게시판 Update
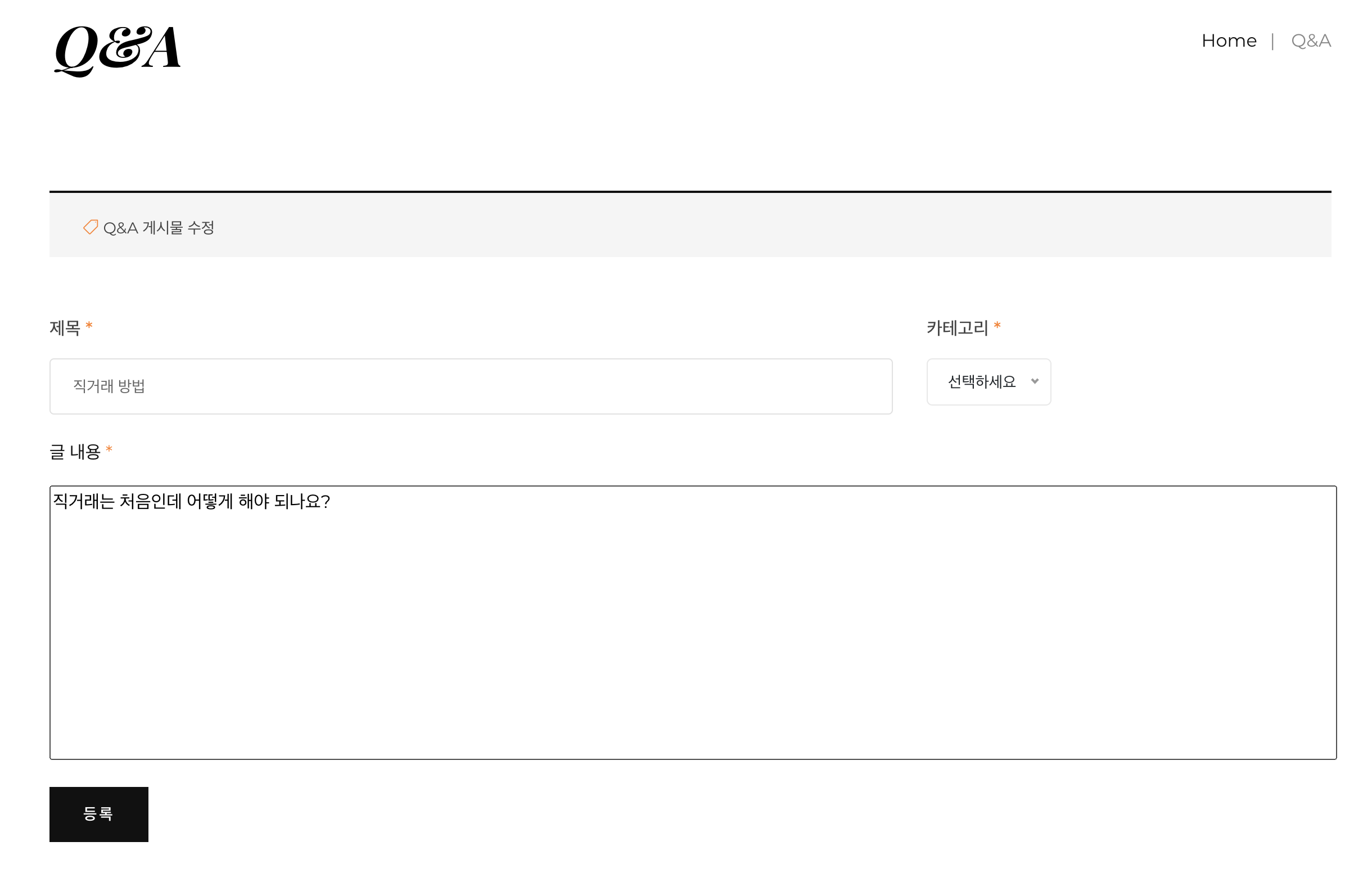
- qnaUpdate.jsp
/* position for grid system */
int offset = 2;
int mywidth = twelve - 2 * offset;
int formleft = 3;
int formright = twelve - formleft;
int rightButton = 2;
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="css/bootstrap.min2.css" type="text/css">
<script type="text/javascript">
function checkForm() {
var title = document.insertform.title.value;
if (title.length < 2) {
alert('제목은 최소 2자리 이상이어야 합니다.');
document.insertform.title.focus();
return false;
} else if (title.length > 20) {
alert('제목은 최소 20자리 이하이어야 합니다.');
document.insertform.title.focus();
return false;
}
var content = document.insertform.content.value;
if (content.length < 5) {
alert('내용은 최소 5자리 이상이어야 합니다.');
document.insertform.content.focus();
return false;
} else if (content.length > 1000) {
alert('내용은 최대 1000자리 이하이어야 합니다.');
document.insertform.content.focus();
return false;
}
var category = document.insertform.category.value;
if (category == "-") {
alert('카테고리를 선택해주세요.');
document.insertform.category.focus();
return false;
}
}
</script>
<style type="text/css">
.checkout__input_2 input{
height: 350px;
width: 100%;
border: 1px solid #e1e1e1;
font-size: 14px;
color: #666666;
padding-left: 20px;
margin-bottom: 20px;
}
.checkout__input_2 p span {
color: #f08632;
}
</style>
</head>
<body>
<div class="breadcrumb-option">
<div class="container">
<div class="row">
<div class="col-lg-6 col-md-6 col-sm-6">
<div class="breadcrumb__text">
<h2>
Q&A
</h2>
</div>
</div>
<div class="col-lg-6 col-md-6 col-sm-6">
<div class="breadcrumb__links">
<a href="<%=FormNo%>main">
Home
</a>
<span>
Q&A
</span>
</div>
</div>
</div>
</div>
</div>
<section class="checkout spad">
<div class="container">
<div class="checkout__form">
<form name="insertform" action="<%=FormYes%>" method="post">
<input type="hidden" name="command" value="qnaUpdate">
<input type="hidden" name="writer" value="${sessionScope.loginfo.no}">
<input type="hidden" name="no" value="${bean.no}">
<div class="row">
<div class="col-lg-12 col-md-6">
<h6 class="coupon__code">
<span class="icon_tag_alt"> </span>
Q&A 게시물 수정
</h6>
<div class="row">
<div class="col-lg-8">
<div class="checkout__input">
<p>
제목
<span>
*
</span>
</p>
<input type="text" class="form-control" id="title"
name="title" value="${bean.title}">
</div>
</div>
<div class="col-lg-4">
<div class="checkout__input">
<p>
카테고리
<span>
*
</span>
</p>
<div>
<select id="category" name="category" class="form">
<option value="-" selected="selected">선택하세요
<option value="불편사항">불편사항
<option value="이용문의">이용문의
</select>
</div>
</div>
</div>
</div>
<div class="input-div">
<div class="checkout__input_2">
<p>
글 내용
<span>
*
</span>
</p>
<textarea rows="10" cols="125" name="content" style="resize: none;">${bean.content}</textarea>
</div>
</div>
<br>
<button type="submit" class="site-btn" onclick="return checkForm();">
등록
</button>
</div>
</div>
</form>
</div>
</div>
</section>
</body>
</html>
- QnAUpdateController.java
package com.veranda.qna.controller;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.veranda.common.controller.SuperClass;
import com.veranda.qna.dao.QnADao;
import com.veranda.qna.vo.QnA;
public class QnAUpdateController extends SuperClass{
