- 매우 큰 데이터에 대해서도 저장할 수 있어야 한다.
- 프로세스가 끝나더라도 정보는 유지되어야 한다.
- Multi-processes가 concurrently하게 정보에 접근할 수 있어야 한다.
File system
- Secondary storage를 위하여 abstraction하게 구현 (file)
- File을 논리적으로 조직한다 (directories, folder)
- 사람/프로세스/기계 간에 공유를 허용한다 (sharing)
- 데이터를 원치 않은 접근으로부터 보호한다 (protection)
File (file + directories)
- Secondary storage에 저장된 연관된 데이터들의 모음
- OS는 files를 통하여 information storage에 대한 일관된 논리적 관점을 제공한다.
File structures
- Flat : byte sequence
- Structured : Lines, Fixed length record, variable length records
- Text file : 각 언어별로 unified된 코드의 형태로 저장 (.txt / .cpp / 헤더파일etc.)
- Binary file : 데이터를 코드화하는 것이 아닌 데이터 자체가 의미가 있다. (.exe / .dll etc.)
- 아래한글이나 ppt파일 같은 경우 헤더에 일부의 text file이 존재하지만, 그림과 같은 데이터도 포함하기 때문에 전체적으로 binary file로 볼 수 있다.
File operations
int creat (const char *pathname, mode_t mode);
int open (const char *pathname, int flags, mode_t mode);
int close (int fd);
ssize_t read (int fd, void *buf, size_t count);
ssize_t write (int fd, const void *buf, size_t count);
off_t lseek (int fd, off_t offset, int whence);
int stat (const char *pathname, struct stat *buf);
int chmod (const char *pathname, mode_t mode);
int chown (const char *pathname, uid_t owner, gid_t grp);
int flock (int fd, int operation);
int fcntl (int fd, int cmd, long arg);
File types
Understood by file systems
- File system의 종류에 따른 유형 : device, directory, symbolic link etc.
Understood by other parts of OS or runtime libraries
- Executable, dll, source code, object code, text etc.
Understood by application programs
- Jpg / mpg / avi / mp3 etc.
Encoding file types
Windows encodes type in name (extension : .앞에 이름을 붙일 수 있다)
- .com, .exe, .bat, .dll, .jpg, .avi, .mp3, etc.
Unix encodes type in contents (extension이 존재하지 않는다)
- magic numbers (e.g., 0xcafebabe for Java class files)
- initial characters (e.g., #! for shell scripts)
file access
Sequential access (제일 많이 사용한다)
- read bytes one at a time, in order
- 실행 파일을 메모리에 올려서 순차적으로 읽어서 사용하며, 일반적으로 많이 사용하는 방식
Direct access
- random access given block/byte number
- 원하는 위치의 데이터만을 읽게 한다.
Record access
- File is an array of fixed- or variable-length records, read/written sequentially or randomly by record #
- Direct access같은 경우 record단위로 읽고 쓰는 등의 작업을 수행한다.
Index access
- File system contains an index to a particular field of each record in a file, reads specify a value for that field and the system finds the records via the index (DBs)
- Record access의 경우 파일은 탐색하는 것이 잦기 때문에 키를 기반으로 한 인덱스를 정의한 후 탐색을 진행한다.
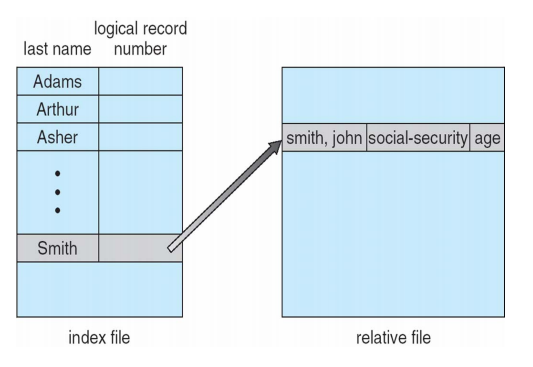
Directories (folder in Windows)
- 유저를 위해서 구조화된 파일 형식을 제공한다.
- File system을 위해서 disk에 물리적인 파일의 위치 정보와 logical file 조직의 구현을 구분하는 편리한 naming interface를 제공한다.
