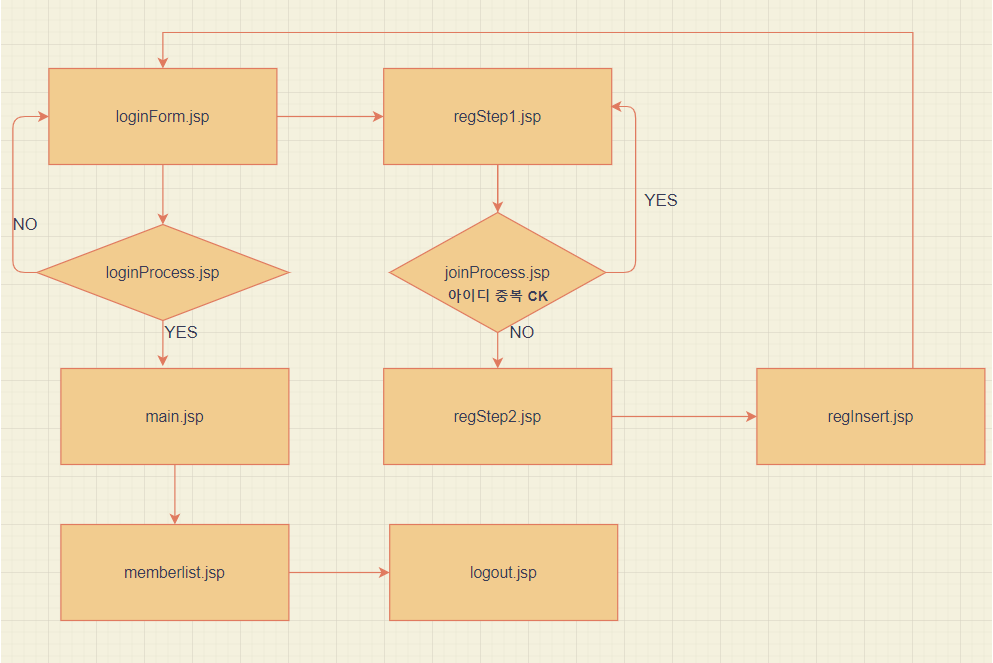
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<style>
fieldset{
margin: 0 auto;
position: relative;
top: 100px;
width: 300px;
height: 300px;
}
#login_box{
width: 230px;
height: 200px;
margin: 0 auto;
}
input{
width: 100%;
height: 50px;
}
.btn_login{
width: 100%;
height: 30px;
}
</style>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form name="loginform" action="loginProcess.jsp" method="post">
<fieldset>
<legend >LOGIN</legend>
<br><br><br>
<div id="login_box">
<input type="text" id="id" name="id" placeholder="ID" >
<input type="password" id="passwd" name="passwd" placeholder="Password" >
<br><br>
<button type="submit" class="btn_login" >로그인</button> <br>
계정이 없으신가요? -><a href="regStep1.jsp">회원가입</a>
</div>
</fieldset>
</form>
</body>
</html>
2.loginProcess.jsp
<%@page import="javax.sql.DataSource"%>
<%@page import="javax.naming.InitialContext"%>
<%@page import="javax.naming.Context"%>
<%@page import="java.sql.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
//로그인폼에서 입력 한 값을 가져온다.
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
String sql ="SELECT * FROM member2 WHERE id=?";
Connection conn = null;
PreparedStatement pstmt =null;
ResultSet rs =null;
try{
//커넥션 풀 생성
Context init = new InitialContext();
DataSource ds =(DataSource)init.lookup("java:comp/env/jdbc/mariaDB");
conn=ds.getConnection();
//sql실행문을 pstmt변수에 담는다.
pstmt = conn.prepareStatement(sql);
//값을 데이터베이스로 보낸다.
