๋ฌธ์ 1
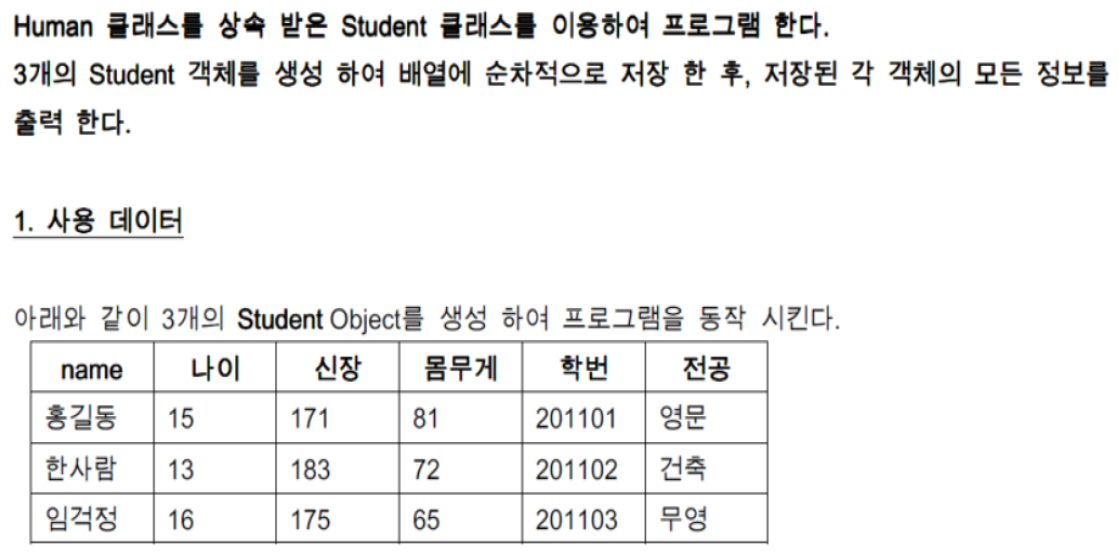
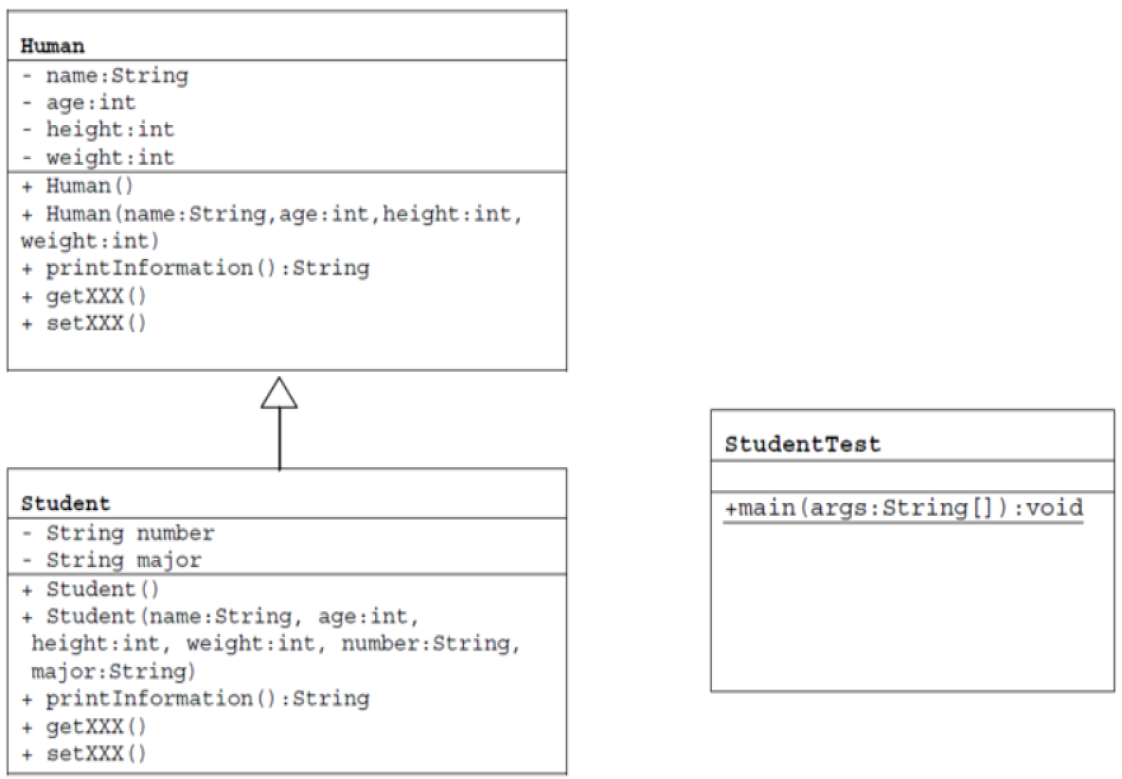
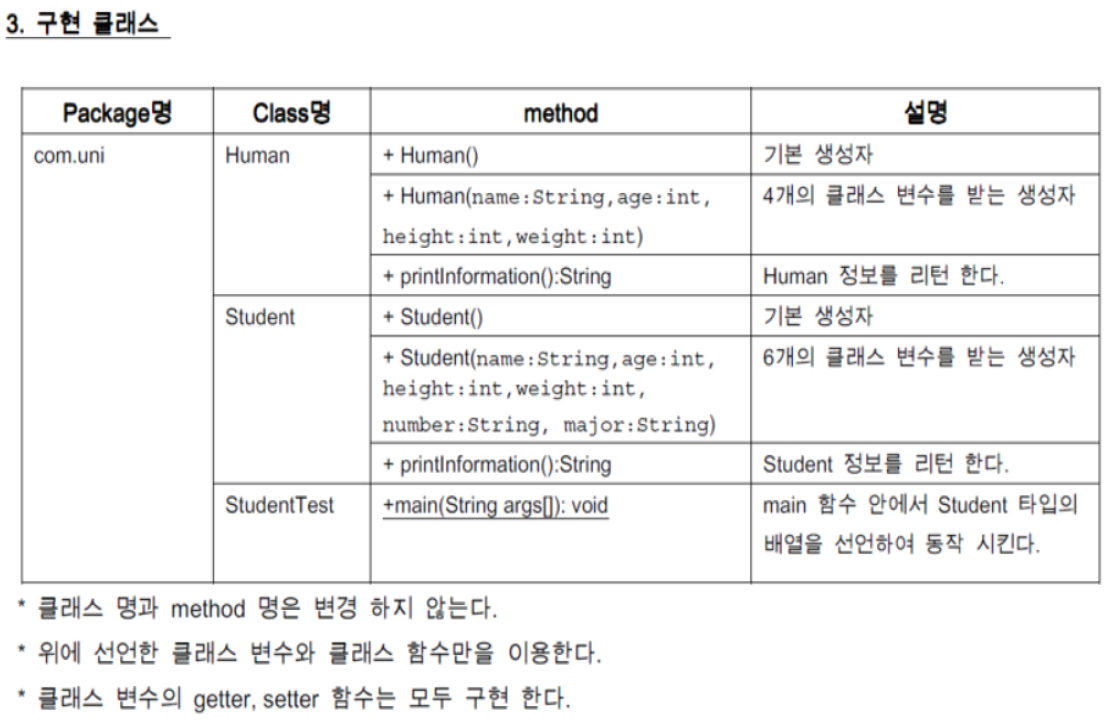
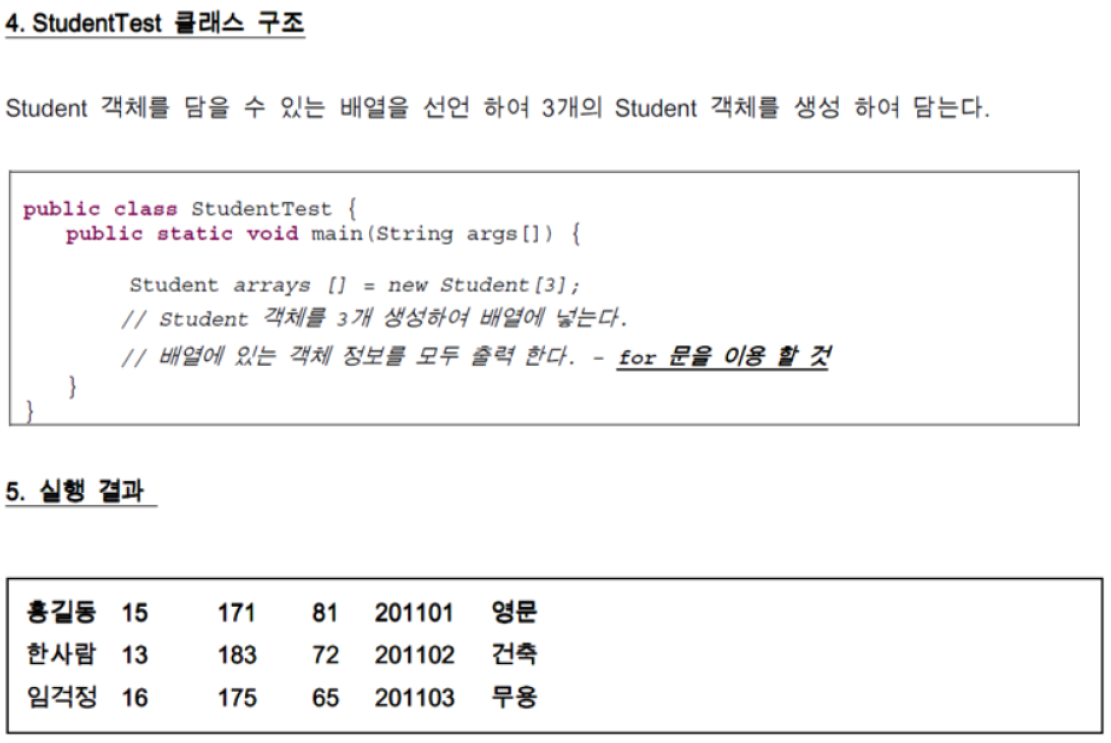
package com.uni;
public class Human {
private String name;
private int age;
private int height;
private int weight;
public Human() {
}
public Human(String name, int age, int height, int weight) {
this.name = name;
this.age = age;
this.height = height;
this.weight = weight;
}
public String printInformation() {
return this.getName() + " " + this.getAge() + " "+ this.getHeight() + " "+ this.getWeight();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
}
public int getWeight() {
return weight;
}
public void setWeight(int weight) {
this.weight = weight;
}
}
package com.uni;
public class Student extends Human {
private String number;
private String major;
public Student() {
}
public Student(String name, int age, int height, int weight, String number, String major) {
super(name, age, height, weight);
this.number = number;
this.major = major;
}
public String printInformation() {
return this.getName() + " " + this.getAge() + " "+ this.getHeight() + " "+ this.getWeight() + " "+ this.getNumber() + " "+ this.getMajor();
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getMajor() {
