게시판 List
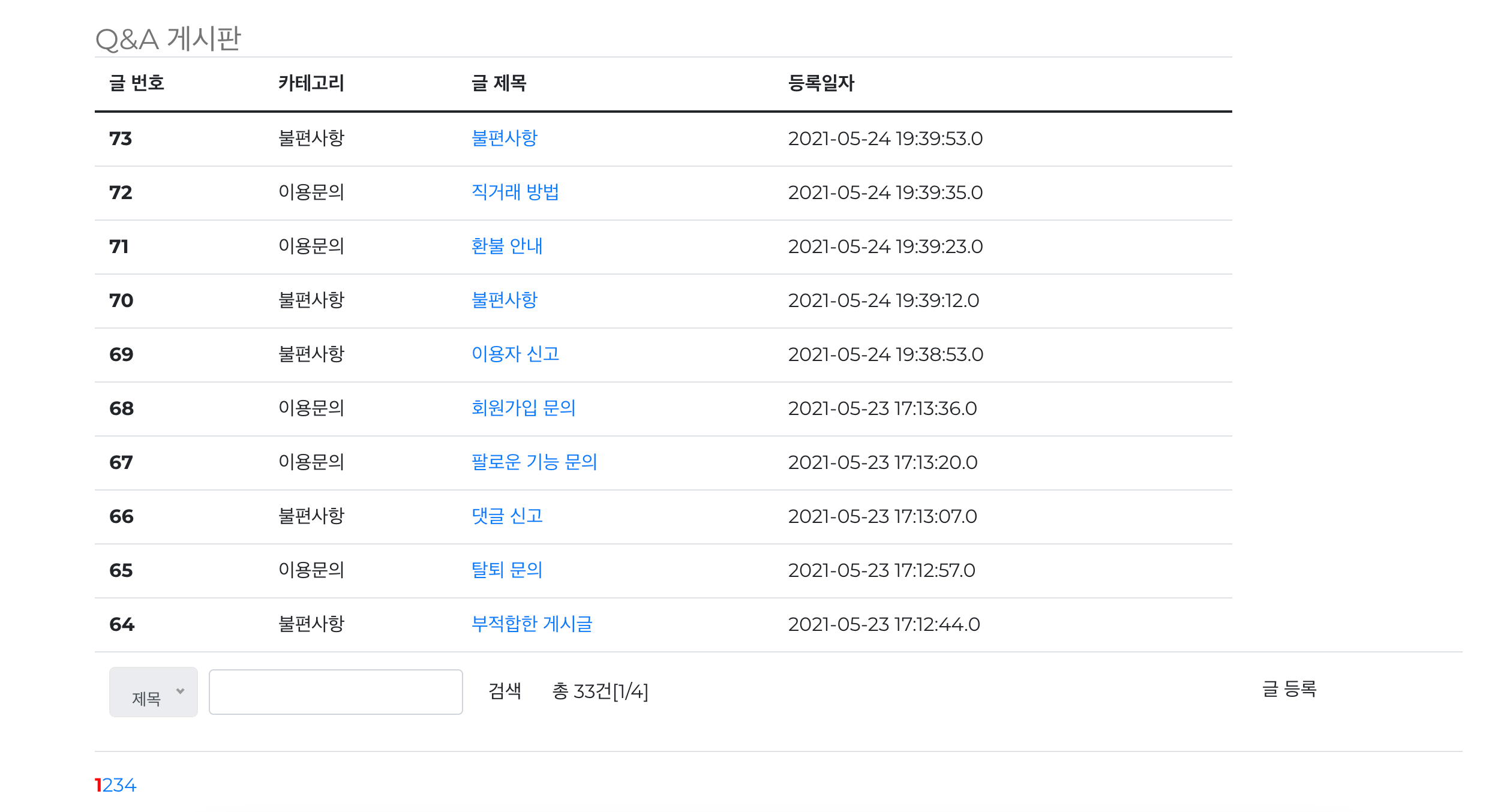
- qnaList.jsp
<head>
<script>
function search(){
var mode = $('#mode').val() ;
var keyword = $('#keyword').val() ;
location.href='<%=FormNo%>qnaList' + '&mode=' + mode + '&keyword=' + keyword ;
}
function searchAll(){
location.href='<%=FormNo%>qnaList';
}
function writeForm(){
location.href='<%=FormNo%>qnaInsert';
}
</script>
<meta charset="UTF-8">
<link rel="stylesheet" href="css/bootstrap.min2.css" type="text/css">
</head>
<body>
<div class="container col-sm-offset-<%=offset%> col-sm-<%=mywidth%>">
<div class="panel panel-primary">
<div class="panel-heading">
<h4>Q&A 게시판</h4>
</div>
<table class="table table-hover">
<thead>
<tr>
<th scope="col">글 번호</th>
<th scope="col">카테고리</th>
<th scope="col" colspan="2">글 제목</th>
<th scope="col">등록일자</th>
</tr>
</thead>
<tbody>
<c:forEach var="bean" items="${requestScope.lists}">
<tr>
<th scope="row">${bean.no}</th>
<td>${bean.category}</td>
<td colspan="2"><a href="<%=FormNo%>qnaDetailView&no=${bean.no}&user_no=${sessionScope.loginfo.no}">${bean.title}</a></td>
<td>${bean.date}</td>
</tr>
</c:forEach>
<tr>
<td align="center" colspan="5">
<form action="" class="form-inline" role="form" name="myform" method="get">
<div class="form-group">
<select id="mode" name="mode" class="form-control">
<option value="qna_title" selected="selected">제목
<option value="qna_content">내용
</select>
</div>
<div class="form-group">
<input type="text" class="form-control" name="keyword" id="keyword">
</div>
<button class="btn btn-default" type="button" onclick="search();">검색</button>
${pageInfo.pagingStatus}
</form>
</td>
<td>
<c:if test="${whologin != 0}">
<button class="btn btn-default" type="button" onclick="writeForm();">
글 등록
</button>
</c:if>
</td>
</tr>
</tbody>
</table>
<div align="center">
<footer>${pageInfo.pagingHtml}</footer>
</div>
</div>
</div>
<br><br><br><br>
<script type="text/javascript">
$('#mode option').each(function(){
if($(this).val() == '${pageInfo.mode}'){
$(this).attr('selected', 'selected');
}
});
$('#keyword').val('${pageInfo.keyword}');
</script>
<br>
</body>
</html>
- QnAListController.java
package com.veranda.qna.controller;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.veranda.common.controller.SuperClass;
import com.veranda.common.utility.FlowParameters;
import com.veranda.common.utility.Paging;
import com.veranda.qna.dao.QnADao;
import com.veranda.qna.vo.QnA;
public class QnAListController extends SuperClass{
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
super.doGet(request, response);
FlowParameters parameters
= new FlowParameters(
request.getParameter("pageNumber"),
request.getParameter("mode"),
request.getParameter("keyword"));
System.out.println("parameter list : ");
System.out.println(parameters.toString());
String contextPath = request.getContextPath();
