1. Representation of numbers
1) Intro
- 유한한 비트수로 숫자를 표현해야한다.
- floating point representation
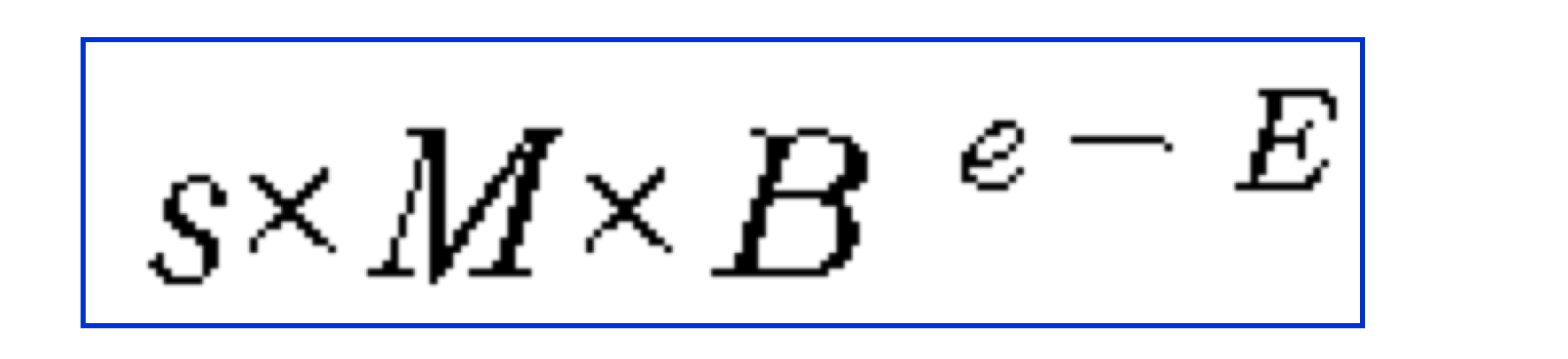
s: sign bit
M: exact positive integer mantissa - 부동 소수점 숫자의 실제 값을 나타내는 부분
B: base( 2진수 혹은 16진수 )
e: exact integer exponent
E: bias of the exponent (machine dependant)
2) IEEE Binary Floating Point Arithmetic Standard 754 - 1985
- Eg. Double precision real numbers (64 bits)

c: 11bit exponent( 0 ~ 2^11 -1 )
f: 52 bit binary fraction
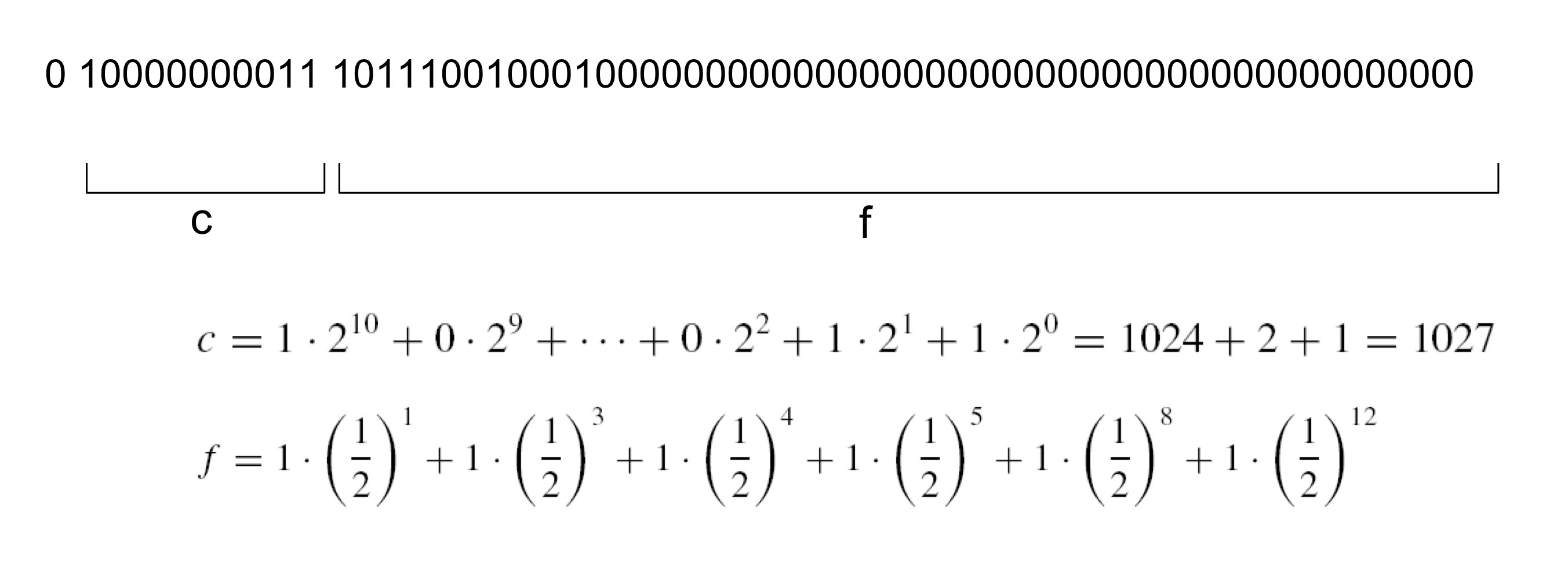
3) Eg. 64 bit floating point number
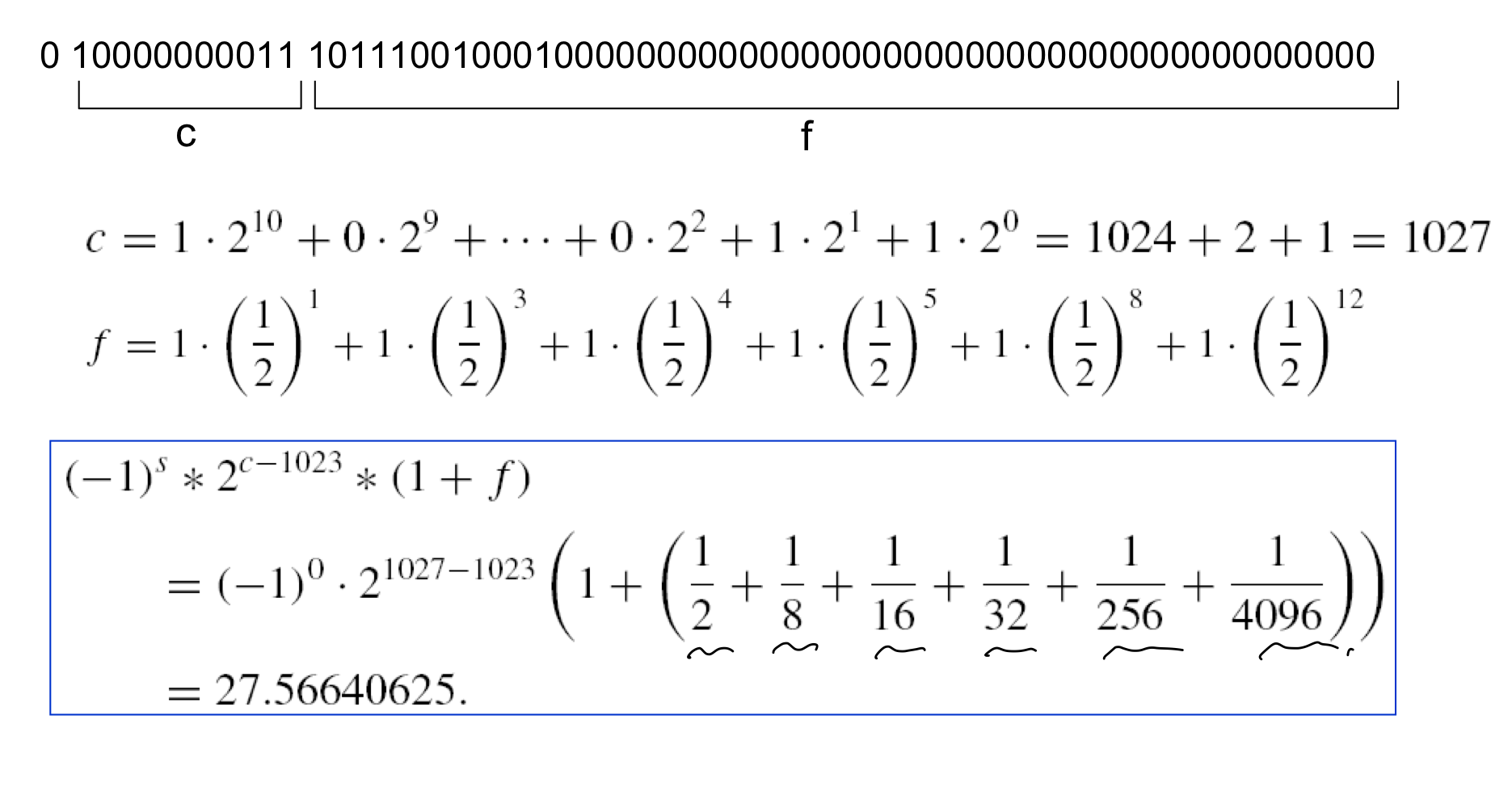
4) Accuracy, range…
- Accuracy
- Smallest number
- 2^-1022(1 + 0 ) ~~ 0.225 10^-307
- Largest number
