11654번 아스키 코드
문제
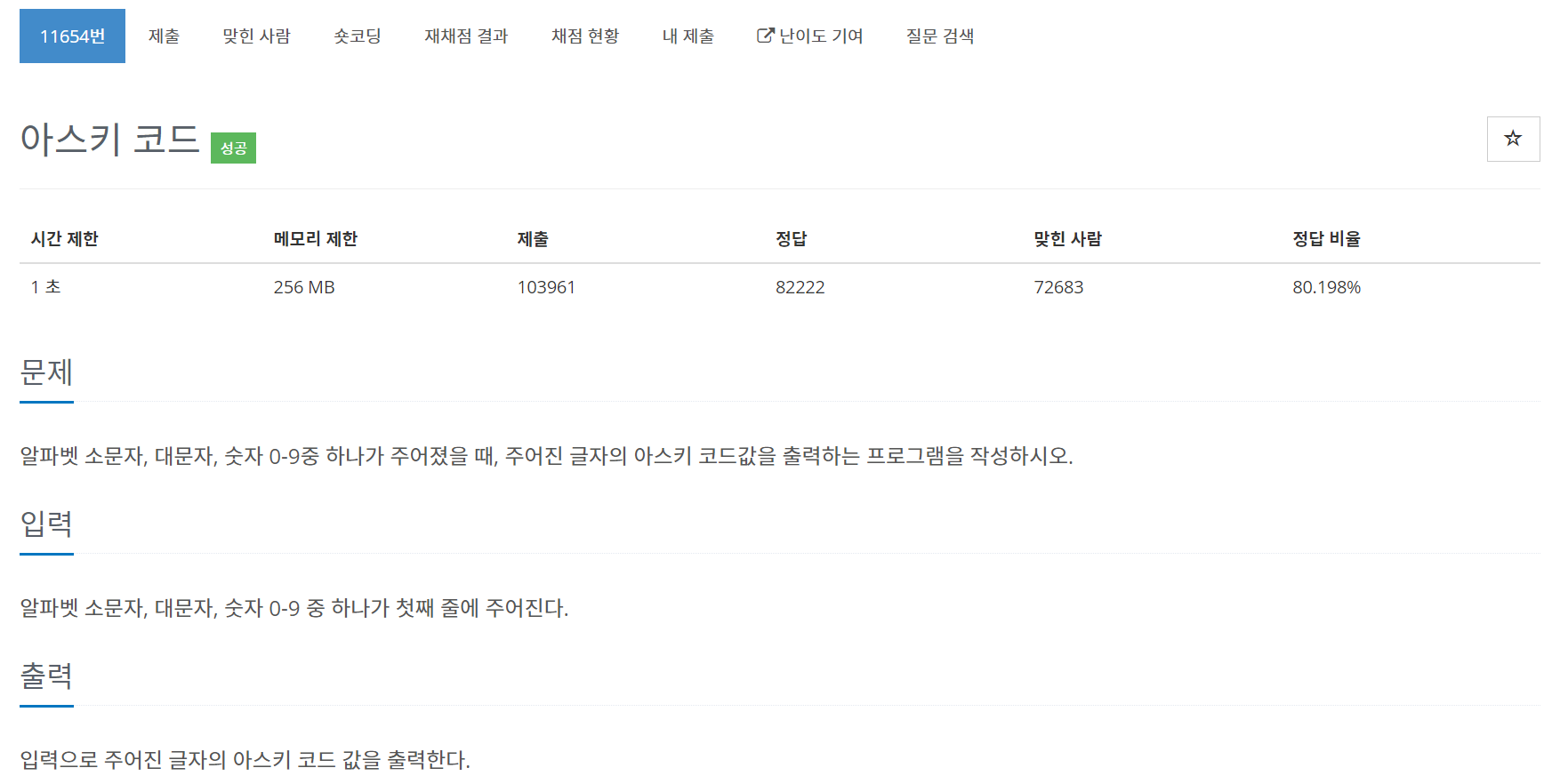
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
char c = sc.next().charAt(0);
System.out.print((int) c);
sc.close();
}
}
11720번 숫자의 합
문제
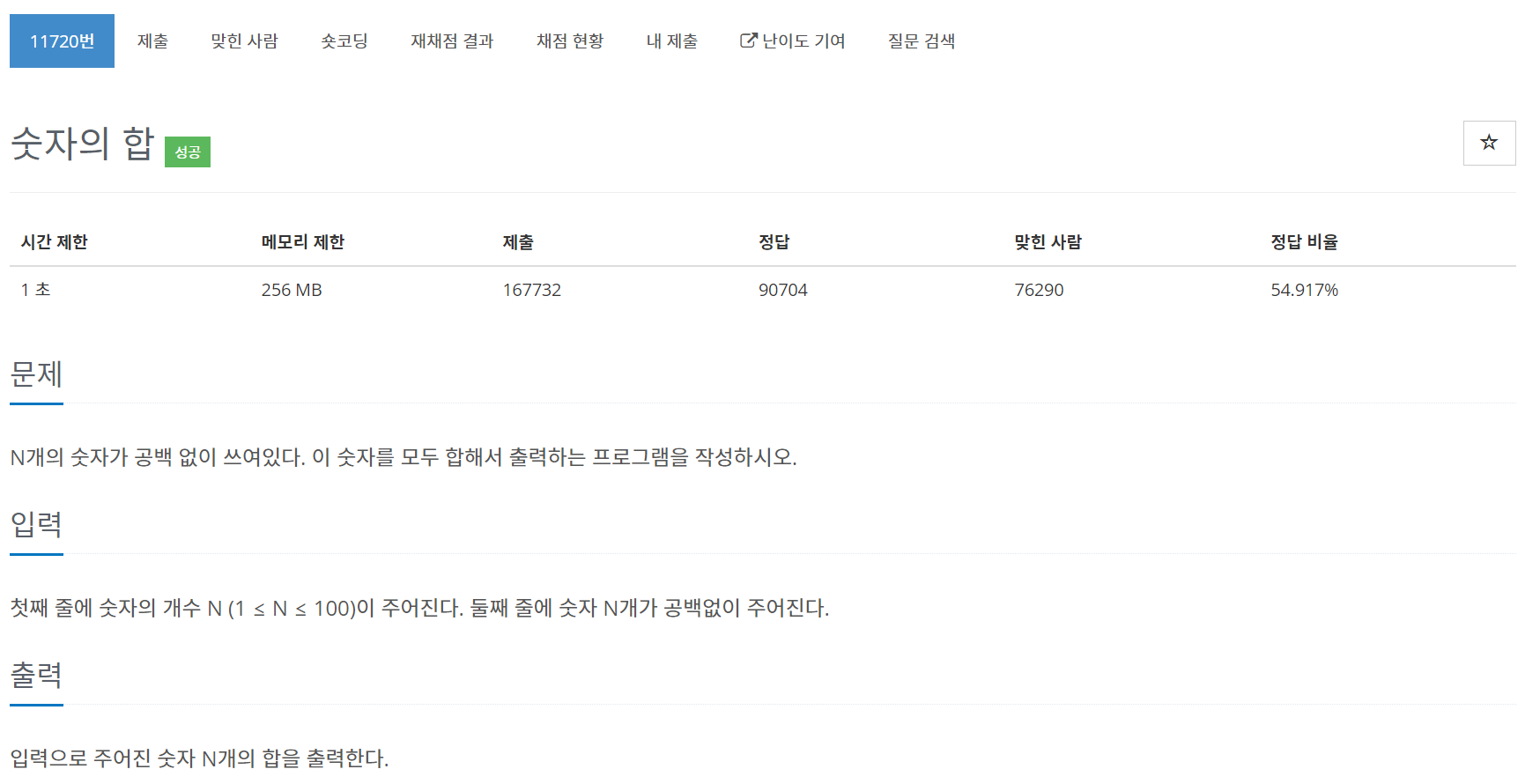
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
String str = sc.next();
int sum = 0;
for(int i = 0; i < n; i++) {
sum += str.charAt(i) -'0';
}
System.out.print(sum);
sc.close();
}
}
10809번 알파벳 찾기
문제
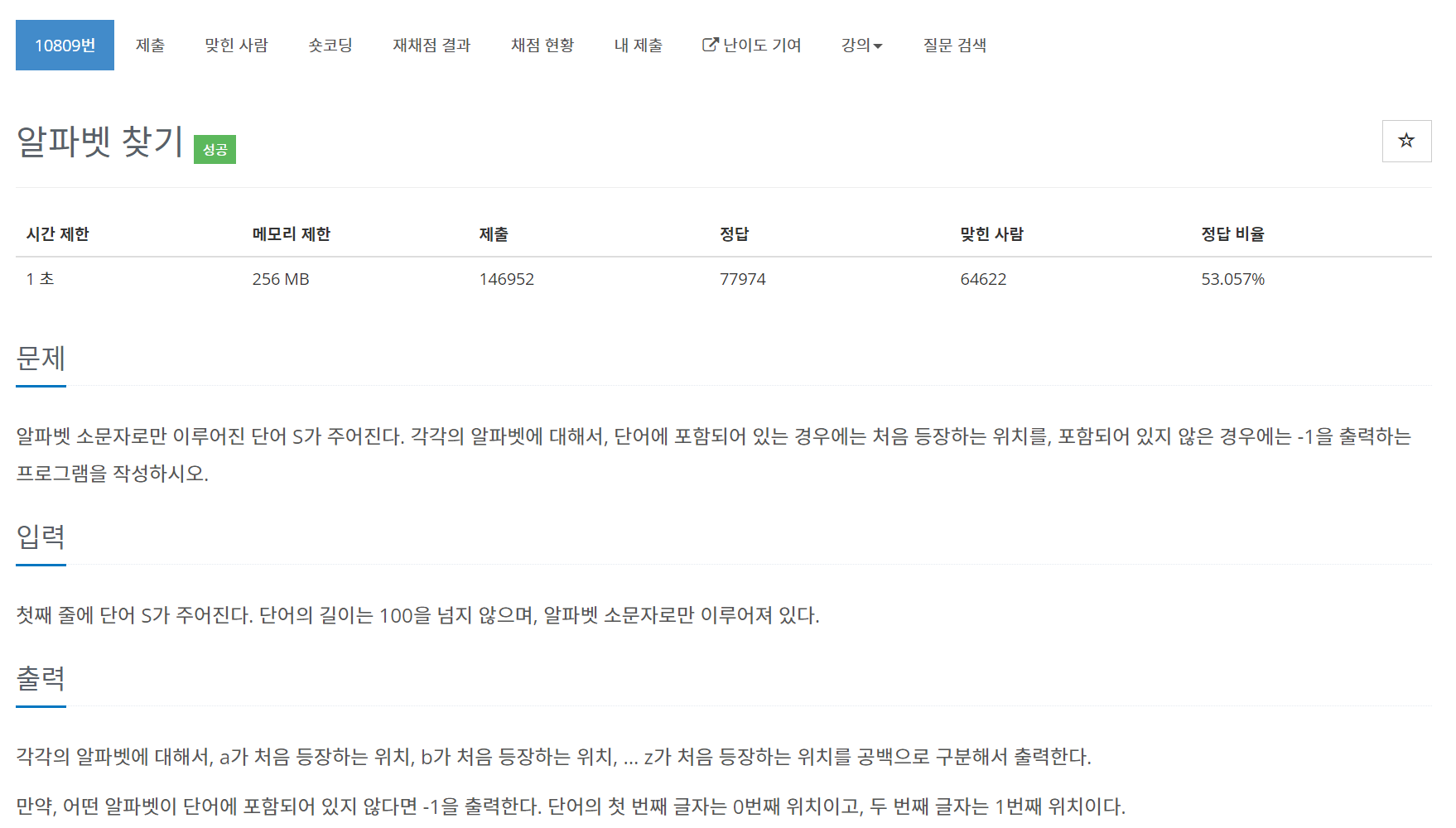
