2739번 구구단
문제
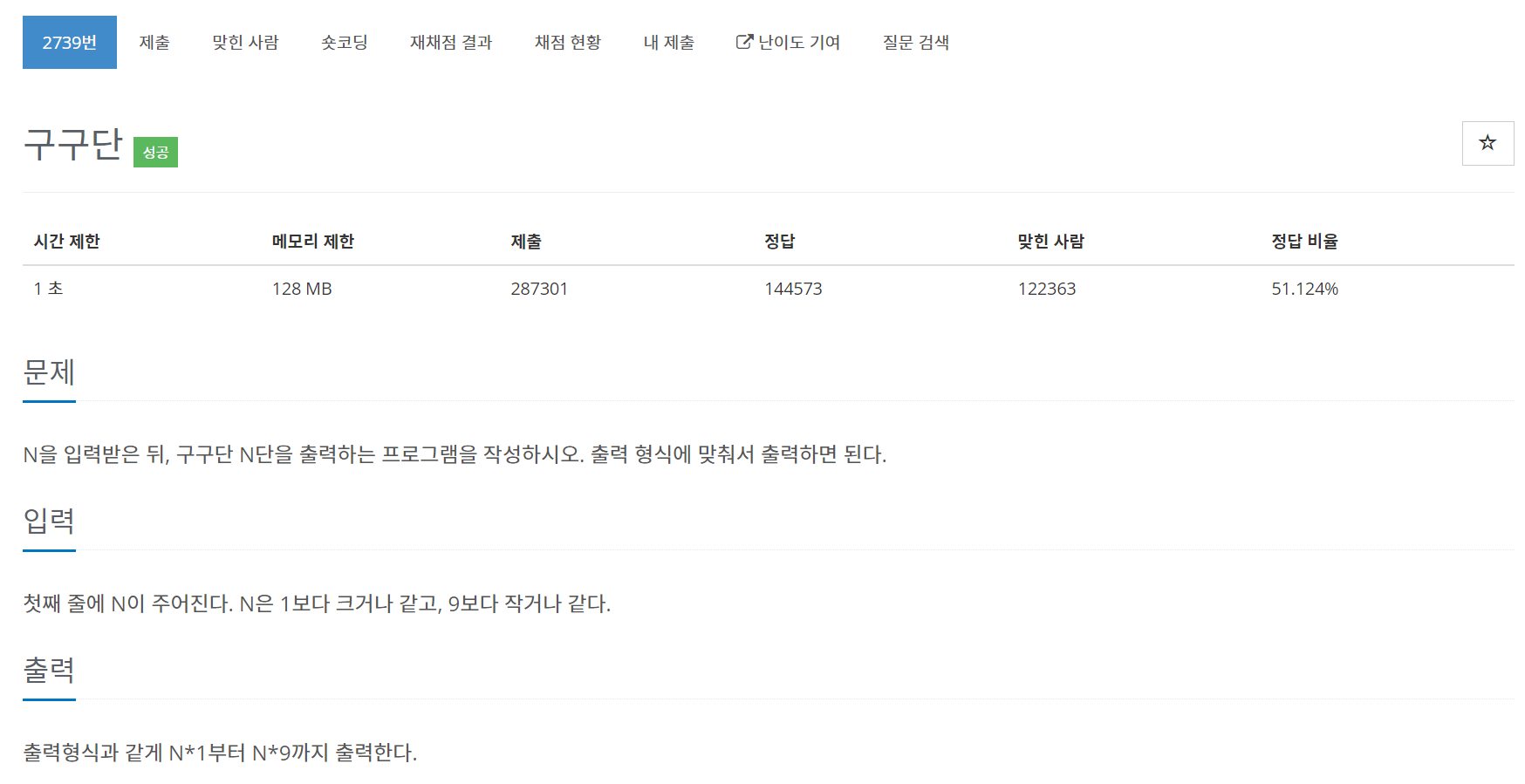
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for(int i = 1; i < 10; i++)
System.out.println(n + " * " + i + " = " + (n * i));
sc.close();
}
}
10950번 A+B-3
문제
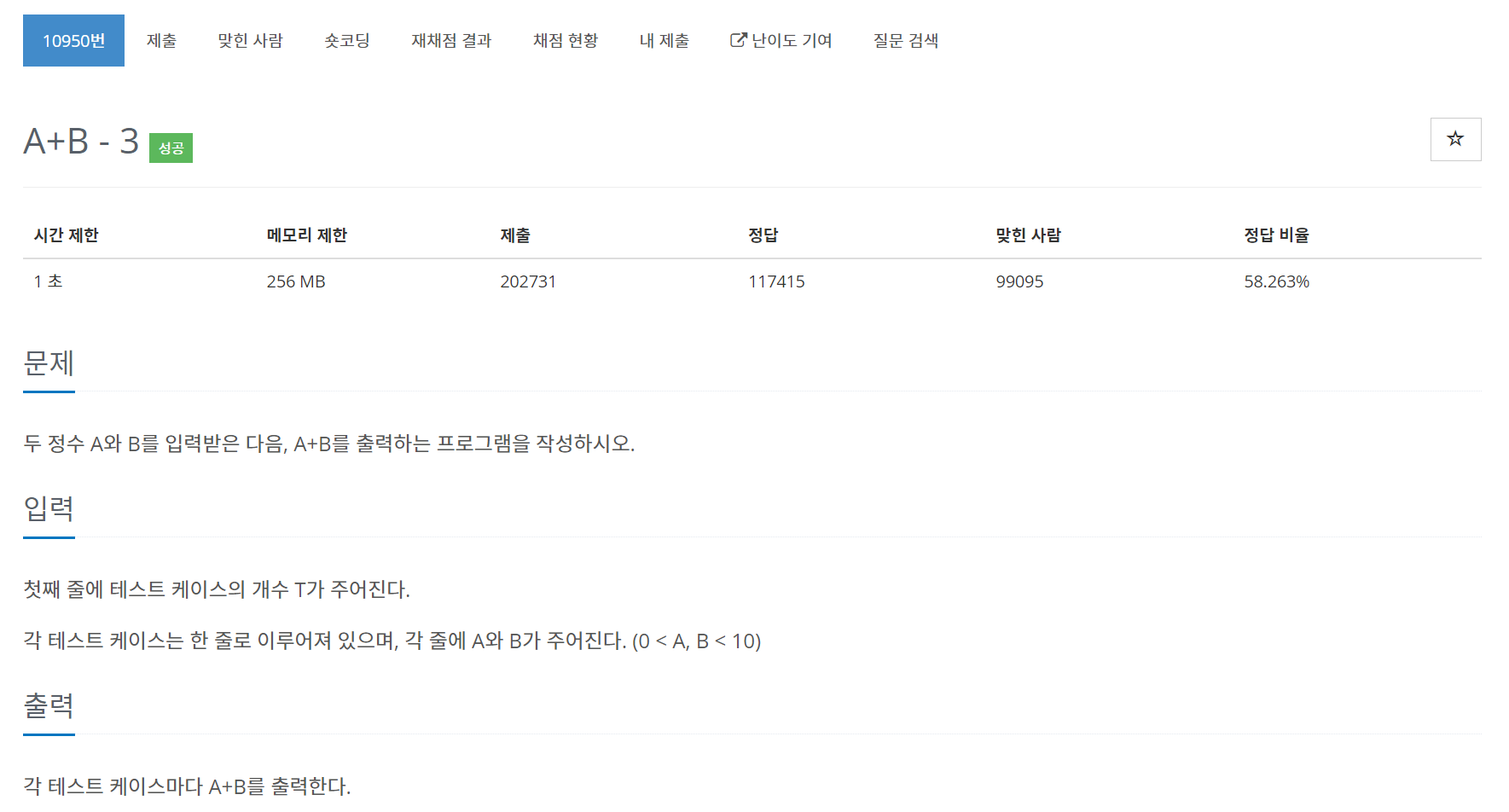
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int a = 0;
int b = 0;
for(int i = 0; i < n; i++) {
a = sc.nextInt();
b = sc.nextInt();
System.out.println(a + b);
}
sc.close();
}
}
8393번 합
문제
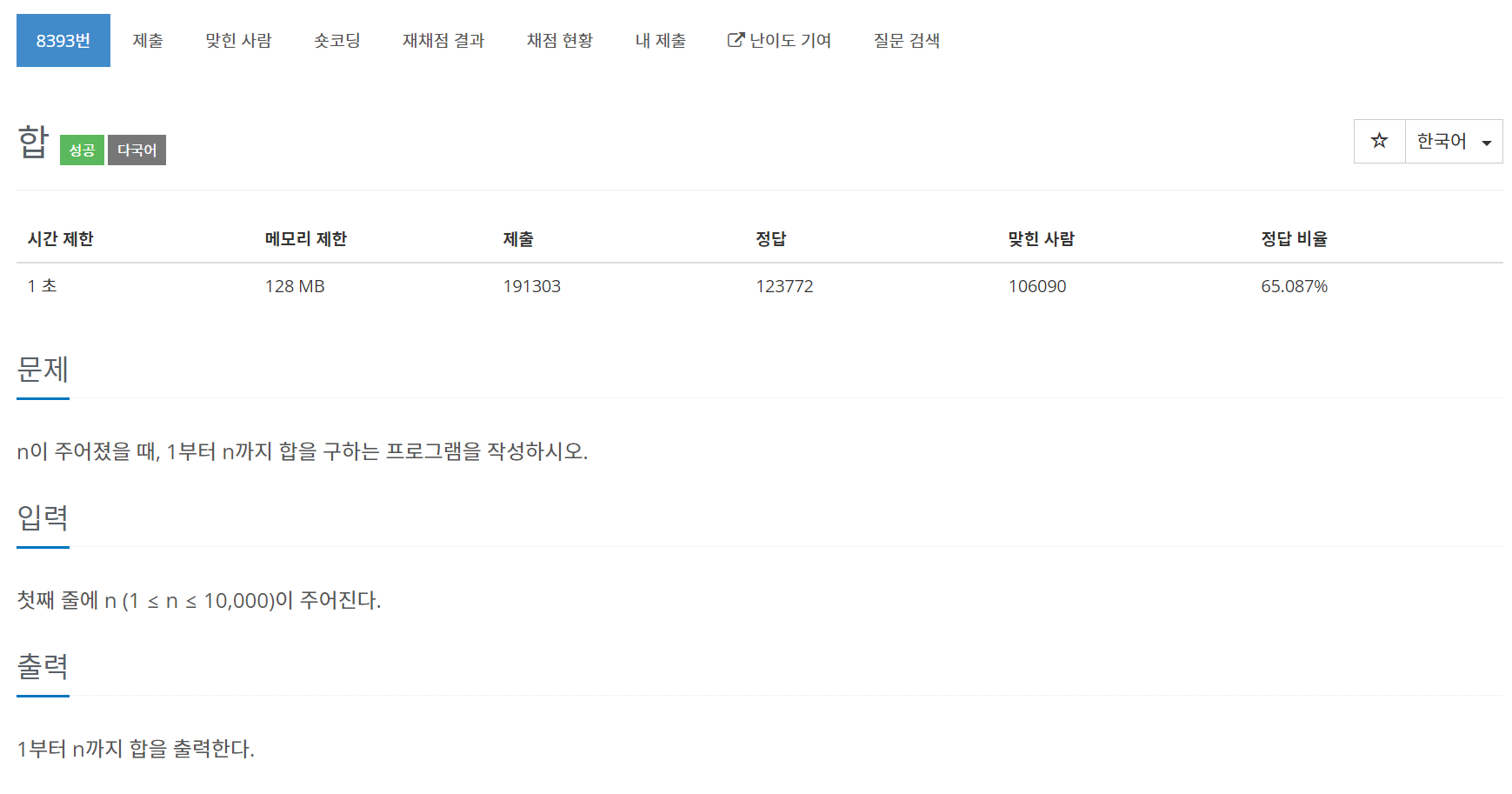
