문제
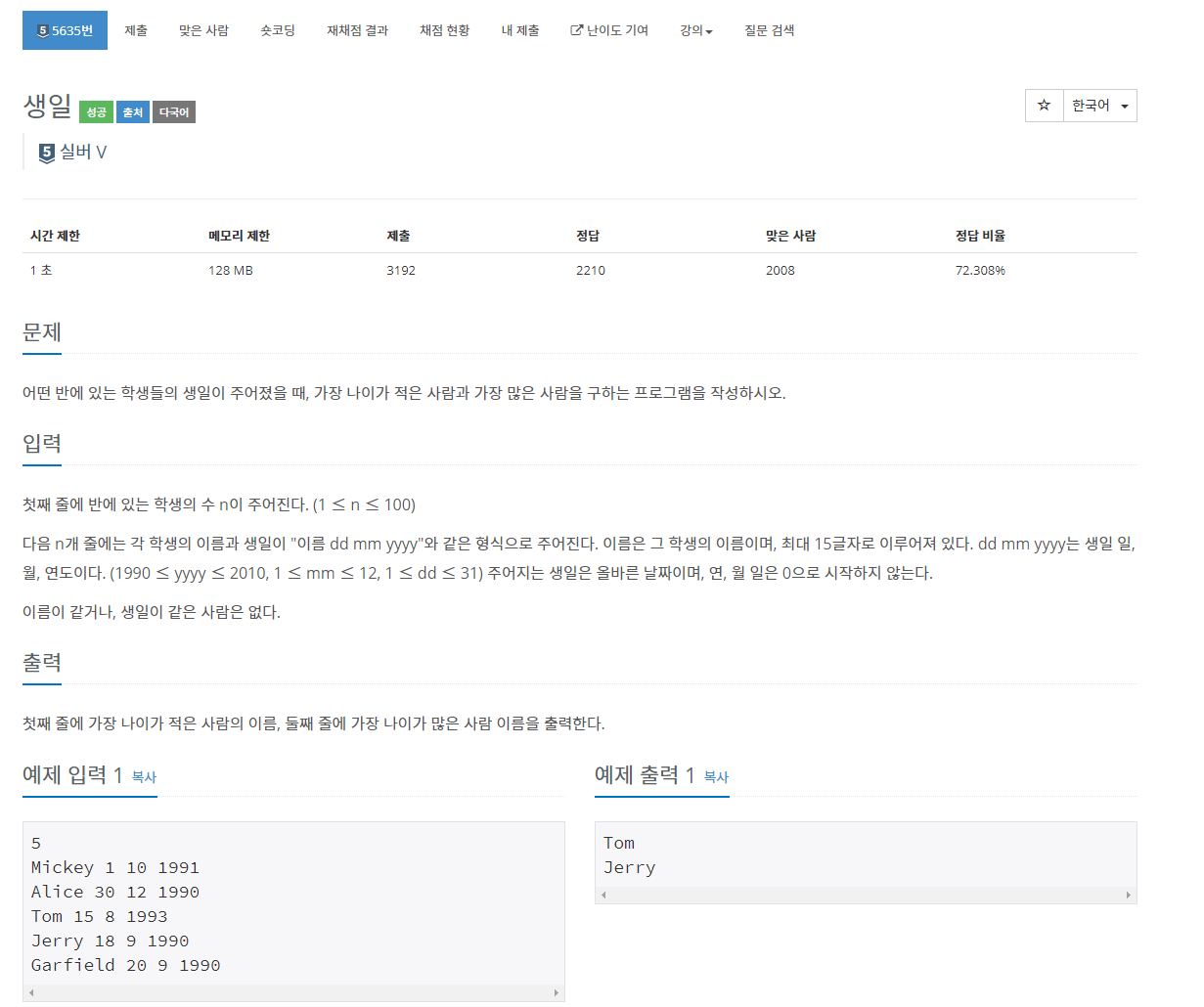
5635번: 생일
접근
- 이름, 생일(년, 월, 일)이 주어지고 이를 바탕으로 가장 나이가 적은 사람, 많은 사람의 이름을 출력한다.
- 말 그대로 정렬이다.
- 입력 정보가 많으니 편한 관리를 위해 클래스를 만들어 본다.
- sort를 하는 방법이 제일 중요하지 않을까?
내 코드
import java.io.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.StringTokenizer;
public class Main {
static BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
static ArrayList<Student> list = new ArrayList<>();
public static void main(String[] args) throws IOException {
int n = Integer.parseInt(br.readLine());
StringTokenizer st;
for (int i = 0; i < n; i++) {
st = new StringTokenizer(br.readLine()," ");
list.add(new Student(st.nextToken(), Integer.parseInt(st.nextToken()), Integer.parseInt(st.nextToken()), Integer.parseInt(st.nextToken())));
}
Collections.sort(list);
System.out.println(list.get(0).getName());
System.out.println(list.get(n-1).getName());
}
static class Student implements Comparable<Student> {
private String name;
private int day;
private int month;
private int year;
public Student(String name, int day, int month, int year) {
this.name = name;
this.day = day;
this.month = month;
this.year = year;
}
@Override
public int compareTo(Student o) {
if (this.getYear() < o.getYear())
return 1;
else if (this.getYear() > o.getYear()) {
return -1;
} else if (this.getMonth() < o.getMonth()) {
return 1;
} else if (this.getMonth() > o.getMonth()) {
return -1;
} else if (this.getDay() < o.getDay()) {
return 1;
}
return -1;
}
public String getName() {
return name;
}
public int getDay() {
return day;
}
public int getMonth() {
return month;
}
public int getYear() {
return year;
}
}
}
- 입력 값이 많아 student 객체를 만들고 이를
ArrayList
에 삽입하여 정렬한다.
- 간단한 문제이지만 굳이 이 문제를 따로 정리하는 이유는, 나중에 좀 더 복잡함을 요구하는 문제들을 보면 객체를 따로두고 응용하는 경우가 종종 있어서 이게 기본기라 생각하고 정리했다.
- 생성자와
Getter
패턴도 개발하다보면 자주 쓰이니까...
- 마찬가지로, 난이도 있는 문제에선
Comparable
인터페이스를 활용해 Override해서 정렬하는 패턴도 자주 등장한다.