class Solution {
class Point{
Point(int r, int c, int d){
row = r;
col = c;
dist = d;
}
int row, col, dist;
}
int [][] D = {{-1,0}, {1,0}, {0,-1}, {0,1}};
boolean bfs(String[] place, int row, int col){
boolean[][]visited = new boolean[5][5];
Queue<Point> q = new LinkedList<>();
visited[row][col] = true;
q.add(new Point(row, col, 0));
while(!q.isEmpty()){
Point curr = q.remove();
if(curr.dist > 2) continue;
if(curr.dist != 0 && place[curr.row].charAt(curr.col) == 'P') return false;
for(int i=0; i<4; i++){
int curr_row = curr.row + D[i][0], curr_col = curr.col + D[i][1];
if(curr_row <0 || curr_row>4 || curr_col < 0|| curr_col>4) continue;
if(visited[curr_row][curr_col]) continue;
if(place[curr_row].charAt(curr_col) == 'X') continue;
visited[curr_row][curr_col] = true;
q.add(new Point(curr_row, curr_col, curr.dist + 1));
}
}
return true;
}
boolean check(String[] place){
for(int r=0; r<5; r++){
for(int c =0; c<5; c++){
if(place[r].charAt(c) == 'P'){
if(bfs(place,r ,c) ==false) return false;
}
}
}
return true;
}
public int[] solution(String[][] places){
int[] answer = new int[5];
for(int i =0; i<5; ++i){
if(check(places[i])) answer[i] = 1;
else answer[i] = 0;
}
return answer;
}
}