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int sum = 0;
for(int i = 1; i <= n; i++) {
sum += i;
}
System.out.println(sum);
sc.close();
}
}
25304번 영수증
문제
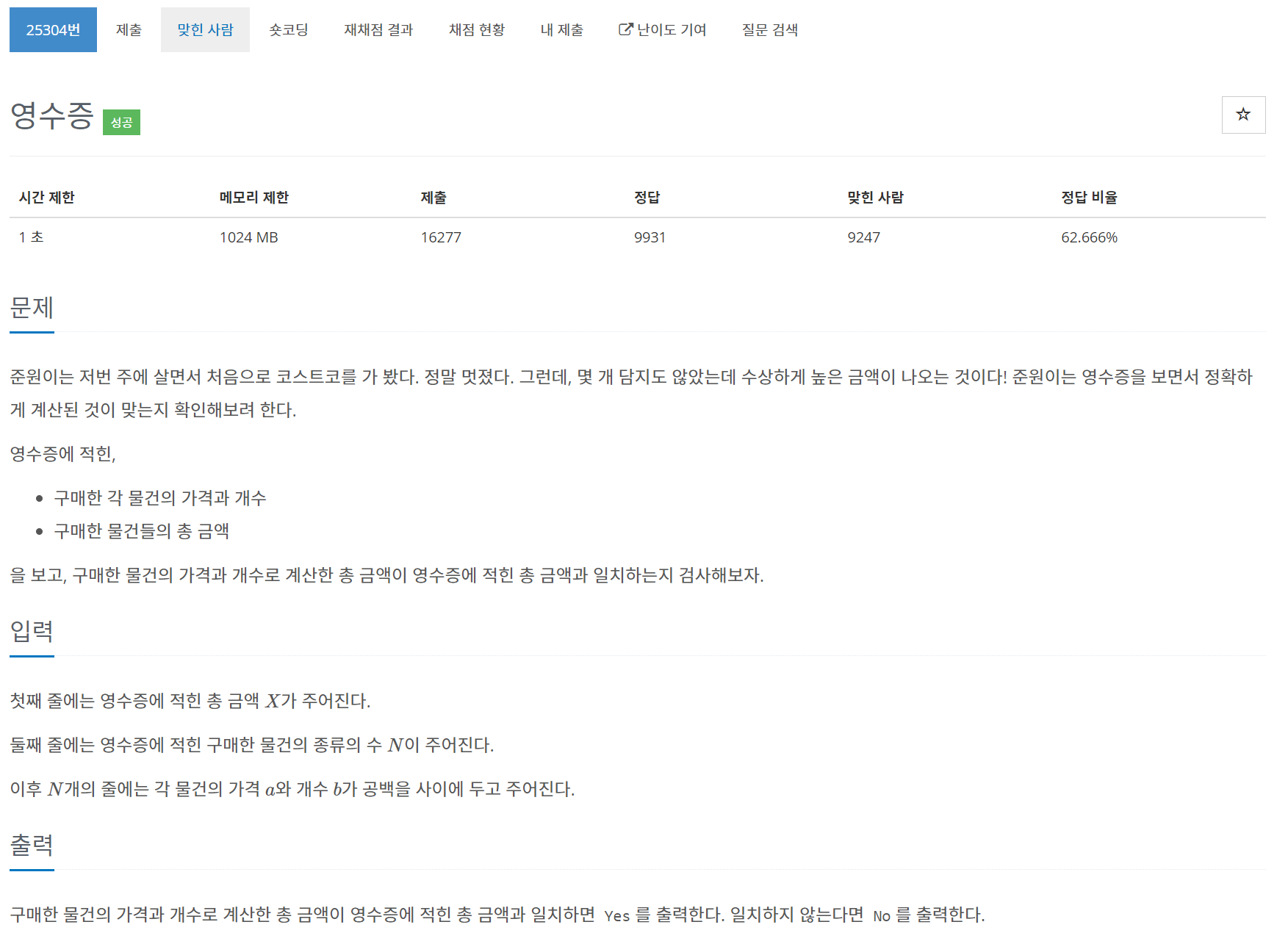
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int sum = sc.nextInt();
int n = sc.nextInt();
for(int i = 0; i < n; i++){
int money = sc.nextInt();
int num = sc.nextInt();
sum -= money * num;
}
if(sum == 0) {
System.out.println("Yes");
} else {
