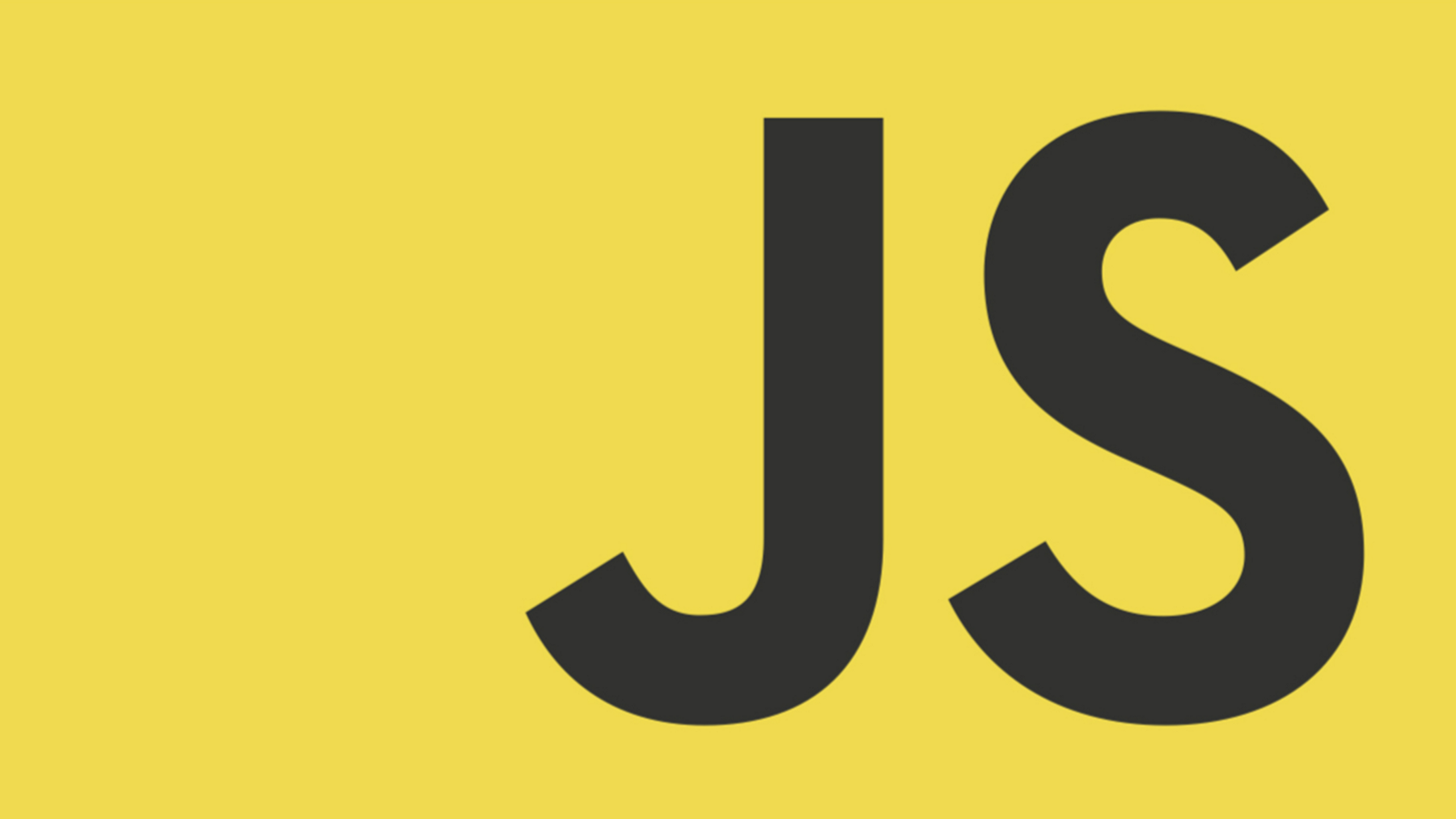
<div id="today"></div>
const ERROR_MESSAGE = {
dataDefind: 'data is not defind',
is_array: 'data type is not array',
type_check: 'type error'
}
const today = [
{
text: 'JS 공부하기',
isComplete: true
}
];
function validate(data) {
if(data === null || undefined) throw new Error(ERROR_MESSAGE.dataDefind);
if(!Array.isArray(data)) throw new Error(ERROR_MESSAGE.is_array);
data.map((item) => {
if(typeof item.text !== 'string' || typeof item.isComplete !== "boolean") throw new Error(ERROR_MESSAGE.type_check)
})
return data;
}
function CreateTodoList(data) {
this.data = validate(data);
this.setState = function(nextData) {
validate(nextData);
this.data = nextData;
this.render();
};
this.render = function(id) {
const todoList = document.querySelector(`#${id}`);
todoList.innerHTML = this.data.map((item) => item.isComplete ? `<strike>${item.text}</strike>`: `${item.text}`).join('');
};
}
const todayList = new CreateTodoList(today);
todayList.render('today');