String url = contextPath + "/veranda?command=qnaList";
QnADao dao = new QnADao();
int totalCount = dao.SelectTotalCount(parameters.getMode(), parameters.getKeyword());
System.out.println("total data size : " + totalCount);
Paging pageInfo = new Paging(
parameters.getPageNumber(),
totalCount,
url,
parameters.getMode(),
parameters.getKeyword());
List<QnA> lists = dao.SelectDataList(
pageInfo.getBeginRow(),
pageInfo.getEndRow(),
parameters.getMode(),
parameters.getKeyword());
System.out.println("qna list count : " + lists.size());
request.setAttribute("lists", lists);
request.setAttribute("pageInfo", pageInfo);
request.setAttribute("parameters", parameters.toString());
String gotopage = "/qna/qnaList.jsp";
super.GotoPage(gotopage);
}
}
- QnADao.java
public class QnADao extends SuperDao {
public int SelectTotalCount(String mode, String keyword) {
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = " select count(*) as cnt from qnas";
if (mode.equalsIgnoreCase("all") == false) {
System.out.println("not all search mode");
sql += " where " + mode + " like '%" + keyword + "%' ";
}
int cnt = -1;
try {
if (this.conn == null) {
this.conn = this.getConnection();
}
pstmt = this.conn.prepareStatement(sql);
rs = pstmt.executeQuery();
if (rs.next()) {
cnt = rs.getInt("cnt");
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) { rs.close(); }
if (pstmt != null) { pstmt.close(); }
this.closeConnection();
} catch (Exception e2) {
e2.printStackTrace();
}
}
return cnt;
}
public List<QnA> SelectDataList(int beginRow, int endRow, String mode, String keyword) {
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = "select ranking, user_no, qna_no, qna_title, qna_content, qna_category, qna_date ";
sql += " from (select user_no, qna_no, qna_title, qna_content, qna_category, qna_date, ";
sql += " rank() over(order by qna_no desc) as ranking ";
sql += " from qnas ";
if (mode.equalsIgnoreCase("all") == false) {
System.out.println("not all search mode");
sql += " where " + mode + " like '%" + keyword + "%' ";
}
sql += " ) where ranking between ? and ? ";
List<QnA> lists = new ArrayList<QnA>();
try {
if (this.conn == null) {
this.conn = this.getConnection();
}
pstmt = this.conn.prepareStatement(sql);
pstmt.setInt(1, beginRow);
pstmt.setInt(2, endRow);
rs = pstmt.executeQuery();
while (rs.next()) {
QnA bean = new QnA();
bean.setUser_no(rs.getInt("user_no"));
bean.setNo(rs.getInt("qna_no"));
bean.setTitle(rs.getString("qna_title"));
bean.setContent(rs.getString("qna_content"));
bean.setCategory(rs.getString("qna_category"));
bean.setDate(rs.getString("qna_date"));
lists.add(bean);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
this.closeConnection();
} catch (Exception e2) {
e2.printStackTrace();
}
}
return lists;
}
}
게시판 DetailView
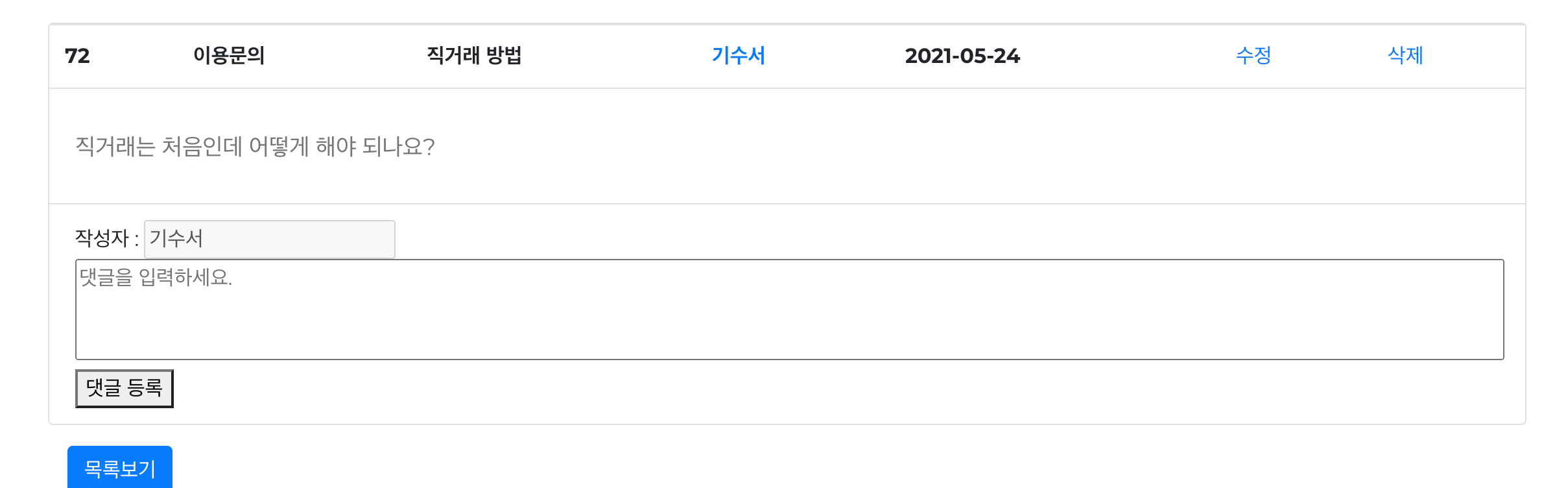
- qnaDetailView.jsp