System.out.println("No");
}
sc.close();
}
}
15552번 빠른 A+B
문제
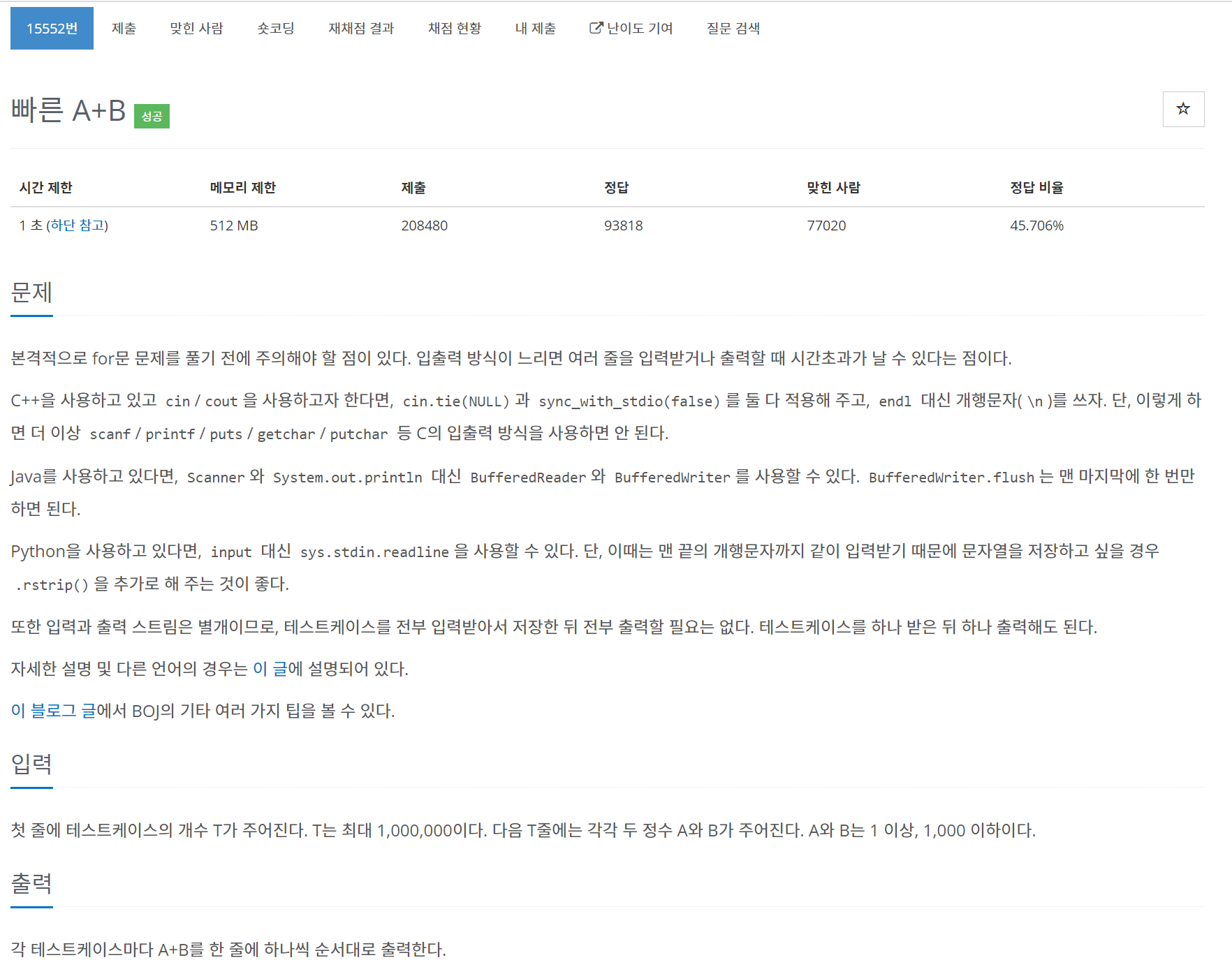
풀이
import java.io.*;
import java.util.StringTokenizer;
public class Main {
public static void main(String[] args) {
try {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
StringTokenizer st;
int n = Integer.parseInt(br.readLine());
int a = 0;
int b = 0;
int sum = 0;
for(int i = 0; i < n; i ++) {
st = new StringTokenizer(br.readLine());
a = Integer.parseInt(st.nextToken());
