🍥구현 기능
- 메인 화면 UI 구현하기
![업로드중..]()
- 화면 상단:
- 하이커스 아이콘 ImageView
- 로그인한 회원 닉네임을 표시할 TextView
- 로그인한 회원 프로필 이미지 ImageView
- 화면 중앙:
- 게시글 10개를 표시할 ScrollView
- 각 게시글:
- 게시글 이미지 ImageView
- 게시글 제목 TextView
- 게시글 위치 정보 TextView
- 게시글 작성자 이름 TextView
- 게시글 작성 시간 TextView
- 게시글 좋아요 여부를 표시할 ImageView
- 화면 하단:
- 글쓰기 Floating Action Button
🍥구현하기
post_item.xml
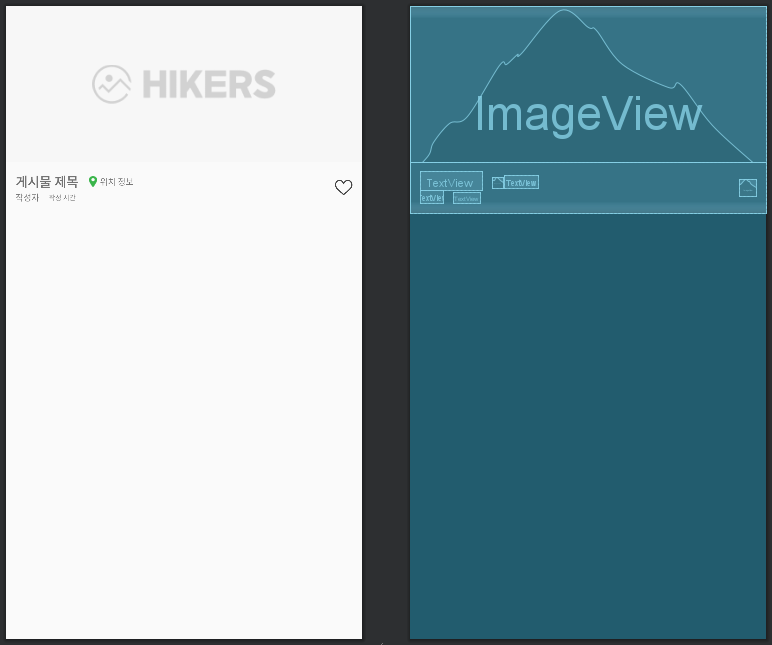
<merge xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools">
<LinearLayout
android:id="@+id/layout_post"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ImageView
android:id="@+id/iv_img"
android:transitionName="@string/trans_post_image"
android:layout_width="match_parent"
android:layout_height="180dp"
android:scaleType="center"
android:background="@color/light_gray"
android:src="@drawable/hikers_icon_small_grey" />
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="11dp">
<TextView
android:id="@+id/tv_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/post_title"
android:ellipsize="end"
android:maxWidth="180dp"
android:maxLines="1"
android:textSize="15sp"
android:textStyle="bold"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/iv_pin"
android:layout_width="12dp"
android:layout_height="12dp"
android:layout_marginStart="11dp"
android:src="@drawable/pin_icon"
app:layout_constraintBottom_toBottomOf="@id/tv_location"
app:layout_constraintStart_toEndOf="@id/tv_title"
app:layout_constraintTop_toTopOf="@id/tv_location" />
<TextView
android:id="@+id/tv_location"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="2dp"
android:text="@string/post_location"
android:textSize="10sp"
app:layout_constraintBottom_toBottomOf="@id/tv_title"
app:layout_constraintStart_toEndOf="@id/iv_pin"
app:layout_constraintTop_toTopOf="@id/tv_title"/>
<TextView
android:id="@+id/tv_writer_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/post_writer_name"
android:textSize="10sp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/tv_title"
app:layout_constraintBottom_toBottomOf="parent"
/>
<TextView
android:id="@+id/tv_time"
android:text="@string/post_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="11dp"
app:layout_constraintStart_toEndOf="@id/tv_writer_name"
app:layout_constraintTop_toBottomOf="@id/tv_title"
app:layout_constraintBottom_toBottomOf="parent"
android:textSize="8sp"
/>
<ImageView
android:id="@+id/iv_heart"
android:layout_width="20dp"
android:layout_height="20dp"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:src="@drawable/empty_heart_icon"
/>
</androidx.constraintlayout.widget.ConstraintLayout>
</LinearLayout>
</merge>
activity_main.xml
- 프로필 이미지를 표시할 ImageView를 원형으로 만들기 위해, CircularImageView 라이브러리를 사용했다.
