Program
- Disk 또는 파일시스템에 저장된 실행 파일 (Executable file on a disk)
- 메모리로 로딩된 후 kernel에 의해 실행된다.
Process
- process(UNIX), job(mainframe), Task(IBM 360, RTOS)
- 메모리에서 실행되는 실행주체 (Executing instance of a program)
- A dynamic and active entity
- An encapsulation of the flow of control in a program
- process ID를 이용하여 이름이 지정된다.(PID, Integer타입)
- 실행과 스케줄링의 기본 단위
- 스케줄러 : 프로세스의 실행 순서를 지정(스케줄링과 Dispatch작업 담당)
Process Address Space
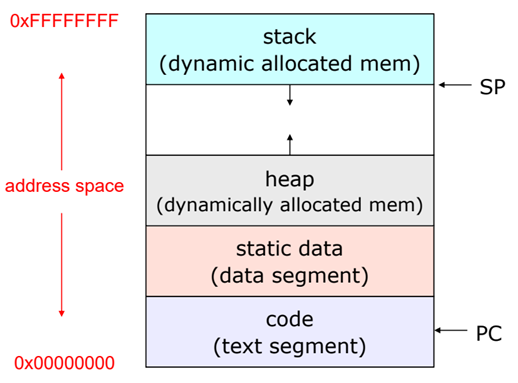
Code(text segment)
- CPU instruction이 있으며, 순차적으로 실행한다.
Data Segment
- 프로그램이 실행되는 동안 항상 메모리를 차지하는 static data, 전역변수 저장
Heap
- Dynamically memory allocated (DMA)에 사용, 동적 할당에 사용 EX) C++ new
Stack
- 지역 변수 저장, stack pointer 탑재, 무한루프 사용시 stack overflow 발생
main(){
func(10, 20)
}
int func(int a, int b) {
int c, d;
}
/*
stack data
return address
20 (int b)
10 (int a)
(int c)
(int d)
*/
Process State
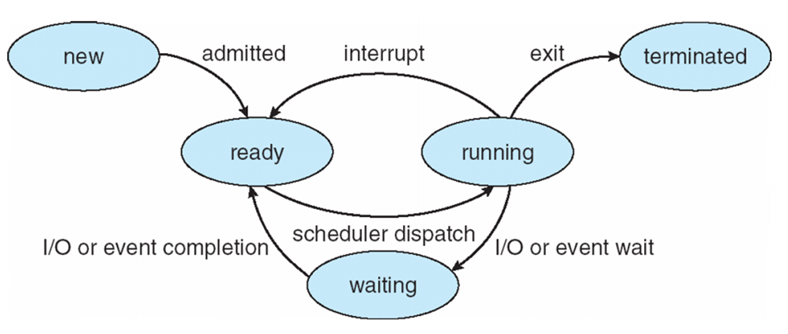
ready
- process를 실행 가능한 상태, ready상태 프로세스를 고르는 작업이 스케줄링
- 수행 가능하나 스케줄링에 의해 실행이 안되고 있는 상태
dispatch
- 스케줄링 된 ready상태의 프로세스를 CPU의 레지스터에 올리는 작업
waiting
- I/O나 Event의 발생까지 대기하거나 disk I/O가 이루어질 때까지 기다리는 상태
- I/O나 이벤트의 발생까지 실행이 불가능한 상태
Waiting상태에서 Running상태로 전환될 수 없다.
- waiting > Ready > Running
idle process
- running상태로 전환될 프로세스가 없는 경우 CPU의 활성화를 위하여 idle process를 runnning한다.
- running상태가 아닐 때는 항상 ready상태이며 우선순위가 가장 낮다.
LINUX Example
- ps명령어를 통하여 process state를 열람할 수 있따.