<%
/* position for grid system */
int offset = 2;
int mywidth = twelve - 2 * offset;
int formleft = 3;
int formright = twelve - formleft;
int rightButton = 2;
%>
<html>
<head>
<style type="text/css">
.checkout__input_2 input{
height: 350px;
width: 100%;
border: 1px solid #e1e1e1;
font-size: 14px;
color: #666666;
padding-left: 20px;
margin-bottom: 20px;
}
.upImg{
height: 350px;
width: 350px;
}
</style>
<meta charset="UTF-8">
<link rel="stylesheet" href="css/bootstrap.min2.css" type="text/css">
<script type="text/javascript">
function checkForm() {
var content = document.insertform.content.value;
if (content.length < 2) {
alert('내용은 최소 2자리 이상이어야 합니다.');
document.insertform.content.focus();
return false;
} else if (content.length > 300) {
alert('내용은 최대 300자리 이하이어야 합니다.');
document.insertform.content.focus();
return false;
}
var category = document.insertform.category.value;
if (category == "-") {
alert('카테고리를 선택해주세요.');
document.insertform.category.focus();
return false;
}
}
</script>
</head>
<body>
<div class="container col-sm-offset-<%=offset%> col-sm-<%=mywidth%>">
<div class="panel panel-primary">
<div class="card mb-3">
<table class="table table-hover">
<tr>
<th scope="row">${bean.no}</th>
<th>${bean.category}</th>
<th>${bean.title}</th>
<c:if test="${bean.user_no == sessionScope.loginfo.no}">
<th><a href="<%=FormNo%>myPage">${writer}</a></th>
</c:if>
<c:if test="${bean.user_no != sessionScope.loginfo.no}">
<th><a href="<%=FormNo%>neighborPage&writer=${writer}&user_no=${bean.user_no}">${writer}</a></th>
</c:if>
<th>${bean.date}</th>
<c:if test="${bean.user_no == sessionScope.loginfo.no}">
<td>
<a href="<%=FormNo%>qnaUpdate&no=${bean.no}&${requestScope.parameters}"> 수정 </a>
</td>
<td>
<a href="<%=FormNo%>qnaDelete&no=${bean.no}"> 삭제 </a>
</td>
</c:if>
</tr>
</table>
<div class="card-body">
<h5 class="card-title">${bean.content}</h5>
</div>
<ul class="list-group list-group-flush">
<li class="list-group-item">
<c:if test="${whologin != 0}">
<form name="insertform" action="<%=FormYes%>" method="post">
<input type="hidden" name="no" value="${bean.no}">
<input type="hidden" name="command" value="qcoInsert">
<input type="hidden" name="writer" value="${sessionScope.loginfo.no}">
작성자 : <input type="text" disabled="disabled" value="${sessionScope.loginfo.user_nickname}">
<textarea name="content" rows="3" cols="120" style="resize: none;" placeholder="댓글을 입력하세요."></textarea>
<button type="submit" onclick="return checkForm();">
댓글 등록
</button>
</form>
</c:if>
</li>
<c:forEach var="comment" items="${requestScope.colists}">
<li class="list-group-item">
<c:if test="${comment.user_no == sessionScope.loginfo.no}">
<th>작성자 : <a href="<%=FormNo%>myPage">${comment.writer}</a></th>
</c:if>
<c:if test="${comment.user_no != sessionScope.loginfo.no}">