- 2^1023(1+(1-2^-52)) ~~ 0.17977 10^309
- Underflow: 가장 작은 숫자보다 더 작을 때,
- Overflow: 가장 큰 숫자보다 더 클 때,
5) Machine Accuracy
- Def. 1.0에 더할 때, 1.0과 다른 부동 소수점 결과를 생성하는 가장 작은 부동 소수점 숫자
- 1.0이 숫자를 줄을 세운다면 가장 중앙에 위치하기 때문이다.

예: b=2(밑수가 2)이고 32비트 단어 길이를 가진 전형적인 기계는 대략 10^-8의 값을 가진다.
2. Errors
1) Roundoff error
- Machine accuracy 때문에 발생한다.
- Chopping
- 숫자를 가장 가까운 값으로 근사하는 것이 아니라, 필요 이상의 자릿수를 단순히 제거하는 방법이다. 예를 들어, 1.235를 소수점 둘째 자리까지만 나타내려고 할 때, 1.23만 나타낸다.
- Symmetric rounding
- 숫자를 가장 가까운 값으로 근사하는 표준적인 방법이다. 즉, 1.235가 1.24가 된다.
- 반올림 오차는 Machine accuracy과는 다르고(실수와 그 다음 실수 사이의 차이), 근사값을 사용 할 때 숫자를 표현할 때 발생하는 오차이다.
a. Minimizing roundoff errors
- Overflow나 Underflow를 피하기 위해서 중간 계산 값들을 +-1(모든 부동 소수점 숫자의 중간에 가까운 값) 주변에 유지한다.
- 오류의 누적을 최소화 하기 위해 산술 계산의 숫자를 최소화한다.
- Eg.) nested multiplication
- Avoid subtracting cancellation
- 두 수치가 상당히 유사 할 때, 뺄셈은 눈에 띄는 오차를 초래 할 수 있다.
- 이를 피하기 위해 가장 작은 숫자부터 연산을 시작한다.
- Use double precision
b. Loss of significant digit
- Eg. Evaluate F(x) = x(sqrt(x+1)-sqrt(x)) at x = 100 with 6s (significant digit = 6). True value: F(100) = 4.98765
- Method 1: Direct calculation sqrt(x+1) = 10.0498, sqrt(x) = 10.0000 x(sqrt(x+1)-sqrt(x)) = 100(10.0498-10.0000) = 4.98000 Error = 0.00756
- Method 2: Modification of fomula F(x) = x/(sqrt(x+1)+sqrt(x)) = 4.98758 Error = 0.00002
2) Truncation error
- 정확한 해와 실제 기계를 사용하여 계산된 해 사이의 오차
- 공식의 근사 때문에 발생한다.
- 수치 해석의 목표는 truncation error를 줄이는 것이다.
