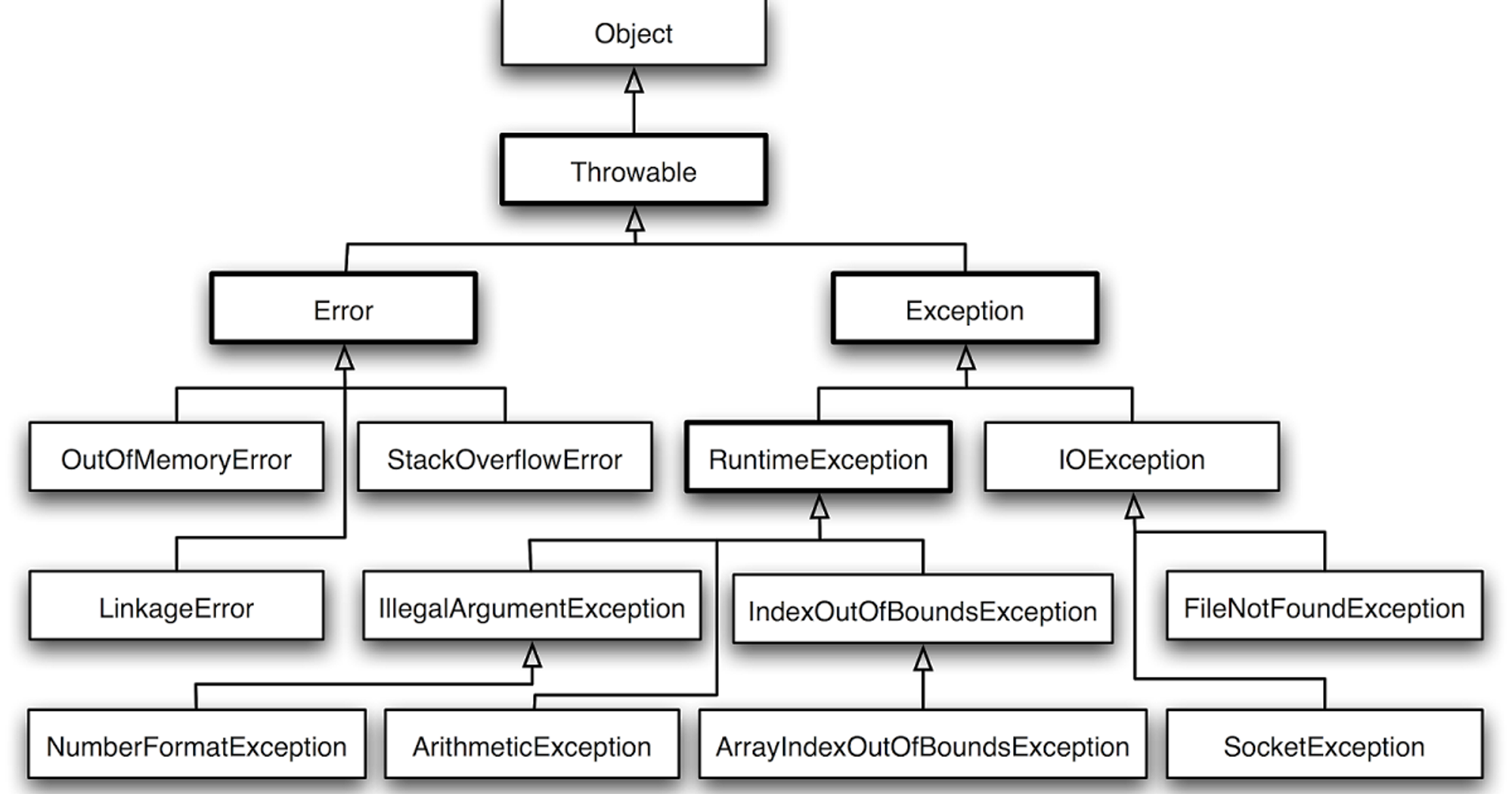
오류
- 일반적으로
회복이 불가능
한 문제
- 시스템 레벨에서, 또는 주로 환경적인 이유로 발생
- 에러가 발생한 경우 이유를 분석해서 대응
예외
- 일반적으로
회복이 가능
한 문제
- 대응 가능
- 코드레벨에서 할 수 있는 문제상황에 대한 대응을 예외 처리라고 함
컴파일 에러(예외)
런타임 에러(예외)
- 프로그램이 실행 도중 맞닥뜨리게 되는 에러 및 예외
예외처리 관점에서 예외의 종류
Checked Exception
- 컴파일 시점에 확인되는 예외
반드시 예외 처리를 해줘
야하는 예외
- ≠ 컴파일 에러
- Checked Exception을 처리하지 않으면, 컴파일 에러가 발생
IOException
Unchecked Exception
- 런타임 시점에 확인되는 예외
- 예외 처리가 반드시 필요하지 않은 예외
RuntimeException
예외처리하는 방법
- 우리가 예외를 어떻게 정의할 것인지
- 예외가 발생할 수 있음을 알리고
- 사용자는 예외가 발생할 수 있음을 알고 예외를 핸들링
package chapter4.exception;
public class BadException extends Exception{
public BadException() {
super("예외 발생");
}
}
package chapter4.logic;
import chapter4.exception.BadException;
public class Test {
public void printSomething(boolean occurException) throws BadException{
if(occurException){
throw new BadException();
}
System.out.println("출력");
}
}
package chapter4;
import chapter4.exception.BadException;
import chapter4.logic.Test;
public class Main {
public static void main(String[] args) {
Test test = new Test();
try {
test.printSomething(true);
System.out.println("try");
} catch (BadException e) {
throw new RuntimeException(e);
} finally {
System.out.println("finally");
}
}
}
finally
Process finished with exit code 0
Chained Exception
- 여러 가지 예외를 하나의 큰 분류의 예외로 묶어서 다루기 위해 쓰임
checked exception
을 unchecked exception
으로 포장하는데 유용하게 사용되기도 함
public class main {
public static void main(String[] args) {
try {
NumberFormatException ex = new NumberFormatException("가짜 예외이유");
ex.initCause(new NullPointerException("진짜 예외이유"));
throw ex;
} catch (NumberFormatException ex) {
ex.printStackTrace();
ex.getCause().printStackTrace();
}
throw new RuntimeException(new Exception("이것이 진짜 예외 이유 입니다."));
}
}
Caused by: java.lang.NullPointerException: 진짜 예외이유