b = Integer.parseInt(st.nextToken());
sum = a + b;
bw.write(sum + "\n");
}
bw.flush();
bw.close();
}
catch (IOException e) {
e.printStackTrace();
System.out.println(e.getMessage());
}
}
}
11021번 A+B - 7
문제
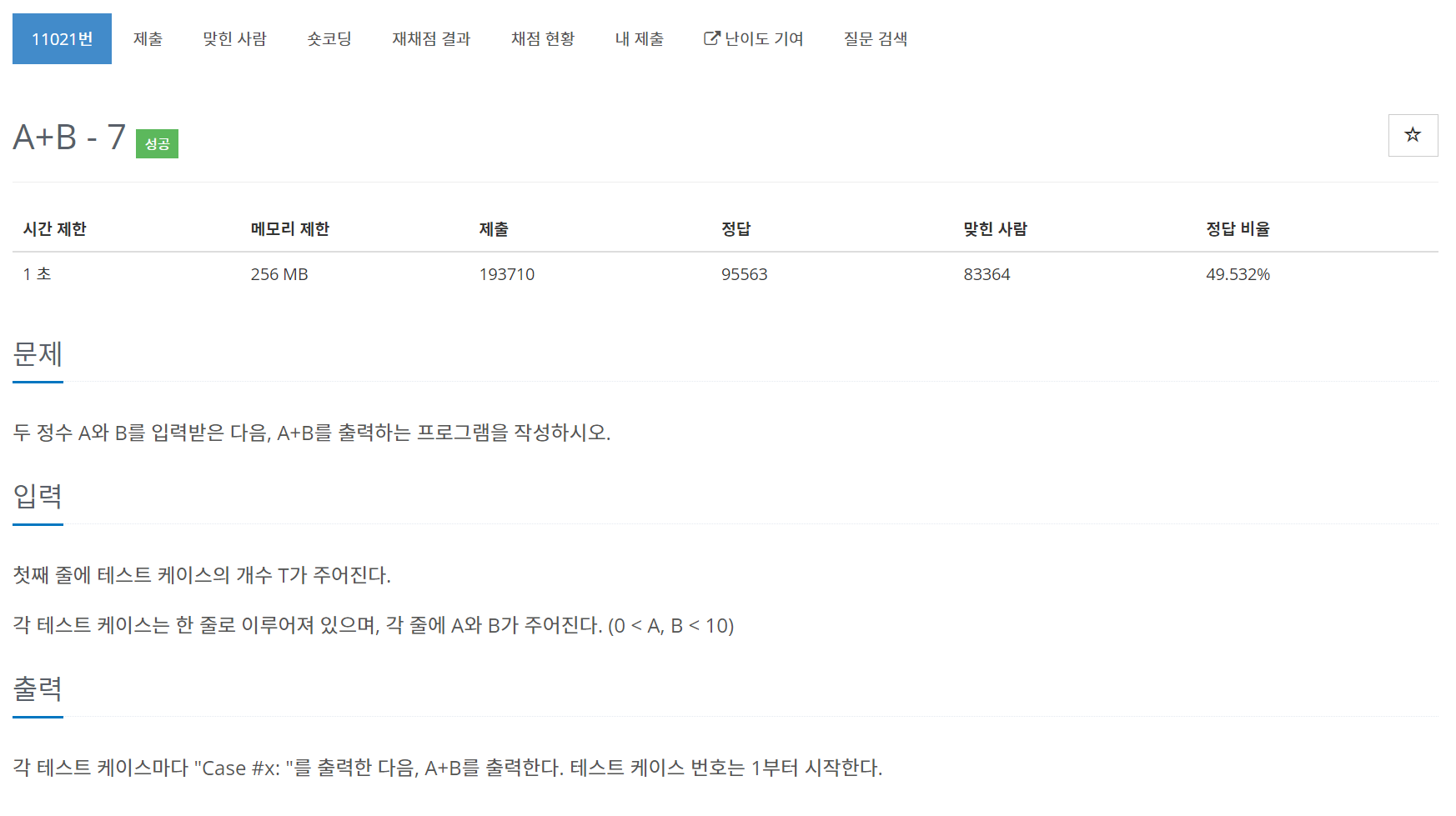
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int a = 0;
int b = 0;
for(int i = 1; i <= n; i++) {
a = sc.nextInt();
b = sc.nextInt();
System.out.println("Case #" + i + ": " + (a + b));
}
sc.close();
}
}
11022번 A+B - 8
문제