pstmt.setString(1,id);
//commit
rs= pstmt.executeQuery();
while(rs.next()){
out.println("성공");
out.println(id);
if(id.equals(rs.getString(1))&&passwd.equals(rs.getString(2))){
out.println(id);
session.setAttribute("id", rs.getString(1));
session.setAttribute("level", rs.getInt(7));
response.sendRedirect("main.jsp");
}
}
response.sendRedirect("loginForm.jsp");
rs.close();
pstmt.close();
conn.close();
} catch (Exception e) {
e.printStackTrace();
} finally{
try{
rs.close();
pstmt.close();
conn.close();
}
catch (Exception e){
e.printStackTrace();
}
}
%>
3.main
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String id = null;
int level = (int)session.getAttribute("level");
if (session.getAttribute("id") != null) {
id = (String) session.getAttribute("id");
} else {
out.println("<scrpit>");
out.println("location.href='loginForm.jsp'");
out.println("</scrpit>");
}
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3><%=id%>로 로그인 하였습니다.
</h3>
<%
//아이디가 admin이거나 level이 5이면
if (id.equals("admin") || level==5) {
%>
<a href="memberList.jsp">관리자 모드 접속(회원목록 보기)</a>
<%
}
%>
<a href="logOut.jsp">로그아웃</a>
</body>
</html>
4.memberlist
<%@page import="javax.sql.DataSource"%>
<%@page import="javax.naming.InitialContext"%>
<%@page import="javax.naming.Context"%>
<%@page import="java.sql.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
Connection conn = null;
PreparedStatement pstmt =null;
ResultSet rs =null;
//커넥션 풀 생성
Context init = new InitialContext();
DataSource ds =(DataSource)init.lookup("java:comp/env/jdbc/mariaDB");
conn=ds.getConnection();
//sql실행문을 pstmt변수에 담는다.
pstmt = conn.prepareStatement("SELECT * FROM member2");
//저장
rs = pstmt.executeQuery();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<style type="text/css">
table {
width: 100%;
}
table, th, td {
border: 1px solid #bcbcbc;
}
</style>
</head>
<body>
<table>
<thead>
<tr>
<th>아이디</th>
<th>비밀번호</th>
<th>이메일</th>
<th>휴대번호</th>
<th>생년월일</th>
<th>등록일자</th>
</tr>
</thead>
<tbody>
<%while(rs.next()){%>
<tr>
<td><%=rs.getString(1) %></td>
<td><%=rs.getString(2) %></td>
<td><%=rs.getString(3) %></td>
<td><%=rs.getString(4) %></td>
<td><%=rs.getString(5) %></td>
<td><%=rs.getString(6) %></td>
</tr>
<%} %>
</tbody>
</table>
<a href="logOut.jsp">로그아웃</a>
</body>
</html>
5.regstep1
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
%>
<!DOCTYPE html>
<html>
<style>
fieldset {
margin: 0 auto;
position: relative;
top: 100px;
width: 300px;
padding: 20px;
}
input {
width: 100%;
height: 30px;
}
input[type="button"] {
width: 80px;
border: 0;
background-color: none;
color: white;
cursor: pointer;
margin: 5px;
}
input[type="reset"] {
width: 80px;
border: 0;
background-color: #2b6699;
color: white;
cursor: pointer;
float: left;
margin: 5px;
}
input[type="submit"] {
width: 80px;
border: 0;
background-color: #2b6699;
color: white;
cursor: pointer;
margin: 5px;
}
p {
font-size: small;
}
</style>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form name="joinForm" action="regStep2.jsp" method="post"
onsubmit="return formCheck1()">
<fieldset>
<h3>회원가입</h3>
<label for="id">아이디</label> <input type="button" id="id_button" value="중복확인" onclick="idCheck()">
<input type="text" id="id" name="id" placeholder="ID" required="required">
<input type="hidden" id="hidden_id" value="0">
<p id="ckeckID">중복확인</p>
<label for="passwd">패스워드</label>