return major;
}
public void setMajor(String major) {
this.major = major;
}
}
package com.uni;
public class StudentTest {
public static void main(String[] args) {
Student arrays [] = new Student[3];
arrays[0] = new Student("ํ๊ธธ๋", 15, 171, 81, "201101", "์๋ฌธ");
arrays[1] = new Student("ํ์ฌ๋", 13, 183, 72, "201102", "๊ฑด์ถ");
arrays[2] = new Student("ํ๊ธธ๋", 16, 175, 65, "201103", "๋ฌด์ฉ");
for(int i=0; i<3; i++) {
System.out.println(arrays[i].printInformation());
}
}
}
์คํ ๊ฒฐ๊ณผ : ํ๊ธธ๋ 15 171 81 201101 ์๋ฌธ
ํ์ฌ๋ 13 183 72 201102 ๊ฑด์ถ
ํ๊ธธ๋ 16 175 65 201103 ๋ฌด์ฉ
๋ฌธ์ 2
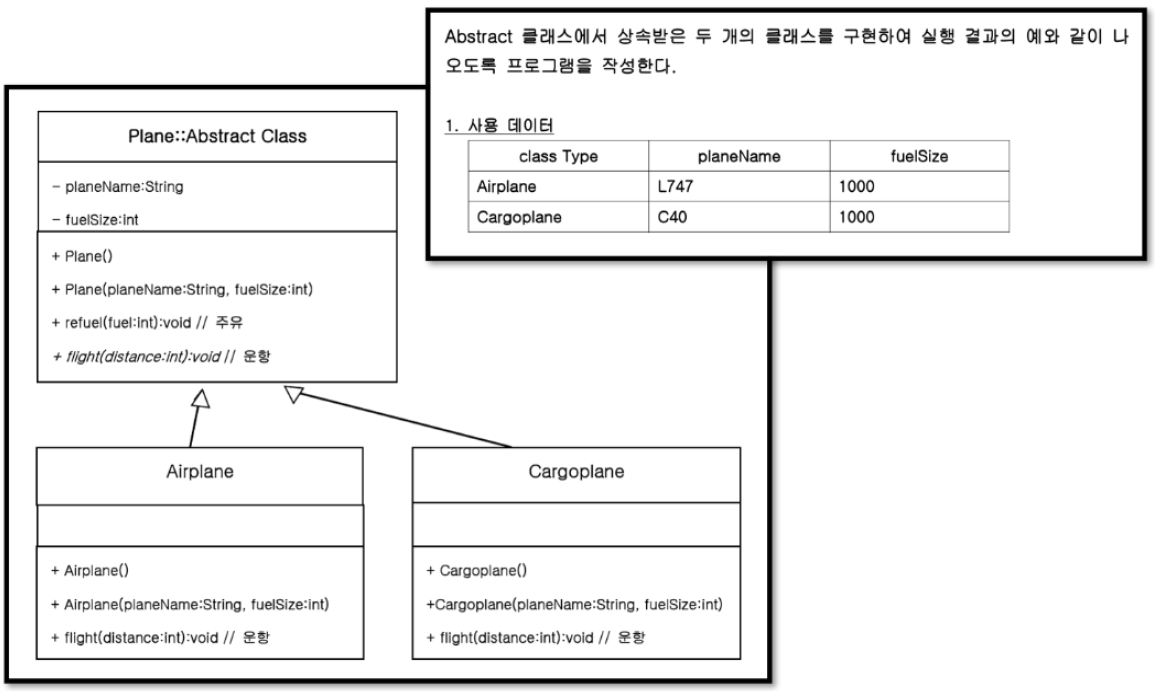
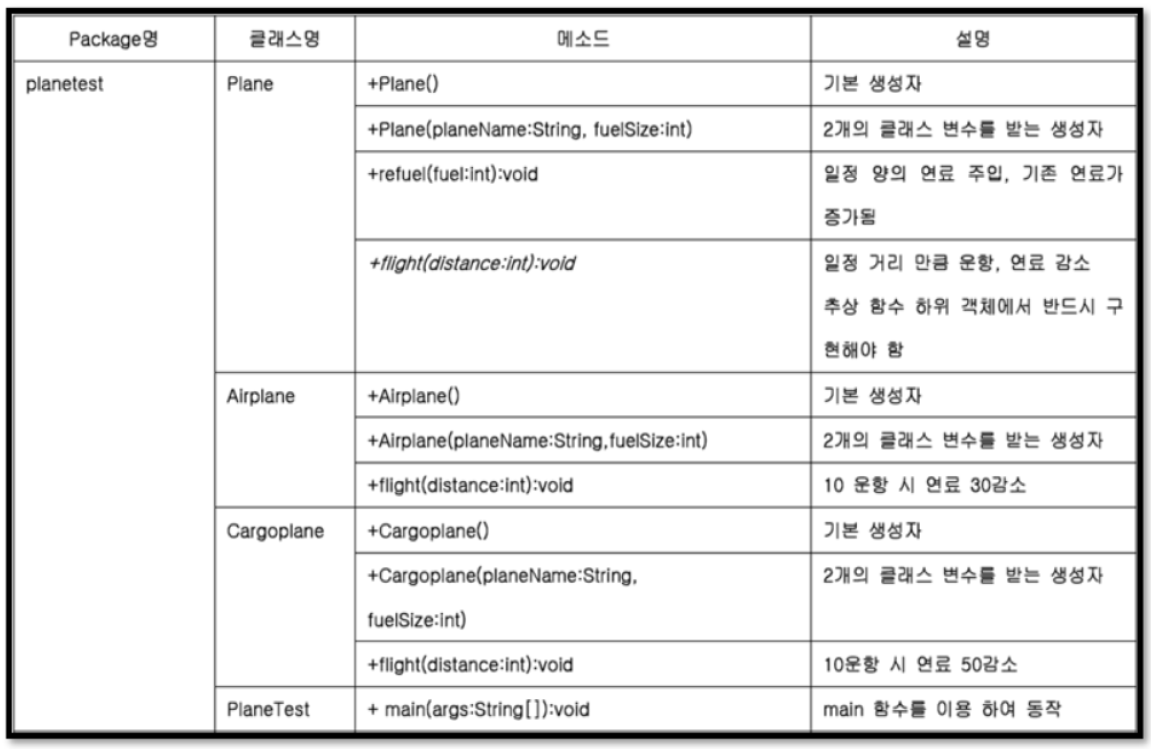
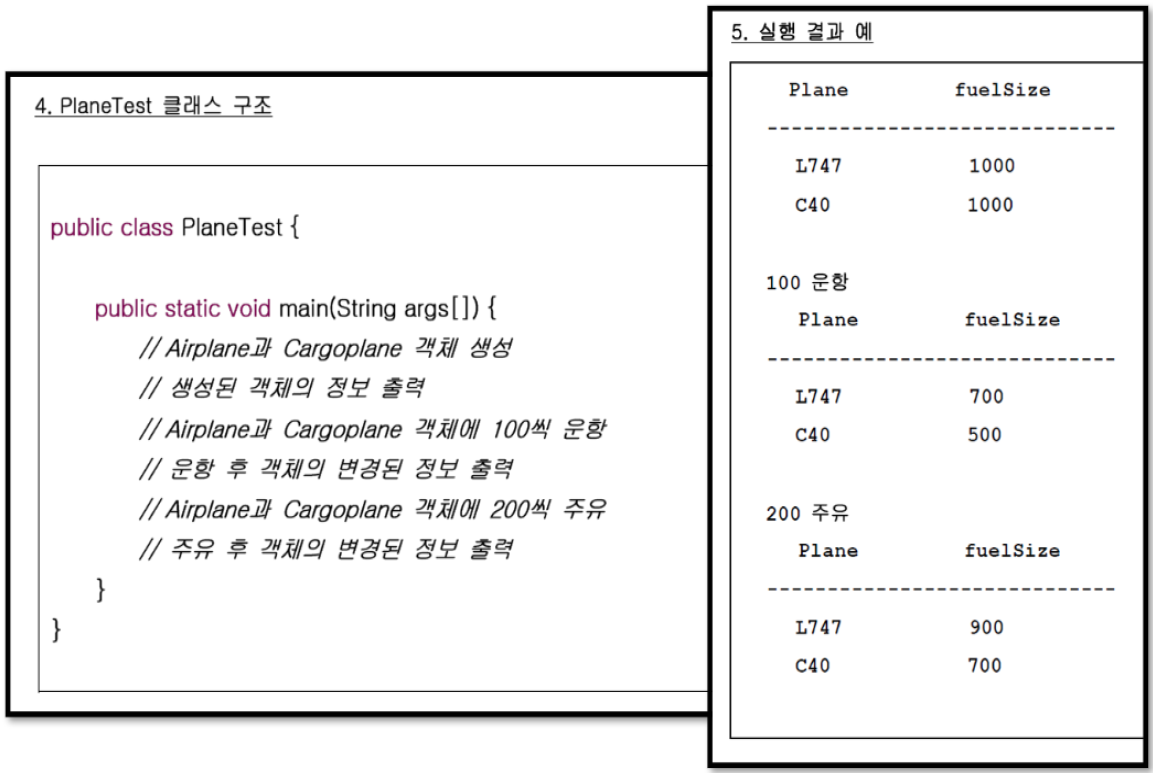
package planetest;
public abstract class Plane {
private String planeName;
private int fuelSize;
public Plane() {
}
public Plane(String planeName, int fuelSize) {
super();
this.planeName = planeName;
this.fuelSize = fuelSize;
}
public void refuel(int fuel) {
this.fuelSize += fuel;
}
public abstract void flight(int distance);
public String getPlaneName() {
return planeName;
}
public void setPlaneName(String planeName) {
this.planeName = planeName;
}
public int getFuelSize() {
return fuelSize;
}
public void setFuelSize(int fuelSize) {