- R(runnable) : 실행 중인 프로세스
- S(sleeping) : Waiting상태
- T(traced or stopped) : 사용자의 명령어에 의해 잠시 중단된 상태
- D(uninterruptible sleep) : 메인 메모리에 존재하지 않는 상태
- Z(zombie) : 프로세스가 종료된 후에 커널 안에 남아있는 상태
Process Control Block(PCB, 프로세스의 정보를 관리하는 Data Structure)
- process state
- program counter
- CPU register
- CPU Scheduling information
- Memory-management information
- Accounting information
- I/O status information
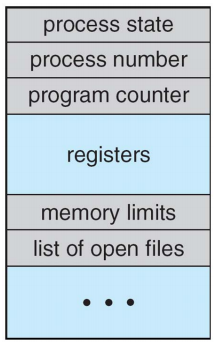
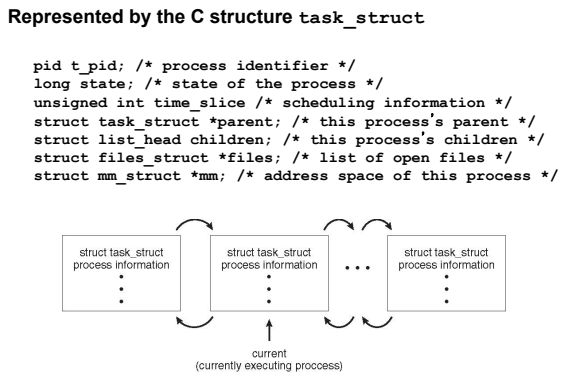
Context switch(CPU Switch)
administrative overhead
- saving and loading registers and memory maps
- flushing and reloading the memory cache
- updating various tables and lists
- overhead가 발생함으로써 성능이 낮아지지만 response time이 향상되기에 사용한다.
Schedulers (OS라고 볼 수 있다)
Long-term scheduler (or job scheduler)
- Virtual Memory Management로 인해 쇠퇴
- 어떤 process를 main memory의 ready queue에 올릴지를 결정한다.
Short-term scheduler (or CPU scheduler)
- ready queue에 있는 프로세스 중에 어떤 것을 CPU에 올릴지를 결정한다.
Medium-term scheduler (or swapper)
- Virtual Memory Management로 인해 쇠퇴
- ready queue에 있는 프로세스를 다시 파일 시스템으로 내리는 것을 결정한다.
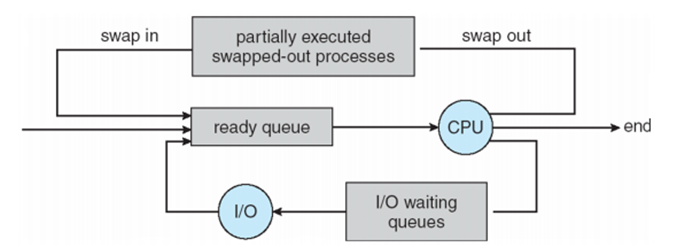
- VMM : Main memory를 무한하다고 가정한다.
representation
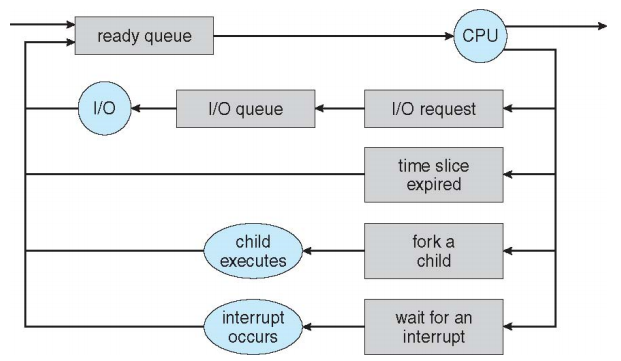
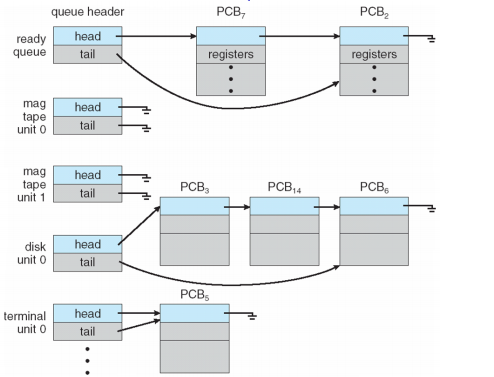
Operations on Processes
Process creation
#include <sys/types.h>
#include <unistd.h>
int main()
{
int pid;
if ((pid = fork()) == 0)
/* child */
printf (“Child of %d is %d\n”, getppid(), getpid());
else
/* parent */
printf (“I am %d. My child is %d\n”, getpid(), pid);
}
% ./a.out
I am 31098. My child is 31099.