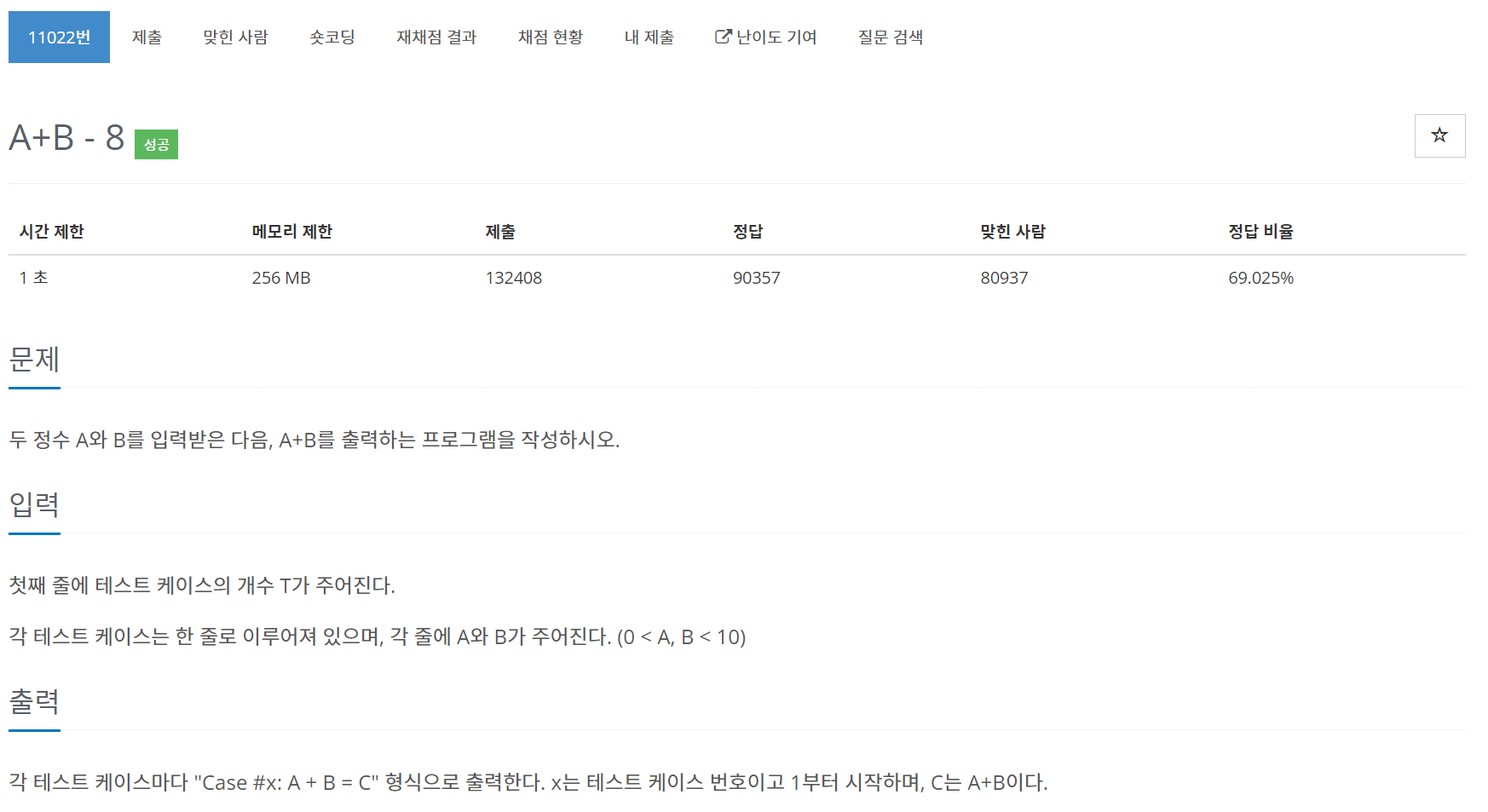
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int a = 0;
int b = 0;
for(int i = 1; i <= n; i++) {
a = sc.nextInt();
b = sc.nextInt();
System.out.println("Case #" + i +": " + a + " + " + b + " = " + (a + b));
}
sc.close();
}
}
2438번 별 찍기 - 1
문제
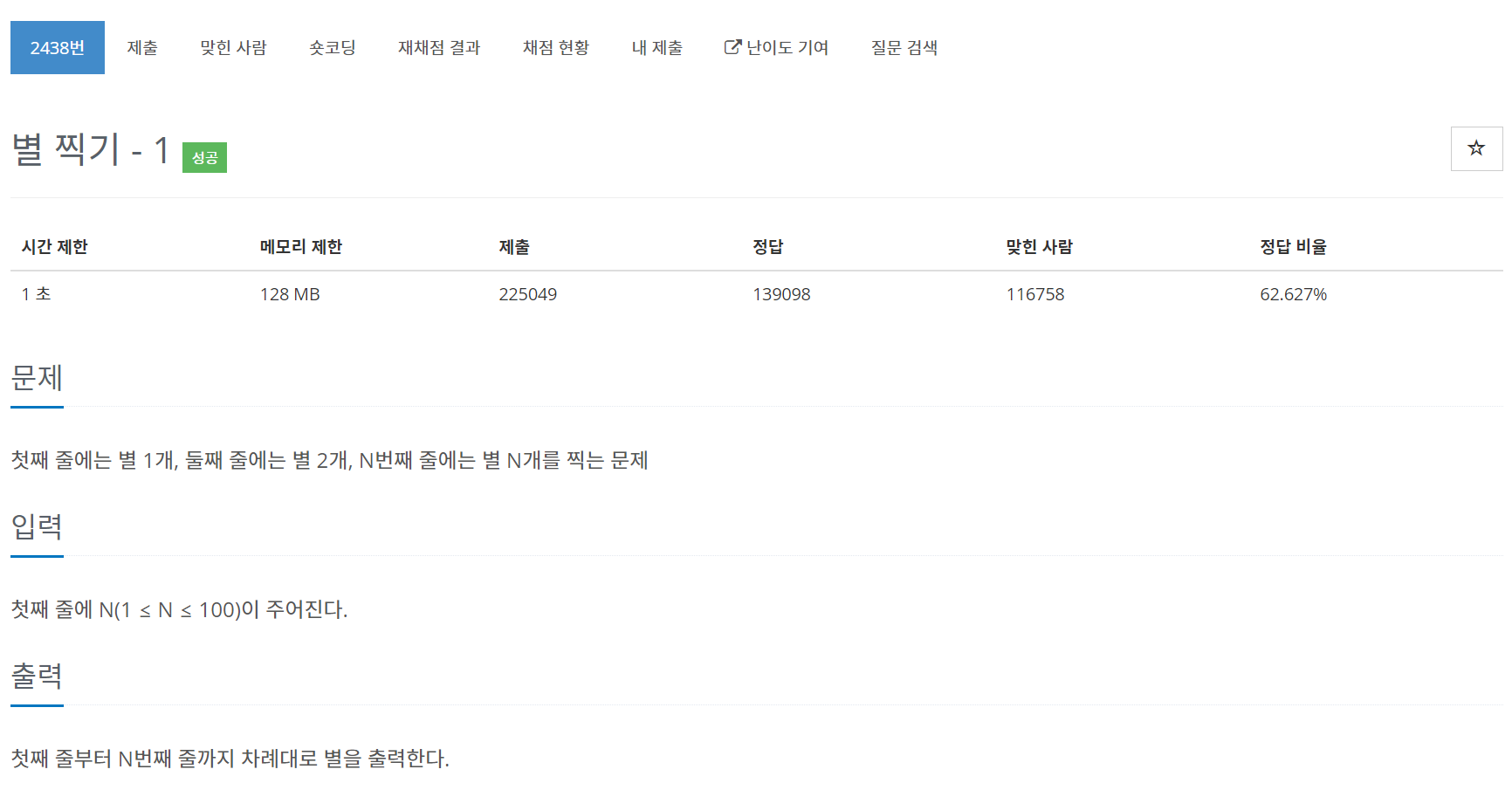
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for(int i = 0; i < n; i++) {
for(int j = 0; j < i + 1; j++) {
System.out.print("*");
}
