cus_SQL.xml
<select id="getTotal" resultType="int">
SELECT COUNT(*)
FROM CUS
</select>
list
<%@ page language="java" contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<link href="https://cdn.jsdelivr.net/npm/simple-datatables@latest/dist/style.css" rel="stylesheet">
<div class="row">
<div class="col">
<div class="card card-small mb-4">
<div class="card-header border-bottom">
<h6 class="m-0">Active Users</h6>
</div>
<div class="card-body p-0 pb-3 text-center">
<table class="table mb-0">
<thead class="bg-light">
<tr>
<th scope="col" class="border-0">번호</th>
<th scope="col" class="border-0">고객명</th>
<th scope="col" class="border-0">연락처</th>
<th scope="col" class="border-0">주소</th>
</tr>
</thead>
<tbody>
<c:forEach var="row" items="${list.content}">
<tr>
<td>${row.rnum}</td>
<td>${row.cusNm}</td>
<td>${row.pne}</td>
<td>${row.addr}</td>
</tr>
</c:forEach>
</tbody>
</table>
</div>
<div class="row">
<div class="col-sm-12 col-md-5">
<div class="dataTables_info" id="dataTable_info" role="status" aria-live="polite">
<c:if test="${list.currentPage*6 > total}">
Showing ${list.currentPage*6-5} to ${total} of ${total} entries
</c:if>
<c:if test="${list.currentPage*6 <= total}">
Showing ${list.currentPage*6-5} to ${list.currentPage*6} of ${total} entries
</c:if>
</div>
</div>
<div class="col-sm-12 col-md-7">
<div class="dataTables_paginate paging_simple_numbers" id="dataTable_paginate">
<ul class="pagination">
<li class="paginate_button page-item previous <c:if test='${list.startPage<6}'>disabled</c:if>" id="dataTable_previous"><a href="/cus/list?currentPage=${list.startPage-5}" aria-controls="dataTable" data-dt-idx="0" tabindex="0" class="page-link">Previous</a></li>
<c:forEach var="pNo" begin="${list.startPage}" end="${list.endPage}" step="1">
<li class="paginate_button page-item <c:if test='${list.currentPage eq pNo}'>active</c:if>"><a href="/cus/list?currentPage=${pNo}" aria-controls="dataTable" data-dt-idx="1" tabindex="0" class="page-link">${pNo}</a></li>
</c:forEach>
<li class="paginate_button page-item next <c:if test='${list.endPage>=list.totalPages}'>disabled</c:if>" id="dataTable_next"><a href="/cus/list?currentPage=${list.startPage+5}" aria-controls="dataTable" data-dt-idx="7" tabindex="0" class="page-link">Next</a></li>
</ul>
</div>
</div>
</div>
</div>
</div>
</div>
<a href="/cus/insert" class="mb-2 btn btn-sm btn-success mr-1"
style="float:right;">고객등록</a>
CusController
package kr.or.ddit.cus.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.validation.FieldError;
import org.springframework.validation.ObjectError;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import kr.or.ddit.cus.service.CusService;
import kr.or.ddit.cus.vo.CusVO;
import kr.or.ddit.util.ArticlePage;
import oracle.jdbc.proxy.annotation.GetProxy;
@RequestMapping("/cus")
@Controller
public class CusController {
private static final Logger logger =
LoggerFactory.getLogger(CusController.class);
@Autowired
CusService cusService;
//http://localhost/cus/list?currentPage=1
@RequestMapping(value="/list", method=RequestMethod.GET)
public String list(Model model
,@RequestParam(defaultValue="1", required=false) int currentPage) {
Map<String,String> pageHeader = new HashMap<String, String>();
pageHeader.put("subtitle", "Customer");
pageHeader.put("title", "고객 목록");
model.addAttribute("pageHeader", pageHeader);
//고객 전체 인원수
int total = this.cusService.getTotal();
//<key,value>
Map<String,Object> map = new HashMap<String, Object>();
// map.put("keyWord", keyWord);
map.put("currentPage", currentPage);
//고객 목록
List<CusVO> list = this.cusService.select(map);
model.addAttribute("list", new ArticlePage(total, currentPage, 6, 5, list));
model.addAttribute("total", total);
//forwarding
return "cus/list";
}
@GetMapping("/insert")
public String insert(Model model) {
Map<String,String> pageHeader = new HashMap<String, String>();
pageHeader.put("subtitle", "Customer");
pageHeader.put("title", "고객 등록");
model.addAttribute("pageHeader", pageHeader);
model.addAttribute("cusVO", new CusVO());
//forwarding
return "cus/insert";
}
@PostMapping("/insert")
public String insertPost(@Validated CusVO cusVO,
BindingResult result){
//검증 오류 발생
if(result.hasErrors()) {
List<ObjectError> allErrors = result.getAllErrors();
List<ObjectError> globalErrors = result.getGlobalErrors();
List<FieldError> fieldErrors = result.getFieldErrors();
//validation 중에 어떤 오류가 나왔는지 확인..
