내용
작가 알림/소식
버튼을 클릭하면 해당 작가명의 소식을 알려준다는 알림창이 뜸
- 백엔드로 구독신청 했다는 알림을 보내줌
- 구독하면
소식받는 중
버튼으로 변경됨
소식받는 중
버튼을 한번 더 클릭하면 알림에서 삭제되었다는 알림과 함께 구독이 취소 됨
결과
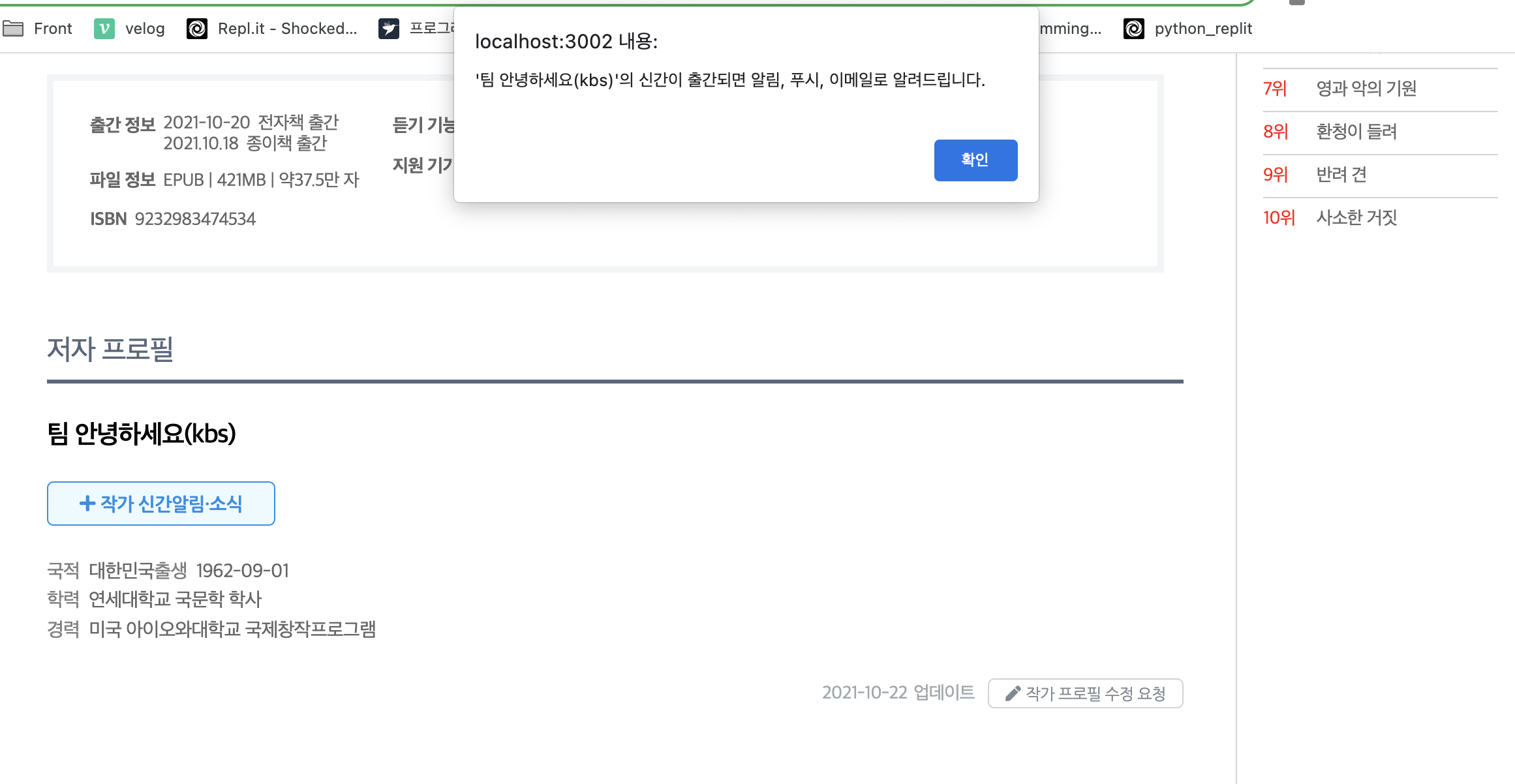
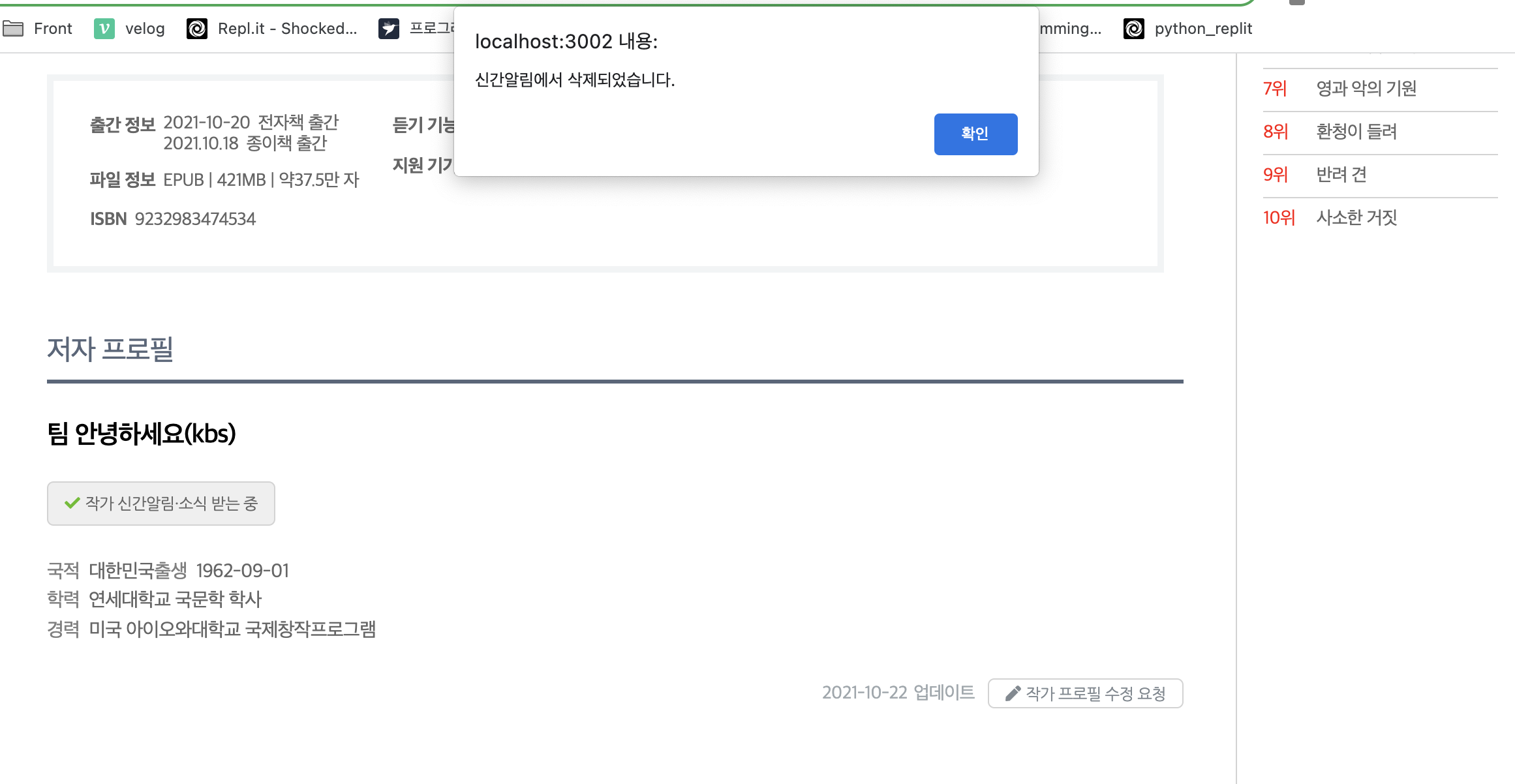
설명
- 소식 버튼을 누르면 onClick이벤트가 발생
- onSubscription 함수를 호출
- 이벤트를 인자로 받은 onSubscription 함수에서 setchangeButton로 changeButton를 true(반대로) 변경해줌
- if문에서 changeButton이 true로 변경되면서 alert가 실행됨
- 조건부 렌더링에서 changeButton가 true가 되면서
작가 신간알림/소식 받는 중
로직이 화면에 나타남
- 백엔드로 구독했다는 알림이 전달
- 한번 더 클릭이벤트가 발생한다
- changeButton이 false로 변경됨
- true가 아니기 때문에 if문의 else로 넘어가서 alert가 실행됨
- 조건부 렌더링에서 !changeButton가 false가 되면서
소식 받는 중
로직이 화면에 나타남
코드
import React, { useEffect, useState } from 'react';
import styled from 'styled-components';
import { Link } from 'react-router-dom';
const Profile = () => {
const [data, setData] = useState([]);
const [changeButton, setchangeButton] = useState(false);
useEffect(() => {
fetch('/data/DetailData.json')
.then(res => res.json())
.then(data => {
setData(data.product_detail[0].author_info[0]);
});
}, []);
const onSubscription = e => {
setchangeButton(!changeButton);
if (!changeButton) {
alert(
`'${data.name}'의 신간이 출간되면 알림, 푸시, 이메일로 알려드립니다.`