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int[] arr = new int[26];
for(int i = 0; i < arr.length; i++) {
arr[i] = -1;
}
String str = sc.nextLine();
for(int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
if(arr[c - 'a'] == -1) {
arr[c - 'a'] = i;
}
}
for(int a : arr) {
System.out.print(a + " ");
}
sc.close();
}
}
2675번 문자열 반복
문제
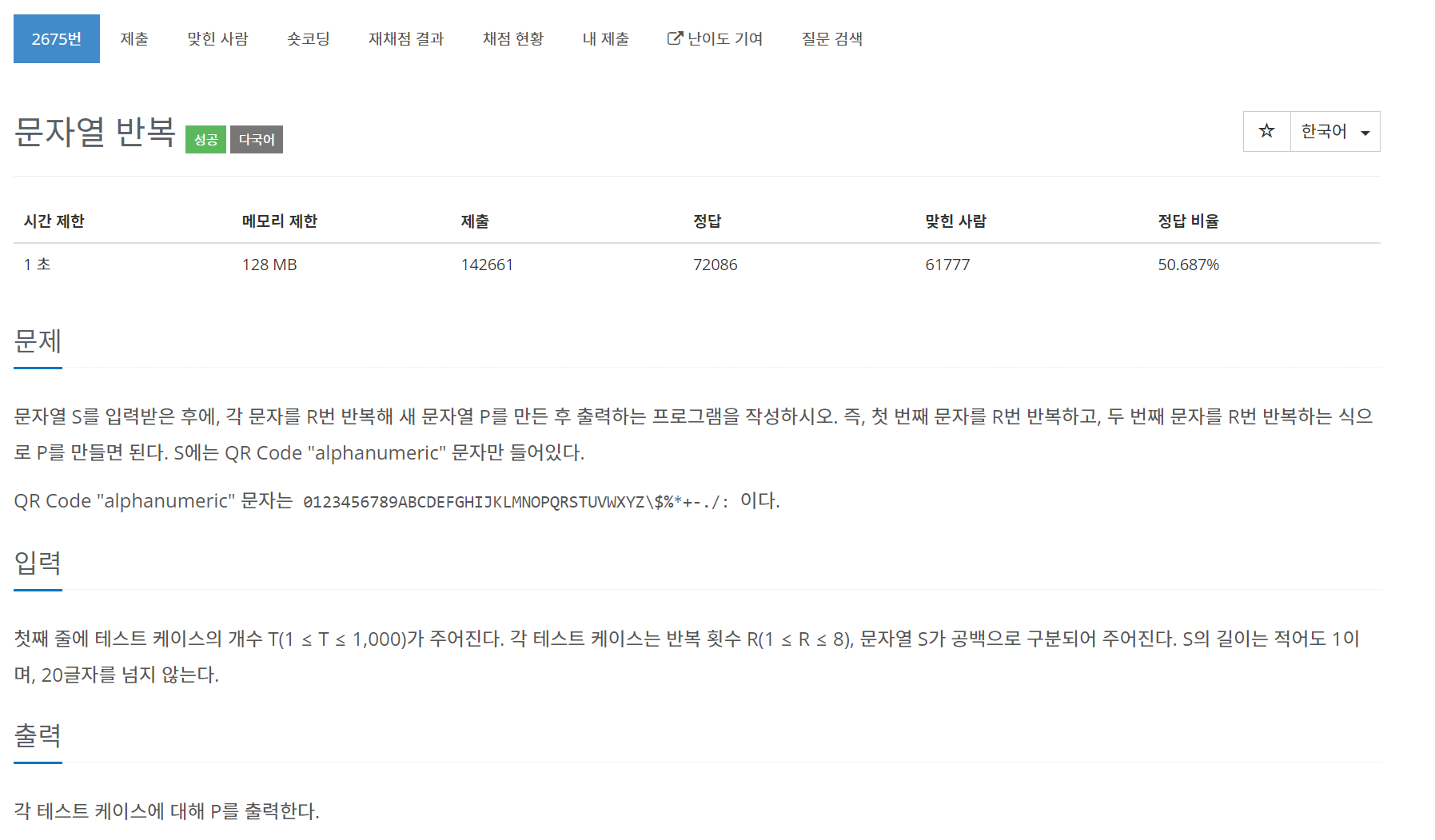
풀이
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int t;
char[] arr;