this.fuelSize = fuelSize;
}
}
package planetest;
public class Airplane extends Plane{
Airplane() {
}
Airplane(String planeName, int fuelSize) {
super(planeName, fuelSize);
}
@Override
public void flight(int distance) {
int curFuleSize = this.getFuelSize();
this.setFuelSize(curFuleSize - distance * 3);
}
}
package planetest;
public class Cargoplane extends Plane{
Cargoplane() {
}
Cargoplane(String planeName, int fuelSize) {
super(planeName, fuelSize);
}
@Override
public void flight(int distance) {
int curFuleSize = this.getFuelSize();
this.setFuelSize(curFuleSize - distance * 5);
}
}
package planetest;
public class PlaneTest {
public static void main(String[] args) {
Airplane airplane = new Airplane("L747", 1000);
Cargoplane cargoplane = new Cargoplane("C40", 1000);
System.out.println(" Plane fuelSize");
System.out.println("---------------------");
System.out.println(" " + airplane.getPlaneName() + " " + airplane.getFuelSize());
System.out.println(" " + cargoplane.getPlaneName() + " " + cargoplane.getFuelSize());
System.out.println();
System.out.println("100 ์ดํญ");
airplane.flight(100);
cargoplane.flight(100);
System.out.println(" Plane fuelSize");
System.out.println("---------------------");
System.out.println(" " + airplane.getPlaneName() + " " + airplane.getFuelSize());