);
} else {
alert('신간알림에서 삭제되었습니다.');
}
};
return (
data && (
<div>
<ProfileBox>
<StyleLink to="/">{data.name}</StyleLink>
{}
<div>
{!changeButton && (
<BlueButtonHover onClick={onSubscription}>
<i className="fas fa-plus" />
작가 신간알림·소식
</BlueButtonHover>
)}
{changeButton && (
<HiddenButton onClick={onSubscription}>
<i className="fas fa-check" />
작가 신간알림·소식 받는 중
</HiddenButton>
)}
</div>
<ProfileListBox>
<li>
<span className="title">국적</span>
<span className="content">{data.country}</span>
<span className="title">출생</span>
<span className="content">{data.birthdate}</span>
</li>
{PROFILE_LIST.map((profile, index) => {
return (
<li key={index}>
<span className="title">{profile.title}</span>
<span className="content">{profile.content}</span>
</li>
);
})}
<UpdateBox>
<span>{data.info_update}</span>
<span>업데이트</span>
<button>
<i className="fas fa-pen" />
작가 프로필 수정 요청
</button>
</UpdateBox>
</ProfileListBox>
</ProfileBox>
</div>
)
);
};
export default Profile;
const PROFILE_LIST = [
{
title: '학력',
content: '연세대학교 국문학 학사',
},
{
title: '경력',
content: '미국 아이오와대학교 국제창작프로그램',
},
];
const StyleLink = styled(Link)``;