Child of 31098 is 31099.
% ./a.out
Child of 31100 is 31101.
I am 31100. My child is 31101.
- 새로운 PCB와 Address space를 만들고 초기화한다.
- fork명령어가 수행되면 똑같은 process를 하나 더 만들고(parent – child), parent process의 address space에 있는 전체 content를 복사하고, parent에 의해 사용되는 resource를 가리키는 kernel resource 또한 초기화한다.
- PCB를 Ready queue에 올린다.
- parent에게 child의 PID를, Child에게 0을 반환한다
- parent와 child의 실행 순서는 상황에 따라 다르다
- child사용의 유용점
- parent와 협업하며, parent의 데이터를 가지고 task를 수행한다 (웹서버에 많이 사용한다)
While (1) {
int sock = accept();
if ((pid = fork()) == 0) {
/* Handle client request */
} else {
/* Close socket */
}
}
- parent가 하던 작업을 이어받거나 가지고 있던 데이터를 기반으로 작업할 수 있다.
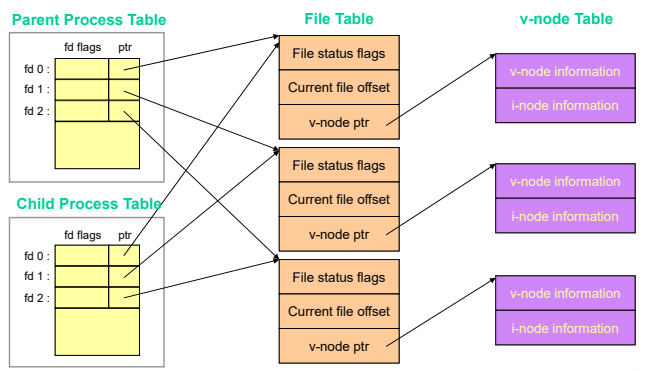
Process execution
-
exec() (system call) / int exec(char prog, char argv[]) [LINUX]
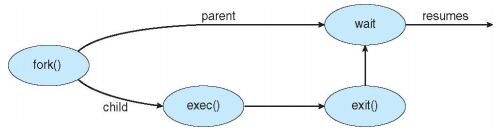
-
현재의 프로세스를 중단하고 프로그램을 로드하여 address space에 올린다.
-
프로세스를 중단하고 실행하기 때문에 fork 후에 exec가 수행된다.
-
exec()의 return value는 이전의 프로세스이기 때문에 실행 실패시에만 값을 반환한다.
-
child가 exec되고 parent는 wait()하면 child가 exit()를 호출하기를 기다린다.
int main()
{
while (1) {
char *cmd = read_command();
int pid;
if ((pid = fork()) == 0) {
/* Manipulate stdin/stdout/stderr for
pipes and redirections, etc. */
exec(cmd);
panic(“exec failed!”);
} else {
wait (pid);
}
}
}
- 백그라운드 상황에서는 부모 프로세스가 waiting하지 않음으로써 수행된다.
process tree in linux
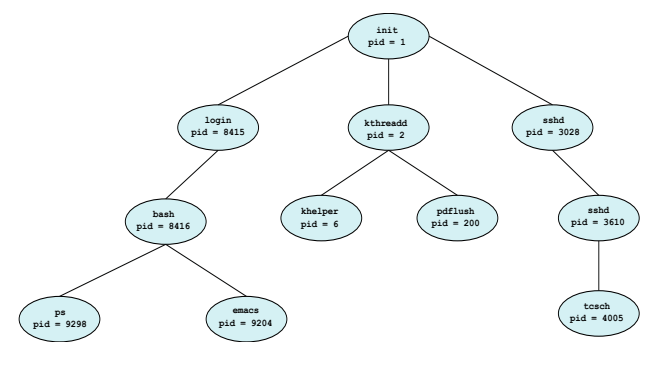
Process Creation/Execution: Windows
-
BOOL CreateProcess (char prog, char args, ...)
