Redux란?
- Javascript의 데이터 상태를 관리해주는 라이브러리이다.
- React에 많이 사용되지만, AngularJS, VueJS, VanillaJS 등 모든 JS환경에서 사용이 가능하다.
- 자세한건 직접 코드로!
만들고자 하는 간단한 Application
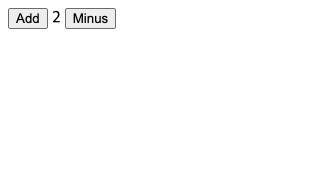
- 숫자 초기값은 0
- Add 버튼을 누르면 숫자가 1 증가한다.
- Minus 버튼을 누르면 숫자가 1 감소한다.
only VanillaJS
const add =document.getElementById("add")
const minus =document.getElementById("minus")
const number = document.getElementById("number")
let count = 0
number.innerText = count;
const updateText = () => {
number.innerText = count
}
const handleAdd=()=> {
count += 1
updateText()
}
const handleMinus=()=> {
count -= 1
updateText()
}
add.addEventListener("click", handleAdd)
minus.addEventListener("click", handleMinus)
- (1) 결과값을 갖고 있는 전역변수
count
를 선언한다.
- (2) 버튼을 클릭했을 때
count
를 적절하게 변경하는 함수를 선언한다.
- (3)
count
의 값이 변경되었을 때, 화면도 함께 변경될 수 있도록 innerText
를 변경한다.
- (4)
eventListener
를 사용하여 (2)(3)에서 이루어지는 기능을 버튼에 추가한다.
VanillaJS with Redux
import { createStore } from 'redux'
const add = document.getElementById("add")
const minus = document.getElementById("minus")
const number = document.getElementById("number")
const ADD = "ADD"
const MINUS = "MINUS"
const countModifier = (count = 0, { type }) => {
switch (type) {
case ADD:
return count + 1
case MINUS:
return count - 1
default:
return count
}
}
const countStore = createStore(countModifier)
const onChange = () => {
number.innerHTML = countStore.getState()
}
countStore.subscribe(onChange)
const handleButton = (type) => {
countStore.dispatch({ type })
}
add.addEventListener("click", () => handleButton(ADD))
minus.addEventListener("click", () => handleButton(MINUS))
- (1) store는 state를 갖는다. redux에서 제공하는 createStore 함수로 store를 생성할 수 있다.
- (2)
modifier
는 store의 state
를 변경(관리)하는 함수이다.
- (3)
createStore
의 파라미터로 countModifier
를 넘겨줌으로써, store의 상태를 해당 countModifier
관리할 수 있게 한다.
- (4)
onChange
함수가 countStore
를 subscribe한다. 따라서 onChange
함수는 countStore
의 상태값이 변경됨을 감지될 경우 실행된다.
- (5) dispatch를 호출할 경우,
createStore
시에 설정했던 countModifier
가 실행된다.
- (6)
eventListener
를 사용하여 클릭 시 적절한 이벤트가 발생할 수 있도록 설정한다.
느낀점
- VanillaJS를 할 때 느낀점은, 코드가 예쁘지 않고 뭔가 중복이 많다는 것이었다.특히 document의 무언가를 가져와서 eventListener를 추가하고, 다시 화면에 적용하는 과정이 귀찮음을 많이 불러일으켰다. (내가 코드 리펙토링을 안한 탓 일수도..)
- 지금은 하지 않았지만, store를 interface로 만들어서
initialize
, subscribe
, dispatch
등의 설정을 빠르게 할 수 있다면, 기존의 VanillaJS보다 편하게 상태관리를 할 수 있을 것 같다.
- 그렇지만 아직은 그렇게 획기적으로 와 ! 리덕스 ! 하는 느낌은 들지 않는다. 그냥 조금 편하구나~ 정도?