문제 설명
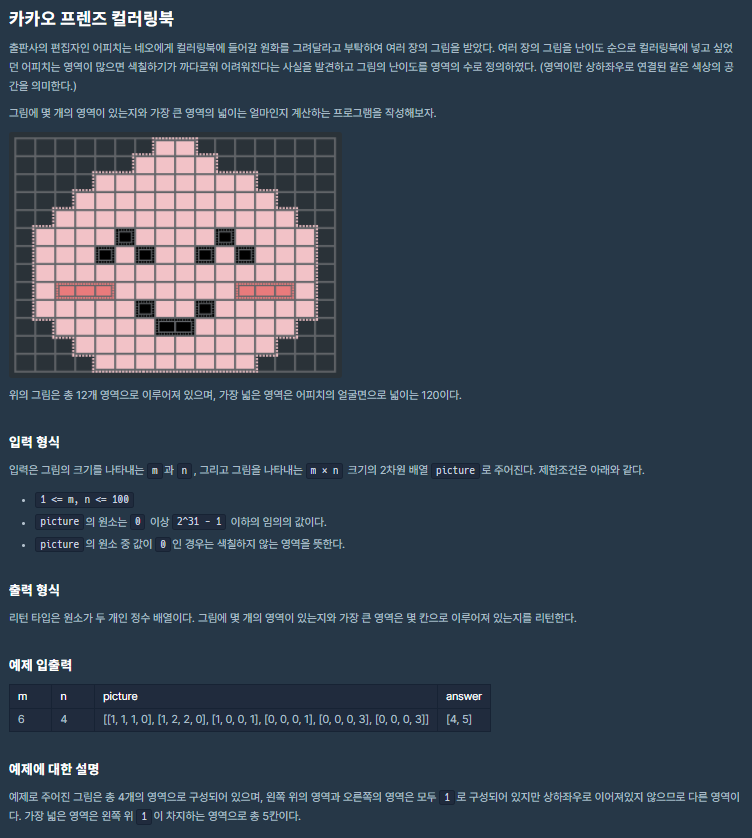
문제 풀이 - 해결방법
- BFS를 활용하여 문제를 해결한다.
- 자료구조 Queue를 사용한다.
문제 풀이 - 코드
import java.util.LinkedList;
import java.util.Queue;
class Solution {
static int[] dx = {1, -1, 0, 0};
static int[] dy = {0, 0, 1, -1};
static class Node{
int x;
int y;
public Node(int x, int y){
this.x = x;
this.y = y;
}
}
static Queue<Node> queue = new LinkedList<Node>();
static boolean[][] isVisited;
static int size = 0;
public int[] solution(int m, int n, int[][] picture) {
int numberOfArea = 0;
int maxSizeOfOneArea = 0;
isVisited = new boolean[m][n];
for(int row = 0; row < m; row++){
for(int col = 0; col < n; col++){
if(picture[row][col] != 0 && isVisited[row][col] != true){
size = 1;
bfs(picture, row, col, m, n);
numberOfArea++;
if(maxSizeOfOneArea < size){
maxSizeOfOneArea = size;
}
}
}
}
int[] answer = new int[2];
answer[0] = numberOfArea;
answer[1] = maxSizeOfOneArea;
return answer;
}
static void bfs(int[][] picture, int x, int y, int m, int n)
{
queue.add(new Node(x, y));
isVisited[x][y] = true;
while(!queue.isEmpty()){
Node curr = queue.poll();
for(int dir = 0; dir < 4; dir++){
int nx = curr.x + dx[dir];
int ny = curr.y + dy[dir];
if(0 <= nx && nx < m && 0 <= ny && ny < n){
if(picture[nx][ny] == picture[x][y] && isVisited[nx][ny] != true){
queue.add(new Node(nx, ny));
isVisited[nx][ny] = true;
size++;
}
}
}
}
}
}