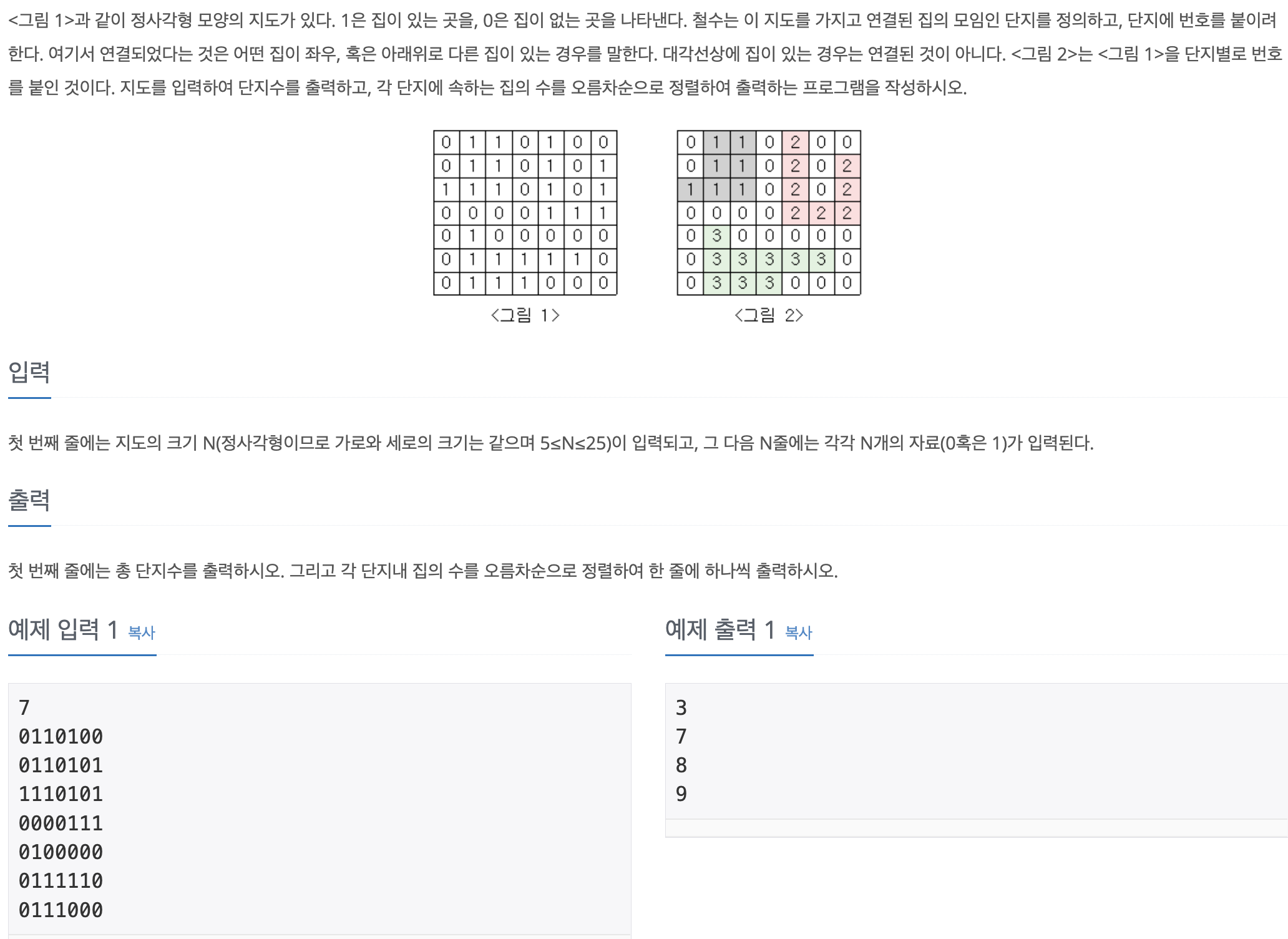
👩🏻🏫 DFS 풀이
import sys
sys.setrecursionlimit(10000)
n = int(sys.stdin.readline())
graph = [[] for i in range(n)]
for i in range(n):
graph[i] = list(map(int,sys.stdin.readline().rstrip()))
apt = []
dx = [-1,1,0,0]
dy = [0,0,-1,1]
def dfs(x,y):
global cnt
graph[x][y] = 0
cnt += 1
for i in range(4):
nx = x + dx[i]
ny = y + dy[i]
if 0 <= nx < n and 0 <= ny < n:
if graph[nx][ny] == 1:
dfs(nx,ny)
for i in range(n):
for j in range(n):
if graph[i][j] == 1:
cnt = 0
dfs(i, j)
apt.append(cnt)
print(len(apt))
apt.sort()
for i in apt:
print(i)
👩🏻🏫 BFS 풀이
import sys
from collections import deque
n = int(sys.stdin.readline())
graph = [sys.stdin.readline() for _ in range(n)]
visited = [[False]*n for _ in range(n)]
apt = []
def bfs(x,y):
if graph[x][y]=='0' or visited[x][y]:
return
dx = [0,0,-1,1]
dy = [-1,1,0,0]
q = deque([(x,y)])
visited[x][y] = True
cnt = 0
while q:
x,y = q.popleft()
cnt += 1
for i in range(4):
nx = x + dx[i]
ny = y + dy[i]
if 0 <= nx < n and 0 <= ny < n:
if graph[nx][ny] == '1' and not visited[nx][ny]:
q.append([nx,ny])
visited[nx][ny] = True
apt.append(cnt)
for i in range(n):
for j in range(n):
bfs(i,j)
print(len(apt))
for i in sorted(apt):
print(i)
✏️ Python 문법
global
- 함수 안에서 전역 변수의 값을 변경하려면
global
키워드를 사용해야 함
x = 10
def foo():
global x
x = 20
print(x)
foo()
print(x)