const ProfileBox = styled.div`
margin-top: 30px;
a {
color: black;
font-size: 20px;
font-weight: 600;
text-decoration: none;
span {
margin-left: 5px;
color: #666;
font-size: 14px;
}
}
button {
margin: 25px 0;
width: 175px;
height: 34px;
i {
margin-right: 4px;
}
}
`;
const ProfileListBox = styled.ul`
font-size: 15px;
line-height: 1.5em;
.title {
margin-right: 7px;
color: gray;
}
.content {
color: #666;
}
`;
const UpdateBox = styled.p`
float: right;
font-size: 14px;
span {
margin-right: 5px;
color: #9ea7ad;
}
button {
margin-left: 5px;
width: 150px;
height: 23px;
color: #808991;
border: 1px solid lightgray;
border-radius: 5px;
cursor: pointer;
background-color: white;
font-size: 12px;
&:hover {
background-color: #dcdcdc;
}
}
`;
const ToggleButton = styled.button`
background-color: white;
border-radius: 5px;
cursor: pointer;
`;
const BlueButtonHover = styled(ToggleButton)`
border: 1px solid #1f8ce6;
font-weight: 600;
color: #1f8ce6;
&:hover {
background-color: #ebfbff;
}
`;
const HiddenButton = styled(ToggleButton)`
border: 1px solid lightgray;
font-size: 12px;
color: #666;
.fa-check {
color: #5abf0d;
}
&:hover {
background-color: rgb(239, 239, 239);
}
`;