<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel = "stylesheet" href = "../css/mystyle.css" type="text/css">
<style>
div{
border : 1px dashed orange;
}
</style>
<script>
function calc(op){
}
var calc = function(op){
xvalue = parseInt(document.getElementById("x").value);
yvalue = parseInt(document.getElementById("y").value);
switch(op){
case "+" :
res = xvalue + yvalue;
break;
case "-" :
res = xvalue - yvalue;
break;
case "*" :
res = xvalue * yvalue;
break;
case "/" :
res = xvalue / yvalue;
res = res.toFixed(2);
break;
}
str = xvalue + op +yvalue + "=" +res+"<br>"
document.getElementById('result1').innerHTML = str;
}
var vcalc = function(but){
xvalue = parseInt(document.getElementById("ix").value);
yvalue = parseInt(document.getElementById("iy").value);
switch(but.value){
case "+":
res = xvalue + yvalue;
break;
case "-":
res = xvalue - yvalue;
break;
case "*":
res = xvalue * yvalue;
break;
case "/":
res = xvalue / yvalue;
res = res.toFixed(2);
break;
}
str = xvalue + but.value + yvalue + "=" +res+"<br>"
document.getElementById('result2').innerHTML = str;
}
</script>
</head>
<body>
<pre>
각각의 버튼을 클릭할때 calc 함수 호출시 파라미터로 +,-,*,/를 전달한다.
onclick="calc('+')
onclick="calc('-')
onclick="calc('*')
onclick="calc('/')
</pre>
<form>
첫번째 수<input type="text" id="x"><br>
두번째 수<input type="text" id="y"><br>
<input type="button" value="+" onclick="calc('+')">
<input type="button" value="-" onclick="calc('-')">
<input type="button" value="*" onclick="calc('*')">
<input type="button" value="/" onclick="calc('/')">
</form>
<br>
<div id ="result1"></div>
<pre>
각각의 버튼을 클릭할때 vcalc 함수 호출
파라미터는 없고 대신 클릭하는 버튼의 객체자신인 this로 전달 하고
script에서 this에 해당하는 버튼의 value값을 비교하여 계산
onclick="vcalc(this)"
</pre>
<form>
첫번째 수<input type="text" id="ix"><br>
두번째 수<input type="text" id="iy"><br>
<input type="button" value="+" onclick="vcalc(this)">
<input type="button" value="-" onclick="vcalc(this)">
<input type="button" value="*" onclick="vcalc(this)">
<input type="button" value="/" onclick="vcalc(this)">
</form>
<br>
<div id ="result2"></div>
</body>
</html>
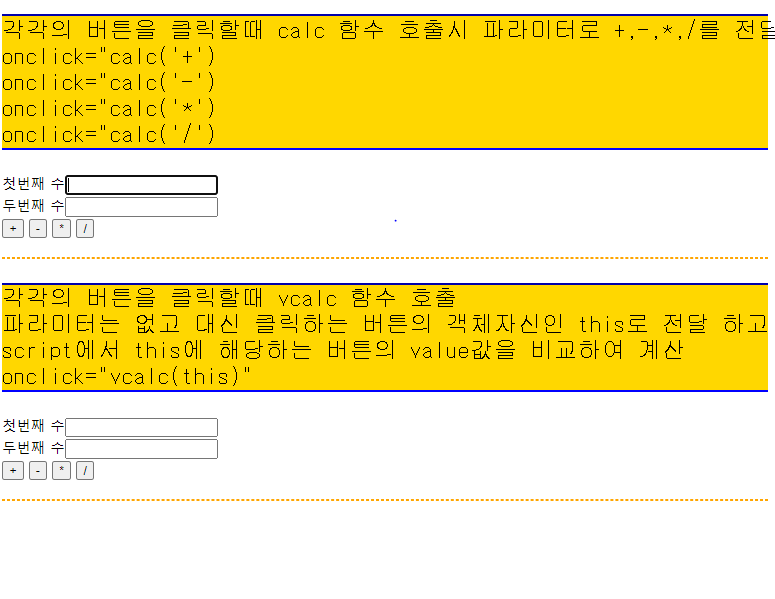