- 특별한 metadata를 가지고 있는 파일이다. List of (file name, file attributes)
- Attributes : size, protection, creation time, access time, location on disk
- 보통 정렬되어 있지 않다
Operations
- Directory는 file안에 구현되어 있기 때문에 파일 operation으로 조작할 수 있다.
- C runtime 라이브러리는 directories를 읽기 위하 높은 추상화 수준을 제공한다.
- Opendir / readdir / seekdir / closedir etc.
Pathname translation
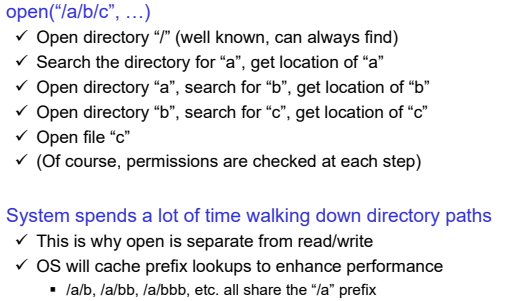
File system mounting
Windows의 경우
- 용량이 부족한 경우 C drive, D drive 등 drive를 사서 부착한다.
UNIX / LINUX의 경우
- Drive의 개념 없이 root directory에서 시작한다.
- Drive를 부착하기 위해서는 파일 시스템을 만들고 OS가 사용할 수 있도록 Mount해야한다.
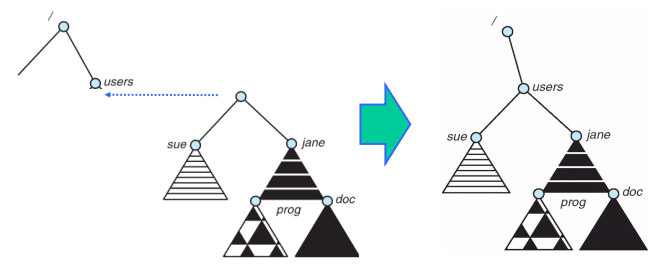
File sharing
- Network로 연결된 다른 컴퓨터의 파일을 local의 파일처럼 사용할 수 있게 한다.
- Windows의 경우에는G drive처럼 drive를 별도로 만들어서 network drive로 사용하며(CIFS) UNIX의 경우에는 NFS 표준을 따른다.
Protection
- 다른 사용자나 프로세스가 내 파일을 마음대로 읽고, 변경하는 등의 작업을 하지 못하도록 한다.
Access control lists(ACLs) – 주로 사용
- 파일이나 directory같은 각각의 object 별로 허용된 작업을 리스트로 관리한다.
- 사용자가 많아지면 복잡해진다.
Capabilities
- 사용자 별로 허용된 작업을 리스트로 관리한다.
- 파일이 하나 생길 때마다 파일별로 모든 사용자에게 작업을 해야 하기 때문에 복잡도가 높다.
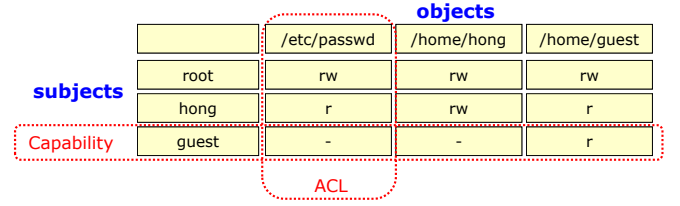
Access lists in UNIX/LINUX

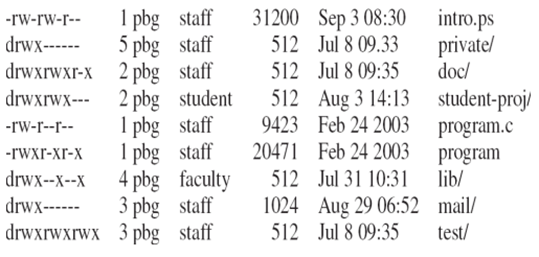
-
-의 의미 : regular file
-
D의 의미 : directory
-
directory에서 x의 의미
- 그 directory로 change directory를 할 수 있다
- X가 없으면 change directory 불가