<th>작성자 : <a href="<%=FormNo%>neighborPage&writer=${comment.writer}">${comment.writer}</a></th>
</c:if>
- 등록일자 ${comment.date}
<c:if test="${comment.user_no == sessionScope.loginfo.no}">
<td>
<a href="<%=FormNo%>qcoUpdate&comm_no=${comment.qna_no}&${requestScope.parameters}"> 수정 </a>
</td>
<td>
<a href="<%=FormNo%>qcoDelete&comm_no=${comment.qna_no}&no=${bean.no}"> 삭제 </a>
</td>
</c:if>
<br>
내용 : ${comment.content}
</li>
</c:forEach>
</ul>
</div>
</div>
<div class="col-sm-offset-5 col-sm-4" align="left">
<button class="btn btn-primary" onclick="history.back();" >
목록보기
</button>
</div>
</div>
</body>
</html>
- QnADetailViewController.java
package com.veranda.qna.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.veranda.common.controller.SuperClass;
import com.veranda.qna.dao.QnADao;
import com.veranda.qna.vo.QnA;
import com.veranda.qnacomment.controller.QnACommentListController;
public class QnADetailViewController extends SuperClass {
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
super.doGet(request, response);
int no = Integer.parseInt(request.getParameter("no"));
String writer = null;
QnADao dao = new QnADao();
QnA bean = dao.SelectDataByPk(no);
writer = dao.SelectWriter(no);
// 게시글 클릭 시 댓글도 같이 나오게 한다.
new QnACommentListController().doGet(request, response);
request.setAttribute("bean", bean);
request.setAttribute("writer", writer);
String gotopage = "/qna/qnaDetailView.jsp";
super.GotoPage(gotopage);
}
}
- QnADao.java
public class QnADao extends SuperDao {
public QnA SelectDataByPk(int no) {
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = " select * from qnas";
sql += " where qna_no = ?";
QnA bean = null;
try {
if (this.conn == null) {
this.conn = this.getConnection();
}
pstmt = this.conn.prepareStatement(sql);
pstmt.setInt(1, no);
rs = pstmt.executeQuery();
if (rs.next()) {
bean = new QnA();
bean.setNo(rs.getInt("qna_no"));
bean.setUser_no(rs.getInt("user_no"));
bean.setTitle(rs.getString("qna_title"));
bean.setContent(rs.getString("qna_content"));
bean.setCategory(rs.getString("qna_category"));
bean.setDate(String.valueOf(rs.getDate("qna_date")));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
this.closeConnection();
} catch (Exception e2) {
e2.printStackTrace();
}
}
return bean;
}
public String SelectWriter(int no) {
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = " select user_nickname ";
sql += " from members m inner join qnas q ";
sql += " on m.user_no = q.user_no ";
sql += " where q.qna_no = ?";
String writer = null;
try {
if (conn == null) {
super.conn = super.getConnection();
}
pstmt = super.conn.prepareStatement(sql);
pstmt.setInt(1, no);
rs = pstmt.executeQuery();
while (rs.next()) {
writer = rs.getString("user_nickname");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
super.closeConnection();
} catch (Exception e2) {
e2.printStackTrace();
}
}
return writer;
}
}