for(int i = 0; i < n; i++){
t = sc.nextInt();
arr = sc.next().toCharArray();
for(int j = 0; j < arr.length; j++){
for(int k = 0; k < t; k++){
System.out.print(arr[j]);
}
}
System.out.println();
}
sc.close();
}
}
1157번 단어 공부
문제
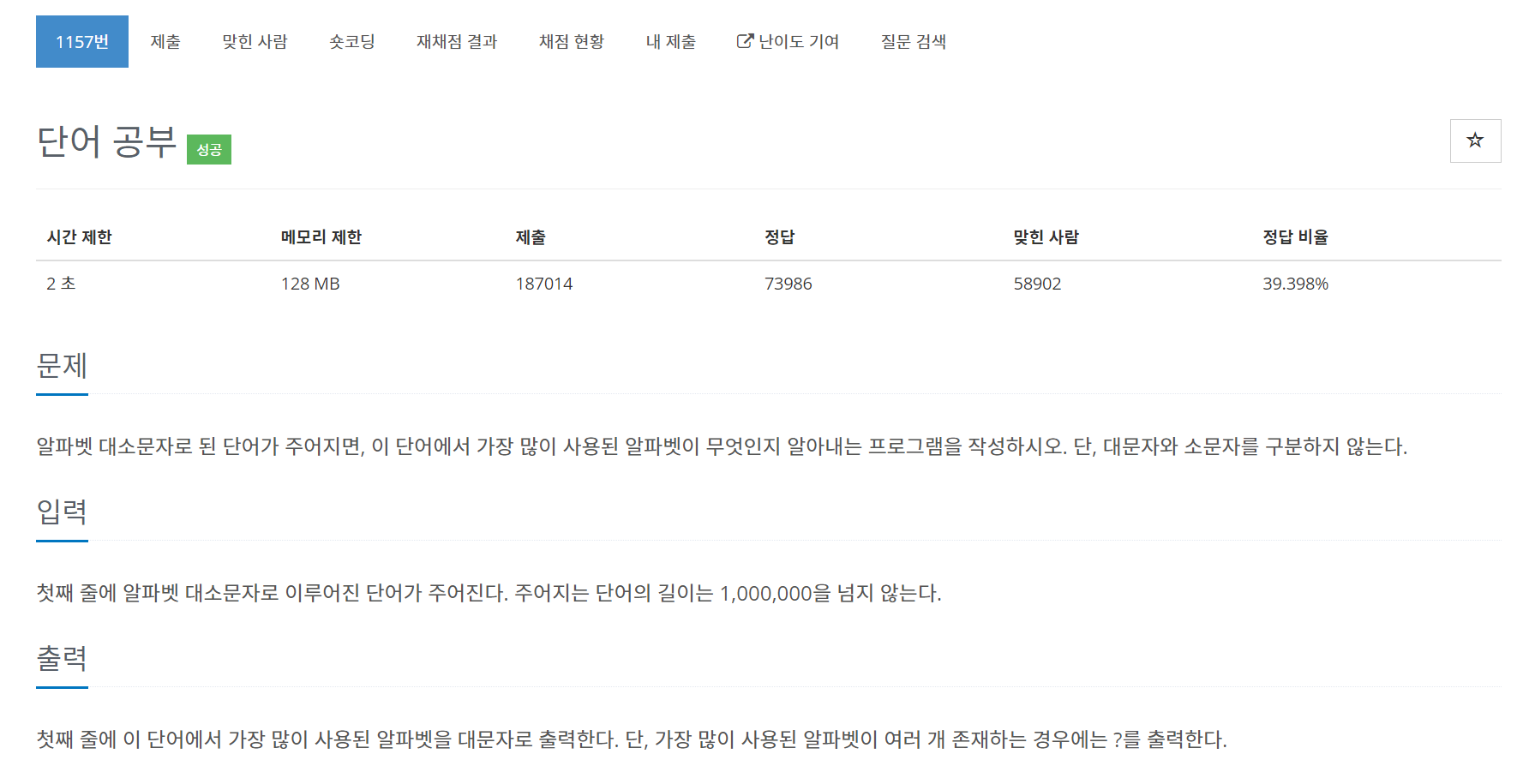
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int[] arr = new int[26];
String str = sc.nextLine().toUpperCase();
int max = 0;
char c = 'a';
for(int i =0; i < str.length(); i++) {
arr[str.charAt(i) - 65]++;