private QnA bean = null;
@Override
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
super.doGet(request, response);
int no = Integer.parseInt(request.getParameter("no"));
QnADao dao = new QnADao();
QnA bean = dao.SelectDataByPk(no);
request.setAttribute("bean", bean);
String gotopage = "/qna/qnaUpdate.jsp" ;
super.GotoPage(gotopage);
}
@Override
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
super.doPost(request, response);
bean = new QnA();
bean.setNo(Integer.parseInt(request.getParameter("no")));
bean.setTitle(request.getParameter("title"));
bean.setContent(request.getParameter("content"));
bean.setCategory(request.getParameter("category"));
System.out.println("bean.information");
System.out.println(toString());
if (this.validate(request) == true) {
System.out.println("qnas update validation check success");
QnADao dao = new QnADao();
int cnt = -1;
cnt = dao.UpdateData(bean);
new QnADetailViewController().doGet(request, response);
} else {
System.out.println("qnas update validation check failure");
request.setAttribute("bean", bean);
String gotopage = "/qna/qnaUpdate.jsp";
super.GotoPage(gotopage);
}
}
@Override
public boolean validate(HttpServletRequest request) {
boolean isCheck = true;
if (bean.getTitle().length() < 3 || bean.getTitle().length() > 10) {
request.setAttribute(super.PREFIX + "title", "제목은 3글자 이상 10자리 이하이어야 합니다.");
isCheck = false;
}
if (bean.getContent().length() < 5 || bean.getContent().length() > 1000) {
request.setAttribute(super.PREFIX + "content", "글내용은 5자리 이상 1000자리 이하이어야 합니다.");
isCheck = false;
}
return isCheck;
}
}
- QnADao.java
public class QnADao extends SuperDao {
public QnA SelectDataByPk(int no) {
PreparedStatement pstmt = null;
ResultSet rs = null;
String sql = " select * from qnas";
sql += " where qna_no = ?";
QnA bean = null;
try {
if (this.conn == null) {
this.conn = this.getConnection();
}
pstmt = this.conn.prepareStatement(sql);
pstmt.setInt(1, no);
rs = pstmt.executeQuery();
if (rs.next()) {
bean = new QnA();
bean.setNo(rs.getInt("qna_no"));
bean.setUser_no(rs.getInt("user_no"));
bean.setTitle(rs.getString("qna_title"));
bean.setContent(rs.getString("qna_content"));
bean.setCategory(rs.getString("qna_category"));
bean.setDate(String.valueOf(rs.getDate("qna_date")));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
this.closeConnection();
} catch (Exception e2) {
e2.printStackTrace();
}
}
return bean;
}
public int UpdateData(QnA bean) {
String sql = " update qnas set qna_title=?, qna_content=?, qna_category=? ";
sql += " where qna_no = ?";
PreparedStatement pstmt = null;
int cnt = -99999;
try {
if (conn == null) {
super.conn = super.getConnection();
}
conn.setAutoCommit(false);
pstmt = super.conn.prepareStatement(sql);
pstmt.setString(1, bean.getTitle());
pstmt.setString(2, bean.getContent());
pstmt.setString(3, bean.getCategory());
pstmt.setInt(4, bean.getNo());
cnt = pstmt.executeUpdate();
conn.commit();
} catch (Exception e) {
SQLException err = (SQLException) e;
cnt = -err.getErrorCode();
e.printStackTrace();
try {
conn.rollback();
} catch (Exception e2) {
e2.printStackTrace();
}
} finally {
try {
if (pstmt != null) {
pstmt.close();
}
super.closeConnection();
} catch (Exception e2) {
e2.printStackTrace();
}
}
return cnt;
}
}