- inflearn 김영한의 '스프링 입문 - 코드로 배우는 스프링 부트, 웹 MVC, DB 접근 기술' 강의를 보고 정리한 내용입니다.
1. 프로젝트 생성
- (Java가 설치되어 있다는 가정하에) 스프링 부트 스타터 사이트로 이동해 스프링 프로젝트 생성
- 다음과 같이 설정
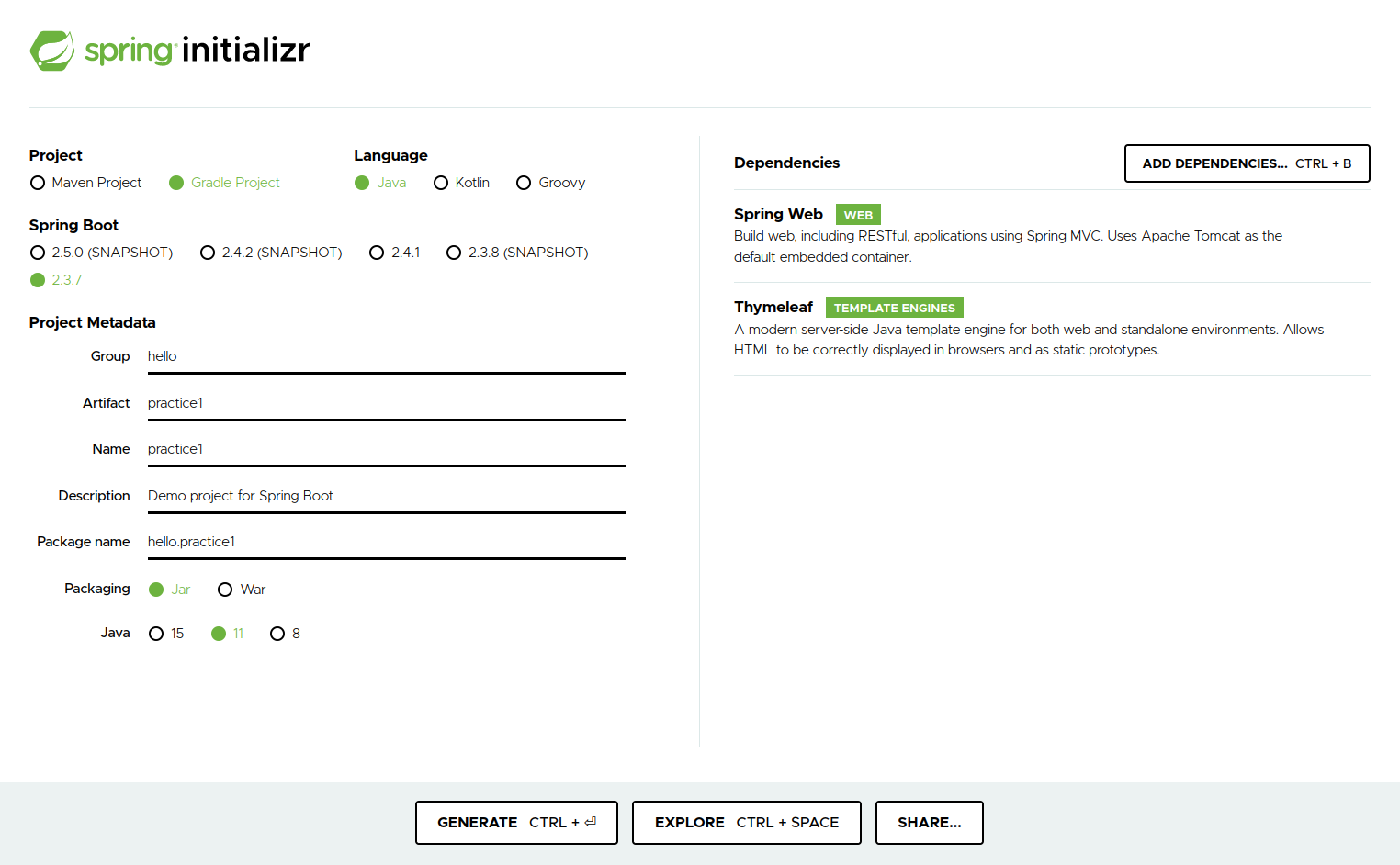
- 생성된 project의 build.gradle 파일
- spring boot가 설정파일을 제공해준다.
- 버전 설정, 라이브러리(Spring Web, Thymeleaf)
- dependencies: test라이브러리가 자동으로 들어간다.(junit5 library)
- mavenCentral()을 통해 다운로드(다른 url로 설정해줘도 된다.)
- 실행방법
- hello.practice1/Practice1Application main함수 실행
2. 라이브러리 살펴보기
- gradle이나 maven과 같은 build tool들은 의존관계를 관리해준다.
- ex) 프로젝트 생성시 추가한 Spring Web library와 의존 관계인 다른 여러 library들을 가져온다.
- 스프링 부트 라이브러리
- spring-boot-starter-web
- spring-boot-starter-tomcat: 톰캣 (웹서버)
- spring-webmvc: 스프링 웹 MVC
- spring-boot-starter-thymeleaf: 타임리프 템플릿 엔진(View)
- spring-boot-starter(공통): 스프링 부트 + 스프링 코어 + 로깅
- spring-boot-starter-logging
- 테스트 라이브러리
- spring-boot-starter-test
- junit: 테스트 프레임워크
- mockito: 목 라이브러리
- assertj: 테스트 코드를 좀 더 편하게 작성하게 도와주는 라이브러리
- spring-test: 스프링 통합 테스트 지원
3. View 환경설정
Welcome Page 만들기
- /resources/static 경로에 index.html을 생성하면 Welcome page기능을 spring boot에서 제공한다.
- resources/static/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
Hello
<a href="/hello">hello</a>
</body>
</html>
thymeleaf 템플릿 엔진
- HelloController : index.html의 a tag의 /hello 처리
- controller/HelloController.java
- @Controller : 해당 클래스를 Cotroller로 사용한다고 Spring Framework에 알린다.
- @GetMapping : 해당 메서드를 HTTP Get요청 핸들러 메서드로 사용한다.
- model.addAttribute("key", "value")
package hello.practice1.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("hello")
public String hello(Model model){
model.addAttribute("data", "Hello!!");
return "hello";
}
}
- hello.html : thymeleaf 템플릿 엔진을 사용한 html
- resources/templates/hello.html
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<p th:text="'안녕하세요. ' + ${data}" >안녕하세요. 손님</p>
</body>
</html>
- 동작 환경 그림
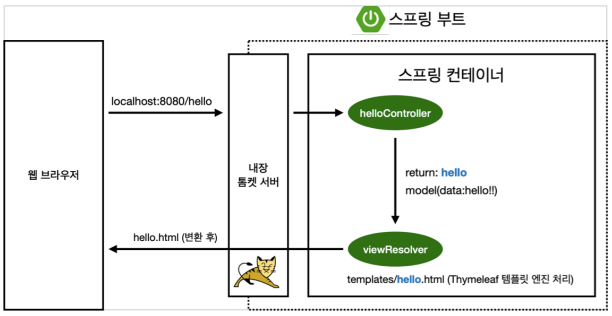
- Controller에서 리턴 값으로 문자를 반환하면 viewResolver가 화면을 찾아서 처리한다.
- 스프링 부트 템플릿엔진 기본 viewName 매핑
- resources:templates/ +{ViewName}+ .html
4. 빌드하고 실행하기
- 해당 프로젝트의 경로로 이동
- $ ./gradelw build
- $ cd build/libs
- $ java -jar 실행파일이름.jar