if (max < arr[str.charAt(i) - 65]) {
max = arr[str.charAt(i) - 65];
c = str.charAt(i);
} else if(max == arr[str.charAt(i) - 65]){
c = '?';
}
}
System.out.println(c);
}
}
1152번 단어 개수
문제
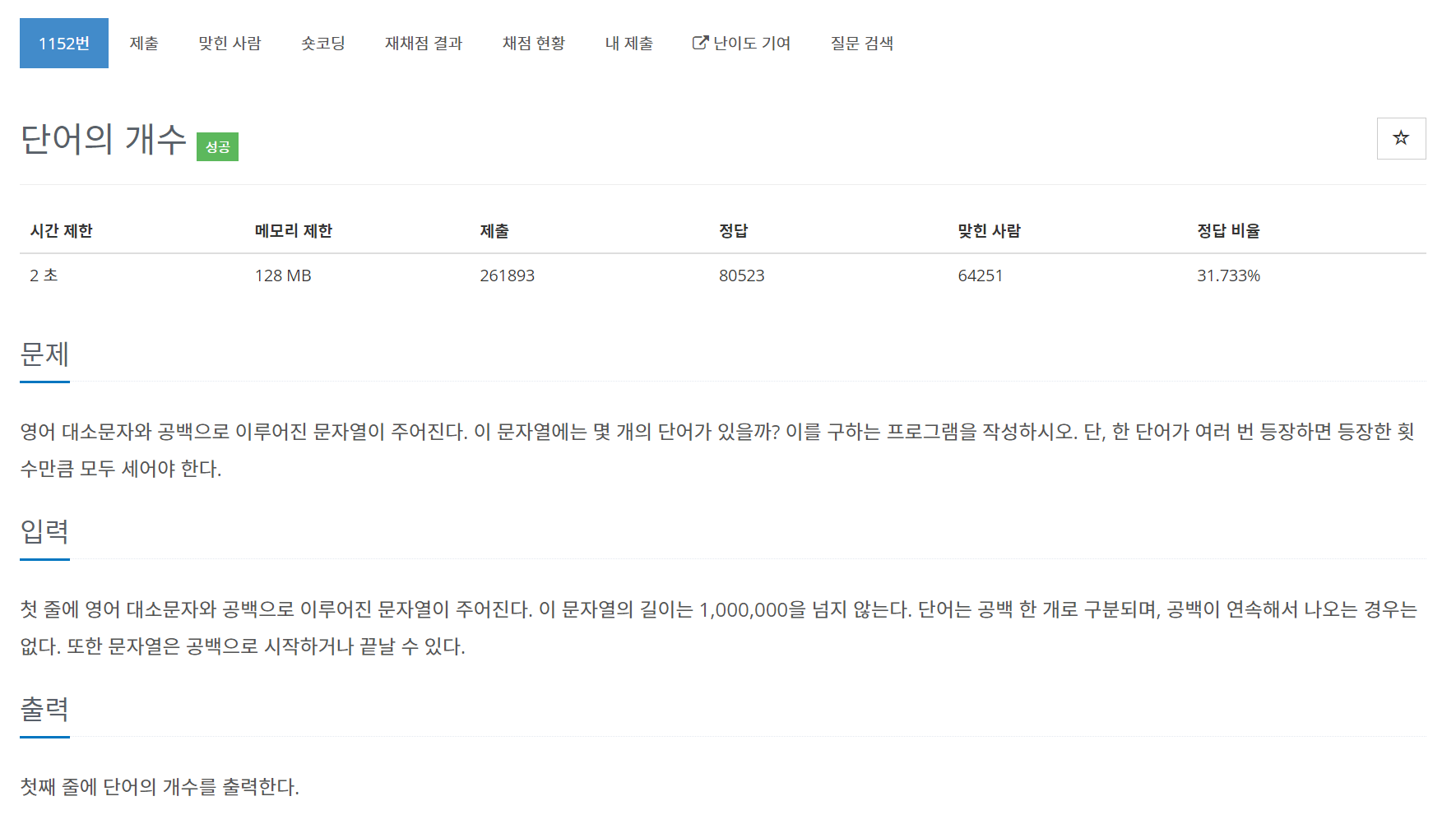
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine().trim();
int cnt = 0;
int wcnt = 0;
for (int i = 0; i < str.length(); i++) {
if (str != null && str.charAt(0) != ' ') {
if (str.charAt(i) == ' ') {
cnt++;
} else if (str != null && str.charAt(i) != ' ') {