<input type="password" name="passwd" id="passwd" placeholder="4자리 이상 입력"><br>
<label for="passwd2">패스워드</label>
<input type="password" name="passck" id="passck">
<p id="cheskPass"></p>
<input type="reset" value="다시입력">
<input type="submit"value="다음단계 ">
</fieldset>
</form>
<script src="regist.js"></script>
</body>
</html>
regstep2
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
%>
<!DOCTYPE html>
<html>
<style>
fieldset {
margin: 0 auto;
position: relative;
top: 100px;
width: 300px;
padding: 20px;
}
input {
width: 100%;
height: 30px;
}
input[type="reset"] {
width: 80px;
border: 0;
background-color: #2b6699;
color: white;
cursor: pointer;
float: left;
margin: 5px;
}
input[type="button"] {
width: 80px;
border: 0;
background-color: #2b6699;
color: white;
cursor: pointer;
float: left;
margin: 5px;
}
input[type="submit"] {
width: 80px;
border: 0;
background-color: #2b6699;
color: white;
cursor: pointer;
margin: 5px;
}
</style>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form name="joinForm" action="regInsert.jsp" method="post"
onsubmit="return formCheck2()">
<input type="hidden" name="id" value="<%=id%>"> <input
type="hidden" name="passwd" value="<%=passwd%>">
<fieldset>
<h3>회원가입</h3>
<h5>
아이디:<%=id%>님
</h5>
<label for="email">이메일</label>
<input type="email" name="email"id="email" placeholder="e-mail@naver.com"> <br>
<label for="tel">휴대번호</label>
<input type="tel" name="phone" id="phone" placeholder="010-1234-4567"> <br>
<label for="birth">생년월일</label>
<input type="date" name="birth" id="birth" placeholder="yyyy.mm.dd">
<br> <br>
<input type="reset" value="다시입력">
<input type="button" value="이전단계" onclick="location.href='regStep1.jsp'">
<input type="submit" value=" 완료 ">
</fieldset>
</form>
<script src="regist.js"></script>
</body>
</html>
joinprocess
<%@page import="java.sql.Connection"%>
<%@ page import="java.sql.*"%>
<%@ page import="javax.sql.*"%>
<%@ page import="javax.naming.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<style>
fieldset {
margin: 0 auto;
position: relative;
top: 100px;
width: 300px;
padding: 20px;
}
</style>
<meta charset="UTF-8">
</head>
<%
//regStep1에서 입력한 아이디를 가져온다.
String id = request.getParameter("id");
String a;
String sql = "SELECT * FROM member2 WHERE id=?";
String resultText = "";
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
//커넥션 풀 생성
Context init = new InitialContext();
DataSource ds = (DataSource) init.lookup("java:comp/env/jdbc/mariaDB");
conn = ds.getConnection();
//sql실행문을 pstmt변수에 담는다.
pstmt = conn.prepareStatement(sql);
//값을 데이터베이스로 보낸다.
pstmt.setString(1, id);
//commit
rs = pstmt.executeQuery();
%>
<body>
<fieldset>
<form action="regStep1.jsp" method="post" name="wfr" onsubmit="return result()">
ID : <input type="text" name="id" value="<%=id%>">
<%
if (!rs.next()) {
resultText = "사용가능한 아이디입니다.";
%>
<input type="hidden" id="conf" value="1">
<%
} else {
resultText = "사용 불가능한 아이디입니다";
%>
<input type="hidden" id="conf" value="0">
<%
}
%>
<input type="submit" value="확인">
</form>
<h3><%=resultText%></h3>
</fieldset>
<script src="regist.js"></script>
</body>
</html>
reginsert
<%@page import="java.sql.Connection"%>
<%@ page import="java.sql.*"%>
<%@ page import="javax.sql.*"%>
<%@ page import="javax.naming.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
request.setCharacterEncoding("UTF-8");
//값 받아온다.