System.out.println(" " + cargoplane.getPlaneName() + " " + cargoplane.getFuelSize());
System.out.println();
System.out.println("200 ์ฃผ์ ");
airplane.refuel(200);
cargoplane.refuel(200);
System.out.println(" Plane fuelSize");
System.out.println("---------------------");
System.out.println(" " + airplane.getPlaneName() + " " + airplane.getFuelSize());
System.out.println(" " + cargoplane.getPlaneName() + " " + cargoplane.getFuelSize());
}
}
๋ฌธ์ 3
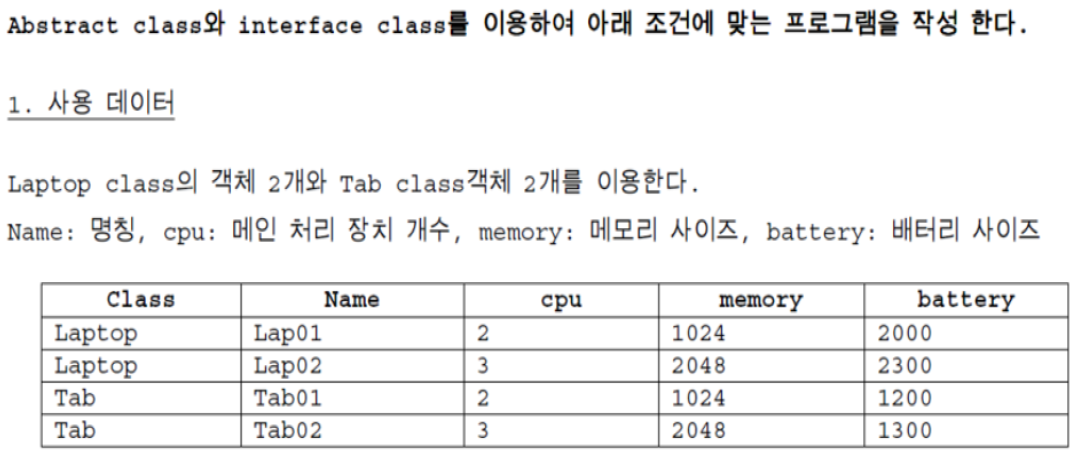
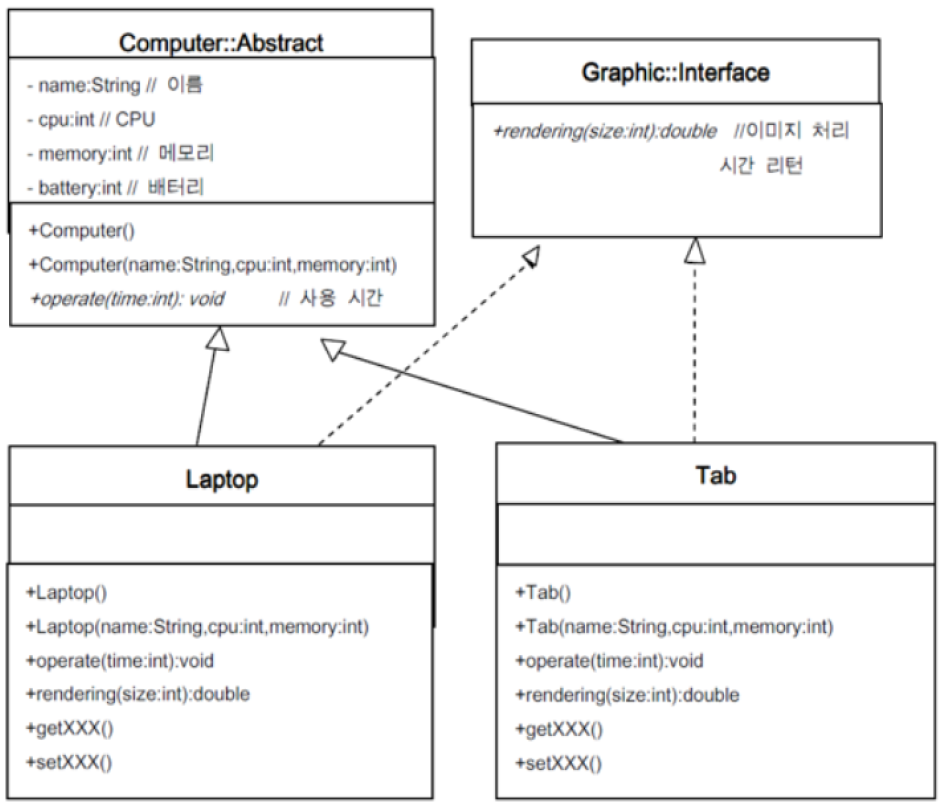
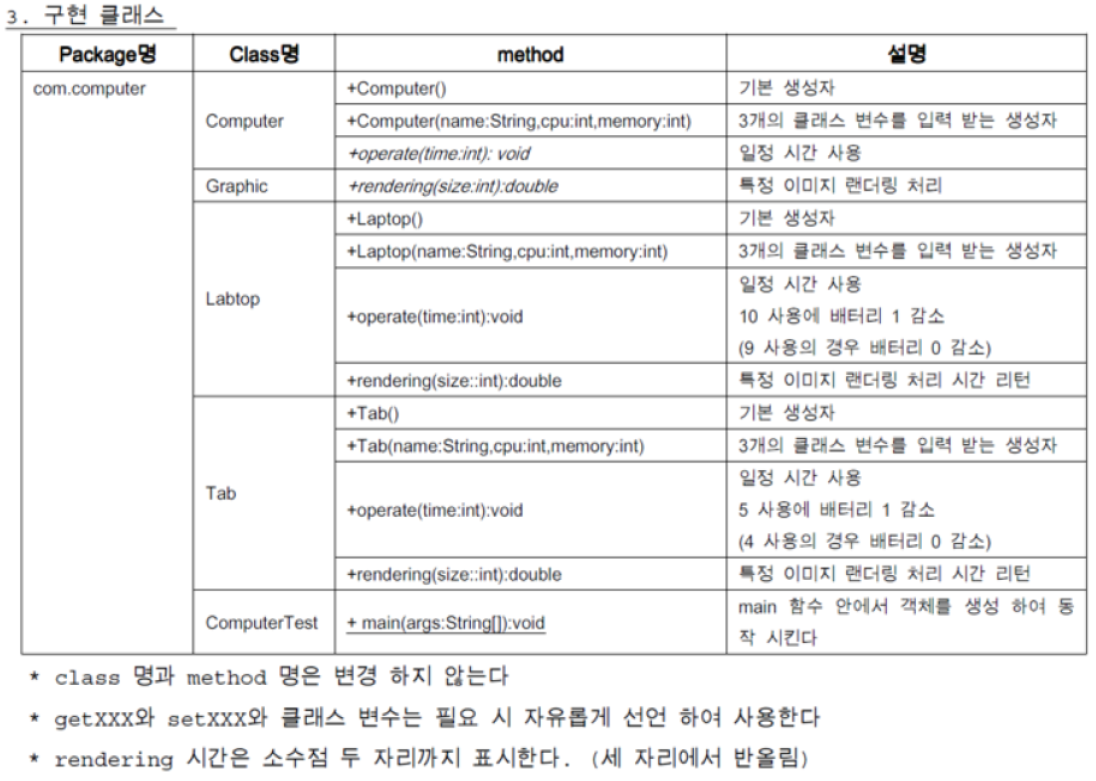
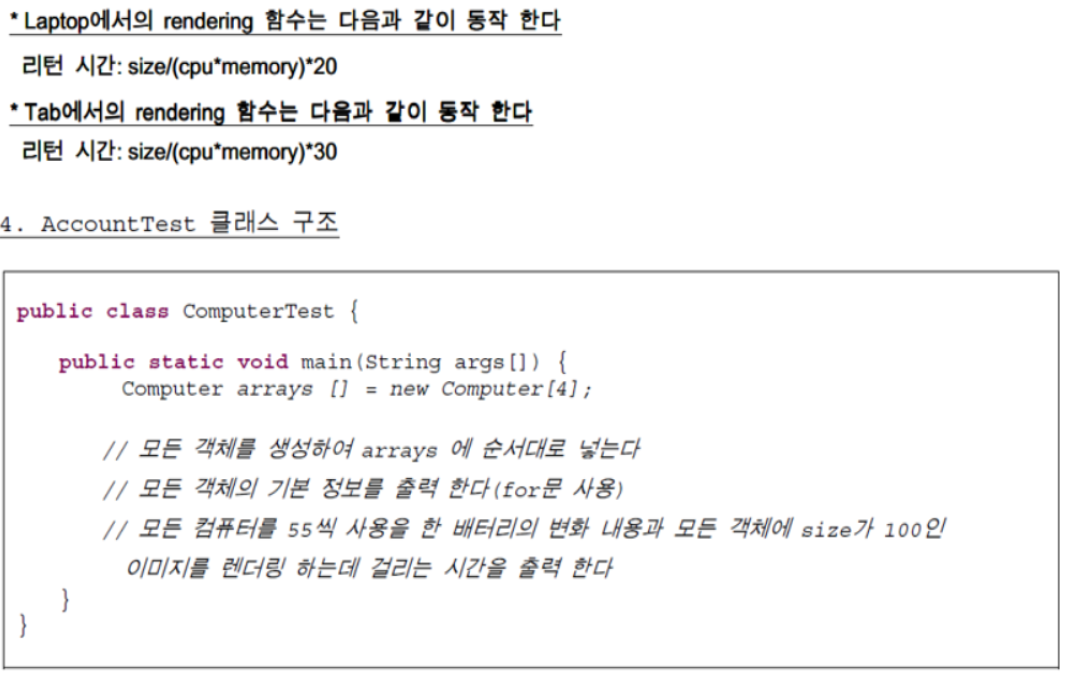
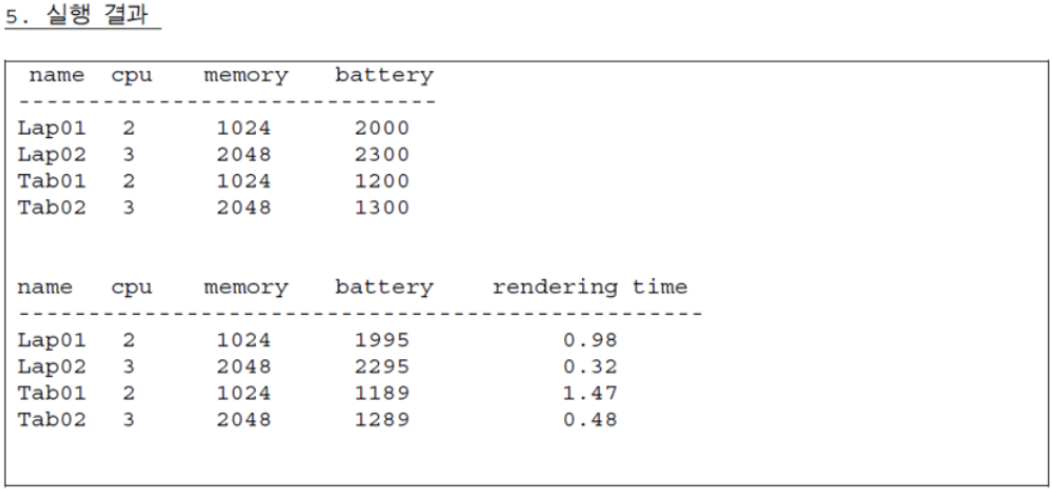
package com.computer;
public abstract class Computer {
private String name;
private int cpu;
private int memory;
private int battery;
public Computer() {
}
public Computer(String name, int cpu, int memory, int battery) {
super();
this.name = name;
this.cpu = cpu;
this.memory = memory;
this.battery = battery;
}
public abstract void opearate(int time);
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getCpu() {
return cpu;
}
public void setCpu(int cpu) {
this.cpu = cpu;
}
public int getMemory() {
return memory;
}
public void setMemory(int memory) {
