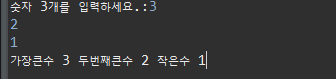
메서드 호출 x
1. if -else사용
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner in = new Scanner(System.in);
System.out.print("숫자 3개를 입력하세요.:");
int x1 = in.nextInt();
int y1 = in.nextInt();
int z1 = in.nextInt();
int result1, result2, result3;
if (x1 > y1 && y1 > z1) {
result1 = x1;
} else if (y1 > x1 && y1 > z1 && (y1 > z1 || z1 > y1)) {
result1 = y1;
} else {
result1 = z1;
}
if ((x1 < y1 && x1 > z1) || (x1 > y1 && x1 < z1)) {
result2 = x1;
} else if ((y1 < x1 && y1 > z1) || (y1 > x1 && y1 < z1)) {
result2 = y1;
} else {
result2 = z1;
}
if (x1 < y1 && x1 < z1 && (y1 < z1 || z1 < y1)) {
result3 = x1;
} else if (y1 < x1 && y1 < z1 && (x1 < z1 || z1 < x1)) {
result3 = y1;
} else {
result3 = z1;
}
System.out.printf("가장큰수=%d 두번째큰수=%d 작은수=%d ", result1, result2, result3);
}
2.삼항연산자 사용
Scanner in = new Scanner(System.in);
System.out.print("숫자 3개를 입력하세요.:");
int a = in.nextInt();
int b = in.nextInt();
int c = in.nextInt();
//
int max1 = (a > b) ? a : b;
int max2 = (max1 > c) ? max1 : c;
int min1 = (a < b) ? a : b;
int min2 = (min1 < c) ? min1 : c;
int middle1 = (a > b) ? b : a;
int middle2 = (middle1 > c) ? middle1 : c;
System.out.printf("가장큰수 %d 두번째큰수 %d 작은수 %d", max2, middle2, min2);
3.메서드 오버로딩 -1
if문
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("숫자 3개를 입력하세요.:");
int x = in.nextInt();
int y = in.nextInt();
int z = in.nextInt();
System.out.printf("가장큰수=%d ", max1(x, y, z));
System.out.printf("두번째큰수=%d ", max2(x, y, z));
System.out.printf("작은수=%d ", max3(x, y, z));
}
public static int max1(int x1, int y1, int z1) {
// TODO Auto-generated method stub
int result;
if (x1 > y1 && x1 > y1 && (y1 > z1 || z1 > y1)) {
result = x1;
} else if (y1 > x1 && y1 > z1) {
result = y1;
} else {
result = z1;
}
return result;
}
public static int max2(int x1, int y1, int z1) {
// TODO Auto-generated method stub
int result;
if ((x1 < y1 && x1 > z1) || (x1 > y1 && x1 < z1)) {
result = x1;
} else if ((y1 < x1 && y1 > z1) || (y1 > x1 && y1 < z1)) {
result = y1;
} else {
result = z1;
}
return result;
}
public static int max3(int x1, int y1, int z1) {
// TODO Auto-generated method stub
int result;
if (x1 < y1 && x1 < z1 && (y1 < z1 || z1 < y1)) {
result = x1;
} else if (y1 < x1 && y1 < z1 && (x1 < z1 || z1 < x1)) {
result = y1;
} else {
result = z1;
}
return result;
}
}
4.오버로딩 메서드-2
if-else(2)
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("숫자 3개를 입력하세요.:");
int x = in.nextInt();
int y = in.nextInt();
int z = in.nextInt();
int max = 0, mid = 0, min = 0;
max = max1(x, y, z);
if (max == x) {
mid = max1(y, z);
if (mid == y) {
min = z;
} else if (mid == z) {
min = y;
}
}
if (max == y) {
mid = max1(x, z);
if (mid == x) {
min = z;
} else if (mid == z) {
min = x;
}
}
if (max == z) {
mid = max1(x, y);
if (mid == x) {
min = y;
} else if (mid == z) {
min = y;
}
}
}
public static int max1(int a, int b) {
int result = a > b ? a : b;
return result;
}
public static int max1(int a, int b, int c) {
return max1(max1(a, b), c);
}
5.오버로딩-3
삼항연산자
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("숫자 3개를 입력하세요.:");
int x = in.nextInt();
int y = in.nextInt();
int z = in.nextInt();
System.out.printf("가장큰수 %d 두번째큰수 %d 작은수 %d", max(x, y, z), mid(x, y, z), min(x, y, z));
}
private static Object max(int x, int y, int z) {
int max1 = (x > y) ? x : y;
int max2 = (max1 > z) ? max1 : z;
return max2;
}
private static Object mid(int x, int y, int z) {
int mid1 = (x > y) ? y : x;
int mid2 = (mid1 > z) ? mid1 : z;
return mid2;
}
private static Object min(int x, int y, int z) {
int min1 = (x < y) ? x : y;
int min2 = (min1 < z) ? min1 : z;
return min2;
}
}