String id = request.getParameter("id");
String passwd = request.getParameter("passwd");
String email = request.getParameter("email");
String phone = request.getParameter("phone");
String birth = request.getParameter("birth");
int level = 1;
//객체 인스턴스 변수 생성 초기화
Connection conn = null;
PreparedStatement pstmt = null;
try {
//커넥션풀 객체 생성
Context init = new InitialContext();
DataSource ds = (DataSource) init.lookup("java:comp/env/jdbc/mariaDB");
conn = ds.getConnection();
//sql실행문을 pstmt변수에 담는다.
pstmt = conn.prepareStatement("INSERT INTO member2 VALUES(?,?,?,?,?,now(),?)");
//받은 값을 데이터베이스로 보내준다(물음표의 값 넣어준다.)
pstmt.setString(1, id);
pstmt.setString(2, passwd);
pstmt.setString(3, email);
pstmt.setString(4, phone);
pstmt.setString(5, birth);
pstmt.setInt(6, level);
// commit,실행
pstmt.executeQuery();
} catch (Exception e) {
out.println("오류 발생!!!!");
e.printStackTrace();
} finally {
try {
pstmt.close();
conn.close();
} catch (Exception e) {
out.println("오류 발생@@@");
e.printStackTrace();
}
}
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<div>
<table>
<tr>
<td colspan=2 class="td_title">회원 등록 완료</td>
</tr>
<tr>
<td>아이디 :</td>
<td><%=id%></td>
</tr>
<tr>
<td>비밀번호 :</td>
<td><%=passwd%></td>
</tr>
<tr>
<td>이메일</td>
<td><%=email%></td>
</tr>
<tr>
<td>휴대번호</td>
<td><%=phone%></td>
</tr>
<tr>
<td>생년월일</td>
<td><%=birth%></td>
</tr>
</table>
<a href="loginForm.jsp">로그인</a>
</div>
</body>
</html>
js
//아이디 중복체크 js
function idCheck() {
var id = joinForm.id.value;
if (id == "") {
alert("아이디를 먼저 입력해 주세요.");
} else {
url = "joinProcess.jsp?id=" + id;
window.open(url, "post", "width=350, height=220");
}
}
function result() {
let a = document.querySelector('#conf');
//joinProcess.jsp #conf value==1 ,사용가능한 아이디이면
if (a.value == "1") {
console.log("체크")
opener.document.joinForm.id.value = document.wfr.id.value;
opener.document.joinForm.id.readOnly = true;
opener.document.querySelector('#ckeckID').innerText = "아이디를 사용할수있습니다.";
//joinform에 input type= "hidden" id="hidden_id" 의 value를 1 로 변경(value=0기본값->false반환 )
opener.document.querySelector('#hidden_id').value = "1";
window.close();
} else {
opener.document.joinForm.id.readOnly = false;
opener.document.querySelector('#ckeckID').innerText = "아이디를 사용할수없습니다.";
self.close();
}
}
//회원가입폼 js
function formCheck1() {
if (joinForm.id.value == "") {
alert("아이디를 먼저 입력해 주세요.");
return false;
}
if (joinForm.hidden_id.value == "0") {
alert("중복체크를 진행해주세요");
joinForm.id_button.focus();
return false;
}
if (joinForm.passwd.value == "") {
alert("비밀번호를 입력하세요");
joinForm.passwd.focus();
return false;
}
if (joinForm.passck.value == "") {
alert("비밀번호 확인을 입력하세요");
joinForm.passck.focus();
return false;
}
if (joinForm.passwd.value != joinForm.passck.value) {
alert("비밀번호가 같지 않습니다.");
return false;
}
}
function formCheck2() {
if (joinForm.email.value == "") {
alert("이메일을 입력하세요");
joinForm.email.focus();
return false;
}
if (joinForm.phone.value == "") {
alert("핸드폰 번호를 입력하세요");
joinForm.phone.focus();
return false;
}
if (joinForm.birth.value == "") {
alert("생년월일을 입력하세요");
joinForm.birth.focus();
return false;
}
}
logout
<%
if((session.getAttribute("id") != null)){
session.removeAttribute("id");
session.removeAttribute("level");
response.sendRedirect("loginForm.jsp");
return;
}
%>