몰랐던거 정리
✔️ -
단항연산자
System.out.println(-(input));
✔️ %
연산자는 음이 아닌 정수에 대해서만 계산 가능
✔️ 시프트연산자
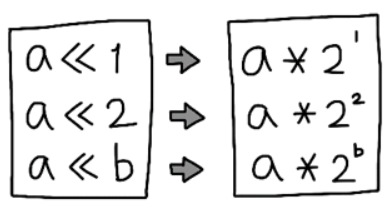
✔️ Convert boolean to int = 삼항연산자
System.out.println((int)q.compare(left,right));
System.out.printf("%d",q.compare(left,right));
int result = q.compare(left,right) ? 1 : 0;
System.out.println(reslut);
✔️ 144번 error : 형변환
long result = input+1;
long result = (long)input+1;
137번
public class Q1037 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int input = sc.nextInt();
sc.close();
System.out.println((char)input);
}
}
138번
public class Q1038 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int first = sc.nextInt();
int second = sc.nextInt();
sc.close();
if ((first+second) >= 2147483647 || (first+second) <= -2147483648) {
System.out.println((long) first + second);
} else {
System.out.println(first + second);
}
}
}
140번
public class Q1040 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int input = sc.nextInt();
sc.close();
System.out.println(-(input));
}
}
141번
public class Q1041 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
char input = (char) br.read();
br.close();
System.out.println((char) (input + 1));
}
}
142번
public class Q1042 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
sc.close();
System.out.println((int)(a / b));
}
}
143번
public class Q1043 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
sc.close();
System.out.println(a % b);
}
}
144번
public class Q1044 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int input = sc.nextInt();
sc.close();
long result = (long)input+1;
System.out.println(result);
}
}
145번
public class Q1045 {
public void plus(int second, int first) {
System.out.println(first + second);
}
public void minus(int first, int second) {
System.out.println(first - second);
}
public void multiple(int first, int second) {
System.out.println(first * second);
}
public void body (int first, int second) {
System.out.println((int)first / second);
}
public void tail (int first, int second) {
System.out.println(first % second);
}
public void division (int first, int second) {
System.out.printf("%.2f\n" , (float)first / second);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int first = sc.nextInt();
int second = sc.nextInt();
sc.close();
Q1045 q = new Q1045();
q.plus(first,second);
q.minus(first,second);
q.multiple(first,second);
q.body(first,second);
q.tail(first,second);
q.division(first,second);
}
}
146번
public class Q1046 {
public int plus(int a,int b,int c) {
return a+b+c;
}
public float average(int a,int b, int c) {
return (float) (a+b+c) / 3;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int first = sc.nextInt();
int second = sc.nextInt();
int third = sc.nextInt();
sc.close();
Q1046 q = new Q1046();
System.out.println(q.plus(first,second,third));
System.out.printf("%.1f",q.average(first,second,third));
}
}
147번
public class Q1047 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int input = sc.nextInt();
sc.close();
System.out.println(input<<1);
}
}
148번
public class Q1048 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int first = sc.nextInt();
int second = sc.nextInt();
sc.close();
System.out.println(first<<second);
}
}
149번
public class Q1049 {
public boolean compare(int left, int right) {
if (left > right) {
return true;
}else { return false; }
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int left = sc.nextInt();
int right = sc.nextInt();
sc.close();
Q1049 q = new Q1049();
int result = q.compare(left,right) ? 1:0;
System.out.println(result);
}
}
150번
public class Q1050 {
public boolean compare(int a, int b) {
if (a == b) {
return true;
} else { return false; }
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int first = sc.nextInt();
int second = sc.nextInt();
sc.close();
Q1050 q = new Q1050();
int result = q.compare(first,second) ? 1:0;
System.out.println(result);
}
}