- Round-off error는, 제어 할 수 없다. (depends on machine)
- Truncation error는 algorithm에 의존한다. (어떻게 우리가 계산했는지.)
3) Error
- Absolute error: Et = (true value - approximation)
- Relative error: et = (true value - approximation) / true value
- Approximate relative error : ea = (Approx.TrueValue - approxi) / Approx.TrueValue
- 반복 알고리즘에서 종료하는 조건,
4) Data errors
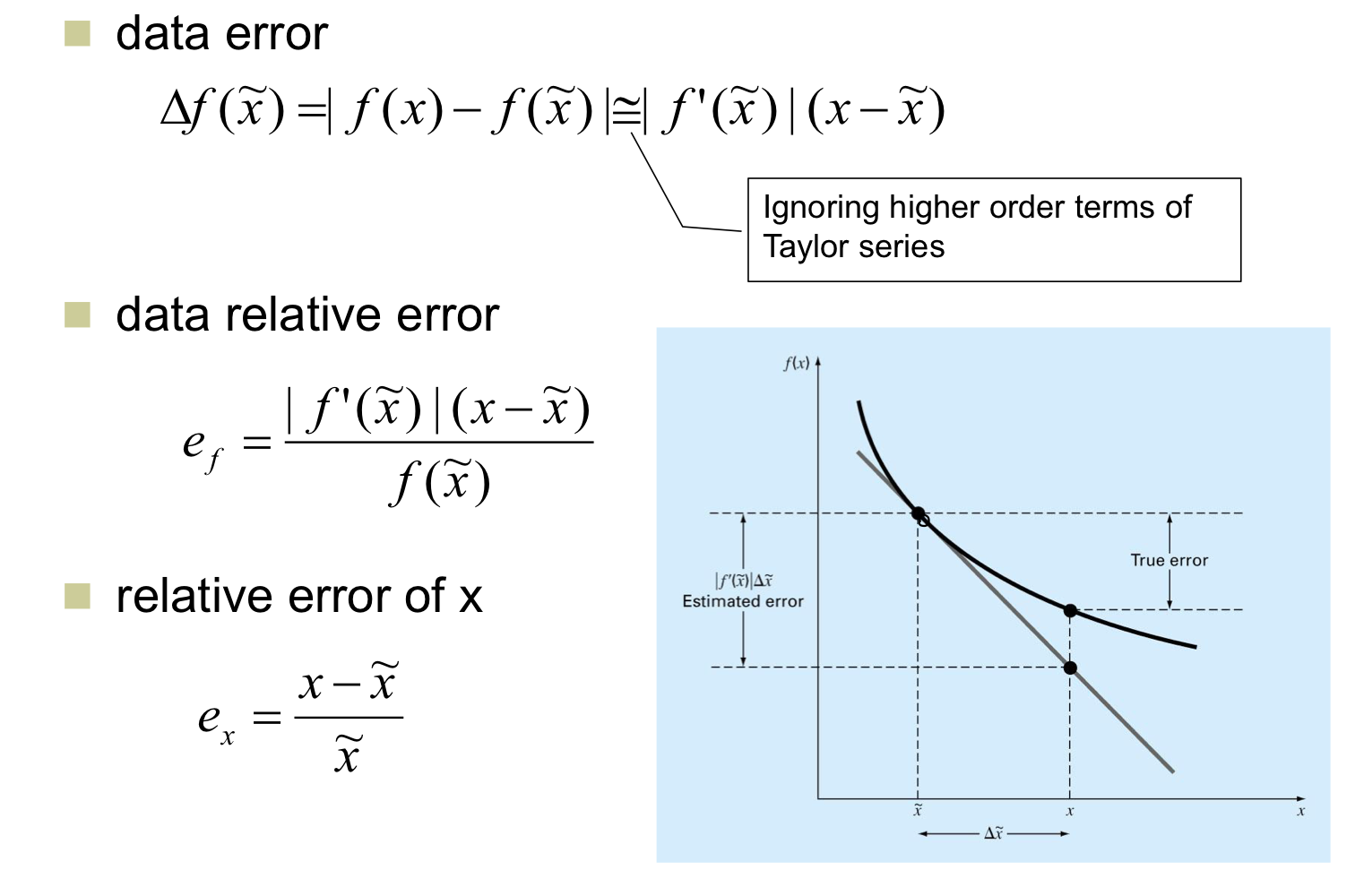
a) Condition Number
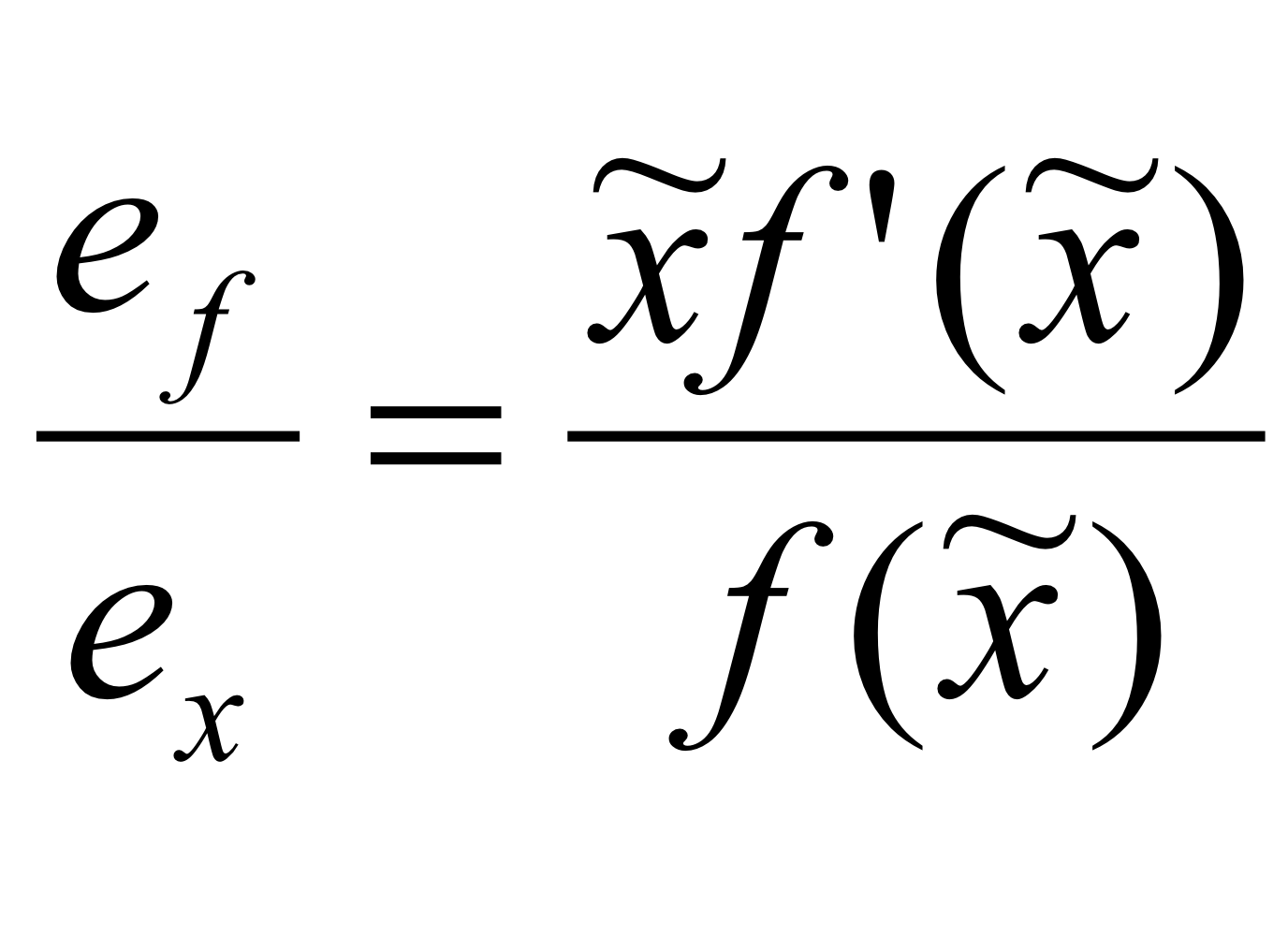
- if condition number < 1 → error reduction
- if condition number >> 1 → ill-conditioned
