지정 가능
- varStatus의 count값은 무조건 1번부터 시작하여 1씩 증가
- varStatus의 index값은 items 속성 값 객체의 실제 인덱스 값 출력
<table>
<tr>
<th>No.</th><th>index</th>
</tr>
<c:forEach var="s" items="${list }" varStatus="i">
<tr>
<td>${i.count }</td>
<td>${i.index }</td>
</tr>
</c:forEach>
</table>
<table>
<tr>
<th>No.</th><th>index</th>
</tr>
<c:forEach var="s" items="${list }" varStatus="i" begin="2" end="4">
<tr>
<td>${i.count }</td>
<td>${i.index }</td>
</tr>
</c:forEach>
</table>
RequestScope & SessionScope
- 변수명에 할당하는 값이 문자열, 숫자가 아닌 List, session, 배열에 저장된 값이라도 ${ }로 출력 가능
- ${ }로 출력 시 ‘requestScope.(변수명)’으로 표기하지만 일반적으로 requestScope는 생략 (Java처럼 request.getAttribute()로 호출하지 않아도 됨)
- 반면 ‘sessionScope.(변수명)’의 sessionScope도 생략 가능하지만 구분을 위해 생략하지 않음
<%
List<String> list=new ArrayList<>();
list.add("red");
list.add("orange");
list.add("magenta");
list.add("blue");
list.add("pink");
request.setAttribute("list", list);
request.setAttribute("totalCount", list.size());
session.setAttribute("id", "angel");
%>
<h3>list에는 총 ${totalCount }개의 색상이 들어있다</h3>
<h3>list에는 총 ${requestScope.totalCount }개의 색상이 들어있다</h3>
<h3>세션 아이디 출력</h3>
<h4>현재 로그인한 아이디는 ${sessionScope.id }</h4>
<hr>
<h3>list전체 출력</h3>
<table>
<tr>
<th>No.</th><th>index</th><th>color</th>
</tr>
<c:forEach var="s" items="${list }" varStatus="i">
<tr>
<td>${i.count }</td>
<td>${i.index }</td>
<td>
<b style="color: ${s}">${s }</b>
</td>
</tr>
</c:forEach>
</table>
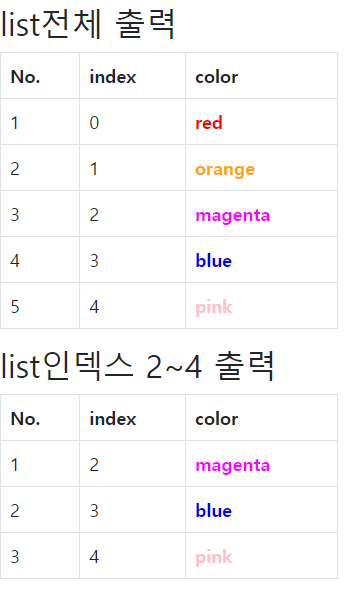
- 위와 유사하지만 List가 아닌 배열도 동일한 방법으로 반복문에 적용 가능
<%
String [] myColor={"red","magenta","orange","blue","pink","yellow","aqua"};
%>
<c:set var="mycolor" value="<%=myColor %>"/>
<table>
<caption align="top">전체값 출력</caption>
<tr>
<th>index</th><th>count</th><th>color</th>
</tr>
<c:forEach var="a" items="${mycolor }" varStatus="i" begin="2" end="5">
<tr align="center">
<td>${i.index }</td>
<td>${i.count }</td>
<td style="background-color: ${a}">${a }</td>
</tr>
</c:forEach>
</table>
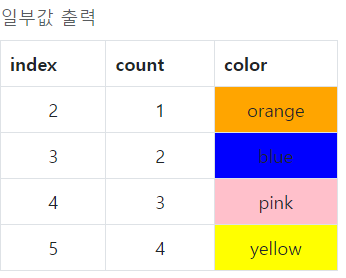
- Java의 split, tokenizer과 같은 기능
- items 속성에 문자열을 입력 후 delims 속성에 분할할 regex 입력
<c:set var="msg" value="민규,성신,영환,성경,희찬"/>
${msg }<br>
<c:forTokens var="s" items="${msg }" delims="," varStatus="i">
<h3>${i.count }: ${s }</h3>
</c:forTokens>