this.memory = memory;
}
public int getBattery() {
return battery;
}
public void setBattery(int battery) {
this.battery = battery;
}
}
package com.computer;
interface Graphic {
public double rendering(int size);
}
package com.computer;
public class Laptop extends Computer implements Graphic{
Laptop() {
}
public Laptop(String name, int cpu, int memory, int battery) {
super(name, cpu, memory, battery);
}
@Override
public void opearate(int time) {
int battery = getBattery();
setBattery(battery - time / 10);
}
@Override
public double rendering(int size) {
return (double)size / (getCpu() * getMemory()) * 20;
}
@Override
public String toString() {
return getName() + "\t" + getCpu() + "\t" + getMemory() + "\t" + getBattery();
}
}
package com.computer;
public class Tab extends Computer implements Graphic{
Tab() {
}
public Tab(String name, int cpu, int memory, int battery) {
super(name, cpu, memory, battery);
}
@Override
public void opearate(int time) {
int battery = getBattery();
setBattery(battery - time / 5);
}
@Override
public double rendering(int size) {
return (double)size / (getCpu() * getMemory()) * 30;
}
@Override
public String toString() {
return getName() + "\t" + getCpu() + "\t" + getMemory() + "\t" + getBattery();
}
}
package com.computer;
public class ComputerTest {
public static void main(String[] args) {
Computer arrays [] = new Computer[4];
arrays[0] = new Laptop("Lap01", 2, 1024, 2000);
arrays[1] = new Laptop("Lap02", 3, 2048, 2300);
arrays[2] = new Tab("Tap01", 2, 1024, 1200);
arrays[3] = new Tab("Tap02", 3, 2048, 1300);
System.out.println("name\tcpu\tmemory\tbattery");
System.out.println("-------------------------------");
for(int i=0; i<4; i++) {
System.out.println(arrays[i]);
}
System.out.println();
for(int i=0; i<4; i++) {
arrays[i].opearate(55);
}
System.out.println("name\tcpu\tmemory\tbattery\t rendering time");
System.out.println("-------------------------------------------------");
for(int i=0; i<4; i++) {
System.out.print(arrays[i] + "\t ");
System.out.println(String.format("%.2f", ((Graphic) arrays[i]).rendering(100)));
}
}
}
๋ฌธ์ 4

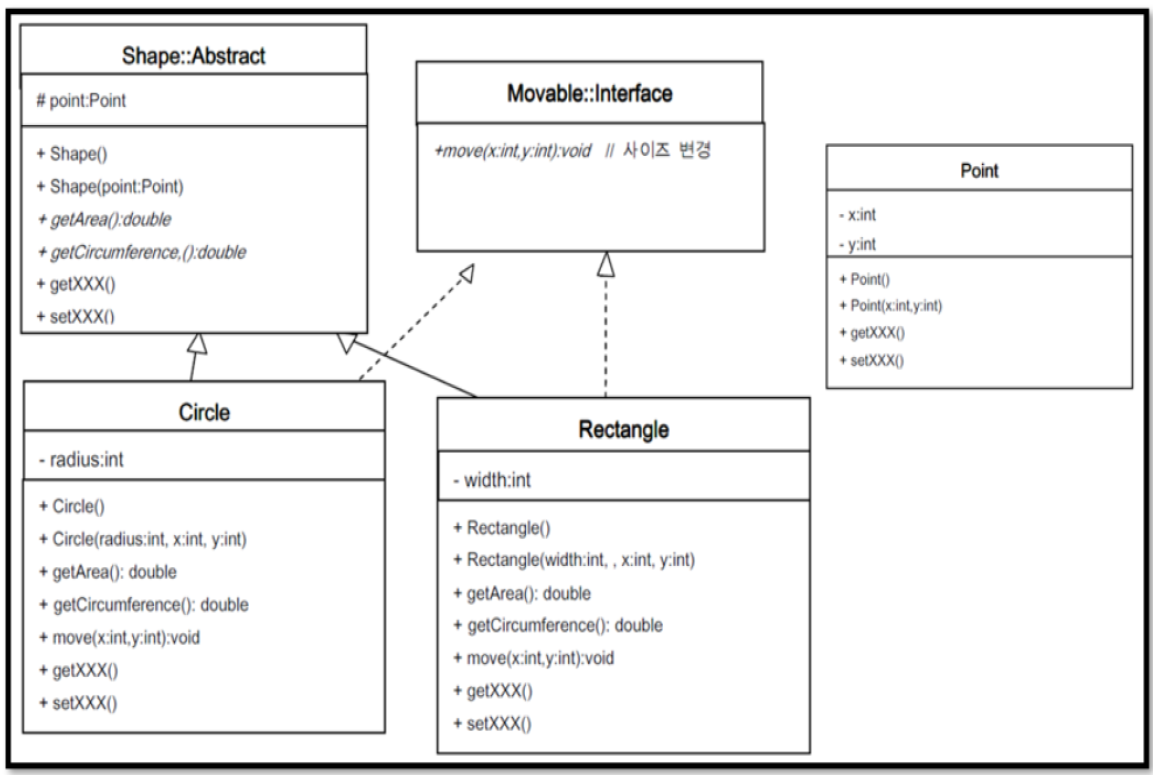
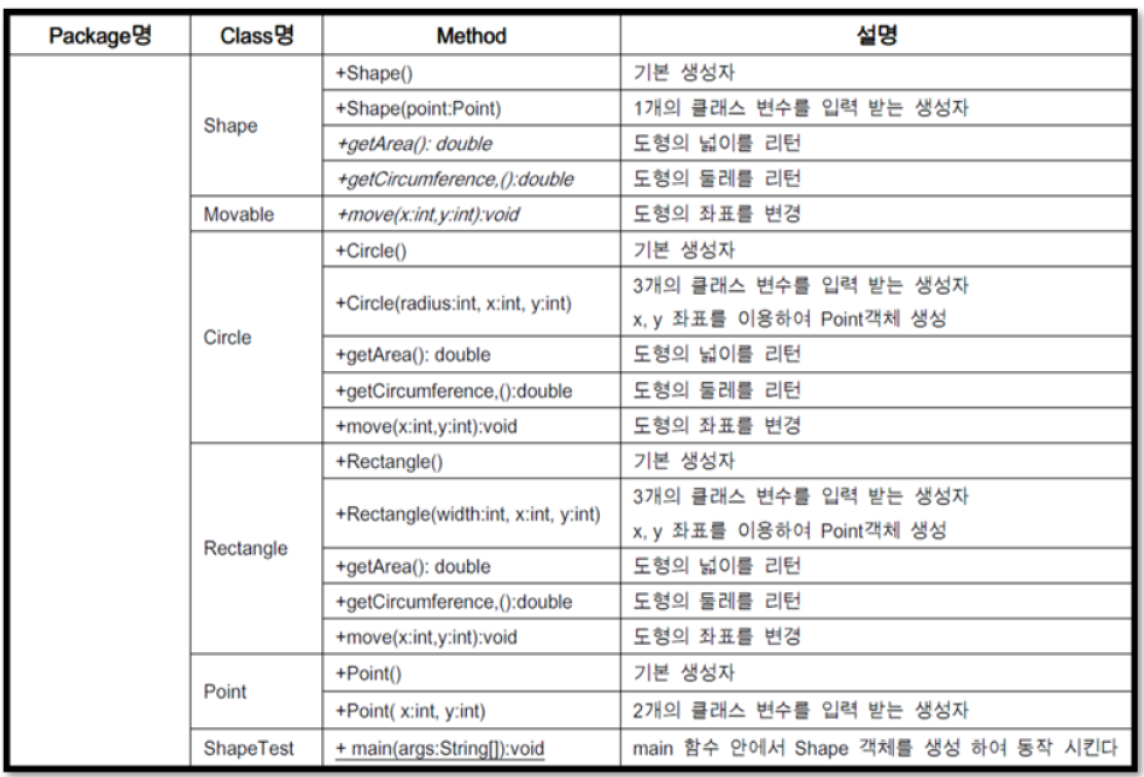
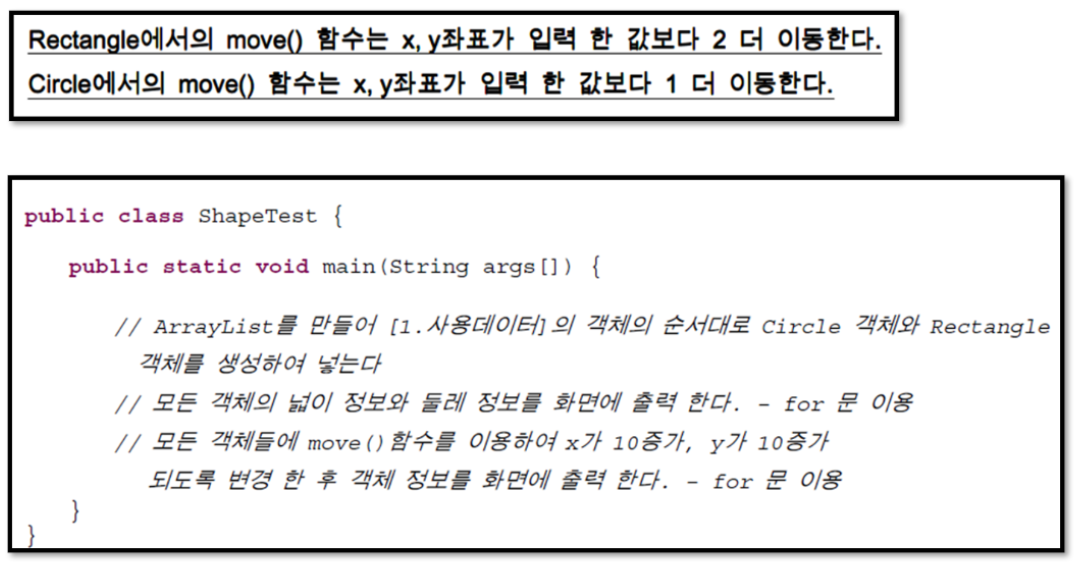
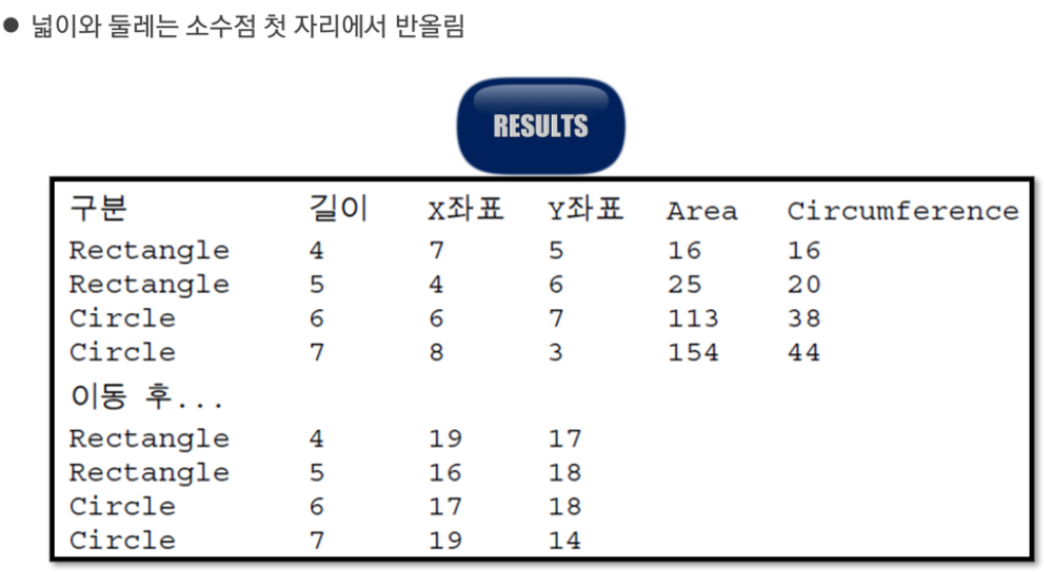
package com.shape;
public class Point {
private int x;
private int y;
public Point() {
}
public Point(int x, int y) {
super();
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
}
package com.shape;
public abstract class Shape {
protected Point point;
public Shape() {