b) Error propagation
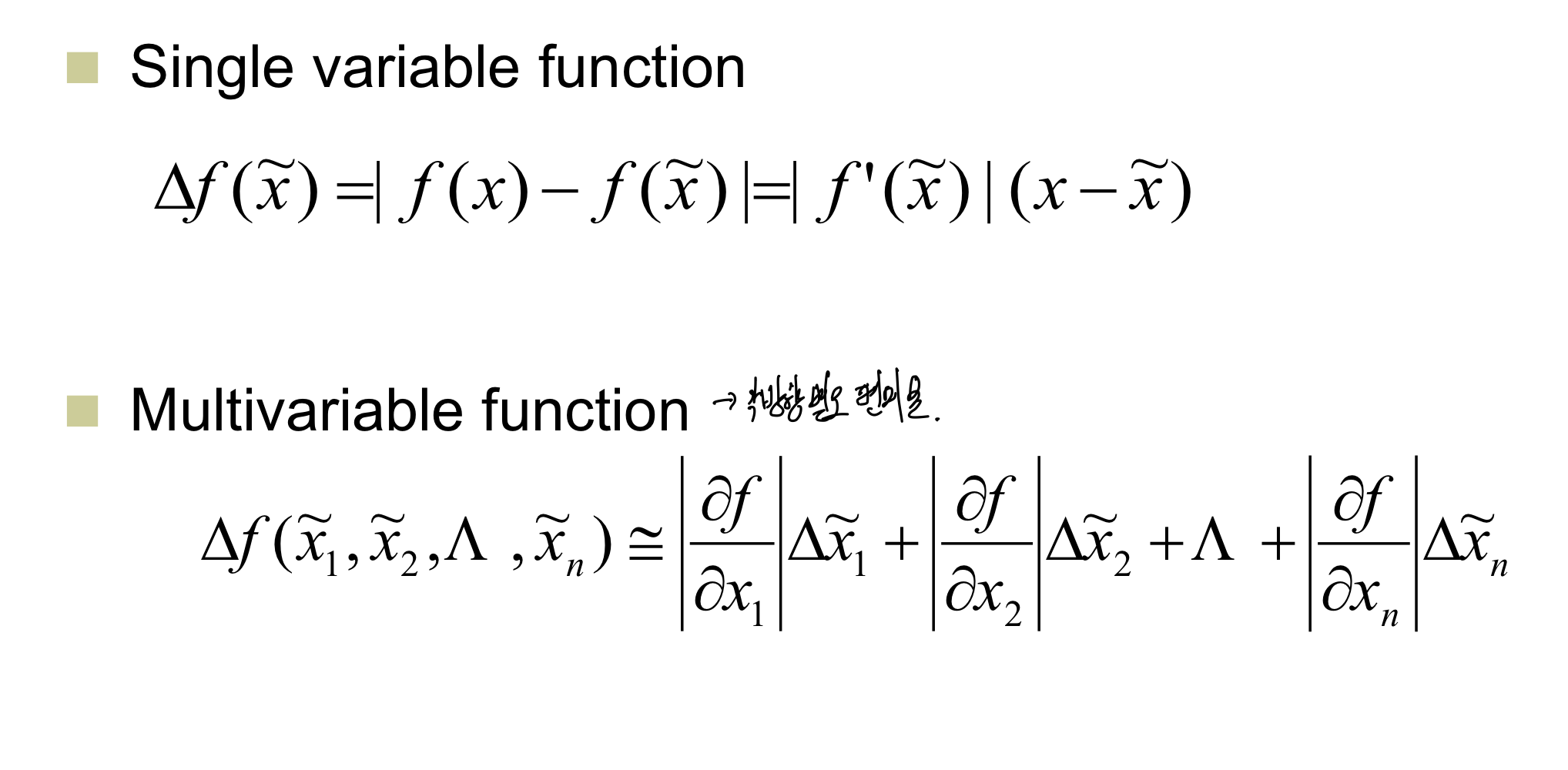
c) Total error
- Total error = roundoff error + truncataion error
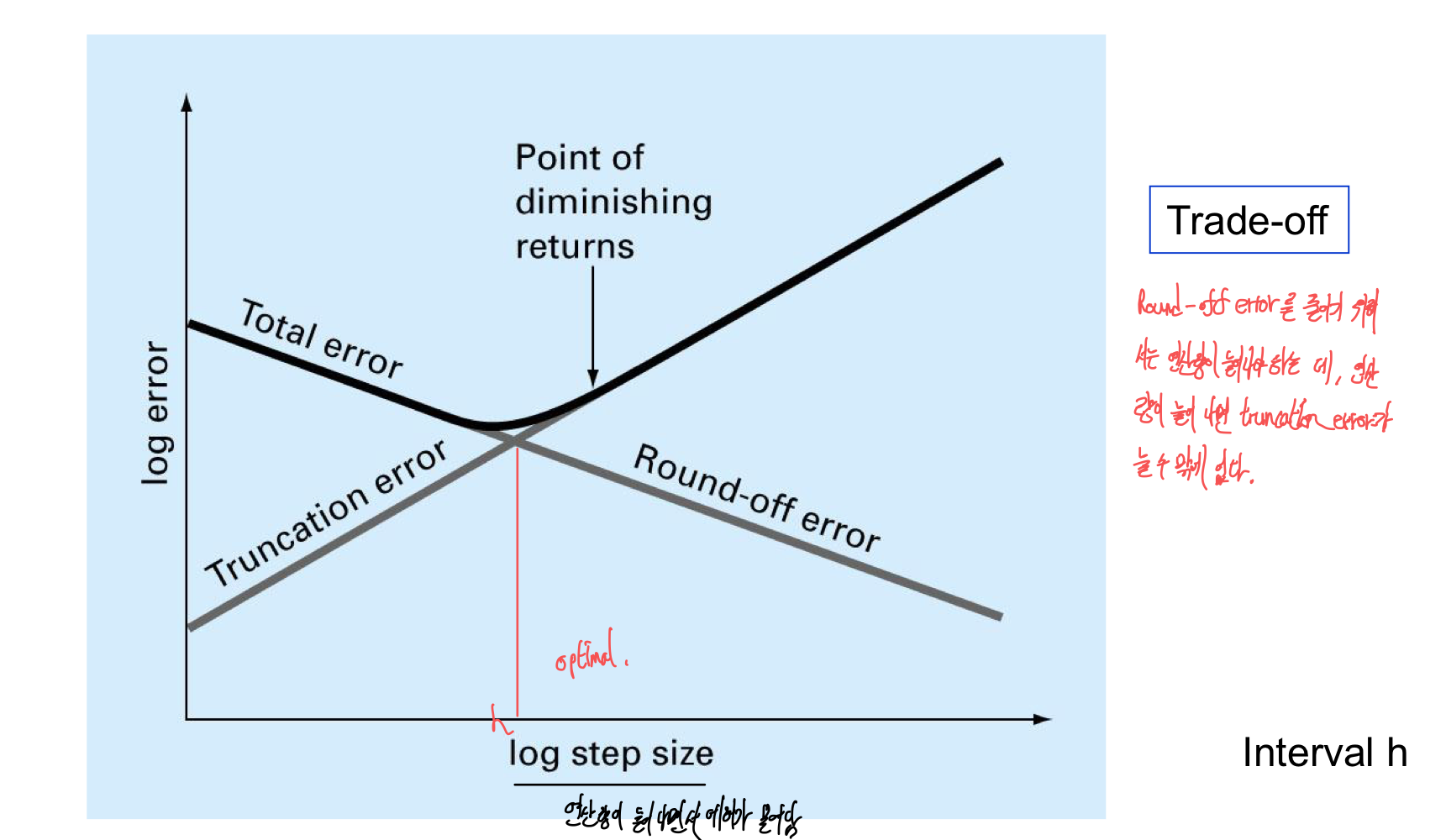