ActionSheet
ActionSheet(iOS 13.0-14.0)
- iOS 14를 끝으로 더이상 사용되지 않지만, iOS 13과 14를 지원하기 위해서는
ActionSheet
로 구현해야한다.
- 일반적으로 두 개 이상의 선택지가 있고, 사용자가 선택을 하면 ActionSheet는 사라지고 해당 액션을 실행한다.
struct ActionSheetStudy: View {
@State var showActionSheet: Bool = false
var body: some View {
Button("Click Here!") {
showActionSheet.toggle()
}
.actionSheet(isPresented: $showActionSheet) {
ActionSheet(
title: Text("Title"),
message: Text("This is message."),
buttons: [
.default(Text("Button 1")),
.destructive(Text("Button 2")),
.cancel()
])
}
}
}
default
는 기본적인 버튼 스타일로, 주로 확인
과 같은 긍정적인 동작을 수행하는 버튼에 사용된다.
cancel
은 주로 취소
와 같은 부정적인 동작을 수행하는 버튼에 사용된다.
destructive
는 삭제
주의를 불러일으키는 동작을 수행하는 버튼에 사용된다.
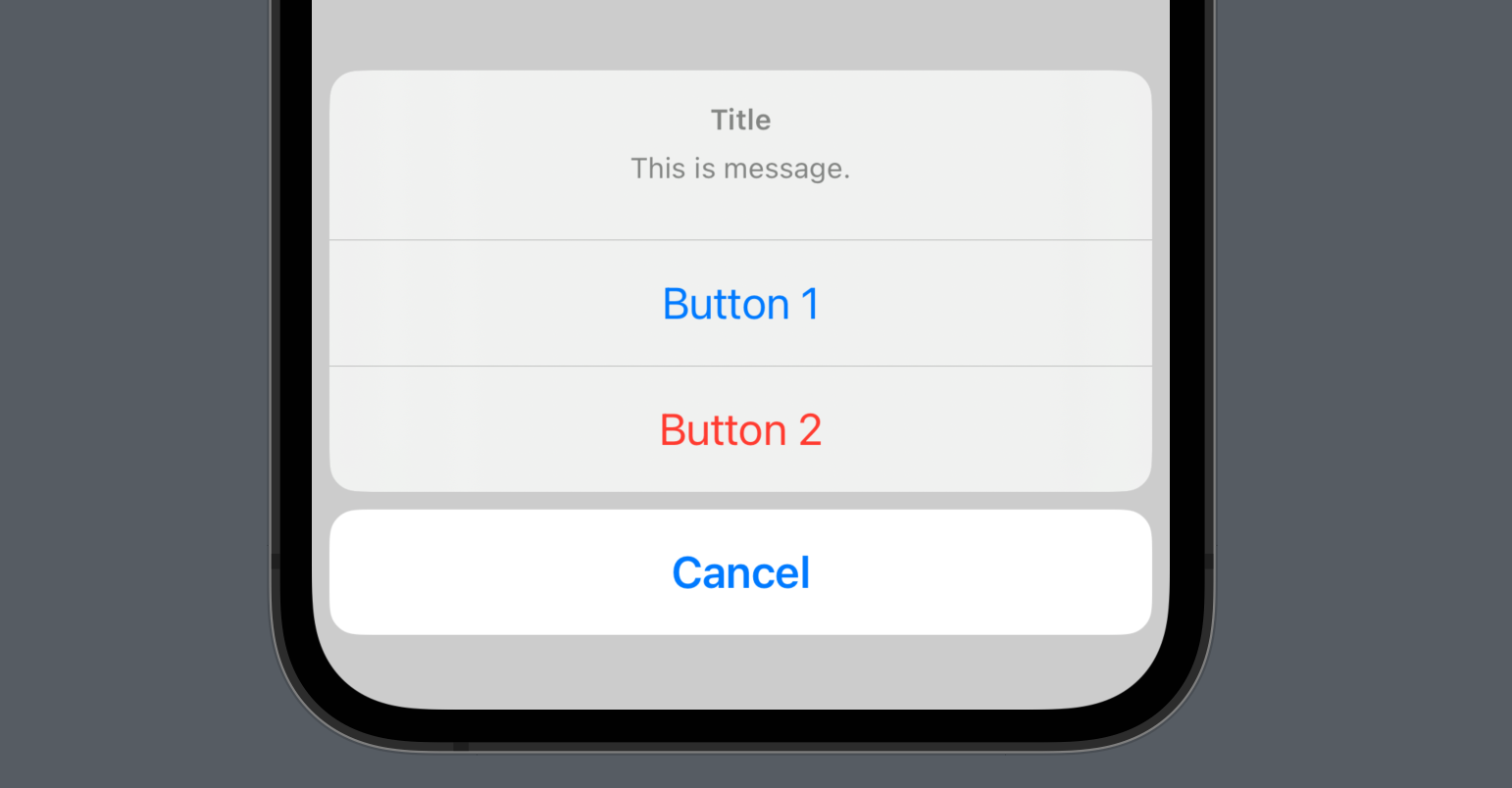
Customizing
- ActionSheet를 함수로 추출하고 enum을 활용하여 각 케이스에 맞는 ActionSheet를 표시할 수 있다.
struct ActionSheetStudy: View {
@State var showActionSheet: Bool = false
@State var actionSheetType: MyActionSheetType = .isOtherPost
enum MyActionSheetType {
case isMyPost
case isOtherPost
}
var body: some View {
VStack(spacing: 40) {
Button("My Post") {
actionSheetType = .isMyPost
showActionSheet.toggle()
}
.actionSheet(isPresented: $showActionSheet, content: getActionSheet)
Button("Other Post") {
actionSheetType = .isOtherPost
showActionSheet.toggle()
}
.actionSheet(isPresented: $showActionSheet, content: getActionSheet)
}
}
func getActionSheet() -> ActionSheet {
let shareButton: ActionSheet.Button = .default(Text("Share"))
let reportButton: ActionSheet.Button = .destructive(Text("Report"))
let deleteButton: ActionSheet.Button = .destructive(Text("Delete"))
switch actionSheetType {
case .isMyPost:
return ActionSheet(
title: Text("My Post"),
buttons: [
shareButton,
deleteButton,
.cancel()
])
case .isOtherPost:
return ActionSheet(
title: Text("Other Post"),
buttons: [
shareButton,
reportButton,
.cancel()
])
}
}
}
ConfirmationDialog(iOS 15.0+)
- iOS 15 이상부터
confirmationDialog
로 actionSheet
가 대체된다.
struct ActionSheetStudy: View {
@State var showActionSheet: Bool = false
var body: some View {
Button("Click Here!") {
showActionSheet.toggle()
}
.confirmationDialog("Title", isPresented: $showActionSheet, titleVisibility: .visible) {
Button("Button 1") {}
Button("Button 2", role: .destructive) {}
Button("Cancel", role: .cancel) {}
} message: {
Text("This is message.")
}
}
}
잘 봤습니다~
ActionSheet를 더이상 사용하지 않는다면 대신에 뭘 사용하게 될까요?