}
public Shape(Point point) {
super();
this.point = point;
}
public abstract double getArea();
public abstract double getCircumference();
public Point getPoint() {
return point;
}
public void setPoint(Point point) {
this.point = point;
}
}
package com.shape;
interface Movable {
public void move(int x, int y);
}
package com.shape;
public class Rectangle extends Shape implements Movable{
private int width;
Rectangle() {
}
public Rectangle(int width, int x, int y) {
super(new Point(x,y));
this.width = width;
}
@Override
public void move(int x, int y) {
int cur_x = getPoint().getX();
int cur_y = getPoint().getY();
setPoint(new Point(cur_x + 2 + x, cur_y + 2 + y));
}
@Override
public double getArea() {
return getWidth() * getWidth();
}
@Override
public double getCircumference() {
return 4 * getWidth();
}
public int getWidth() {
return width;
}
public void setWidth(int width) {
this.width = width;
}
@Override
public String toString() {
return "Rectangle\t" + getWidth() + '\t' + getPoint().getX() + '\t' + getPoint().getY();
}
}
package com.shape;
public class Circle extends Shape implements Movable{
private int radius;
Circle() {
}
public Circle(int radius, int x, int y) {
super(new Point(x,y));
this.radius = radius;
}
@Override
public void move(int x, int y) {
int cur_x = getPoint().getX();
int cur_y = getPoint().getY();
setPoint(new Point(cur_x + 1 + x, cur_y + 1 + y));
}
@Override
public double getArea() {
return getRadius() * getRadius() * 3.14;
}
@Override
public double getCircumference() {
return 2 * 3.14 * getRadius();
}
public int getRadius() {
return radius;
}
public void setRadius(int radius) {
this.radius = radius;
}
@Override
public String toString() {
return "Circle\t\t" + getRadius() + '\t' + getPoint().getX() + '\t' + getPoint().getY();
}
}
package com.shape;
import java.util.ArrayList;
import java.util.List;
public class ShapeTest {
public static void main(String[] args) {
List<Shape> shapeList = new ArrayList<Shape>();
shapeList.add(new Rectangle(4, 7, 5));
shapeList.add(new Rectangle(5, 4, 6));
shapeList.add(new Circle(6, 6, 7));
shapeList.add(new Circle(7, 8, 3));
System.out.println("๊ตฌ๋ถ\t\t๊ธธ์ด\tX์ขํ\tY์ขํ\tArea\tCircumference");
for(Shape shape : shapeList) {
System.out.println(shape + "\t" + String.format("%.0f", shape.getArea()) + "\t" + String.format("%.0f", shape.getCircumference()));
}
for(Shape shape : shapeList) {
((Movable)shape).move(10, 10);
}
System.out.println("์ด๋ ํ...");
for(Shape shape : shapeList) {
System.out.println(shape);
}
}
}