wcnt = 1;
}
}
}
if (cnt > 0) {
wcnt = cnt + 1;
}
System.out.println(wcnt);
sc.close();
}
}
2908번 상수
문제
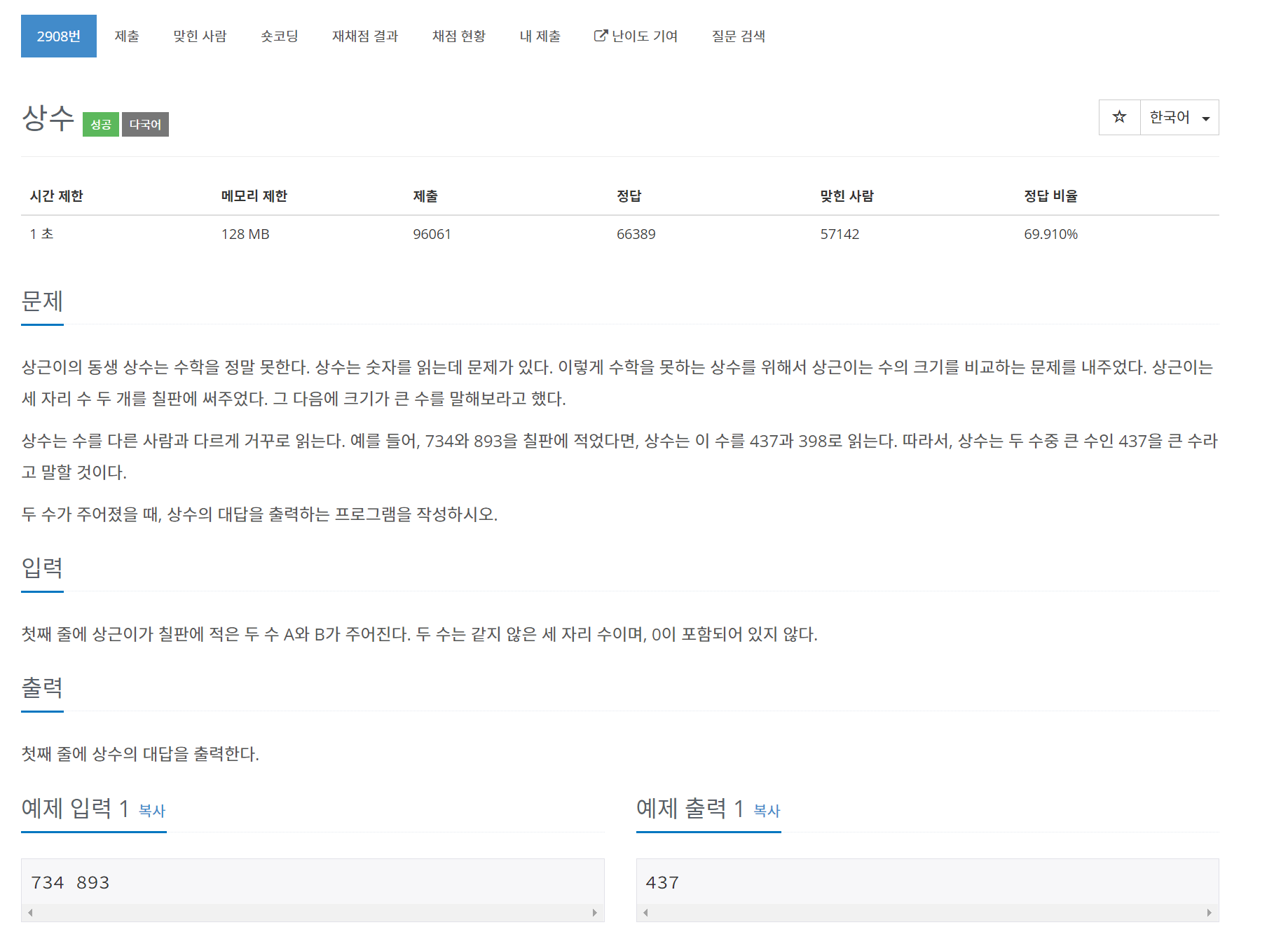
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
a = (a % 10) * 100 + ((a % 100) / 10) * 10 + (a / 100);
b = (b % 10) * 100 + ((b % 100) / 10) * 10 + (b / 100);
if(a > b) {
System.out.println(a);
} else {
System.out.println(b);
}
}
}
5622번 다이얼
문제
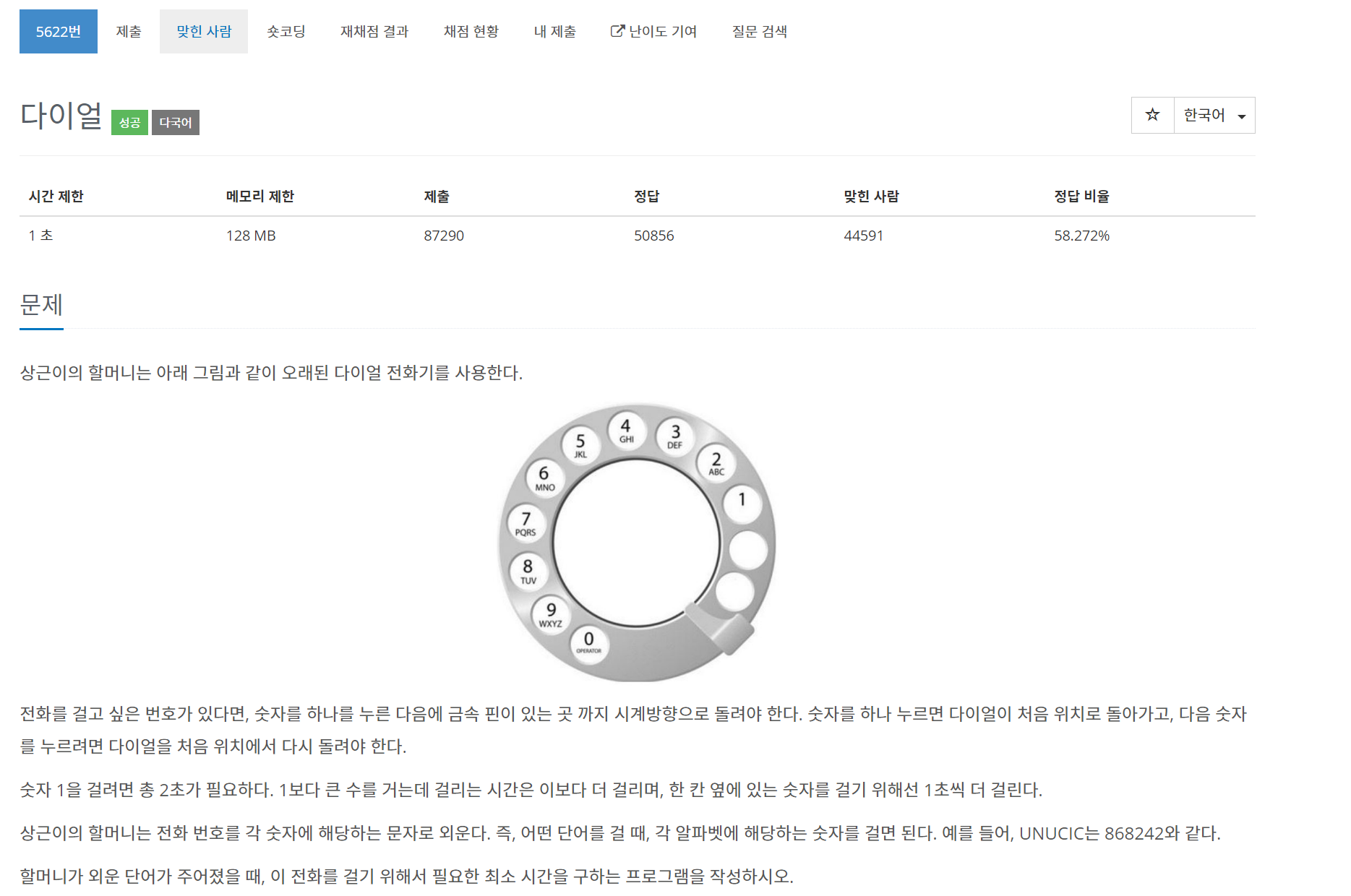