System.out.print("\n");
}
sc.close();
}
}
2439번 별 찍기 - 2
문제
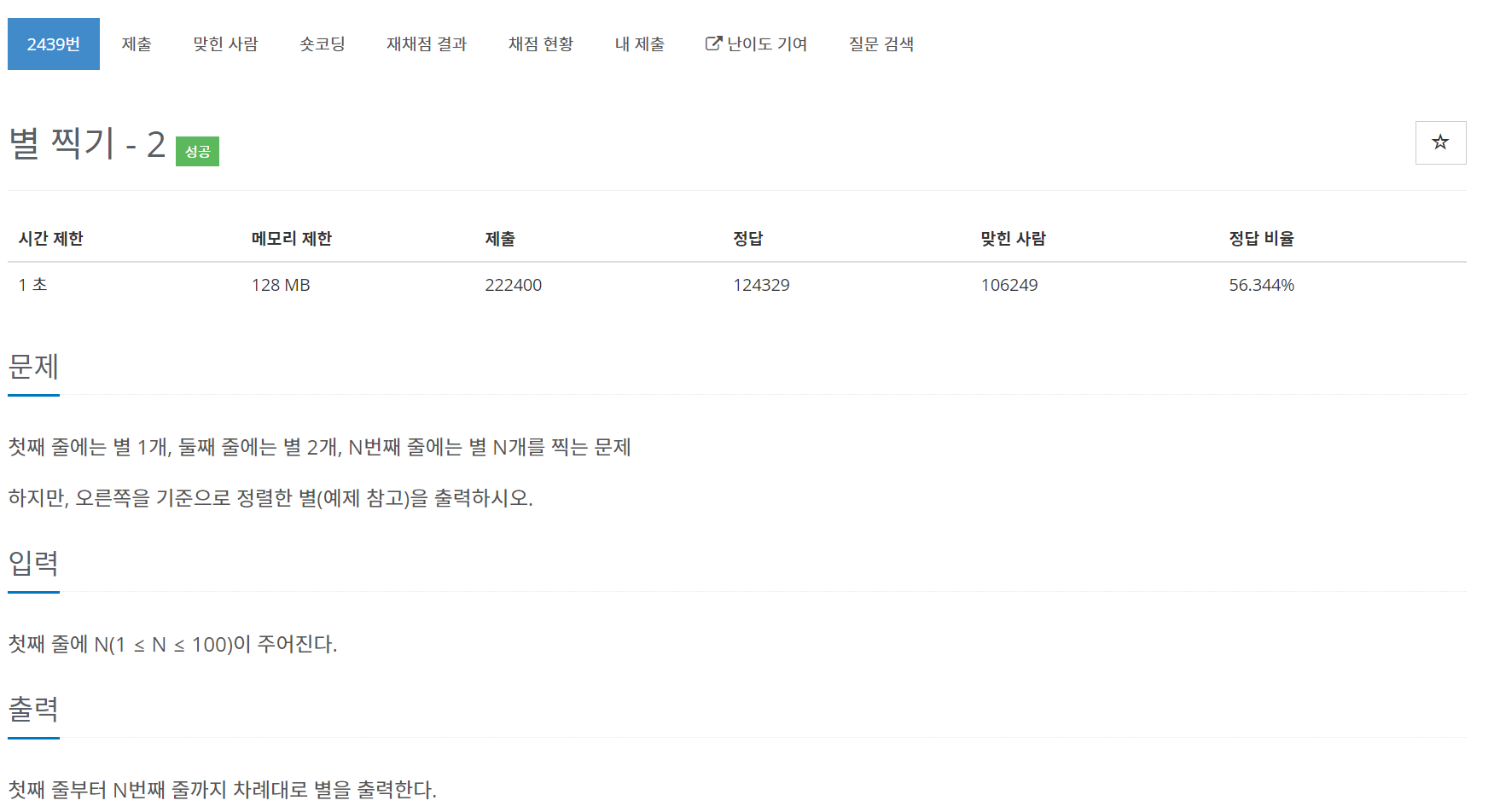
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for (int i = 1; i <= n; i++) {
for (int j = n; j > i; j--) {
System.out.print(" ");
}
for (int j = 1; j <= i; j++) {
System.out.print("*");
}
System.out.println();
}
sc.close();
}
}
10871번 X보다 작은 수
문제
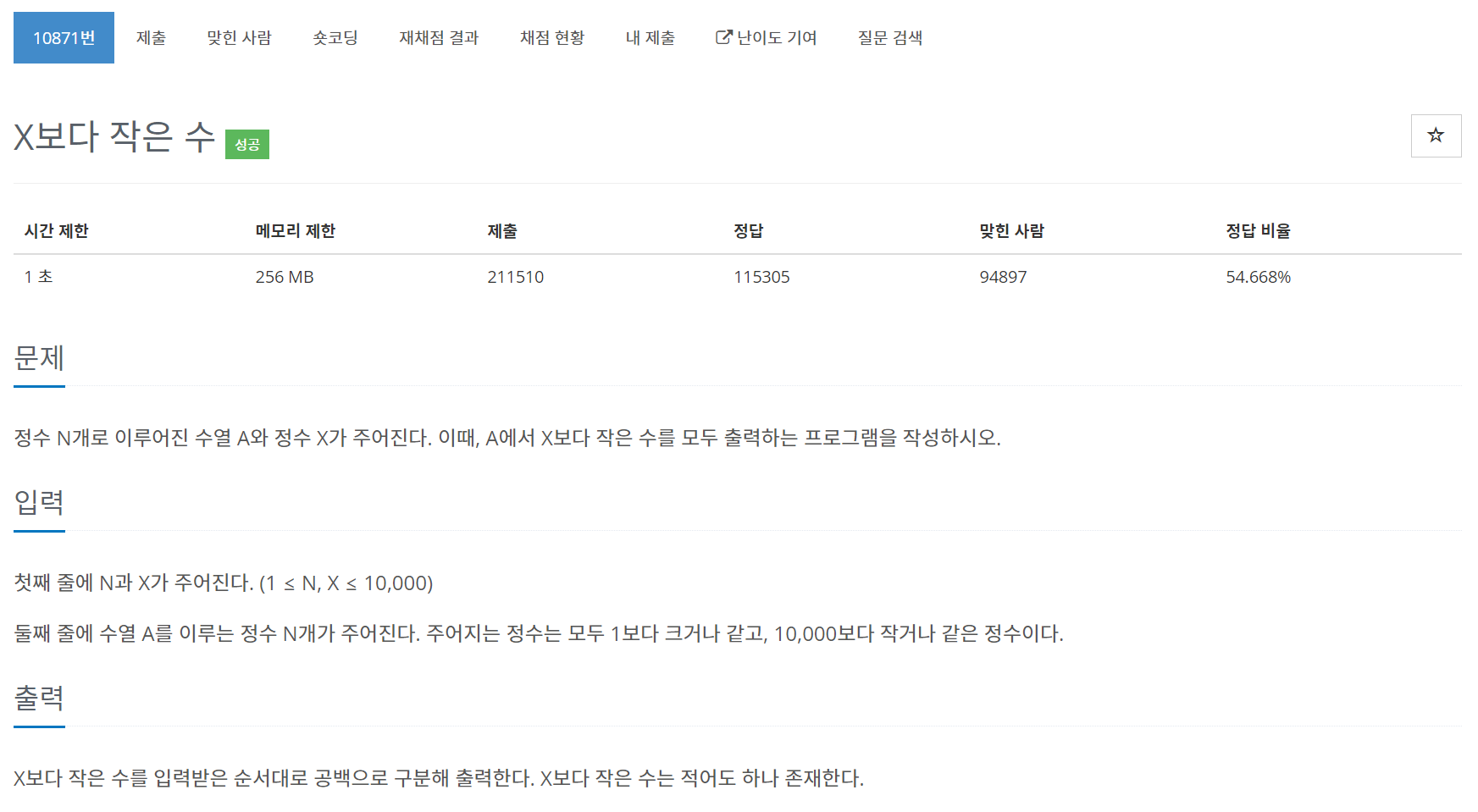
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int a = sc.nextInt();
int[] arr = new int[n];