for(int i=0;i<allErrors.size();i++) {
ObjectError objectError = allErrors.get(i);
logger.info("objectError : " + objectError);
}
for(ObjectError objectError : globalErrors) {
logger.info("objectError : " + objectError);
}
for(FieldError fieldError : fieldErrors) {
logger.info("fieldError : " + fieldError.getDefaultMessage());
}
//redirect(X) => 데이터를 보낼 수 없음
//forwarding(o)
return "cus/insert";
}
//insert처리
logger.info("cusVO : " + cusVO.toString());
int insertResult = cusService.insert(cusVO);
if(insertResult>0) {//고객 등록 성공
//목록으로 이동
return "redirect:/cus/list";
}else {//고객 등록 실패
//등록 화면으로 forwarding
return "cus/insert";
}
}
}
CusService
//고객 전체 인원수
public int getTotal();
CusServiceImpl
//고객 전체 인원수
@Override
public int getTotal() {
return this.cusMapper.getTotal();
}
CusMapper
//고객 전체 인원수
public int getTotal();
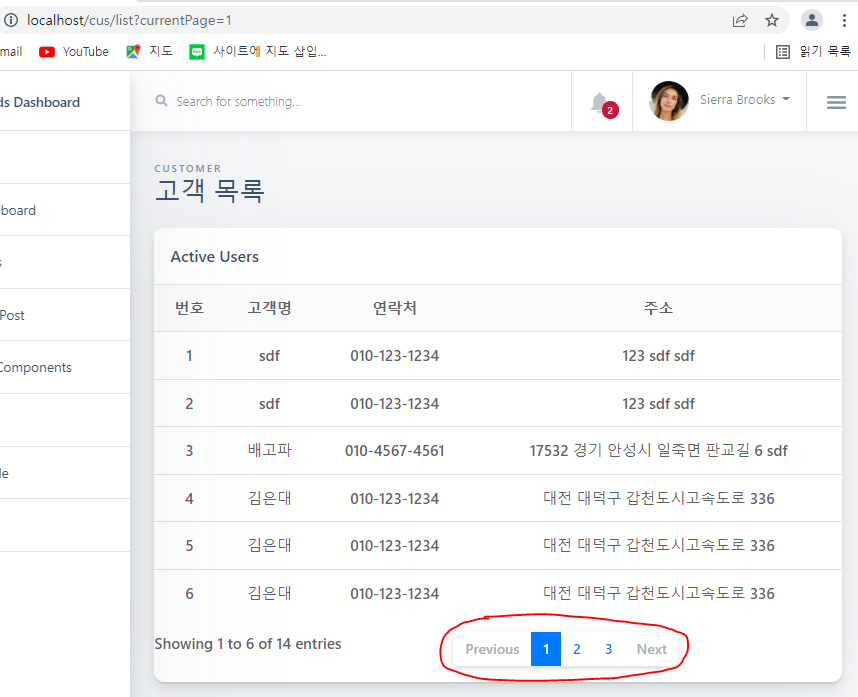