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.next();
int t = 0;
char c;
for(int i = 0; i < str.length(); i++) {
c = str.charAt(i);
if(c >= 'A' && c <= 'C') {
t += 3;
} else if(c >= 'D' && c <= 'F') {
t += 4;
} else if(c >= 'G' && c <= 'I') {
t += 5;
} else if(c >= 'J' && c <= 'L') {
t += 6;
} else if(c >= 'M' && c <= 'O') {
t += 7;
} else if(c >= 'P' && c <= 'S') {
t += 8;
} else if(c >= 'T' && c <= 'V') {
t += 9;
} else if(c >= 'W' && c <= 'Z') {
t += 10;
}
}
System.out.println(t);
sc.close();
}
}
2941번 크로아티아 알파벳
문제
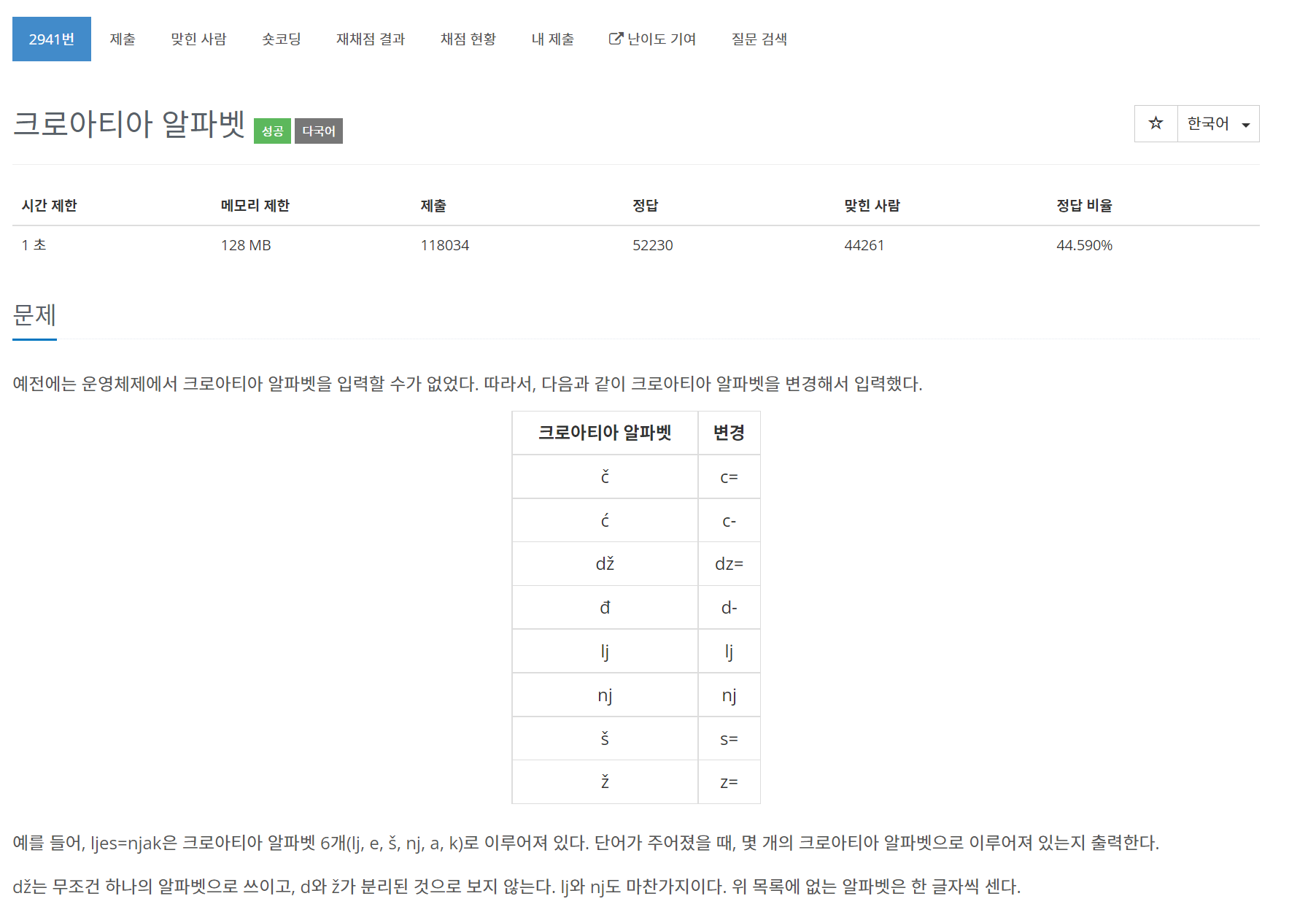
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str = sc.nextLine();
String[] ca = { "c=", "c-", "dz=", "d-", "lj", "nj", "s=", "z=" };
for(int i = 0; i < ca.length; i++) {
str = str.replace(ca[i], "c");
}
System.out.println(str.length());
sc.close();
}
}
1316번 그룹 단어 체커
문제
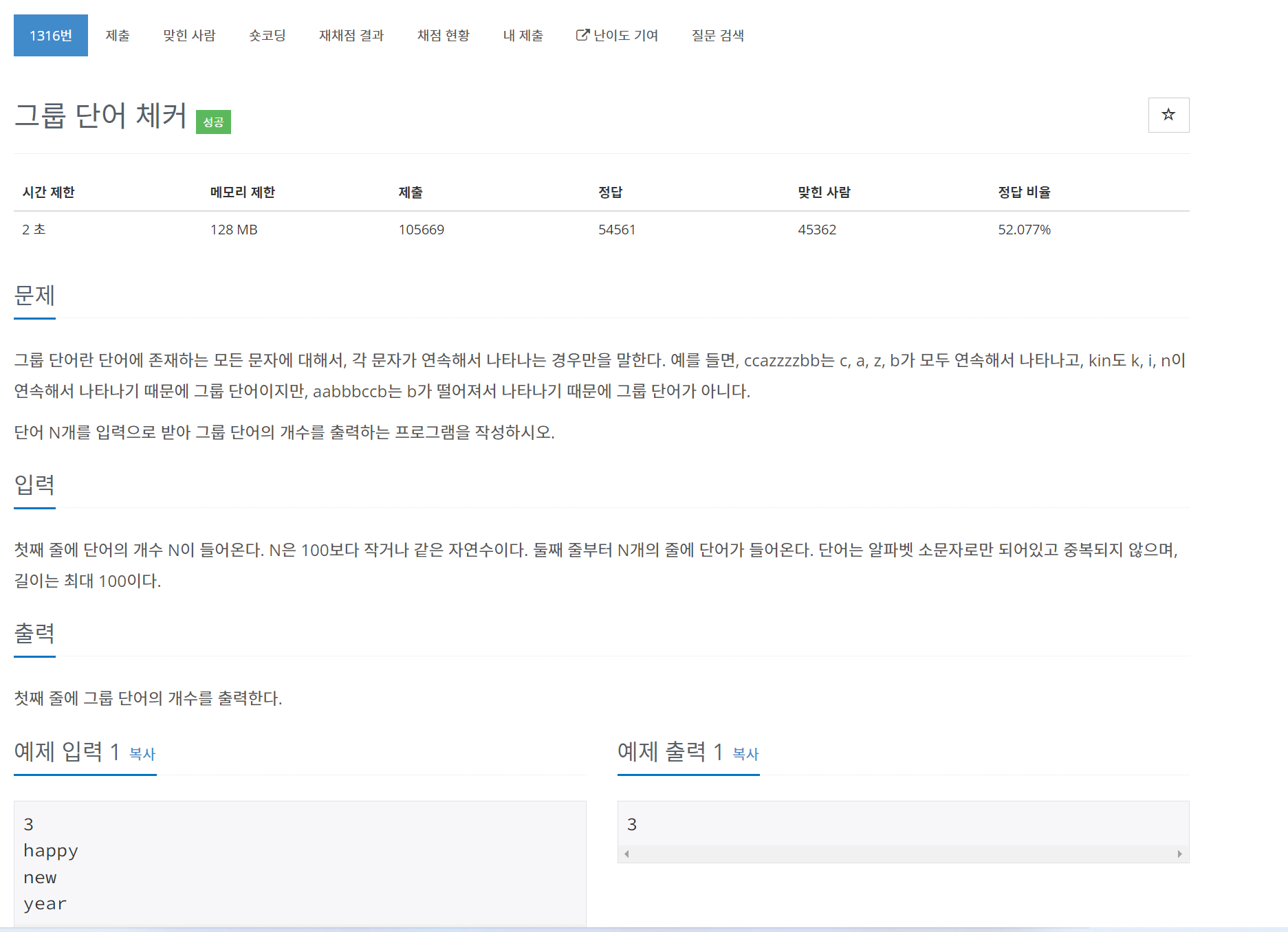
풀이
import java.util.Scanner;
public class Main {
public static boolean check() {
String str = sc.next();
boolean[] arr = new boolean[26];
int prev = 0;
for(int i = 0; i < str.length(); i++) {
int now = str.charAt(i);
if(prev != now) {
if(arr[now - 'a'] == false) {
arr[now - 'a'] = true;
prev = now;
} else {
return false;
}
}
}
return true;
}
static Scanner sc = new Scanner(System.in);
public static void main(String[] args) {
int n = sc.nextInt();
int cnt = 0;
for(int i = 0; i < n; i++) {
if(check() == true) cnt++;
}
System.out.println(cnt);
sc.close();;
}
}