for(int i = 0; i < n; i++) {
arr[i] = sc.nextInt();
}
for(int i = 0; i < n; i++) {
if(arr[i] < a) {
System.out.print(arr[i] + " ");
}
}
sc.close();
}
}
10952번 A+B - 5
문제
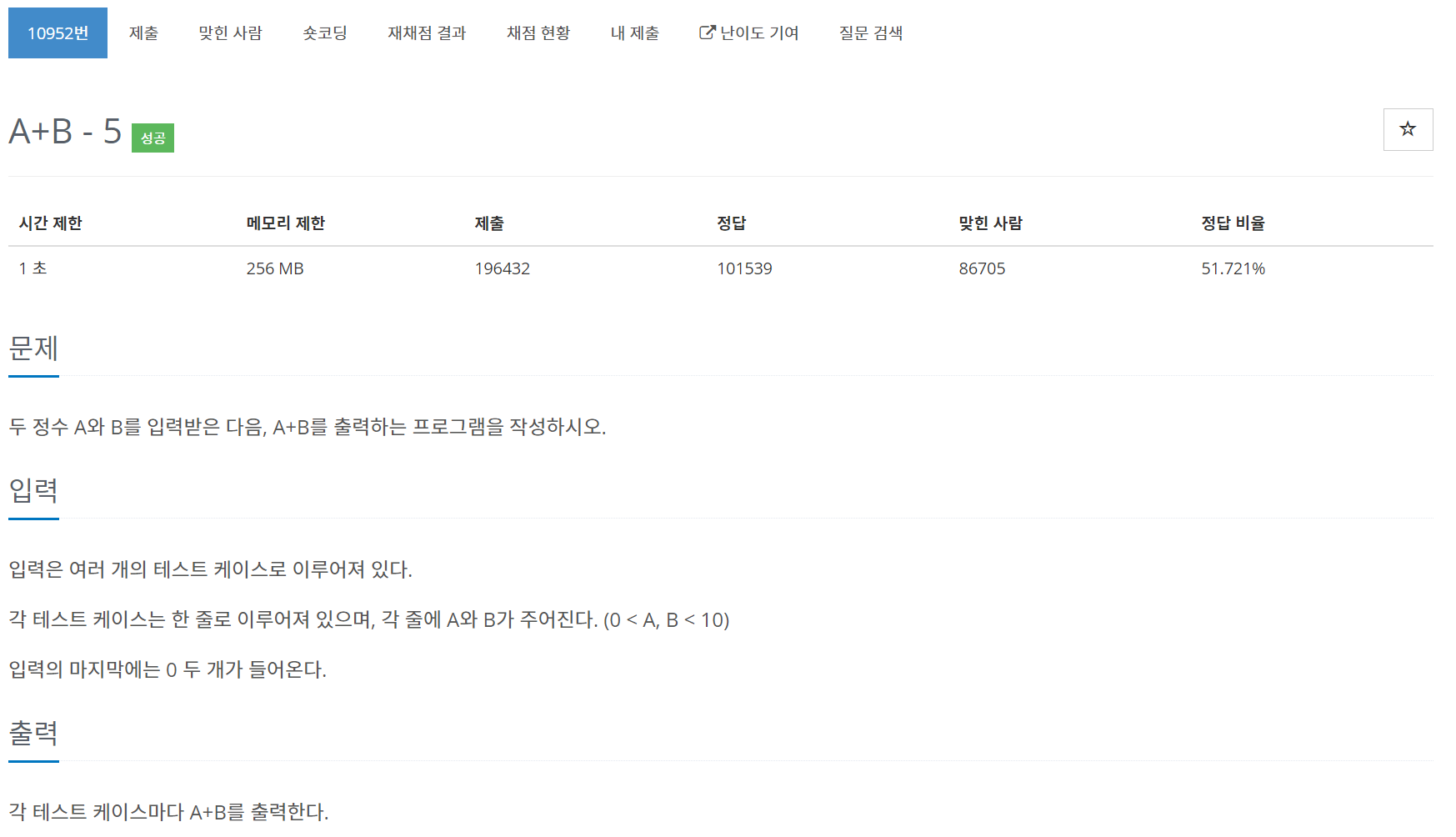
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = 0;
int b = 0;
while(true) {
a = sc.nextInt();
b = sc.nextInt();
if(a == 0 && b == 0) {
break;
} else {
System.out.println(a + b);
}
}
sc.close();
}
}
10951번 A+B - 4
문제
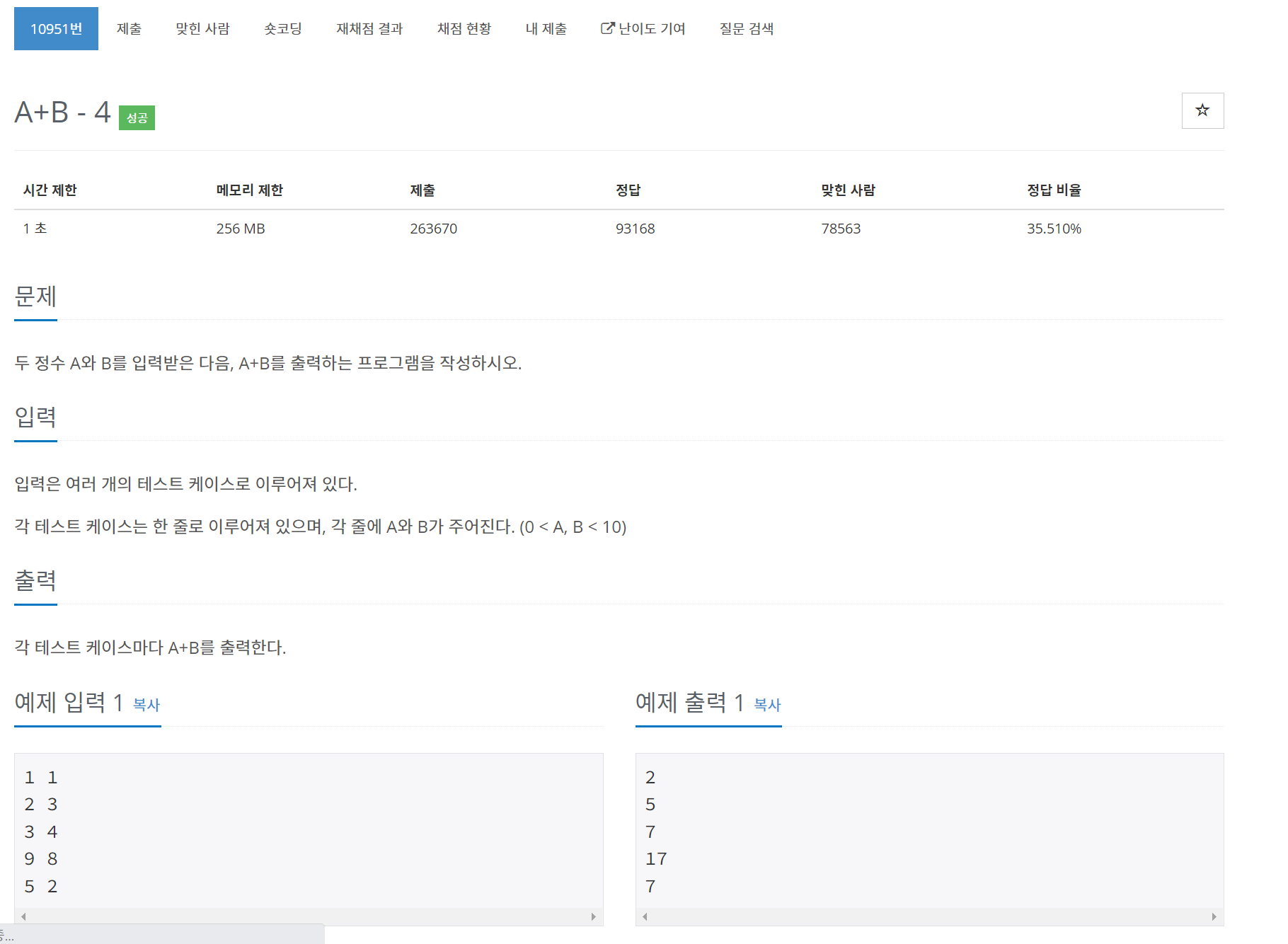