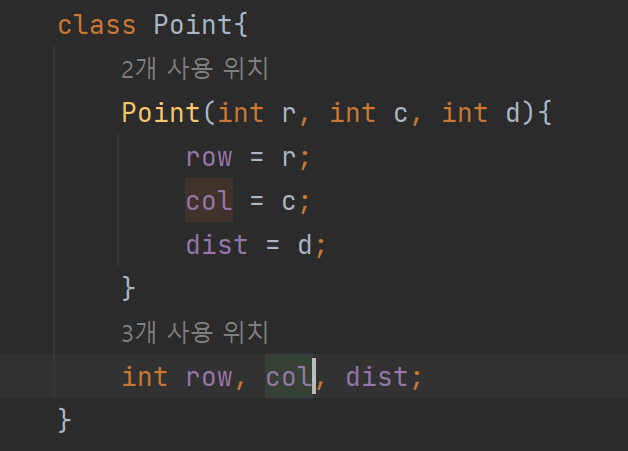
- Point를 선언하여 row, col, dist를 받게한다
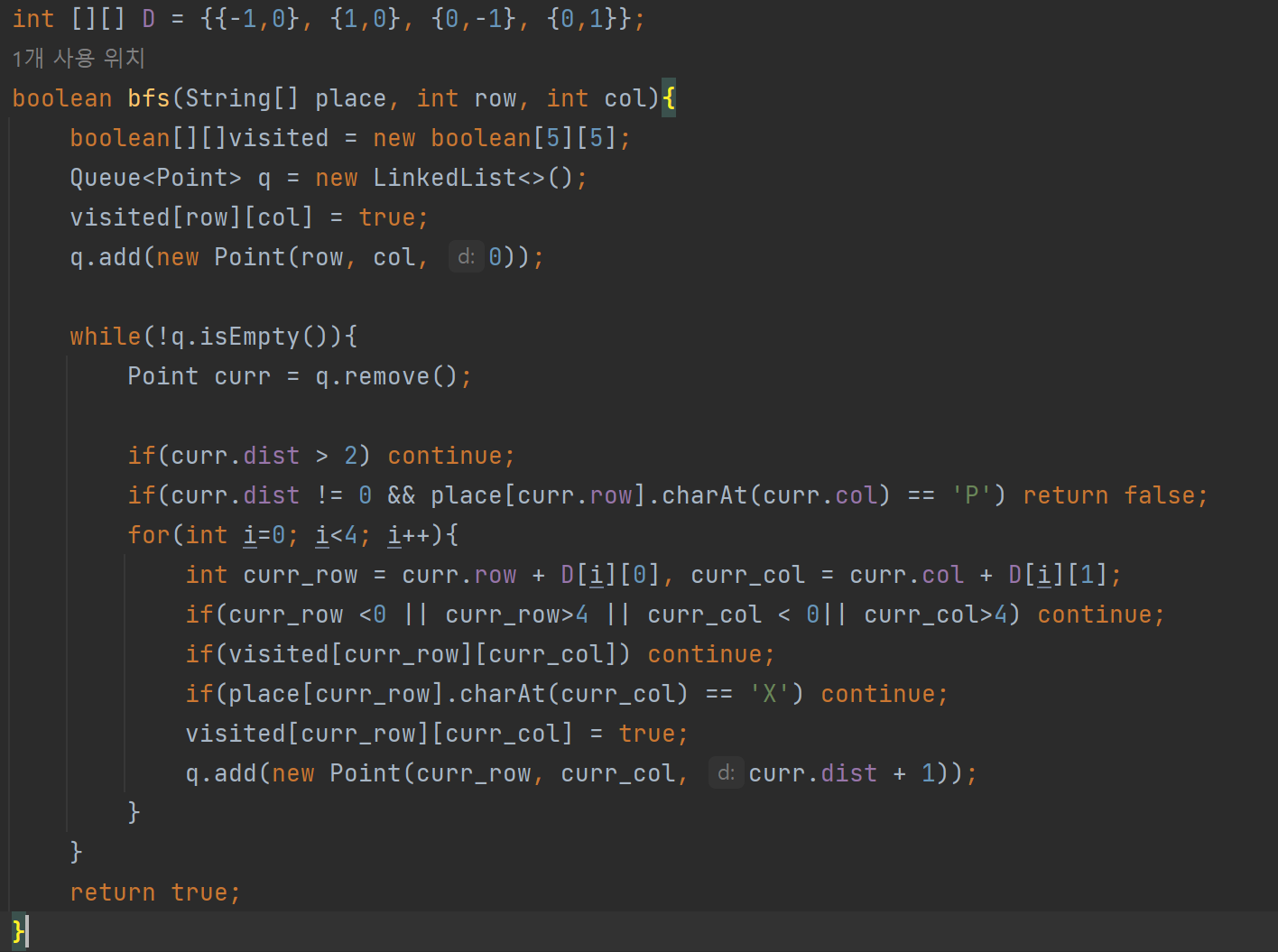
- 배열 D를 통해 상, 하, 좌, 우를 만든다
- bfs함수로
class Solution {
static int[] dx = {-1, 0, 1, 0};
static int[] dy = {0, 1, 0, -1};
static boolean[][] visit;
static int[] answer;
public void dfs(int num, int x, int y, int count, String[] places){
if (count > 2) return;
if (count > 0 && count <= 2 && places[x].charAt(y) == 'P'){
//2칸 범위내에 다른 응시자가 있을 경우 거리두기 미준수로 0처리
answer[num] = 0;
return;
}
for (int i = 0; i < 4; i++) {
int nx = x + dx[i];
int ny = y + dy[i];
//배열 범위 밖으로 초과하는지 여부 검사, 파티션으로 분리되어 있는 경우 상관 없음.
if (nx >= 0 && nx < 5 && ny >= 0 && ny < 5 && places[nx].charAt(ny) != 'X') {
if (visit[nx][ny]) continue; //이미 방문한 곳일 경우 생략
visit[nx][ny] = true;
dfs(num, nx, ny, count + 1, places);
visit[nx][ny] = false;
}
}
}
public int[] solution(String[][] places) {
answer = new int[places.length];
for (int i = 0; i < places.length; i++) {
answer[i] = 1;
}
for (int i = 0; i < places.length; i++) {
visit = new boolean[5][5];
for (int j = 0; j < 5; j++) {
for (int k = 0; k < 5; k++) {
if (places[i][j].charAt(k) == 'P'){
visit[j][k] = true;
dfs(i, j, k, 0, places[i]);
visit[j][k] = false;
}
}
}
}
return answer;
}
}