- 게시물을 표시할 레이아웃(post_item_layout.xml)을 include 태그로 추가할 때,
id 속성을 include 태그 안에 정의하는 대신, include 태그를 감싸는 LinearLayout안에 정의했다.
- 표시할 최신 게시물이 없는 경우, 안내 메세지를 표시하기 위해
최신 게시글 목록을 표시하는 스크롤 뷰와 같은 위치에 TextView를 만들어두었다.
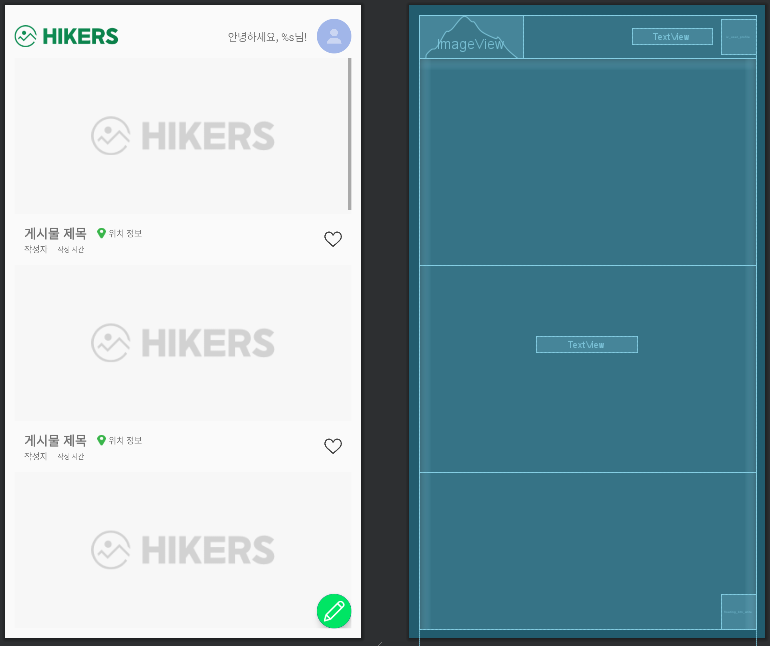
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="11dp"
tools:context=".MainActivity">
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/top_constraint_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="11dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<ImageView
android:layout_width="120dp"
android:layout_height="50dp"
android:src="@drawable/hikers_icon_small"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/tv_user_greeting"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginEnd="11dp"
android:text="@string/user_greeting"
android:textSize="12sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@id/iv_user_profile"
app:layout_constraintTop_toTopOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/iv_user_profile"
android:layout_width="40dp"
android:layout_height="40dp"
android:scaleType="centerCrop"
android:src="@drawable/default_profile"
android:transitionName="@string/trans_profile_image"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
<TextView
android:id="@+id/tv_no_post"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/no_post"
android:textSize="12sp"
android:visibility="gone"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/top_constraint_layout"
tools:visibility="visible" />
<ScrollView
android:id="@+id/scroll_view_post"
android:layout_width="match_parent"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/top_constraint_layout"
app:layout_constraintVertical_bias="1.0">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:id="@+id/post_item1"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item2"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item3"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item4"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item5"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item6"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item7"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item8"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item9"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<LinearLayout
android:id="@+id/post_item10"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<include layout="@layout/post_item_layout" />
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="80dp"/>
</LinearLayout>
</ScrollView>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/floating_btn_write"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:backgroundTint="@color/theme_green1"
android:src="@drawable/write_icon"
app:fabCustomSize="40dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>