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = 0;
int b = 0;
while(sc.hasNextInt()) {
a = sc.nextInt();
b = sc.nextInt();
System.out.println(a + b);
}
sc.close();
}
}
1110번 더하기 사이클
문제
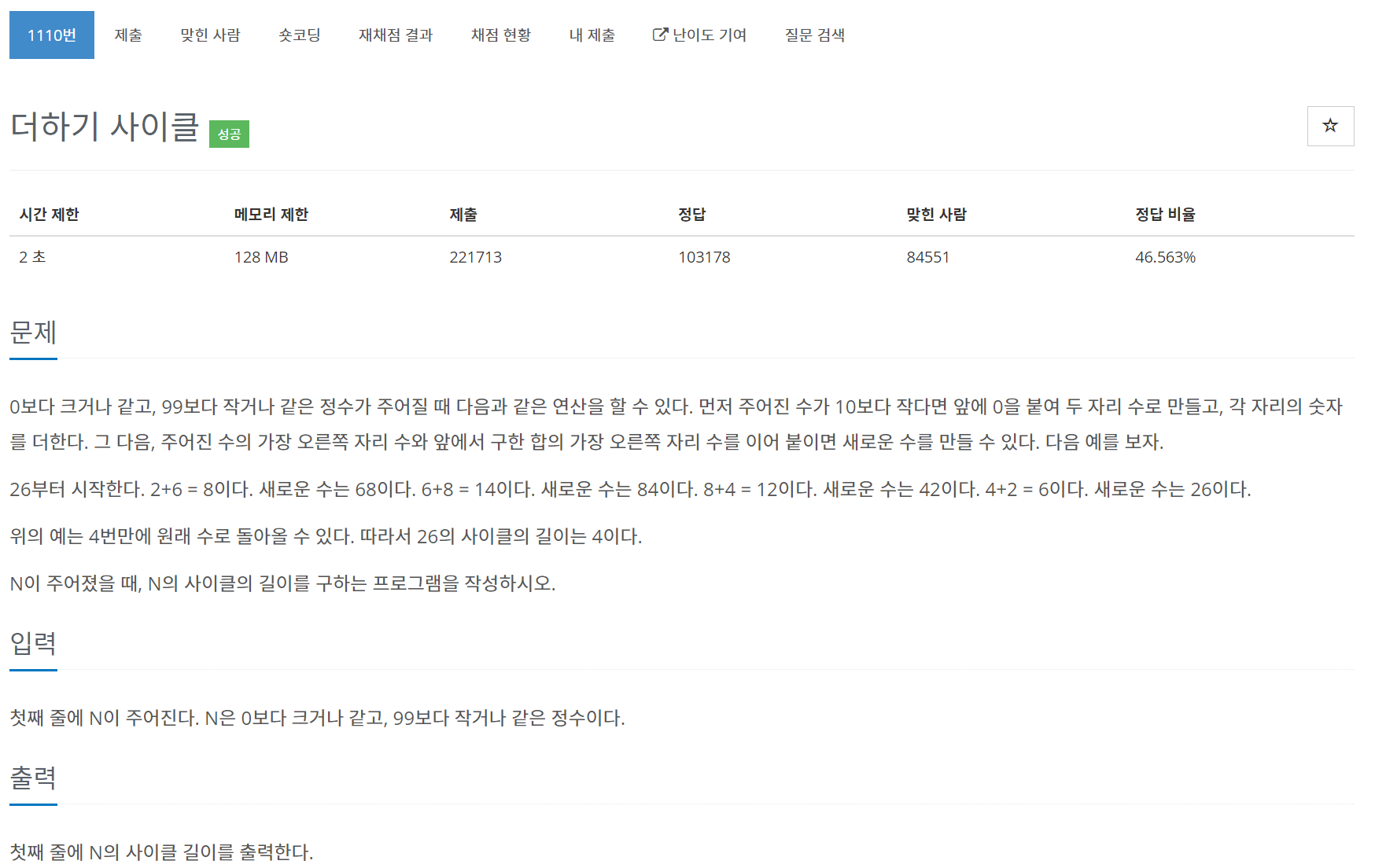
풀이
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
int nn = n;
int cnt = 0;
int a = 0;
int b = 0;
while(true) {
a = nn / 10;
b = nn % 10;
nn = b * 10 + (a + b) % 10;
cnt++;
if(nn == n) {
break;
}
}
System.out.println(cnt);
sc.close();
}
}