- Payload : 함수의 매개변수같은 느낌, 내가 원하는 정보를 보내줄 수 있음
import { useDispatch, useSelector } from "react-redux/es/exports";
import "./App.css";
import Box from "./component/Box";
function App() {
let count = useSelector((state) => state.count);
const dispatch = useDispatch();
const minus = () => {
if (count !== 0) {
dispatch({ type: "MINUS" });
} else {
count = 0;
}
};
const plus = () => {
dispatch({ type: "PLUS", payload: { num: 5 } });
};
const login = () => {
dispatch({ type: "LOGIN", payload: { id: "siwon", password: "123" } });
};
let initialState = {
count: 0,
id: "",
password: "",
};
function reducer(state = initialState, action) {
switch (action.type) {
case "PLUS":
return { ...state, count: state.count + action.payload.num };
case "MINUS":
return { ...state, count: state.count - 1 };
case "LOGIN":
return {
...state,
id: action.payload.id,
password: action.payload.password,
};
default:
return { ...state };
}
}
export default reducer;
import { useDispatch, useSelector } from "react-redux/es/exports";
import "./App.css";
import Box from "./component/Box";
function App() {
let count = useSelector((state) => state.count);
let id = useSelector((state) => state.id);
let pwd = useSelector((state) => state.password);
const dispatch = useDispatch();
const minus = () => {
if (count !== 0) {
dispatch({ type: "MINUS" });
} else {
count = 0;
}
};
const plus = () => {
dispatch({ type: "PLUS", payload: { num: 5 } });
};
const login = () => {
dispatch({ type: "LOGIN", payload: { id: "siwon", password: "123" } });
};
return (
<div>
<h1>
{id},{pwd}
</h1>
<h1>{count}</h1>
<button onClick={minus}>-1</button>
<button onClick={plus}>+1</button>
<button onClick={login}>Login</button>
<Box />
</div>
);
}
export default App;
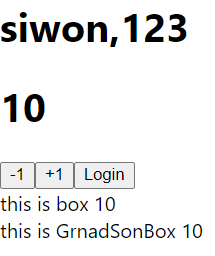