-
CreateProcess 명령문은 생성과 프로세스 실행을 동시에 수행하며 LINUX의 exec() 명령어의 매개변수와 동일한 매개변수를 가진다.
-
thread를 만들어 처리함으로써 fork() 단계를 수행할 필요가 없다.
-
새로운 PCB와 Address space를 만들고 초기화한다.
-
prog를 adress space에 load하면 args를 메로리가 할당된 주소로 복사한다.
-
main 함수를 실행하기 위하여 hardware context를 초기화한다.
-
PCB를 Ready queue에 놓는다.
Process termination
- exit() / _exit() / abort( ) / wait() (system call)
- parent가 수행 중이며 wait하지 않을 때 child가 종료되면 zombie가 된다.
- parent가 wait 수행 없이 종료되면 child는 orphan process가 된다.
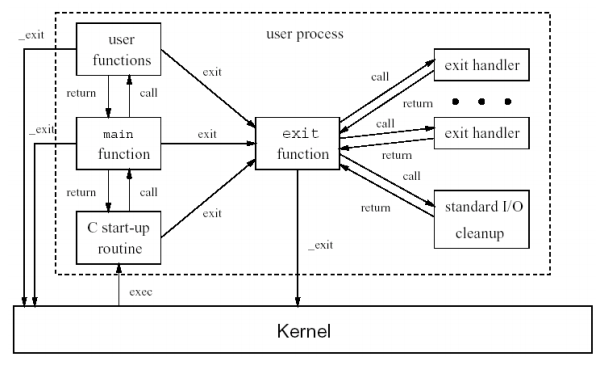
Interprocess communication(IPC, 프로세스 간의 협력을 위한 방법)
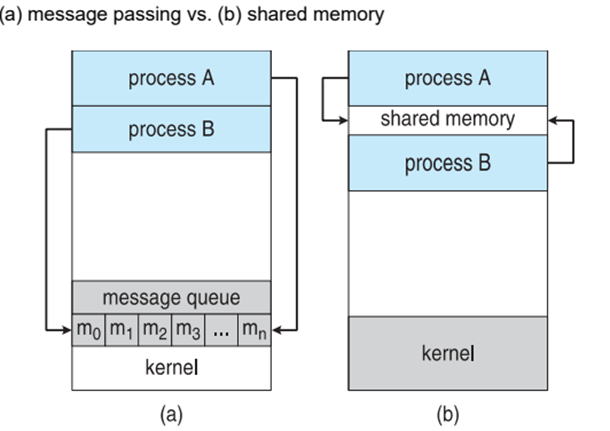
- process간 통신 시 process의 메모리를 직접 사용하면 memory protection에 위배된다.
message passing
- pipes / FIFOs / message queue 방법이 있다
- 운영체제에 message queue를 만들어 읽고 쓰기를 가능하게 한다.
- 장점 : 운영체제가 동기화 작업을 관리해줄 수 있다.
- 단점 : 데이터 복사가 많기 때문에 성능이 좋지 않다.
shared memory
- 프로세스들이 공통적으로 접근할 수 있는 메모리 영역을 kernel(OS)이 만든다.
- 장점 : 데이터 복사가 더 적고, 데이터 읽기/쓰기에 OS가 개입하지 않아 성능이 더 좋다
- 단점 : 프로세스 간의 동기화 작업을 애플리케이션이 직접 해주어야 한다.
Socket
- 하나의 운영체제 안에서 통신하는 위의 방법과는 달리 다른 컴퓨터 프로세스 간의 통신
- 네트워크로 연결된 프로세스 간의 통신 IPC
- Remote Procedure Call(RPC)
- Remote Method Invocation(RMI in Java)
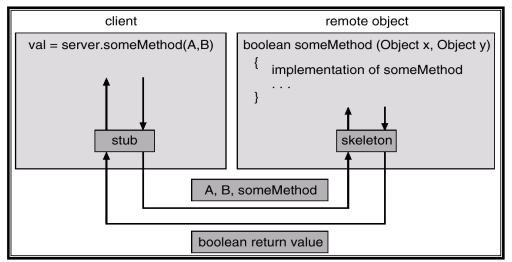